Top 250 Java Interview Questions and Answers
Updated 15 Jul 2025
Asked in Caspex Corp

Q. What is the difference between findFirst and findAny?
findFirst returns the first element in a stream, while findAny returns any element in a stream.
findFirst is deterministic and will always return the first element in a stream, while findAny is non-deterministic and can return any element.
findAny is u...read more
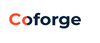
Asked in Coforge

Q. Where is the Observer pattern used in Spring Boot?
The Observer pattern is used in SpringBoot for implementing event handling and notification mechanisms.
The Observer pattern is commonly used in SpringBoot for implementing event listeners and publishers.
It allows objects to subscribe to and receive n...read more
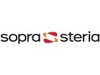
Asked in Sopra Steria

Q. What is Metaspace in Java 8?
Meta space is a memory space in Java 8 used to store class metadata.
Meta space replaces the permanent generation (PermGen) space in Java 8.
It stores class metadata such as class name, access modifiers, and constant pool.
Meta space is dynamically size...read more
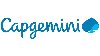
Asked in Capgemini

Q. What is concurrency in Java?
Concurrency in Java refers to the ability of multiple tasks to run simultaneously within a program.
Concurrency allows for better utilization of resources by executing multiple tasks at the same time.
Java provides built-in support for concurrency thro...read more
Asked in Dataquad

Q. How do you handle lazy loading in Hibernate?
Lazy loading in Hibernate is a technique used to load data only when it is needed, improving performance.
Lazy loading is achieved by setting the fetch type to LAZY in the mapping of the entity relationship.
When an entity is loaded, associated entitie...read more
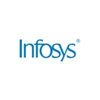
Asked in Infosys

Q. What is an abstract method?
An abstract method is a method that is declared without an implementation in an abstract class or interface.
Abstract methods do not have a body and must be implemented by subclasses.
Abstract classes can have both abstract and non-abstract methods.
Int...read more

Q. Tell me about the Executor framework.
Executor framework is a Java framework that provides a way to execute tasks asynchronously using a thread pool.
It provides a way to manage threads and execute tasks in a thread pool
It allows for better resource management and improved performance
It s...read more
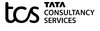
Asked in TCS

Q. what is java and new features of java
Java is a popular programming language known for its platform independence and object-oriented approach. New features include modules, var keyword, and more.
Java is a high-level, class-based, object-oriented programming language.
New features in Java ...read more
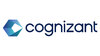
Asked in Cognizant

Q. How do you set the default value of a date field to the current date and time?
To set the default value of a date field to the current date time value, use a script to assign the value.
Create a client script or business rule to set the default value
Use the GlideDateTime API to get the current date time value
Assign the current d...read more
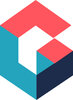
Asked in Genpact

Q. What is the difference between super and super()?
super is a keyword used to access methods and properties of a superclass, while super() is used to call the constructor of a superclass.
super is used to access methods and properties of a superclass in a subclass.
super() is used to call the construct...read more
Java Jobs
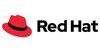
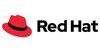
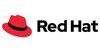
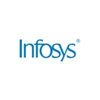
Asked in Infosys and 2 others

Q. What is the use of the super keyword?
The super keyword is used in Java to refer to the immediate parent class object.
Used to access methods and variables of the parent class
Helps in achieving method overriding in inheritance
Can be used to call the constructor of the parent class
Example:...read more
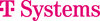
Asked in T-Systems ICT India

Q. Explain Wrapper Class in Java 8.
Wrapper class in Java 8 is used to convert primitive data types into objects.
Wrapper classes provide a way to use primitive data types as objects.
They are used for converting primitive data types into objects and vice versa.
Examples include Integer, ...read more
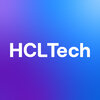
Asked in HCLTech

Q. What is a parallel stream?
Parallel stream is a feature in Java that allows processing elements of a stream concurrently.
Parallel stream can be created from a regular stream using parallel() method.
It utilizes multiple threads to process elements in parallel, improving perform...read more
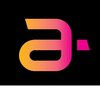
Asked in Amdocs

Q. What is a Spring Actuator?
Spring Actuator is a feature in Spring Boot that allows monitoring and managing the application.
Spring Actuator provides endpoints to monitor application health, metrics, info, etc.
It helps in understanding the internal state of the application and i...read more
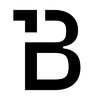
Asked in Backbase

Q. What are Spring bean scopes?
Spring bean scopes define the lifecycle and visibility of beans in a Spring application context.
Singleton: One instance per Spring IoC container. Example: @Bean(name = 'myBean') in a configuration class.
Prototype: A new instance is created each time ...read more
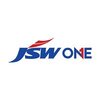
Asked in JSW One Platforms

Q. MVC layers for Java web service
MVC layers in Java web service help separate concerns for better organization and maintainability.
Model layer: Represents the data and business logic
View layer: Handles the presentation of data to the user
Controller layer: Manages user input and upda...read more
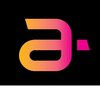
Asked in Amdocs

Q. How would you implement a database connection pool in Java?
Database connection pool in Java helps manage multiple database connections efficiently.
Use a connection pool library like HikariCP or Apache DBCP.
Set up the pool with a maximum number of connections, timeout settings, etc.
Acquire and release connect...read more
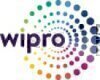
Asked in Wipro

Q. Explain the internal workings of a Java HashMap.
Java HashMap is a data structure that stores key-value pairs and uses hashing to efficiently retrieve values.
HashMap uses an array of buckets to store key-value pairs.
The key is hashed to find the index of the bucket where the value is stored.
If mult...read more
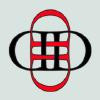
Asked in DigiValet

Q. What are the advantages of using Spring Boot Starters?
Spring Boot starters are dependency descriptors that simplify the dependency management process in Spring Boot applications.
Spring Boot starters provide a set of pre-configured dependencies that can be easily included in your project.
They help in red...read more
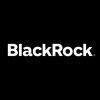
Asked in Blackrock

Q. Java 8 in depth
Java 8 introduced several new features including lambda expressions, streams, and functional interfaces.
Lambda expressions allow for more concise code by enabling functional programming.
Streams provide a way to process collections of objects in a fun...read more
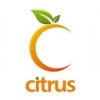
Asked in Citrus Informatics

Q. Design principles in Java.
Design principles in Java focus on creating modular, reusable, and maintainable code.
Single Responsibility Principle - Each class should have only one responsibility.
Open/Closed Principle - Classes should be open for extension but closed for modifica...read more
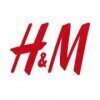
Asked in H&M

Q. Java 8 advantages
Java 8 introduced several new features and improvements, including lambda expressions, functional interfaces, streams, and default methods.
Lambda expressions allow for more concise and readable code.
Functional interfaces enable the use of lambda expr...read more
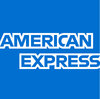
Asked in American Express

Q. How have you leveraged Spring Java in your projects?
I leverage Spring Java for dependency injection, MVC framework, and transaction management in my projects.
Utilize Spring's dependency injection to manage object dependencies and improve code maintainability
Leverage Spring MVC framework for building w...read more
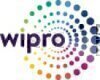
Asked in Wipro

Q. Different technologies available in java
Some technologies available in Java include Java EE, Spring Framework, Hibernate, Apache Struts, and JavaFX.
Java EE (Enterprise Edition) for building enterprise applications
Spring Framework for dependency injection and aspect-oriented programming
Hibe...read more
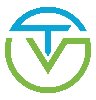
Asked in TechVerito

Q. How to deploy Java apps?
Java applications can be deployed using various methods such as manual deployment, using build tools like Maven or Gradle, or using containerization platforms like Docker.
Use build tools like Maven or Gradle to package the application into a JAR or W...read more
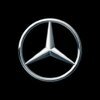

Q. What is the return type for getWindowHandles?
The return type for get window handles is an array of strings.
Return type should be an array of strings
Each string in the array represents a window handle
Example: ['handle1', 'handle2', 'handle3']
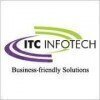
Asked in ITC Infotech

Q. JPA vs Hibernate
JPA is a specification while Hibernate is an implementation of JPA.
JPA is a Java specification for managing relational data in applications.
Hibernate is an ORM framework that implements the JPA specification.
Hibernate provides additional features bey...read more
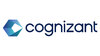
Asked in Cognizant

Q. All Java 8 new features
Java 8 introduced several new features including lambda expressions, functional interfaces, streams, and default methods.
Lambda expressions allow you to pass functionality as an argument to a method.
Functional interfaces have a single abstract method...read more
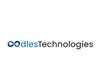
Asked in OodlesTechnologies

Q. How does AWT Token work?
AWT Token is a security token used for authentication and authorization in Java applications.
AWT stands for Abstract Window Toolkit
AWT Token is used for managing user authentication and authorization in Java applications
It provides a secure way to co...read more
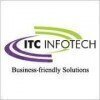
Asked in ITC Infotech

Q. Spring JPA vs Hibernate
Spring JPA is a part of the Spring Data project that makes it easier to work with JPA. Hibernate is a popular ORM framework.
Spring JPA is a higher level abstraction on top of JPA, providing more features and simplifying development.
Hibernate is a pow...read more
Top Interview Questions for Related Skills
Interview Experiences of Popular Companies
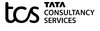
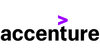
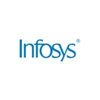
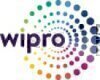
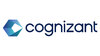
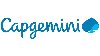
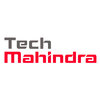
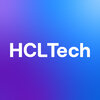
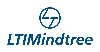
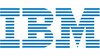
Interview Questions of Java Related Designations
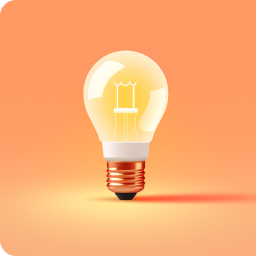
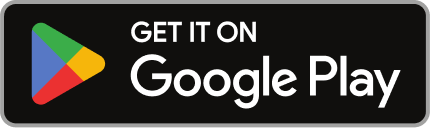
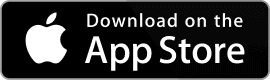
Reviews
Interviews
Salaries
Users
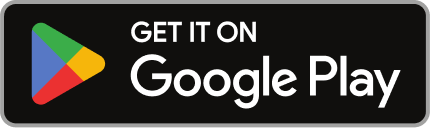
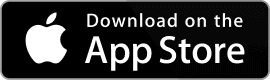