Software Engineer
1500+ Software Engineer Interview Questions and Answers for Freshers
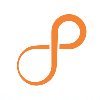
Asked in Persistent Systems

Q. Index of First Occurrence Problem Statement
Given two strings A
and B
, determine the index of the first occurrence of A
in B
. If A
is not present in B
, return -1.
Example:
Input:
A = "bc", B = "abcddbc"
Output:...read more
Find the index of the first occurrence of string A in string B.
Iterate through string B and check if a substring of length equal to A matches A.
Return the index of the first occurrence of A in B, or -1 if not found.
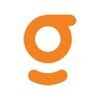
Asked in Grey Orange

Q. Print All Permutations of a String
Given an input string STR
, generate and print all possible permutations of the string.
Input:
str
Output:
All permutations of the input string, each on a new line.
Example:
In...read more
Generate and print all possible permutations of a given input string.
Use recursion to generate all permutations by swapping characters in the string.
Maintain a visited array to keep track of characters already used in a particular permutation.
Print each permutation as it is generated.
Handle duplicate characters by skipping swapping if the character is already in the current position.
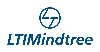
Asked in LTIMindtree

Q. Prime Numbers Identification
Given a positive integer N
, your task is to identify all prime numbers less than or equal to N
.
Explanation:
A prime number is a natural number greater than 1 that has no positive d...read more
Identify all prime numbers less than or equal to a given positive integer N.
Iterate from 2 to N and check if each number is prime
Use the Sieve of Eratosthenes algorithm for efficient prime number identification
Optimize by only checking up to the square root of N for divisors
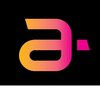
Asked in Amdocs

Q. Reverse Stack with Recursion
Reverse a given stack of integers using recursion. You must accomplish this without utilizing extra space beyond the internal stack space used by recursion. Additionally, you must r...read more
Reverse a given stack of integers using recursion without using extra space or loops.
Use recursion to pop all elements from the original stack and store them in function call stack
Once the stack is empty, push the elements back in reverse order using recursion
Use the top(), pop(), and push() methods to manipulate the stack
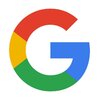
Asked in Google

Q. Sudoku Solver
Given a 9x9 Sudoku board, your task is to fill the empty slots and return the completed Sudoku solution.
A Sudoku is a grid composed of nine 3x3 smaller grids. The challenge is to fill in the numb...read more
Implement a Sudoku solver to fill empty slots in a 9x9 grid with numbers 1-9 satisfying constraints.
Create a recursive function to solve the Sudoku puzzle by trying out different numbers in empty slots.
Use backtracking to backtrack and try a different number if a conflict is encountered.
Ensure each number appears only once per row, column, and 3x3 grid.
Return the completed Sudoku grid as the output.
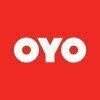
Asked in Oyo Rooms

Q. Water Jug Problem Statement
You have two water jugs with capacities X
and Y
liters respectively, both initially empty. You also have an infinite water supply. The goal is to determine if it is possible to measu...read more
The Water Jug Problem involves determining if a specific amount of water can be measured using two jugs of different capacities.
Start by considering the constraints and limitations of the problem.
Think about how the operations allowed can be used to reach the target measurement.
Consider different scenarios and test cases to come up with a solution.
Implement a function that takes the capacities of the jugs and the target measurement as input and returns True or False based on ...read more
Software Engineer Jobs
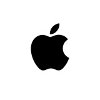
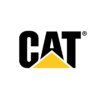
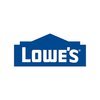
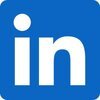
Asked in LinkedIn

Q. Generate All Parentheses Combinations
Given an integer N
, your task is to create all possible valid parentheses configurations that are well-formed using N
pairs. A sequence of parentheses is considered well-fo...read more
Generate all possible valid parentheses configurations with N pairs.
Use backtracking to generate all possible combinations of parentheses.
Keep track of the number of open and close parentheses used.
Add '(' if there are remaining open parentheses, and add ')' if there are remaining close parentheses.
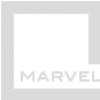
Asked in Marvel Realtors

Q. In a two-player game, players take turns placing coins on a round table without overlapping. The player who cannot place a coin loses. Assuming both players have an infinite supply of coins, and you go first, w...
read moreTo win the coin placement game, start by placing a coin in the center and mirror your opponent's moves.
Place your first coin in the center of the table.
After your opponent places a coin, identify the position relative to the center.
Reflect their move across the center point to maintain symmetry.
This strategy ensures that you always have a valid move until the table is full.
Share interview questions and help millions of jobseekers 🌟

Asked in UnitedHealth

Q. Bubble Sort Problem Statement
Sort the given unsorted array consisting of N non-negative integers in non-decreasing order using the Bubble Sort algorithm.
Input:
The first line contains an integer 'T' represent...read more
Bubble Sort is used to sort an array of non-negative integers in non-decreasing order.
Iterate through the array and compare adjacent elements, swapping them if they are in the wrong order.
Repeat this process until the array is sorted.
Time complexity of Bubble Sort is O(n^2) in worst case.
Space complexity of Bubble Sort is O(1) as it sorts the array in place.
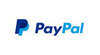
Asked in PayPal

Q. Maximum Difference Problem Statement
Given an array ARR
of N
elements, your task is to determine the maximum difference between any two elements in ARR
.
If the maximum difference is even, print EVEN
. If the max...read more
Find the maximum difference between any two elements in an array and determine if it is even or odd.
Iterate through the array to find the maximum and minimum elements.
Calculate the difference between the maximum and minimum elements.
Check if the difference is even or odd and return the result.
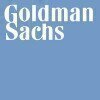
Asked in Goldman Sachs

On an Island, there is an airport that has an unlimited number of identical air-planes. Each air-plane has a fuel capacity to allow it to fly exactly 1/2 way around the world, along a great circle. The pl...read more
Minimum number of airplanes needed to get one airplane all the way around the world and return safely to the airport.
To achieve this, we need 3 airplanes in total.
The first airplane flies halfway around the world, then transfers half of its fuel to the second airplane.
The first airplane then returns to the airport, while the second airplane continues to fly the remaining half of the journey.
When the second airplane reaches the destination, it transfers all its fuel to the thi...read more
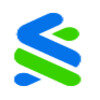
Asked in Standard Chartered

Q. Ways To Make Coin Change
Given an infinite supply of coins of varying denominations, determine the total number of ways to make change for a specified value using these coins. If it's not possible to make the c...read more
The task is to determine the total number of ways to make change for a specified value using given denominations.
Create a dynamic programming table to store the number of ways to make change for each value up to the target value.
Iterate through each denomination and update the table accordingly based on the current denomination.
The final value in the table will represent the total number of ways to make change for the target value.
Consider edge cases such as when the target v...read more
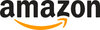
Asked in Amazon

Q. Minimum Number of Platforms Needed Problem Statement
You are given the arrival and departure times of N trains at a railway station for a particular day. Your task is to determine the minimum number of platform...read more
The task is to determine the minimum number of platforms needed at a railway station based on arrival and departure times of trains.
Sort the arrival and departure times in ascending order.
Use two pointers to track overlapping schedules.
Increment platform count when a new train arrives before the previous one departs.
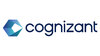
Asked in Cognizant

Q. Nth Fibonacci Number Problem Statement
Calculate the Nth term in the Fibonacci sequence, where the sequence is defined as follows: F(n) = F(n-1) + F(n-2)
, with initial conditions F(1) = F(2) = 1
.
Input:
The inp...read more
Calculate the Nth Fibonacci number efficiently using dynamic programming.
Use dynamic programming to store previously calculated Fibonacci numbers to avoid redundant calculations.
Start with base cases F(1) and F(2) as 1, then iterate to calculate F(N) efficiently.
Time complexity can be optimized to O(N) using dynamic programming.
Example: For N = 5, the 5th Fibonacci number is 5.
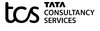
Asked in TCS

Q. Write a program for Fibonacci series for n terms where n is the user input.
Program for Fibonacci series for n terms with user input.
Take user input for n
Initialize variables for first two terms of Fibonacci series
Use a loop to generate the series up to n terms
Print the series
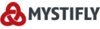
Asked in Mystifly Consulting

Q. On a dark night, 4 people want to cross a bridge and they have only one torch. At a time at max 2 people can cross the bridge. The time required for each individual to cross the bridge is 1, 2, 5, and 10 minute...
read more4 people with different crossing times need to cross a bridge with one torch. Only 2 can cross at a time. What's the minimum time?
The person with the longest time should always be accompanied by someone else
The two fastest people should cross together to save time
The torch needs to be brought back to the starting side every time two people cross
The total time taken will be the sum of all individual crossing times
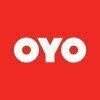
Asked in Oyo Rooms

Q. Boolean Matrix Transformation Challenge
Given a 2-dimensional boolean matrix mat
of size N x M, your task is to modify the matrix such that if any element is 1, set its entire row and column to 1. Specifically,...read more
Modify a boolean matrix such that if any element is 1, set its entire row and column to 1.
Iterate through the matrix and mark rows and columns to be modified
Use additional arrays to keep track of rows and columns to be modified
Update the matrix in-place based on the marked rows and columns
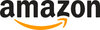
Asked in Amazon

Q. Left View of a Binary Tree Problem Statement
Given a binary tree, your task is to print the left view of the tree.
Example:
Input:
The input will be in level order form, with node values separated by a space. U...read more
Print the left view of a binary tree given in level order form.
Traverse the tree level by level and print the first node of each level (leftmost node).
Use a queue to keep track of nodes at each level.
Time complexity should be O(n) where n is the number of nodes in the tree.
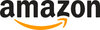
Asked in Amazon

Q. Missing Number Problem Statement
You are provided with an array named BINARYNUMS
consisting of N
unique strings. Each string represents an integer in binary, covering every integer from 0 to N except for one. Y...read more
Identify the missing integer in an array of binary strings and return its binary representation without leading zeros.
Iterate through the binary strings to convert them to integers and find the missing number using the formula for sum of integers from 0 to N.
Calculate the sum of all integers from 0 to N using the formula (N*(N+1))/2 and subtract the sum of the integers in the array to find the missing number.
Convert the missing integer to binary representation and return it a...read more
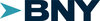
Asked in BNY

Q. Cycle Detection in a Singly Linked List
Determine if a given singly linked list of integers forms a cycle or not.
A cycle in a linked list occurs when a node's next
points back to a previous node in the list. T...read more
Detect if a singly linked list forms a cycle by checking if a node's next points back to a previous node.
Traverse the linked list using two pointers, one moving one step at a time and the other moving two steps at a time.
If the two pointers meet at any point, it indicates the presence of a cycle in the linked list.
If one of the pointers reaches the end of the list (null), it means there is no cycle.
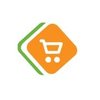
Asked in Paxcom India

Q. Maximize Expression Value
Given an arithmetic expression EXP
containing integer values separated by any of the operators ‘+’, ‘-’, and ‘*’, your task is to place parentheses such that the value of the expressio...read more
Given an arithmetic expression, place parentheses to maximize the value of the expression.
Identify the operators in the expression ('+', '-', '*').
Consider the precedence of operators to determine where to place parentheses.
Calculate the value of the expression with different placements of parentheses to find the maximum value.
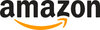
Asked in Amazon

Q. Reverse a Singly Linked List
Given a singly linked list of integers, your task is to return the head of the reversed linked list.
Explanation:
Reverse a given singly linked list so that the last element becomes...read more
Reverse a singly linked list of integers.
Iterate through the linked list and reverse the pointers to point to the previous node instead of the next node.
Keep track of the current, previous, and next nodes while traversing the list.
Update the head of the linked list to be the last node encountered during traversal.
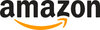
Asked in Amazon

Q. Sum Between Zeroes Problem Statement
Given a singly linked list containing a series of integers separated by the integer '0', modify the list by merging nodes between two '0's into a single node. This merged no...read more
Merge nodes between zeroes in a linked list to store the sum of included nodes.
Traverse the linked list and keep track of sum between zeroes
Merge nodes between zeroes by updating the sum in the merged node
Handle edge cases like empty list or single node between zeroes
Ensure the list starts and ends with a zero
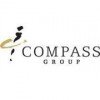
Asked in Compass Group Support Services

Q. Sum Of Zeroes Coverage Calculation
You are provided with a binary matrix containing dimensions 'N * M', comprised only of 0s and 1s. Your task is to compute the aggregated sum of coverages for all the zeros in ...read more
Calculate the total coverage of zeros in a binary matrix based on adjacent ones in a defined neighborhood.
Matrix Traversal: Iterate through each cell in the N x M matrix to identify zeros.
Neighbor Counting: For each zero, check its top, bottom, left, and right neighbors to count the number of adjacent ones.
Boundary Conditions: Ensure that checks for neighbors do not go out of matrix bounds to avoid errors.
Example: In a 3x3 matrix with zeros at (0,0), (0,2), and (1,1), the cov...read more
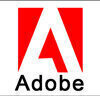
Asked in Adobe

Q. Wildcard Pattern Matching Problem Statement
Implement a wildcard pattern matching algorithm to determine if a given wildcard pattern matches a text string completely.
The wildcard pattern may include the charac...read more
The task is to implement a wildcard pattern matching algorithm that checks if a given wildcard pattern matches a given text.
The wildcard pattern can include the characters '?' and '*'
'?' matches any single character
'*' matches any sequence of characters (sequence can be of length 0 or more)
The matching should cover the entire text, not partial text
Implement a function that takes the wildcard pattern and the text as input and returns True if the text matches the pattern, False...read more
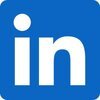
Asked in LinkedIn

Q. Check if Two Trees are Mirror
Given two arbitrary binary trees, your task is to determine whether these two trees are mirrors of each other.
Explanation:
Two trees are considered mirror of each other if:
- The r...read more
Check if two binary trees are mirrors of each other based on specific criteria.
Compare the roots of both trees.
Check if the left subtree of the first tree is the mirror of the right subtree of the second tree.
Verify if the right subtree of the first tree is the mirror of the left subtree of the second tree.
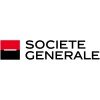

Q. Cube of Matrix Problem Statement
Given a 2D array 'MATRIX' of size M x N, find and return the value (i * i + j * j) for those elements where the sum of the cubes of its digits equals the element itself. Here, '...read more
Given a 2D array, find elements where sum of cube of digits equals element itself and return (i * i + j * j) value.
Iterate through the 2D array and check if the sum of cube of digits equals the element itself.
Calculate (i * i + j * j) for such elements and return the values.
If no such element exists, return -1.
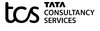
Asked in TCS

Q. Matrix Multiplication Task
Given two sparse matrices MAT1
and MAT2
of integers with dimensions 'N' x 'M' and 'M' x 'P' respectively, the goal is to determine the resulting matrix produced by their multiplicatio...read more
Implement a function to multiply two sparse matrices and return the resulting matrix.
Create a function that takes two sparse matrices as input and returns the resulting matrix after multiplication.
Iterate through the non-zero elements of the matrices to perform the multiplication efficiently.
Ensure to handle the sparse nature of the matrices to optimize the multiplication process.
Consider the constraints provided to ensure the function works within the specified limits.
Test t...read more
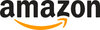
Asked in Amazon

Q. Maximum Path Sum in a Matrix
Given an N*M matrix filled with integer numbers, determine the maximum sum that can be obtained from a path starting from any cell in the first row to any cell in the last row.
You ...read more
Find the maximum sum path in a matrix from top row to bottom row by moving down or diagonally.
Use dynamic programming to keep track of maximum sum at each cell.
At each cell, consider the maximum sum from the cell above, left diagonal, and right diagonal.
Add the current cell value to the maximum of the three possibilities.
Repeat this process for all cells in the matrix to find the maximum sum path.
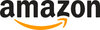
Asked in Amazon

Q. Sort Linked List Based on Actual Values
Given a Singly Linked List of integers that are sorted based on their absolute values, the task is to sort the linked list based on the actual values.
The absolute value ...read more
Sort a Singly Linked List based on actual values instead of absolute values.
Traverse the linked list and store the values in an array.
Sort the array based on actual values.
Update the linked list with the sorted values.
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
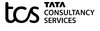
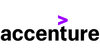
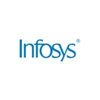
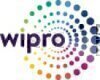
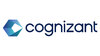
Top Interview Questions for Software Engineer Related Skills
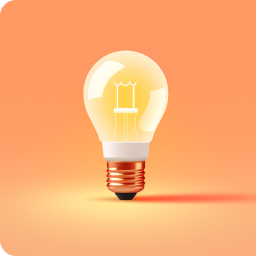
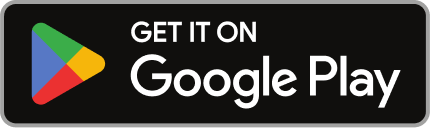
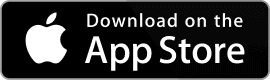
Reviews
Interviews
Salaries
Users
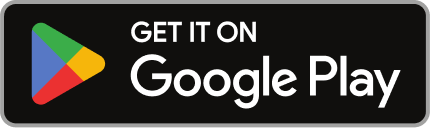
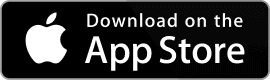