Java Developer
2000+ Java Developer Interview Questions and Answers
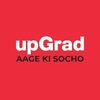
Asked in upGrad

Q. Sort 0 and 1 Problem Statement
Given an integer array ARR
of size N
containing only integers 0 and 1, implement a function to sort this array. The solution should scan the array only once without using any addi...read more
The function sorts an integer array containing only 0s and 1s in linear time complexity.
Use two pointers, one starting from the beginning and the other from the end of the array.
Swap the elements at the pointers if the element at the beginning pointer is 1 and the element at the end pointer is 0.
Continue moving the pointers towards each other until they meet in the middle of the array.
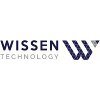
Asked in Wissen Technology

Q. Parent class has run() and walk(). Parent run() calls walk(). Child Class overrides Parent class. Inside run(), it calls super.run(). Walk() calls super.walk(). In the main class, Parent p = new child(); p.run(...
read moreThe order of execution will be: parent's run() -> child's run() -> parent's walk()
When p.run() is called, it will first execute the run() method of the parent class
Inside the parent's run() method, it will call the walk() method of the parent class
Since the child class overrides the parent class, the child's run() method will be executed next
Inside the child's run() method, it will call the run() method of the parent class using super.run()
Finally, the walk() method of the pa...read more
Java Developer Interview Questions and Answers for Freshers
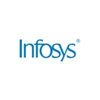
Asked in Infosys

String should be preferred for frequent updates due to its immutability.
String is immutable, so every update creates a new instance, leading to memory overhead.
StringBuffer is mutable and allows for efficient updates without creating new instances.
Use StringBuffer when frequent updates are required to avoid unnecessary memory allocation.
Example: StringBuffer is preferred for building dynamic strings in loops.
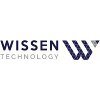
Asked in Wissen Technology

Q. How do you sort a list of students based on their first name?
To sort a list of students based on their first name, use the Collections.sort() method with a custom Comparator.
Create a custom Comparator that compares the first names of the students.
Implement the compare() method in the Comparator to compare the first names.
Use the Collections.sort() method to sort the list using the custom Comparator.
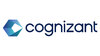
Asked in Cognizant

Q. What array list and linkedlist difference,how hashmap internally working,what is synchronised and it's type,what is difference between abstract and interface,class loading mechanism in Java, you hat is single t...
read moreJava Developer interview questions covering array list, linkedlist, hashmap, synchronization, abstract vs interface, singleton class, Spring framework, database configuration, and Java 8 features.
ArrayList and LinkedList differ in terms of implementation, performance, and usage
HashMap uses hashing to store and retrieve key-value pairs
Synchronized is used to ensure thread safety and prevent race conditions
Abstract classes cannot be instantiated and can have both abstract and n...read more
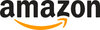
Asked in Amazon

Q. Convert BST to Greater Sum Tree
Given a Binary Search Tree (BST) of integers, your task is to convert it into a greater sum tree. In the greater sum tree, each node's value should be replaced with the sum of al...read more
The task is to convert a Binary Search Tree into a Greater Sum Tree.
Traverse the BST in reverse inorder (right, root, left) to visit nodes in descending order.
Keep track of the sum of all greater nodes encountered so far.
Update the value of each node by adding the sum of greater nodes and update the sum.
Continue this process until all nodes have been visited.
Return the modified BST.
Java Developer Jobs
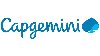
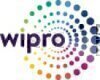
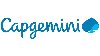
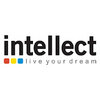
Asked in Intellect Design Arena

Q. Remove Duplicates from a Sorted Array
Given a sorted integer array ARR
of size N
, you need to remove duplicates such that each element appears only once and return the length of this new array.
Input:
The first...read more
Remove duplicates from a sorted array in-place and return the length of the modified array.
Use two pointers approach to track unique elements and duplicates.
Modify the input array in-place without using extra space.
Return the length of the modified array.
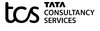
Asked in TCS

Q. what are the difference between abstract class and interface, and throw and throws, and why we use throws?? Why String is Immutable?
Explaining differences between abstract class and interface, throw and throws, and why we use throws. Also, why String is immutable.
Abstract class can have both abstract and non-abstract methods, while interface can only have abstract methods.
A class can implement multiple interfaces, but can only extend one abstract class.
Throw is used to explicitly throw an exception, while throws is used to declare the exceptions that a method may throw.
We use throws to inform the caller o...read more
Share interview questions and help millions of jobseekers 🌟
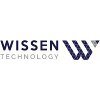
Asked in Wissen Technology

Q. What will happen if hashCode only returns a constant? How will it affect the internal working of the HashMap?
If hashcode returns a constant, all elements will be stored in the same bucket, resulting in poor performance.
All elements will be stored in the same bucket of the HashMap.
This will lead to poor performance as the HashMap will essentially become a linked list.
Retrieving elements will take longer as the HashMap will have to iterate through the linked list to find the desired element.
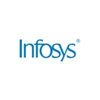
Asked in Infosys

Q. 1.What is Singleton in java and create your own singleton class countering all breakable conditions? 2. What is Auto Configuration? 3. @Primary vs @Qualifier 4. What is idempotent? 5. What is class loader? Type...
read moreThe interview questions cover various topics related to Java development, including Singleton pattern, memory management, exception handling, Spring framework, and design patterns.
Singleton pattern ensures a class has only one instance and provides a global point of access to it.
Auto Configuration in Spring Boot automatically configures the Spring application based on dependencies present in the classpath.
@Primary annotation is used to give higher preference to a bean when mu...read more
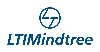
Asked in LTIMindtree

Q. Longest Harmonious Subsequence Problem Statement
Determine the longest harmonious subsequence within a given array of integers, where the difference between the maximum and minimum elements of the subsequence i...read more
Find the longest harmonious subsequence in an array where the difference between max and min elements is 1.
Iterate through the array and count the frequency of each element.
For each element, check if the count of element+1 is greater than 0, if so, update the length of the harmonious subsequence.
Return the maximum length found.
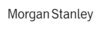
Asked in Morgan Stanley

Q. Max Element After Update Operations
Given an array A
of size N
, initialized with all zeros, and another array ARR
of M
pairs of integers representing operations. Each operation consists of a range where each el...read more
Find the maximum element in an array after performing a series of increment operations on specified ranges.
Initialize an array of size N with all zeros
Iterate through the operations and increment elements within specified ranges
Return the maximum element in the array after all operations
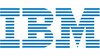
Asked in IBM

Q. 1. Abstraction vs Inheritance 2. What is Garbage collector? 3. What is class loader? 4. Spring security 5. Scopes of bean 6. Wait , notify and notify all 7. Class level vs object level local 8. Arraylist vs Lin...
read moreJava interview questions covering topics like abstraction, inheritance, garbage collector, class loader, Spring security, bean scopes, wait-notify, class vs object level local, ArrayList vs LinkedList, Singleton class, and RestController vs Controller.
Abstraction focuses on hiding implementation details while inheritance allows a class to inherit properties and methods from another class.
Garbage collector is a program that automatically frees up memory by deleting objects tha...read more
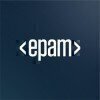
Asked in EPAM Systems

Q. Explain OOPS Concept? What is Polymorphism and Types of polymorphism? Write Code for compile time and Run time Polymorphism? What are singleton class and factory method? What is Exception and Exception Hierarch...
read moreJava interview questions on OOPS concepts, polymorphism, exceptions, data structures, and threading.
OOPS concepts include encapsulation, inheritance, and polymorphism.
Polymorphism refers to the ability of an object to take on multiple forms.
Singleton class is a class that can only have one instance, while factory method is a method that creates objects.
Exception is an error that occurs during program execution, with a hierarchy of exceptions.
Volatile keyword is used to indica...read more
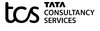
Asked in TCS

Q. What are the main OOPS concepts in Java, and can you explain them?
Java OOPS concepts include inheritance, polymorphism, encapsulation, and abstraction.
Inheritance allows a class to inherit properties and methods from another class.
Polymorphism allows objects to take on multiple forms and behave differently based on their context.
Encapsulation hides the implementation details of a class and only exposes necessary information.
Abstraction allows for the creation of abstract classes and interfaces that can be implemented by other classes.
Exampl...read more

Asked in CitiusTech

Q. Word Frequency Counter
Given a string 'S' consisting of words, your task is to calculate how many times each word appears in the string. A word is a series of one or more non-space characters. In 'S', words can...read more
Count the frequency of each word in a given string.
Split the string by spaces to get individual words.
Use a hashmap to store each word and its frequency.
Iterate through the words and update the hashmap accordingly.
Print out the words and their frequencies in any order.
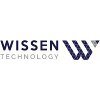
Asked in Wissen Technology

Q. Write a program to return the length of the longest word from a string whose length is even.
This program returns the length of the longest word from a string whose length is even.
Split the string into an array of words using a space as the delimiter.
Initialize a variable to store the length of the longest even word.
Iterate through each word in the array and check if its length is even.
If the length is even and greater than the current longest even word length, update the variable.
Finally, return the length of the longest even word.
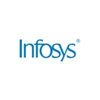
Asked in Infosys

Q. Write code to filter out loans with an incomplete status using Java 8 features.
Code to filter out loans with incomplete status using Java 8 features.
Use stream() method to convert the list of loans into a stream
Use filter() method to filter out loans with incomplete status
Use collect() method to collect the filtered loans into a new list
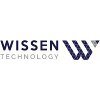
Asked in Wissen Technology

Q. Write a query to find the names of authors who have written more than 10 books given a table named Bookauthor with columns Book and Author.
Query to find authors who have written more than 10 books
Use the GROUP BY clause to group the records by author
Use the HAVING clause to filter the groups with a count greater than 10
Join the Bookauthor table with the Author table to get the author names
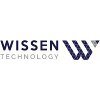
Asked in Wissen Technology

Q. If a class contains a synchronized method M1() with a Thread.Sleep(500) call, and two different objects of this class are created, how will threads behave when Ob1.M1() and Ob2.M1() are invoked? Will they execu...
read moreSynchronized methods ensure that only one thread can execute the method at a time per object.
Synchronized methods lock the object instance, preventing concurrent access.
When Ob1.M1() is called, it locks the instance of the object Ob1.
If Ob2.M1() is called on a different instance, it does not block Ob1's execution.
Both threads will execute M1() one after the other, but independently for each object.
Example: If Ob1 calls M1() and sleeps, Ob2 can still call M1() without waiting.
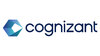
Asked in Cognizant

Q. write program fibonacci series, write program using boolean, write class cast exceptn, singleton design pattern(like you have to jvm one have jdk 1.7 and other have jdk 1.8 how many instance created if one then...
read moreThis interview question covers various topics including programming, design patterns, data structures, and exception handling in Java.
Write a program to generate the Fibonacci series
Write a program using boolean variables
Explain the ClassCastException and how to handle it
Discuss the Singleton design pattern and its implementation with different JDK versions
Explain abstract classes and interfaces in your current project
Explain hashing in HashMap and the number of buckets
Differ...read more
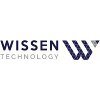
Asked in Wissen Technology

Q. Can we use an object of a Class as a key in a HashMap?
Yes, we can keep an object of a Class as a key in HashMap.
The key in a HashMap can be any non-null object.
The key's class must override the equals() and hashCode() methods for proper functioning.
If two objects have the same hashCode(), they are stored in the same bucket and equals() is used to find the correct key-value pair.
If the key is mutable, its state should not be modified in a way that affects its hashCode() or equals() methods after it is added to the HashMap.
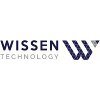
Asked in Wissen Technology

Q. If we have a mutable object inside an immutable class, how will you handle it?
To handle a mutable object inside an immutable class, make the object private and provide only read-only access methods.
Make the mutable object private to prevent direct modification
Provide read-only access methods to retrieve the object's state
If necessary, create defensive copies of the object to ensure immutability
Avoid exposing any mutator methods that modify the object's state
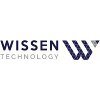
Asked in Wissen Technology

Q. 3. What if we do not override Equals method ? What is we do not override Hashcode method?
Not overriding equals method can lead to incorrect comparison of objects. Not overriding hashCode method can lead to incorrect behavior in hash-based data structures.
If equals method is not overridden, the default implementation from Object class will be used which compares object references.
This can lead to incorrect comparison of objects where two different objects with same content are considered unequal.
If hashCode method is not overridden, the default implementation from...read more
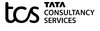
Asked in TCS

Q. What is the use of private variables if they are accessible via getters and setters from another class?
Private variables provide encapsulation and data hiding.
Private variables can only be accessed within the class they are declared in, providing encapsulation and preventing direct modification from outside the class.
Getters and setters provide controlled access to private variables, allowing for validation and manipulation of the data before it is accessed or modified.
For example, a class may have a private variable for a person's age, but the getter can return a calculated a...read more
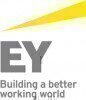
Asked in Ernst & Young

Java is platform independent because it can run on any system with JVM, which is platform dependent due to its need to interact with the underlying hardware.
Java code is compiled into bytecode, which can be executed on any system with a JVM installed.
JVM acts as an intermediary between the Java code and the underlying hardware of the system.
JVM is platform dependent because it needs to interact with the specific hardware and operating system of the system it is running on.
Exa...read more
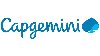
Asked in Capgemini

Q. Is Java platform-independent, and if so, why?
Yes, Java is platform-independent because of its 'write once, run anywhere' principle.
Java programs are compiled into bytecode, which can be executed on any platform with a Java Virtual Machine (JVM).
The JVM acts as an interpreter, translating the bytecode into machine code specific to the underlying platform.
This allows Java programs to run on different operating systems and hardware architectures without modification.
For example, a Java program developed on a Windows machin...read more
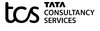
Asked in TCS

Q. 1. What is JDK, JVM, JRE.
JDK is a development kit, JRE is a runtime environment, and JVM is a virtual machine for executing Java code.
JDK includes JRE and development tools like javac and java
JRE includes JVM and necessary libraries to run Java applications
JVM is responsible for interpreting Java bytecode and executing it
Example: JDK 8 includes JRE 8 and development tools like javac and java
Example: JRE 8 includes JVM 8 and necessary libraries to run Java applications
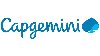
Asked in Capgemini

Q. //design patterns //markers interface // example of functional interface . can you override Default method. Can you directly or do you need object. give some examples of functional interface //one try catch fin...
read moreThe interview questions cover a wide range of topics including design patterns, exception handling, Hibernate, MongoDB, Java collections, Spring framework, and microservices.
Design patterns like markers interface, functional interface, and Singleton pattern are important in Java development.
Understanding exception handling with try-catch-finally blocks is crucial for handling errors in Java applications.
Knowing the differences between load and get in Hibernate, as well as the...read more
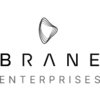
Asked in Brane Enterprises

Static variables and methods belong to the class rather than instances of the class.
Static variables are shared among all instances of a class.
Static methods can be called without creating an instance of the class.
Example: public static int count = 0; public static void incrementCount() { count++; }
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
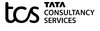
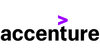
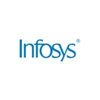
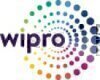
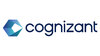
Top Interview Questions for Java Developer Related Skills
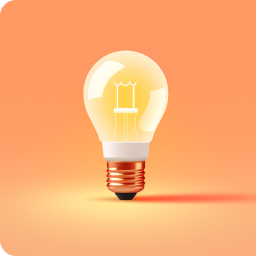
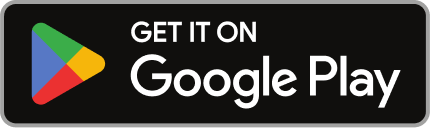
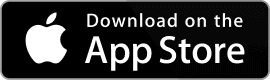
Reviews
Interviews
Salaries
Users
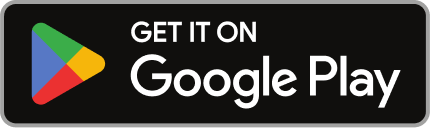
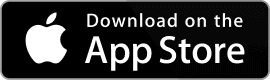