Software Engineer
8000+ Software Engineer Interview Questions and Answers
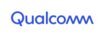
Asked in Qualcomm

Q. Four people need to cross a bridge at night with only one torch that can only illuminate two people at a time. Person A takes 1 minute, B takes 2 minutes, C takes 7 minutes, and D takes 10 minutes to cross. Whe...
read moreFour people with different crossing times need to cross a bridge with a torch. Only two can cross at a time. What is the least time taken?
Person A and B cross first, taking 2 minutes
A goes back with the torch, taking 1 minute
C and D cross together, taking 10 minutes
B goes back with the torch, taking 2 minutes
A and B cross together, taking 2 minutes
Total time taken is 17 minutes
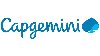
Asked in Capgemini

Q. In a dark room, there is a box of 18 white and 5 black gloves. You are allowed to pick one, keep it, and check it outside. How many turns do you need to take to find a perfect pair?
You need to take 36 turns to find a perfect pair.
You need to pick 19 gloves to ensure a perfect pair.
The worst case scenario is picking 18 white gloves and then the 19th glove is black.
In that case, you need to pick 17 more gloves to find a black one and complete the pair.
Software Engineer Interview Questions and Answers for Freshers
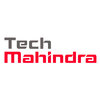
Asked in Tech Mahindra

Q. Tell me something about yourself. Define encapsulation. What is inheritance. What is constructor. What is method overloading. Can we overload a constructor. What is overriding. Difference between overloading an...
read moreThe interview questions cover topics like encapsulation, inheritance, constructors, method overloading, overriding, exception handling, and hobbies.
Encapsulation is the process of hiding the internal details of an object and providing access through methods.
Inheritance is a mechanism in which one class inherits the properties and behaviors of another class.
A constructor is a special method used to initialize objects.
Method overloading is the ability to define multiple methods...read more
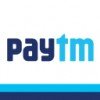
Asked in Paytm

Q. Puzzle : 100 people are standing in a circle .each one is allowed to shoot a person infront of him and he hands the gun to the next to next person for e.g 1st person kills 2nd and hands gun to 3rd .This continu...
read moreA puzzle where 100 people shoot the person in front of them until only one person remains.
Each person shoots the person in front of them and hands the gun to the next to next person.
This continues until only one person remains.
The last person standing is the one who was not shot by anyone.
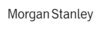
Asked in Morgan Stanley

Q. Find the Duplicate Number Problem Statement
Given an integer array 'ARR' of size 'N' containing numbers from 0 to (N - 2). Each number appears at least once, and there is one number that appears twice. Your tas...read more
Given an array of size N containing numbers from 0 to (N-2), find and return the duplicate number.
Iterate through the array and keep track of the frequency of each number using a hashmap.
Return the number with a frequency of 2.
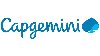
Asked in Capgemini

Q. How can you cut a rectangular cake in 8 symmetric pieces in three cuts?
Cut the cake in half horizontally, then vertically, and finally cut each half diagonally.
Cut the cake horizontally through the middle.
Cut the cake vertically through the middle.
Cut each half diagonally from corner to corner.
Ensure each cut is made symmetrically to get 8 equal pieces.
Example: https://www.youtube.com/watch?v=ZdGmuCJzQFo
Software Engineer Jobs



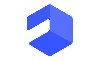
Asked in AmbitionBox

Q. What is the difference between a stack and a queue? Give an example where you would use each.
A stack is a LIFO data structure while a queue is a FIFO data structure.
Stack: Last In First Out (LIFO), used in undo-redo functionality, backtracking, and recursion.
Queue: First In First Out (FIFO), used in scheduling, buffering, and breadth-first search.
Example: A stack can be used to store the history of visited web pages while a queue can be used to manage incoming requests in a web server.

Asked in JPMorgan Chase & Co.

Q. Split Binary String Problem Statement
Chintu has a long binary string str
. A binary string is a string that contains only 0 and 1. He considers a string to be 'beautiful' if and only if the number of 0's and 1'...read more
The task is to split a binary string into beautiful substrings with equal number of 0's and 1's.
Count the number of 0's and 1's in the string.
Iterate through the string and split it into beautiful substrings whenever the count of 0's and 1's becomes equal.
Return the maximum number of beautiful substrings that can be formed.
If it is not possible to split the string into beautiful substrings, return -1.
Share interview questions and help millions of jobseekers 🌟
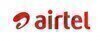
Asked in Bharti Airtel

Q. N-th Fibonacci Number Problem Statement
Given an integer ‘N’, your task is to find and return the N’th Fibonacci number using matrix exponentiation.
Since the answer can be very large, return the answer modulo ...read more
The task is to find the Nth Fibonacci number using matrix exponentiation and return the answer modulo 10^9 + 7.
Implement a function to find the Nth Fibonacci number using matrix exponentiation.
Return the answer modulo 10^9 + 7 to handle large values.
Use the formula F(n) = F(n-1) + F(n-2) with initial values F(1) = F(2) = 1.
Optimize the solution to achieve better than O(N) time complexity.
Consider solving the problem using dynamic programming or matrix exponentiation technique...read more
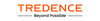
Asked in Tredence

Q. Reverse String Operations Problem Statement
You are provided with a string S
and an array of integers A
of size M
. Your task is to perform M
operations on the string as specified by the indices in array A
.
The ...read more
Given a string and an array of indices, reverse substrings based on the indices to obtain the final string.
Iterate through the array of indices and reverse the substrings accordingly
Ensure the range specified by each index is non-empty
Return the final string after all operations are completed
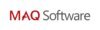
Asked in MAQ Software

Q. Factorial Calculation Problem
Given an integer N
, calculate and print the factorial of N
. The factorial of a number N
is defined as the product of all positive integers up to N
.
Example:
Input:
N = 4
Output:
24...read more
Calculate and print the factorial of a given integer N.
Iterate from 1 to N and multiply each number to calculate factorial.
Handle edge cases like N=0 or N=1 separately.
Use recursion to calculate factorial efficiently.
Consider using dynamic programming to optimize factorial calculation.
Ensure to handle large values of N to prevent overflow issues.
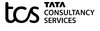
Asked in TCS

Q. What is the reason that the Iterative Waterfall model was introduced?
Iterative Waterfall model was introduced to address the limitations of the traditional Waterfall model.
Iterative Waterfall model allows for feedback and changes during the development process.
It breaks down the development process into smaller, more manageable stages.
It reduces the risk of project failure by identifying and addressing issues early on.
It allows for better collaboration between developers and stakeholders.
Examples include Rational Unified Process (RUP) and Agil...read more
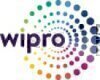
Asked in Wipro

Q. Knapsack Problem Statement
There is a potter with a limited amount of pottery clay (denoted as 'K' units) who can make 'N' different items. Each item requires a specific amount of clay and yields a certain prof...read more
The Knapsack Problem involves maximizing profit by choosing which items to make with limited resources.
Understand the problem statement and constraints provided.
Implement a dynamic programming solution to solve the Knapsack Problem efficiently.
Iterate through the items and clay units to calculate the maximum profit that can be achieved.
Consider both the clay requirement and profit of each item while making decisions.
Ensure the solution runs within the given time limit of 1 se...read more
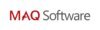
Asked in MAQ Software

Q. Nth Element Of Modified Fibonacci Series
Given two integers X
and Y
as the first two numbers of a series, and an integer N
, determine the Nth element of the series following the Fibonacci rule: f(x) = f(x - 1) ...read more
Calculate the Nth element of a modified Fibonacci series given the first two numbers and N, with the result modulo 10^9 + 7.
Implement a function to calculate the Nth element of the series using the Fibonacci rule f(x) = f(x - 1) + f(x - 2)
Return the answer modulo 10^9 + 7 due to the possibility of a very large result
The series starts with the first two numbers X and Y, and the position N in the series
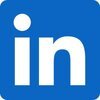
Asked in LinkedIn

Q. Split the String Problem Statement
You are given a string str
consisting of N
lowercase alphabets. Your task is to determine if it is possible to divide the string into three non-empty substrings such that one ...read more
Determine if it is possible to divide a string into three non-empty substrings where one is a substring of the other two.
Check if any substring of the string is a substring of the other two substrings.
Iterate through all possible divisions of the string into three non-empty substrings.
Use two pointers to find all possible substrings efficiently.
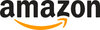
Asked in Amazon

Q. Find All Pairs Adding Up to Target
Given an array of integers ARR
of length N
and an integer Target
, your task is to return all pairs of elements such that they add up to the Target
.
Input:
The first line conta...read more
Find all pairs of elements in an array that add up to a given target.
Iterate through the array and use a hashmap to store the difference between the target and current element.
Check if the current element exists in the hashmap, if so, print the pair.
If no pair is found, print (-1, -1).
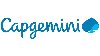
Asked in Capgemini

Q. One question of sorting for a list of people belonging to different cities and states.
Sort a list of people by their cities and states.
Use a sorting algorithm like quicksort or mergesort.
Create a custom comparator function that compares the city and state of each person.
If two people belong to the same city and state, sort them by their names.
Example: [{name: 'John', city: 'New York', state: 'NY'}, {name: 'Jane', city: 'Boston', state: 'MA'}]
Example output: [{name: 'Jane', city: 'Boston', state: 'MA'}, {name: 'John', city: 'New York', state: 'NY'}]
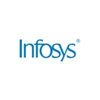
Asked in Infosys

Q. String Compression Problem Statement
Ninja needs to perform basic string compression. For any character that repeats consecutively more than once, replace the repeated sequence with the character followed by th...read more
Implement a function to compress a string by replacing consecutive characters with the character followed by the count of repetitions.
Iterate through the input string and keep track of consecutive characters and their counts
Replace consecutive characters with the character followed by the count of repetitions if count is greater than 1
Return the compressed string
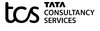
Asked in TCS

Q. Can you describe a challenging technical problem you faced and how you solved it?
Developing a real-time chat application with high scalability and low latency.
Designing a distributed architecture to handle a large number of concurrent users.
Implementing efficient data synchronization between multiple servers.
Optimizing network communication to minimize latency.
Ensuring data consistency and reliability in a distributed environment.
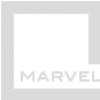
Asked in Marvel Realtors

Q. Given two queues, q1 and q2, where a background process copies elements from q1 to q2, write an error handling function to copy elements from q2 back to q1 if an error occurs. Use the following functions: q_len...
read moreThe error handling function copies elements from q2 back to q1 in case of an error.
Use q_len() to get the length of the queues
Use q_insert() and q_remove() to manipulate the queues
Iterate through q2 and use q_insert() to copy elements back to q1
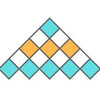
Asked in InterviewBit

Q. Sum of Two Numbers Represented as Arrays
Given two numbers in the form of two arrays where each element of the array represents a digit, calculate the sum of these two numbers and return this sum as an array.
E...read more
Given two numbers represented as arrays, calculate their sum and return the result as an array.
Iterate through the arrays from right to left, adding digits and carrying over if necessary
Handle cases where one array is longer than the other by considering the remaining digits
Ensure the final sum array does not have any leading zeros

Asked in UnitedHealth

Q. Reverse a Number Problem Statement
Create a program to reverse a given integer N
. The output should be the reversed integer.
Note:
If a number has trailing zeros, their reversed version should not include them....read more
Reverse a given integer while excluding trailing zeros.
Create a program to reverse the given integer by converting it to a string and then reversing it.
Remove any trailing zeros from the reversed string before converting it back to an integer.
Handle the constraints of the input integer being between 0 and 10^8.
Example: For input 1230, the output should be 321.
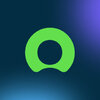
Asked in ServiceNow

Q. Set Matrix Zeros Problem Statement
Given an N x M
integer matrix, if an element is 0, set its entire row and column to 0's, and return the matrix. Specifically, if a cell has a value 0 (i.e., matrix[i][j] == 0
)...read more
To solve the Set Matrix Zeros problem, we can use O(1) space by utilizing the first row and column to store information about zeros in the rest of the matrix.
Iterate through the matrix and use the first row and column to mark rows and columns that need to be zeroed out.
After marking, iterate through the matrix again and zero out the rows and columns based on the marks in the first row and column.
Remember to handle the special case of the first row and column separately.
Exampl...read more
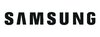
Asked in Samsung

Q. Reverse Linked List Problem Statement
Given a singly linked list of integers, return the head of the reversed linked list.
Example:
Initial linked list: 1 -> 2 -> 3 -> 4 -> NULL
Reversed linked list: 4 -> 3 -> 2...read more
Reverse a singly linked list of integers and return the head of the reversed linked list.
Iterate through the linked list and reverse the pointers to point to the previous node instead of the next node.
Use three pointers to keep track of the current, previous, and next nodes while reversing the linked list.
Update the head of the reversed linked list as the last node encountered during the reversal process.
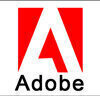
Asked in Adobe

Q. Spiral Matrix Path Problem
You are provided with a two-dimensional array named MATRIX
of size N x M, consisting of integers. Your task is to return the elements of the matrix following a spiral order.
Input:
Th...read more
Implement a function to return elements of a matrix in spiral order.
Iterate through the matrix in a spiral order by adjusting boundaries as you move along.
Keep track of the direction of movement (right, down, left, up) to traverse the matrix correctly.
Handle edge cases like when the matrix is a single row or column separately.
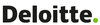
Asked in Deloitte

Q. What are the differences between stored procedures and triggers in SQL?
Stored procedures are precompiled SQL statements that can be executed on demand, while triggers are automatically executed in response to specific events.
Stored procedures are explicitly called by the user, while triggers are automatically invoked by the database system.
Stored procedures can accept parameters and return values, while triggers cannot.
Stored procedures can be executed independently, while triggers are always associated with a specific table or view.
Stored proce...read more
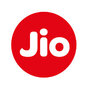
Asked in Jio Platforms

Q. Maximum Sum After Removing K Corner Elements
Given an array arr
of 'N' integer elements, your goal is to remove 'K' elements from either the beginning or the end of the array. The task is to return the maximum ...read more
Given an array of integers, remove K elements from either end to maximize sum of remaining elements.
Iterate through all possible combinations of removing K elements from start and end
Calculate sum of remaining elements for each combination
Return the maximum sum obtained
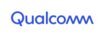
Asked in Qualcomm

Q. Given an array A[n], write a C program to find P and Q (P>Q) such that A[P] - A[Q] is maximum
C program to find P and Q (P>Q) such that A[P] - A[Q] is maximum
Iterate through array and keep track of maximum difference and corresponding indices
Initialize P and Q to first two elements and update as necessary
Time complexity: O(n)
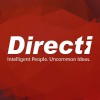
Asked in Directi

Q. Maximum Sum Subarray Problem Statement
Given an array of integers, find the maximum sum of any contiguous subarray within the array.
Example:
Input:
array = [34, -50, 42, 14, -5, 86]
Output:
137
Explanation:
Th...read more
Find the maximum sum of any contiguous subarray within an array in O(N) time complexity.
Use Kadane's algorithm to find the maximum sum subarray in O(N) time complexity
Initialize max_sum and current_sum to the first element of the array
Iterate through the array, updating current_sum and max_sum as needed
Return max_sum as the maximum sum of any contiguous subarray
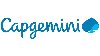
Asked in Capgemini

Q. What are the seven layers of networking?
The seven layers of networking refer to the OSI model which defines how data is transmitted over a network.
The seven layers are: Physical, Data Link, Network, Transport, Session, Presentation, and Application.
Each layer has a specific function and communicates with the layers above and below it.
For example, the Physical layer deals with the physical transmission of data, while the Application layer deals with user interfaces and applications.
Understanding the OSI model is imp...read more
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
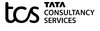
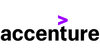
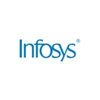
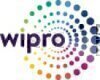
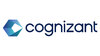
Top Interview Questions for Software Engineer Related Skills
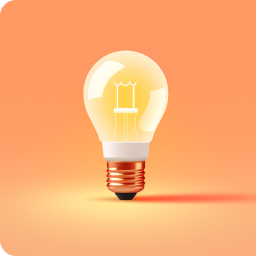
Calculate your in-hand salary
Confused about how your in-hand salary is calculated? Enter your annual salary (CTC) and get your in-hand salary
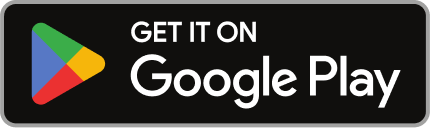
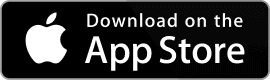
Reviews
Interviews
Salaries
Users
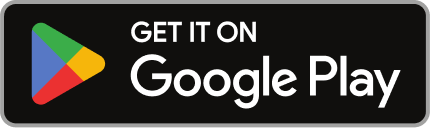
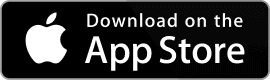