Senior Software Engineer 1
100+ Senior Software Engineer 1 Interview Questions and Answers
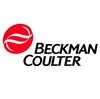
Asked in Beckman Coulter

Q. What are some commonly used design patterns in software development?
Design patterns are reusable solutions to common software design problems, enhancing code maintainability and scalability.
Singleton: Ensures a class has only one instance (e.g., a configuration manager).
Observer: Allows objects to subscribe and receive notifications (e.g., event handling in UI frameworks).
Factory: Creates objects without specifying the exact class (e.g., a shape factory that produces circles or squares).
Strategy: Defines a family of algorithms and makes them ...read more
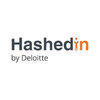
Asked in HashedIn by Deloitte

Q. Why should one use Node.JS?
Node.js is a powerful and efficient server-side JavaScript runtime environment.
Node.js is fast and scalable, making it ideal for building real-time applications.
It uses an event-driven, non-blocking I/O model, which makes it lightweight and efficient.
Node.js has a large and active community, with many useful libraries and modules available.
It allows for easy sharing of code between the server and client, using JavaScript on both sides.
Node.js is cross-platform, meaning it can...read more
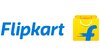
Asked in Flipkart

Q. Design a calendar app to resolve meeting conflicts and return meetings that can be attended based on given preference criteria.
Design a calendar app to resolve meeting conflicts and return preferred meetings
Implement a feature to detect conflicts in meeting schedules
Allow users to set preference criteria such as importance, duration, time of day, etc.
Prioritize meetings based on preference criteria and availability
Provide users with a list of meetings that can be attended without conflicts
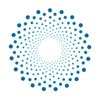
Asked in Catchpoint

Q. Explain how to apply the Liskov Substitution Principle.
Liskov Substitution Principle states that objects of a superclass should be replaceable with objects of its subclasses without affecting the program's correctness.
Subclasses should be able to substitute their parent classes without altering the behavior of the program.
Ensure that subclasses do not override or change the behavior of parent class methods.
Avoid using type checking or casting to subclasses in the code.
Example: If a method expects a superclass object, it should wo...read more
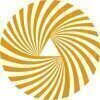
Asked in Altimetrik

Q. Given an array of digits consisting of 0s, 1s, and 2s, sort the array so that all 0s come first, followed by all 1s, and then all 2s.
Sort an array of digits (0s, 1s, 2s) so that all 0s come first, followed by 1s, and then 2s.
Use the Dutch National Flag algorithm for efficient sorting.
Initialize three pointers: low, mid, and high.
Iterate through the array with mid pointer; swap elements based on their value.
Example: Input: [2, 0, 1, 2, 0, 1] -> Output: [0, 0, 1, 1, 2, 2].
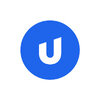
Asked in Upland Software

Q. What is your opinion on Nested Views in SQL Server?
Nested views in SQL Server can be useful for simplifying complex queries and improving performance.
Nested views can help in breaking down complex queries into smaller, more manageable parts.
They can improve query performance by allowing the optimizer to optimize each view separately before combining them.
However, excessive nesting of views can lead to performance issues and make the code harder to maintain.
It is important to strike a balance between using nested views for cla...read more
Senior Software Engineer 1 Jobs
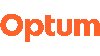
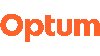
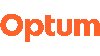
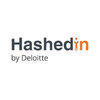
Asked in HashedIn by Deloitte

Q. What are OS processes?
OS processes are instances of a program that are being executed by the operating system.
Processes are managed by the operating system's scheduler.
Each process has its own memory space and system resources.
Processes can communicate with each other through inter-process communication (IPC).
Examples of processes include web browsers, media players, and text editors.
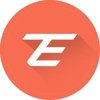
Asked in ZeMoSo Technologies

Q. Given a string, count the continuous unique characters. For example, for the input string "aaaaBbbbCDEaAb", the expected output is: a4 B4 C1 D1 E1 a2 b2
Count the continuous unique characters in a given string.
Iterate through the string and keep track of the current character and its count
If the current character is different from the previous one, print the count and reset it
Handle both uppercase and lowercase characters separately
Share interview questions and help millions of jobseekers 🌟
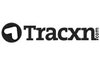
Asked in Tracxn

Q. Given a set of non-negative integers, arrange them such that they form the largest number.
Arrange non-negative integers to form the largest number
Convert integers to strings for comparison
Sort strings in descending order based on custom comparator
Join sorted strings to form the largest number
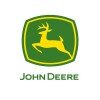
Asked in John Deere

Q. What is the memory layout in microcontrollers?
Memory layout in microcontrollers includes various sections for code, data, and stack, optimizing resource usage.
Flash Memory: Stores program code (e.g., firmware) and is non-volatile.
SRAM: Used for runtime data storage, such as variables and stack.
EEPROM: Non-volatile memory for storing configuration settings.
Memory Map: Defines address ranges for different memory types, e.g., 0x0000 to 0xFFFF for Flash.
Peripheral Registers: Mapped in memory for hardware control, e.g., GPIO ...read more
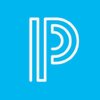
Asked in PowerSchool India

Q. What branching strategies have you used?
I have used GitFlow and feature branch strategies in previous projects.
Utilized GitFlow branching model for managing feature development and releases
Created feature branches for each new feature or bug fix
Merged feature branches back into development branch after code review and testing
Used tags to mark releases in the master branch
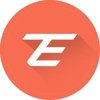
Asked in ZeMoSo Technologies

Q. How do you find the number of unique elements in an array with in-place modification?
Use hash set to track unique elements while iterating through array and modify array in-place
Iterate through array and add elements to hash set to track unique elements
Modify array in-place by removing duplicates using hash set
Return the size of the hash set as the number of unique elements
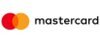
Asked in MasterCard

Q. Write Java code to find the number of repeated characters in a String.
Java code to find number of repeated characters in a String.
Create a HashMap to store characters and their counts.
Iterate through the String and update the counts in the HashMap.
Count the characters with counts greater than 1 to find repeated characters.
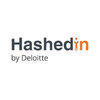
Asked in HashedIn by Deloitte

Q. Low Level Design for Authentication
Low level design for authentication
Define authentication requirements
Choose appropriate authentication mechanism
Design authentication flow
Implement secure storage of credentials
Consider multi-factor authentication
Include error handling and logging
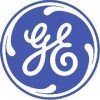
Asked in GE Healthcare

Q. Describe the system design of a lift mechanism.
Design a lift mechanism system for efficient vertical transportation in buildings.
Define the lift capacity (e.g., 1000 kg for standard passenger lifts).
Determine the number of floors and lift speed (e.g., 1 meter/second).
Implement a control system for call buttons and floor selection.
Consider safety features like emergency brakes and alarms.
Design for energy efficiency, possibly using regenerative drives.
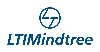
Asked in LTIMindtree

Q. What are adapters and what types of adaptables are there?
Adapters are used to convert the interface of a class into another interface that a client expects. Types of adaptables include object adapters and class adapters.
Adapters are used to make two incompatible interfaces work together.
Object adapters use composition to adapt the interface of a class.
Class adapters use multiple inheritance to adapt the interface of a class.
Adaptables can be objects or classes that need to be adapted.
Examples of adaptables include legacy systems, t...read more
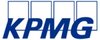
Asked in KPMG India

Q. What are the transient and volatile keywords?
transient and volatile are keywords in Java used for different purposes
transient keyword is used to indicate that a field should not be serialized when converting an object to byte stream
volatile keyword is used to indicate that a variable's value will be modified by different threads
transient keyword is used in serialization, while volatile keyword is used in multithreading
example: transient keyword - private transient int age; example: volatile keyword - private volatile bo...read more
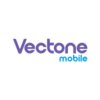
Asked in Vectone Mobile

Q. What is redux , use memo, use reducer
Redux is a state management library for JavaScript applications. useMemo and useReducer are hooks in React for optimizing performance.
Redux is used for managing the state of a JavaScript application in a predictable way.
useMemo is a hook in React that memoizes the result of a function to optimize performance by avoiding unnecessary re-computations.
useReducer is a hook in React that is an alternative to useState for managing complex state logic in components.
Asked in Centime Technology

Q. What is Babel, and why do you use it?
Babel is a JavaScript compiler that allows you to use the latest ECMAScript features in your code.
Babel transpiles modern JavaScript code into older versions that are compatible with all browsers.
It allows developers to write code using the latest syntax without worrying about browser support.
Babel can also be configured to support specific plugins and presets for additional functionality.
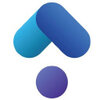
Asked in Atrina Technologies

Q. What is lazy loading in Angular?
Lazy loading in Angular is a technique used to load modules only when they are needed, improving performance by reducing initial load time.
Lazy loading helps in splitting the application into multiple bundles which are loaded on demand.
It allows for faster initial loading times as only essential modules are loaded upfront.
Lazy loading is achieved by using the loadChildren property in the route configuration of Angular.
Example: loadChildren: () => import('./lazy-loaded-module'...read more
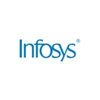
Asked in Infosys

Q. What is the difference between an abstract class and an interface?
Abstract class is a class that cannot be instantiated and can have both abstract and non-abstract methods. Interface is a collection of abstract methods.
Abstract class can have constructors while interface cannot
A class can implement multiple interfaces but can only inherit from one abstract class
Abstract class can have instance variables while interface cannot
Abstract class is used when we want to provide a common base implementation to derived classes while interface is use...read more
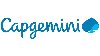
Asked in Capgemini

Q. Write string manipulation programs using LINQ.
Using LINQ to manipulate string arrays in C#
Use LINQ methods like Where, Select, OrderBy, etc. to filter, transform, or sort string arrays
Example: string[] names = {"Alice", "Bob", "Charlie"}; var result = names.Where(name => name.Length > 4);
Example: string[] fruits = {"apple", "banana", "cherry"}; var sortedFruits = fruits.OrderBy(fruit => fruit);
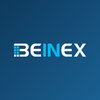
Asked in Beinex

Q. What is clean architecture?
Clean architecture is a software design approach that separates concerns and enforces a clear separation of layers.
Clean architecture focuses on separating business logic from implementation details.
It promotes testability, maintainability, and scalability of the software.
Common components in clean architecture include entities, use cases, and interfaces.
Examples of clean architecture patterns include Hexagonal Architecture and Onion Architecture.
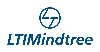
Asked in LTIMindtree

Q. LLM model list which is better and why
Comparing LLMs involves evaluating performance, architecture, and use cases to determine the best fit for specific applications.
GPT-3: Known for its versatility and ability to generate human-like text, making it suitable for creative writing and chatbots.
BERT: Excels in understanding context and semantics, ideal for tasks like sentiment analysis and question answering.
T5: A text-to-text transformer that can handle various NLP tasks by framing them as text generation problems,...read more
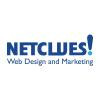
Asked in Netclues

Q. What is the difference between let, const, and var?
let, const, and var are all used to declare variables in JavaScript, but they have different scopes and behaviors.
let: block-scoped, can be reassigned but not redeclared
const: block-scoped, cannot be reassigned or redeclared, but its properties can be modified
var: function-scoped, can be reassigned and redeclared
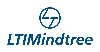
Asked in LTIMindtree

Q. What are run modes in AEM, and how do custom run modes work?
Run modes in AEM are configurations that determine how the AEM instance will behave. Custom run modes are additional configurations created by users.
Run modes in AEM include author, publish, and dispatcher.
Custom run modes can be created by adding custom configurations in the runmodes folder.
Custom run modes can be used to define specific behaviors or settings for the AEM instance.
Asked in Imaginnovate

Q. Solve problems related to JavaScript arrays and objects.
Understanding JavaScript arrays and objects is crucial for effective problem-solving in software engineering.
Arrays are ordered collections of values, e.g., const arr = [1, 2, 3];
Objects are key-value pairs, e.g., const obj = { name: 'Alice', age: 25 };
You can access array elements using indices, e.g., arr[0] returns 1;
Object properties can be accessed using dot notation or bracket notation, e.g., obj.name or obj['age'];
Arrays can be manipulated using methods like push(), pop...read more
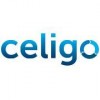
Asked in Celigo

Q. All components of social media application.
A social media application consists of various components that facilitate user interaction, content sharing, and community building.
User Profiles: Each user has a profile containing personal information, profile picture, and posts, e.g., Facebook profiles.
News Feed: A dynamic stream of updates from friends and followed accounts, showcasing posts, images, and videos, e.g., Twitter feed.
Messaging System: Enables direct communication between users through private messages or gro...read more
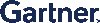
Asked in Gartner

Q. Describe a coding problem that can be solved using recursion.
Implementing a factorial function using recursion
Define a function that takes an integer as input
Base case: if input is 0, return 1
Recursive case: return input multiplied by the factorial of input-1
Example: factorial(5) = 5 * factorial(4) = 5 * 4 * factorial(3) = ... = 5 * 4 * 3 * 2 * 1
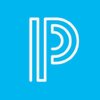
Asked in PowerSchool India

Q. Explain Android Architecture Components.
Android architecture components are a collection of libraries that help you design robust, testable, and maintainable apps.
Android architecture components include LiveData, ViewModel, Room, and WorkManager.
LiveData is an observable data holder class that is lifecycle-aware.
ViewModel provides data to the UI and survives configuration changes.
Room is a SQLite object mapping library that provides an abstraction layer over SQLite.
WorkManager is used for managing deferrable, async...read more
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
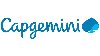
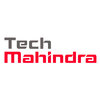
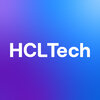
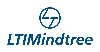
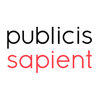
Top Interview Questions for Senior Software Engineer 1 Related Skills
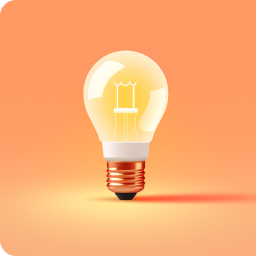
Calculate your in-hand salary
Confused about how your in-hand salary is calculated? Enter your annual salary (CTC) and get your in-hand salary
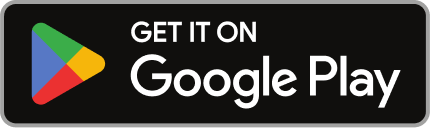
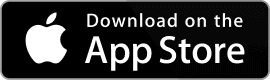
Reviews
Interviews
Salaries
Users
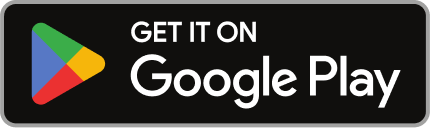
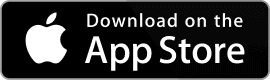