Associate Software Engineer
1500+ Associate Software Engineer Interview Questions and Answers
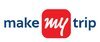
Asked in MakeMyTrip

Q. Sub Sort Problem Statement
You are given an integer array ARR
. Determine the length of the shortest contiguous subarray which, when sorted in ascending order, results in the entire array being sorted in ascendi...read more
The question asks to find the length of the shortest contiguous subarray that needs to be sorted in order to sort the whole array.
Iterate through the array and find the first element that is out of order from the left side.
Iterate through the array and find the first element that is out of order from the right side.
Find the minimum and maximum element within the subarray from the above steps.
Expand the subarray from both sides until all elements within the subarray are in the...read more
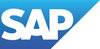
Asked in SAP

Q. Rearrange Array: Move Negative Numbers to the Beginning
Given an array ARR
consisting of N
integers, rearrange the elements such that all negative numbers are located before all positive numbers. The order of e...read more
Yes, this can be achieved by using a two-pointer approach to rearrange the array with negative numbers at the beginning.
Use two pointers, one starting from the beginning and one from the end of the array.
Swap elements at the two pointers if one is negative and the other is positive, and move the pointers accordingly.
Continue this process until the two pointers meet in the middle of the array.
The resulting array will have all negative numbers before positive numbers, with the ...read more
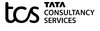
Asked in TCS

Q. Constellation Identification Problem
Given a matrix named UNIVERSE
with 3 rows and 'N' columns, filled with characters {#, *, .}, where:
- '*' represents stars.
- '.' represents empty space.
- '#' represents a separ...read more
The task is to identify constellations shaped like vowels within a matrix filled with characters {#, *, .}.
Iterate through the matrix to find 3x3 constellations shaped like vowels.
Check for vowels 'A', 'E', 'I', 'O', 'U' in each 3x3 constellation.
Print the vowels found in each constellation for each test case.
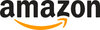
Asked in Amazon

Q. Find All Pairs Adding Up to Target
Given an array of integers ARR
of length N
and an integer Target
, your task is to return all pairs of elements such that they add up to the Target
.
Input:
The first line conta...read more
Given an array of integers and a target, find all pairs of elements that add up to the target.
Iterate through the array and for each element, check if the target minus the element exists in a hash set.
If it exists, print the pair (element, target-element), otherwise add the element to the hash set.
If no pair is found, print (-1, -1).
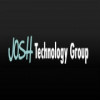
Asked in Josh Technology Group

Q. N Queens Problem
Given an integer N
, find all possible placements of N
queens on an N x N
chessboard such that no two queens threaten each other.
Explanation:
A queen can attack another queen if they are in the...read more
The N Queens Problem involves finding all possible placements of N queens on an N x N chessboard where no two queens threaten each other.
Understand the constraints of the problem: N queens on an N x N chessboard, no two queens can threaten each other.
Implement a backtracking algorithm to explore all possible configurations.
Check for conflicts in rows, columns, and diagonals before placing a queen.
Generate and print valid configurations where queens do not attack each other.
Co...read more
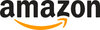
Asked in Amazon

Q. String Transformation Problem
Given a string (STR
) of length N
, you are tasked to create a new string through the following method:
Select the smallest character from the first K
characters of STR
, remove it fr...read more
Given a string and an integer, create a new string by selecting the smallest character from the first K characters of the input string and repeating the process until the input string is empty.
Iterate through the input string, selecting the smallest character from the first K characters each time.
Remove the selected character from the input string and append it to the new string.
Continue this process until the input string is empty.
Return the final new string formed after the...read more
Associate Software Engineer Jobs
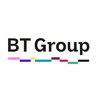
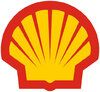
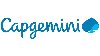

Asked in Bosch Global Software Technologies

Q. Two Sum Problem Statement
Given an array A
of size N
, sorted in non-decreasing order, return two distinct indices whose values add up to a given 'target'. The array is 0 indexed. If multiple answers exist, retu...read more
Given a sorted array, find two distinct indices whose values add up to a given target.
Use two pointers approach to find the indices that add up to the target.
Start with one pointer at the beginning and another at the end of the array.
Move the pointers towards each other based on the sum of their values compared to the target.
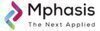
Asked in Mphasis

Q. 1. All types of database commands- DDL, DML, DCL, TCL 2. Write a java code to reverse a given string or sentence 3. Questions based on a major and minor project 4. Questions based on Industrial Training/Interns...
read moreInterview questions for Associate Software Engineer position
Be familiar with all types of database commands and their usage
Practice writing code to reverse a given string or sentence in Java
Be prepared to discuss major and minor projects, as well as industrial training/internship experiences
Have a good understanding of operating system concepts such as job scheduling, deadlock, and starvation
Know the differences between C++ and Java programming languages
Be able to implement l...read more
Share interview questions and help millions of jobseekers 🌟
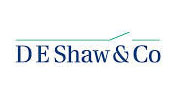
Asked in DE Shaw

Q. Buy and Sell Stock Problem Statement
Imagine you are Harshad Mehta's friend, and you have been given the stock prices of a particular company for the next 'N' days. You can perform up to two buy-and-sell transa...read more
The task is to determine the maximum profit that can be achieved by performing up to two buy-and-sell transactions on a given set of stock prices.
Iterate through the array of stock prices to find the maximum profit that can be achieved by buying and selling stocks.
Keep track of the maximum profit that can be achieved by considering all possible combinations of buy and sell transactions.
Ensure that you sell the stock before buying again to adhere to the constraints of the prob...read more
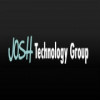
Asked in Josh Technology Group

Q. Height of a Binary Tree
You are provided with an arbitrary binary tree consisting of 'N' nodes where each node is associated with a certain value. The task is to determine the height of the tree.
Explanation:
T...read more
The height of a binary tree is the maximum number of edges from the root to a leaf node.
Traverse the tree recursively and keep track of the maximum height
If the current node is null, return 0
Otherwise, calculate the height of the left and right subtrees and return the maximum height plus 1
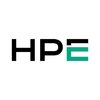
Asked in Hewlett Packard Enterprise

Q. Merge Sort Problem Statement
You are given a sequence of numbers, ARR
. Your task is to return a sorted sequence of ARR
in non-descending order using the Merge Sort algorithm.
Explanation:
The Merge Sort algorit...read more
Implement Merge Sort algorithm to sort a sequence of numbers in non-descending order.
Divide the input array into two halves recursively until each array has only one element.
Merge the sorted halves to produce a completely sorted array.
Time complexity of Merge Sort is O(n log n).
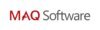
Asked in MAQ Software

Q. Remove String from Linked List Problem
You are provided with a singly linked list where each node contains a single character, along with a string 'STR'. Your task is to remove all occurrences of the string 'ST...read more
Remove all occurrences of a given string from a singly linked list by starting from the end of the list.
Traverse the linked list from the end to the beginning to efficiently remove the string occurrences.
Check for the string 'STR' in each node and remove it if found.
Continue checking for new occurrences of 'STR' after removal to ensure all instances are removed.
Return the head of the modified linked list after processing each test case.
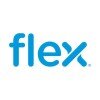
Asked in Flex

Q. Remove Vowels from a Given String
Given a string STR
of length N
, your task is to remove all the vowels present in that string and return the modified string.
Explanation:
English alphabets ‘a’, ‘e’, ‘i’, ‘o’, ...read more
Remove vowels from a given string while maintaining the relative position of other characters.
Iterate through each character in the string and check if it is a vowel (a, e, i, o, u).
If the character is not a vowel, add it to the modified string.
Return the modified string as the output.
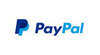
Asked in PayPal

Q. Replace Spaces in a String
Given a string STR
consisting of words separated by spaces, your task is to replace all spaces between words with the characters "@40".
Input:
The first line contains an integer ‘T’ d...read more
Replace spaces in a string with '@40'.
Iterate through the string and replace spaces with '@40'.
Return the modified string for each test case.
Handle multiple test cases by iterating through each one.
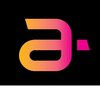
Asked in Amdocs

Q. Subarray with Equal Occurrences Problem Statement
You are provided with an array/list ARR
of length N
containing only 0s and 1s. Your goal is to determine the number of non-empty subarrays where the number of 0...read more
Count the number of subarrays where the number of 0s is equal to the number of 1s in a given array of 0s and 1s.
Iterate through the array and keep track of the count of 0s and 1s encountered so far.
Use a hashmap to store the count of 0s and 1s encountered at each index.
For each index, check if the count of 0s and 1s encountered so far are equal. If yes, increment the total count of subarrays.
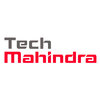
Asked in Tech Mahindra

Q. 1) What is NullPointerExceprion and give me a example?
NullPointerException is a runtime exception that occurs when a program tries to access or use an object reference that is null.
It is a common exception in Java programming.
It is thrown when a program attempts to use an object reference that has not been initialized.
It indicates that there is an attempt to access or invoke a method on an object that is null.
Example: String str = null; str.length();
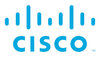
Asked in Cisco

Q. Intersection of Linked List Problem
You are provided with two singly linked lists containing integers, where both lists converge at some node belonging to a third linked list.
Your task is to determine the data...read more
Find the node where two linked lists merge, return -1 if no merging occurs.
Traverse both lists to find the lengths and the last nodes
Align the starting points of the lists by adjusting the pointers
Traverse again to find the merging point
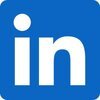
Asked in LinkedIn

Q. DFS Traversal Problem Statement
Given an undirected and disconnected graph G(V, E)
, where V
is the number of vertices and E
is the number of edges, the connections between vertices are provided in the 'GRAPH' m...read more
The question asks to print the DFS traversal of an undirected and disconnected graph.
Implement a Depth First Search (DFS) algorithm to traverse the graph.
Use a visited array to keep track of visited vertices.
For each unvisited vertex, start a DFS traversal and print the connected component.
Sort the vertices of each connected component in ascending order before printing.
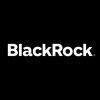
Asked in Blackrock

Q. Merge Two Sorted Arrays Problem Statement
Given two sorted integer arrays ARR1
and ARR2
of size M and N, respectively, merge them into ARR1
as one sorted array. Assume that ARR1
has a size of M + N to hold all ...read more
The task is to merge two sorted arrays into one sorted array.
Create a new array with size M + N to store the merged array
Use two pointers to iterate through the elements of ARR1 and ARR2
Compare the elements at the current pointers and add the smaller element to the new array
Move the pointer of the array from which the element was added
Repeat the process until all elements are merged
If there are remaining elements in ARR2, add them to the new array
Return the merged array
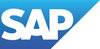
Asked in SAP

Q. Problem: Total Area of Overlapping Rectangles
You are given two rectangles situated on a 2-D coordinate plane, which may overlap. Your task is to compute the total area covered by these rectangles.
Input:
The f...read more
Calculate total area covered by two overlapping rectangles on a 2-D plane.
Calculate the area of each rectangle separately using the given coordinates.
Find the overlapping region by determining the intersection of the two rectangles.
Calculate the area of the overlapping region by multiplying its width and height.
Add the areas of the two rectangles and subtract the area of the overlapping region to get the total area covered.
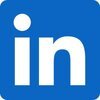
Asked in LinkedIn

Q. Check if Two Trees are Mirror
Given two arbitrary binary trees, your task is to determine whether these two trees are mirrors of each other.
Explanation:
Two trees are considered mirror of each other if:
- The r...read more
Check if two binary trees are mirrors of each other based on specific criteria.
Compare the roots of both trees.
Check if the left subtree of the first tree is the mirror of the right subtree of the second tree.
Verify if the right subtree of the first tree is the mirror of the left subtree of the second tree.
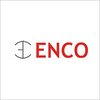
Asked in Enco Engineers Combine

Q. Colorful Knapsack Problem
You are given a set of 'N' stones, each with a specific weight and color. The goal is to fill a knapsack with exactly 'M' stones, choosing one stone of each color, so that the total we...read more
The goal is to fill a knapsack with exactly 'M' stones, one of each color, minimizing unused capacity.
Iterate through stones, keeping track of weights for each color
Sort stones by weight and color, then select one stone of each color
Calculate total weight for each combination and minimize unused capacity
If knapsack cannot be filled, return -1
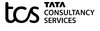
Asked in TCS

Q. Linked List Value Search Problem Statement
Given a Singly Linked List of integers accessible via a head pointer, where each node contains a specific integer value. You are required to determine if a node with a...read more
Given a singly linked list of integers, determine if a specified value exists within the list.
Iterate through the linked list to check if the specified value exists.
Return 1 if the value is found, else return 0.
Handle multiple test cases by looping through each one separately.
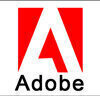
Asked in Adobe

Q. Maximum Sum Path in a Binary Tree Problem Statement
You are provided with a binary tree consisting of N
nodes where each node has an integer value. The task is to determine the maximum sum achievable by a simpl...read more
Find the maximum sum achievable by a simple path between any two nodes in a binary tree.
Traverse the binary tree to find all possible paths and calculate their sums.
Keep track of the maximum sum encountered during traversal.
Consider paths that may include the same node twice.
Implement a recursive function to explore all paths in the tree.
Optimize the solution to avoid redundant calculations.
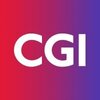
Asked in CGI Group

Q. Remove Vowels from a String
Given a string STR
of length N
, your task is to remove all the vowels from that string and return the modified string.
Input:
The first line of input contains an integer 'T' represen...read more
Remove all vowels from a given string and return the modified string.
Iterate through each character in the string and check if it is a vowel (a, e, i, o, u or A, E, I, O, U).
If the character is not a vowel, add it to the modified string.
Return the modified string after processing all characters.
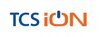
Asked in TCS iON

Q. Minimum Cash Flow Problem
Consider 'N' friends who have borrowed money from one another. They now wish to settle their debts by giving or taking cash among themselves in a way that minimizes the total cash flow...read more
The problem involves settling debts among friends by minimizing total cash flow.
Create a graph where nodes represent friends and edges represent debts.
Calculate the net amount each friend owes or is owed by summing up the debts and credits.
Use a recursive algorithm to settle debts by finding the maximum and minimum amounts owed.
Repeat the process until all debts are settled.
Output the total cash flow required to settle all debts.
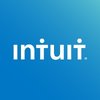
Asked in Intuit

Q. Problem Statement: Delete Node In A Linked List
Given a singly linked list of integers and a reference to a node, your task is to delete that specific node from the linked list. Each node in the linked list has...read more
Delete a specific node from a singly linked list given the reference to the node.
Traverse the linked list to find the node to be deleted.
Update the pointers to skip over the node to be deleted.
Print the modified linked list after deletion.
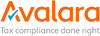
Asked in Avalara Technologies

Q. Queue Using Stacks Implementation
Design a queue data structure following the FIFO (First In First Out) principle using only stack instances.
Explanation:
Your task is to complete predefined functions to suppor...read more
Implement a queue using stacks with enQueue, deQueue, peek, and isEmpty operations.
Use two stacks to simulate a queue - one for enqueueing and one for dequeueing.
For enQueue operation, push elements onto the enqueue stack.
For deQueue operation, if the dequeue stack is empty, transfer elements from enqueue stack to dequeue stack.
For peek operation, return the top element of the dequeue stack.
For isEmpty operation, check if both stacks are empty.
Example: enQueue(5), enQueue(10)...read more
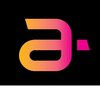
Asked in Amdocs

Q. Swap Two Numbers Problem Statement
Given two integers a
and b
, your task is to swap these numbers and output the swapped values.
Input:
The first line contains a single integer 't', representing the number of t...read more
Swap two numbers 'a' and 'b' and output the swapped values.
Create a temporary variable to store one of the numbers before swapping
Swap the values of 'a' and 'b' using the temporary variable
Output the swapped values as 'b' followed by 'a'
Example: If 'a' = 3 and 'b' = 4, after swapping 'a' will be 4 and 'b' will be 3
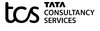
Asked in TCS

Q. Minimise Sum Problem Statement
You are given a matrix of 'N' rows and 'M' columns and a non-negative integer 'K'. Determine the minimum possible sum of all elements in each submatrix after performing at most 'K...read more
Given a matrix and a non-negative integer K, find the minimum possible sum of all elements in each submatrix after performing at most K decrements.
Iterate through all submatrices and find the minimum possible sum after performing decrements
Keep track of the number of decrements performed on each element
Use dynamic programming to optimize the solution
Ensure not to decrease any number below 0
Return the minimum possible sum modulo 10^9 + 7
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
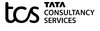
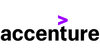
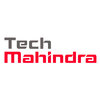
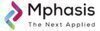
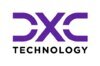
Top Interview Questions for Associate Software Engineer Related Skills
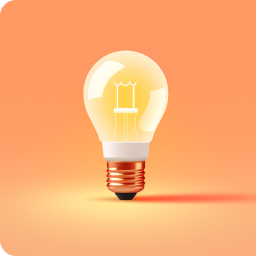
Calculate your in-hand salary
Confused about how your in-hand salary is calculated? Enter your annual salary (CTC) and get your in-hand salary
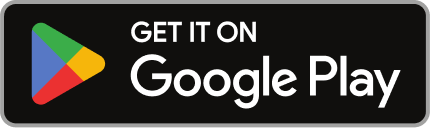
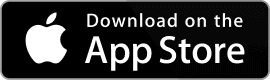
Reviews
Interviews
Salaries
Users
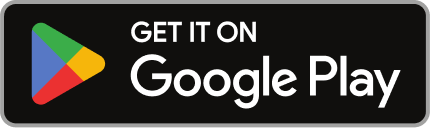
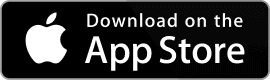