Senior Software Engineer
4500+ Senior Software Engineer Interview Questions and Answers
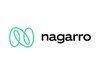
Asked in Nagarro

Q. Nth Prime Number Problem Statement
Find the Nth prime number given a number N.
Explanation:
A prime number is greater than 1 and is not the product of two smaller natural numbers. A prime number has exactly two...read more
The task is to find the Nth prime number given a number N.
A prime number is a number greater than 1 that is not a product of two smaller natural numbers.
Prime numbers have only two factors - 1 and the number itself.
Start with a counter at 0 and a number at 2.
Increment the number by 1 and check if it is prime.
If it is prime, increment the counter.
Repeat until the counter reaches N.
Return the last prime number found.
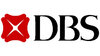
Asked in DBS Bank

Q. Tell me about yourself. What technology are you using? What is a Collection? What are the different types of collection there? What is the difference between ArrayList and LinkedList What are the basic building...
read moreThe interview questions cover a wide range of topics including software engineering, technology, design patterns, databases, web development, and more.
The questions cover topics such as technology used, collections, streams, design patterns, dependency injection, microservices, database communication, annotations in Spring, React and JavaScript, Redux, API development, SOAP-based web services, Jenkins, Docker, production support, and more.
Some specific questions include the d...read more
Senior Software Engineer Interview Questions and Answers for Freshers
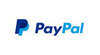
Asked in PayPal

Q. K Largest Elements Problem Statement
You are given an integer k
and an array of integers that contain numbers in random order. Write a program to find the k
largest numbers from the given array. You need to sav...read more
Given an unsorted array, find the K largest elements in non-decreasing order.
Sort the array in non-decreasing order.
Return the last K elements of the sorted array.
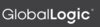
Asked in GlobalLogic

Q. MapSum Pair Implementation
Create a data structure named 'MapSum' with the ability to perform two operations and a constructor.
Explanation:
MapSum()
: Initializes the MapSum.insert(...read more
The question asks to implement a data structure called 'MapSum' with functions to initialize, insert key-value pairs, and find the sum of values with a given prefix.
Implement a class called 'MapSum' with a constructor to initialize the data structure.
Implement the 'insert' function to insert key-value pairs into the 'MapSum'. If a key is already present, replace its value with the new one.
Implement the 'sum' function to find the sum of values whose key has a prefix equal to t...read more
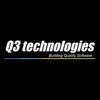
Asked in Q3 Technologies

Q. If you have to prioritize between coding standards and project delivery when the deadline is very tight, which would be your priority?
In tight deadlines, delivering the project is prioritized, but coding standards should not be completely ignored for long-term maintainability.
Prioritize delivery to meet deadlines, especially in critical situations.
Implement a minimal set of coding standards to ensure code quality.
Use code reviews post-delivery to address any coding standard violations.
Consider the impact of technical debt; prioritize refactoring after delivery.
Example: In a previous project, we delivered a ...read more

Asked in JPMorgan Chase & Co.

Q. Pascal's Triangle Construction
You are provided with an integer 'N'. Your task is to generate a 2-D list representing Pascal’s triangle up to the 'N'th row.
Pascal's triangle is a triangular array where each el...read more
Generate Pascal's triangle up to the Nth row using a 2-D list.
Iterate through each row up to N, starting with [1] as the first row.
Calculate each element in a row by summing the two elements directly above from the previous row.
Append each row to the 2-D list until reaching the Nth row.
Example: For N = 4, the output would be [ [1], [1, 1], [1, 2, 1], [1, 3, 3, 1] ]
Senior Software Engineer Jobs
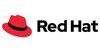
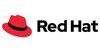
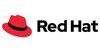
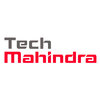
Asked in Tech Mahindra

Q. How to apply SOLID principle and what is dependency injection and why to use it and scenarios on where to use
SOLID principles ensure maintainable and scalable code. Dependency Injection helps in achieving loose coupling and testability.
SOLID principles are Single Responsibility, Open-Closed, Liskov Substitution, Interface Segregation, and Dependency Inversion
Dependency Injection is a design pattern that allows objects to be loosely coupled and easily testable
Use Dependency Injection to reduce tight coupling between classes and make them more modular
Use SOLID principles to ensure mai...read more
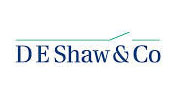
Asked in DE Shaw

Q. Buy and Sell Stock Problem Statement
Imagine you are Harshad Mehta's friend, and you have been given the stock prices of a particular company for the next 'N' days. You can perform up to two buy-and-sell transa...read more
The task is to determine the maximum profit that can be achieved by performing up to two buy-and-sell transactions on a given set of stock prices.
Iterate through the array of stock prices to find the maximum profit that can be achieved by buying and selling stocks at different points.
Keep track of the maximum profit that can be achieved by considering all possible combinations of buy and sell transactions.
Ensure that you sell the stock before you can buy again to adhere to th...read more
Share interview questions and help millions of jobseekers 🌟
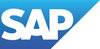
Asked in SAP

Q. Duplicate Integer in Array
Given an array ARR
of size N
, containing each number between 1 and N-1
at least once, identify the single integer that appears twice.
Input:
The first line contains an integer, 'T', r...read more
Identify the duplicate integer in an array containing numbers between 1 and N-1.
Iterate through the array and keep track of the frequency of each element using a hashmap.
Return the element with a frequency greater than 1 as the duplicate integer.
Ensure the constraints are met and a duplicate number is guaranteed to be present.

Asked in VMware Software

Q. Reverse the String Problem Statement
You are given a string STR
which contains alphabets, numbers, and special characters. Your task is to reverse the string.
Example:
Input:
STR = "abcde"
Output:
"edcba"
Input...read more
The task is to reverse a given string containing alphanumeric and special characters.
Iterate through the string from the last character to the first character
Append each character to a new string to get the reversed string
Return the reversed string as the output
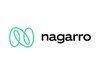
Asked in Nagarro

Q. Digits Decoding Problem Statement
A few days back, Ninja encountered a string containing characters from ‘A’ to ‘Z’ which indicated a secret message. For security purposes, he encoded each character of the stri...read more
The problem involves decoding a numeric sequence into a valid string using a given mapping of characters to numbers.
Use dynamic programming to count the number of ways to decode the sequence.
Consider different cases for decoding single digits and pairs of digits.
Keep track of the number of ways to decode at each position in the sequence.
Return the final count modulo 10^9 + 7 as the answer.
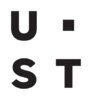
Asked in UST

Q. Excel Column Number Conversion
Given a column title as it appears in an Excel sheet, your task is to return its corresponding column number.
Example:
Input:
S = "AB"
Output:
28
Explanation:
The sequence is as f...read more
Convert Excel column title to corresponding column number.
Iterate through the characters in the input string from right to left
Calculate the corresponding value of each character based on its position and multiply by 26^position
Sum up all the values to get the final column number
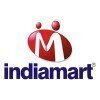
Asked in Indiamart Intermesh

Q. Prime Numbers within a Range
Given an integer N, determine and print all the prime numbers between 2 and N, inclusive.
Input:
Integer N
Output:
Prime numbers printed on separate lines
Example:
Input:
N = 10
Out...read more
Generate and print all prime numbers between 2 and N, inclusive.
Iterate from 2 to N and check if each number is prime
A prime number is only divisible by 1 and itself
Print each prime number on a new line
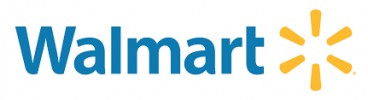
Asked in Walmart

Q. Find All Anagrams Problem Statement
Given a string STR and a non-empty string PTR, identify all the starting indices of anagrams of PTR within STR.
Explanation:
An anagram of a string is another string that can...read more
Given a string STR and a non-empty string PTR, find all starting indices of anagrams of PTR within STR.
Create a frequency map of characters in PTR.
Use sliding window technique to check anagrams in STR.
Return the starting indices of anagrams found.
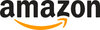
Asked in Amazon

Q. LRU Cache Design Question
Design a data structure for a Least Recently Used (LRU) cache that supports the following operations:
1. get(key)
- Return the value of the key if it exists in the cache; otherwise, re...read more
Design a Least Recently Used (LRU) cache data structure that supports get and put operations with capacity constraint.
Implement a doubly linked list to keep track of the order of keys based on their recent usage.
Use a hashmap to store key-value pairs for quick access and updates.
When capacity is reached, evict the least recently used item before inserting a new item.
Update the order of keys in the linked list whenever a key is accessed or updated.
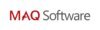
Asked in MAQ Software

Q. Sum of Digits Problem Statement
Given an integer 'N', continue summing its digits until the result is a single-digit number. Your task is to determine the final value of 'N' after applying this operation iterat...read more
Given an integer, find the final single-digit value after summing its digits iteratively.
Iteratively sum the digits of the input integer until the result is a single-digit number
Output the final single-digit integer for each test case
Handle multiple test cases as input
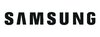
Asked in Samsung

Q. Triplets with Given Sum Problem
Given an array or list ARR
consisting of N
integers, your task is to identify all distinct triplets within the array that sum up to a specified number K
.
Explanation:
A triplet i...read more
The task is to identify all distinct triplets within an array that sum up to a specified number.
Iterate through the array and use nested loops to find all possible triplets.
Keep track of the sum of each triplet and compare it with the target sum.
Print the triplet if the sum matches the target sum, else print -1.
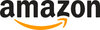
Asked in Amazon

Q. Boundary Traversal of a Binary Tree
Given a binary tree of integers, your task is to return the boundary nodes of the tree in Anti-Clockwise direction starting from the root node.
Input:
The first line contains...read more
Return the boundary nodes of a binary tree in Anti-Clockwise direction starting from the root node.
Traverse the left boundary nodes in top-down order
Traverse the leaf nodes in left-right order
Traverse the right boundary nodes in bottom-up order
Handle cases where duplicates occur in boundary nodes

Asked in Visa

Q. Given a grid containing 0s and 1s and a source row and column, in how many ways can we reach the target from the source? (1s represent blockades and 0s represent accessible points.)
Count the number of ways to reach target from source in a grid with 0s and 1s.
Use dynamic programming to solve the problem efficiently.
Traverse the grid using DFS or BFS to count the number of ways.
Consider edge cases like when source and target are the same or when there is no path.
Example: Given grid = [[0,0,0],[0,1,0],[0,0,0]], source = (0,0), target = (2,2), answer is 2.
Example: Given grid = [[0,1],[0,0]], source = (0,0), target = (1,1), answer is 1.
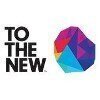
Asked in TO THE NEW

Q. Character Counting Challenge
Create a program that counts and prints the total number of specific character types from user input. Specifically, you need to count lowercase English alphabets, numeric digits (0-...read more
Create a program to count lowercase alphabets, digits, and white spaces from user input until '$' is encountered.
Read characters from input stream until '$' is encountered
Count lowercase alphabets, digits, and white spaces separately
Print the counts of each character type as three integers separated by spaces
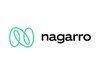
Asked in Nagarro

Q. Secret Message Decoding Problem
Ninja encountered an encoded secret message where each character from 'A' to 'Z' is mapped to a numeric value (A = 1, B = 2, ..., Z = 26). Given a numeric sequence (SEQ) derived ...read more
The problem involves decoding a numeric sequence back into a valid string based on a given mapping of characters to numeric values.
Use dynamic programming to keep track of the number of ways to decode the sequence at each position.
Consider edge cases such as '0' and '00' which do not have valid decodings.
Handle cases where two digits can be combined to form a valid character (e.g., '12' corresponds to 'L').
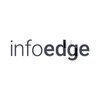
Asked in Info Edge

Q. Number of Bit Flips Problem Statement
Ninja is practicing binary representations and stumbled upon an interesting problem. Given two numbers 'A' and 'B', you are required to determine how many bits need to be f...read more
Calculate the number of bit flips required to convert one number to another in binary representation.
Convert both numbers to binary representation
Count the number of differing bits between the two numbers
Output the count of differing bits as the number of bit flips required
Example: A = 13 (1101), B = 7 (0111) -> 2 bit flips required
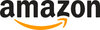
Asked in Amazon

Q. String Palindrome Verification
Given a string, your task is to determine if it is a palindrome considering only alphanumeric characters.
Input:
The input is a single string without any leading or trailing space...read more
Check if a given string is a palindrome considering only alphanumeric characters.
Remove non-alphanumeric characters from the input string before checking for palindrome.
Use two pointers approach to compare characters from start and end of the string.
Convert all characters to lowercase for case-insensitive comparison.
Return true if the string is a palindrome, false otherwise.
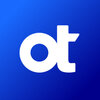
Asked in OpenText Technologies

Q. Write a program to find the Nth term in the infinite series 2, 4, 8, 2, 4, 8, ...
Program to find the Nth term in an infinite series
The series has a repeating pattern
Use modulo operator to find the index of the repeating pattern
Calculate the value of Nth term based on the pattern
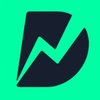
Asked in Dunzo

Q. Anagram Substring Search
Given two strings 'STR' and 'PTR', identify all starting indices of 'PTR' anagram substrings in 'STR'. Two strings are anagrams if one can be rearranged to form the other.
Input:
First ...read more
Implement a function to find all starting indices of anagram substrings of a given string in another string.
Create a frequency map of characters in the 'PTR' string.
Use a sliding window approach to check for anagrams in 'STR'.
Return the starting indices of anagram substrings found.
Example: For input 'BACDGABCD' and 'ABCD', output should be [0, 5].
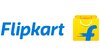
Asked in Flipkart

Q. K-th Largest Number in a BST
Given a binary search tree (BST) consisting of integers and containing 'N' nodes, your task is to find and return the K-th largest element in this BST.
If there is no K-th largest e...read more
Find the K-th largest element in a BST.
Perform reverse in-order traversal of the BST to find the K-th largest element.
Keep track of the count of visited nodes to determine the K-th largest element.
Return -1 if there is no K-th largest element in the BST.
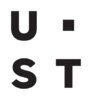
Asked in UST

Q. LRU Cache Design Problem Statement
Design and implement a data structure for a Least Recently Used (LRU) cache that supports the following operations:
get(key)
- Retrieve the value associated with the specifie...read more
The question is about designing and implementing a data structure for LRU cache to support get and put operations.
LRU cache is a cache replacement policy that removes the least recently used item when the cache reaches its capacity.
The cache is initialized with a capacity and supports get(key) and put(key, value) operations.
For each get operation, return the value of the key if it exists in the cache, otherwise return -1.
For each put operation, insert the value in the cache i...read more
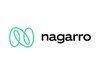
Asked in Nagarro

Q. Trapping Rain Water Problem Statement
Given a long type array/list ARR
of size N
, representing an elevation map where ARR[i]
denotes the elevation of the ith
bar, calculate the total amount of rainwater that ca...read more
Calculate the total amount of rainwater that can be trapped in given elevation map.
Iterate through the array to find the maximum height on the left and right of each bar.
Calculate the amount of water that can be trapped above each bar by taking the minimum of the maximum heights on the left and right.
Sum up the trapped water above each bar to get the total trapped water for the entire elevation map.
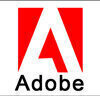
Asked in Adobe

Q. Search In Rotated Sorted Array Problem Statement
Given a sorted array of distinct integers that has been rotated clockwise by an unknown amount, you need to search for a specified integer in the array. For each...read more
Implement a search function to find a specified integer in a rotated sorted array with O(logN) time complexity.
Implement a binary search algorithm to efficiently search for the target integer.
Handle the rotation of the array by finding the pivot point first.
Return the index of the target integer if found, else return -1.
Ensure the time complexity of the search function is O(logN) for each query.
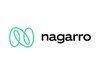
Asked in Nagarro

Q. Count Ways to Reach the N-th Stair Problem Statement
You are provided with a number of stairs, and initially, you are located at the 0th stair. You need to reach the Nth stair, and you can climb one or two step...read more
The problem involves counting the number of distinct ways to climb N stairs by taking 1 or 2 steps at a time.
Use dynamic programming to solve this problem efficiently.
The number of ways to reach the Nth stair is equal to the sum of ways to reach (N-1)th stair and (N-2)th stair.
Handle modulo 10^9+7 to avoid overflow issues.
Consider base cases for 0th and 1st stair.
Optimize the solution by using constant space instead of an array.
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
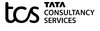
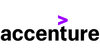
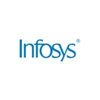
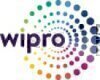
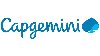
Top Interview Questions for Senior Software Engineer Related Skills
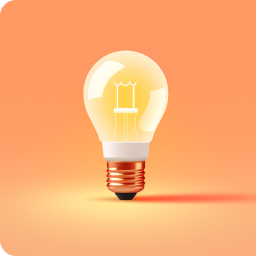
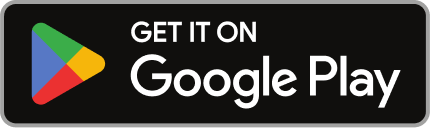
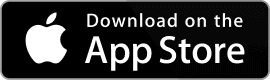
Reviews
Interviews
Salaries
Users
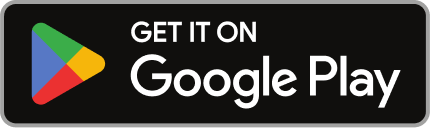
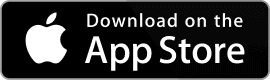