Java Developer
300+ Java Developer Interview Questions and Answers for Freshers
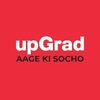
Asked in upGrad

Q. Sort 0 and 1 Problem Statement
Given an integer array ARR
of size N
containing only integers 0 and 1, implement a function to sort this array. The solution should scan the array only once without using any addi...read more
The function sorts an integer array containing only 0s and 1s in linear time complexity.
Use two pointers, one starting from the beginning and the other from the end of the array.
Swap the elements at the pointers if the element at the beginning pointer is 1 and the element at the end pointer is 0.
Continue moving the pointers towards each other until they meet in the middle of the array.
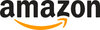
Asked in Amazon

Q. Convert BST to Greater Sum Tree
Given a Binary Search Tree (BST) of integers, your task is to convert it into a greater sum tree. In the greater sum tree, each node's value should be replaced with the sum of al...read more
The task is to convert a Binary Search Tree into a Greater Sum Tree.
Traverse the BST in reverse inorder (right, root, left) to visit nodes in descending order.
Keep track of the sum of all greater nodes encountered so far.
Update the value of each node by adding the sum of greater nodes and update the sum.
Continue this process until all nodes have been visited.
Return the modified BST.
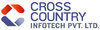
Asked in Cross Country Infotech

Q. Besides inheritance, what other mechanisms can you use to connect two classes?
Interfaces can be used to connect two classes in Java.
Interfaces define a contract that implementing classes must adhere to.
A class can implement multiple interfaces.
Interfaces can be used to achieve loose coupling and abstraction.
Example: Connecting a Car class with a Fuelable interface.
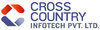
Asked in Cross Country Infotech

Q. Do you have any Java certifications?
No, I don't have any Java certificate.
No, I don't possess any Java certification.
Unfortunately, I haven't obtained any Java certificate.
Regrettably, I do not hold any Java certification.
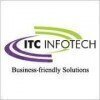
Asked in ITC Infotech

Q. How do you change the background color of a div element?
Use CSS to change the background color of a div element.
Use the 'background-color' property in CSS to specify the color.
You can use color names, hex codes, RGB values, or HSL values to set the background color.
Example: div { background-color: blue; }
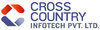
Asked in Cross Country Infotech

Q. When is memory allocated: when a class is created, when an instance is created, or at another time?
Memory is allocated when an instance of a class is created.
Memory is allocated dynamically when an object is instantiated.
Each instance of a class has its own memory allocation.
Memory allocation occurs at runtime, not during class creation.
Java Developer Jobs
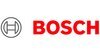
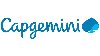
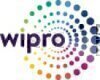
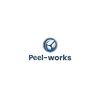
Asked in Peel-works

Q. What is the difference between a local variable, a static variable, and an instance variable?
Local, static, and instance variables differ in their scope and lifetime.
Local variables are declared inside a method and have a limited scope.
Static variables belong to the class and are shared among all instances.
Instance variables are unique to each object and can be accessed using the object reference.
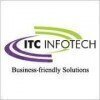
Asked in ITC Infotech

Q. What is a session in Java?
Session in Java is a way to store information about a user across multiple requests.
Session is used to maintain stateful information about a user.
It is created when a user first accesses a web application and remains active until the user logs out or the session times out.
Session data is stored on the server and can be accessed by multiple requests from the same user.
It is commonly used for user authentication, shopping carts, and personalization.
The HttpSession interface in ...read more
Share interview questions and help millions of jobseekers 🌟

Q. How do we call stored procedures in Java?
Stored procedures in Java can be called using JDBC API.
Use the CallableStatement interface to call stored procedures
Register the OUT parameters using the registerOutParameter method
Set the IN parameters using the setXXX methods
Execute the stored procedure using the execute method
Retrieve the OUT parameters using the getXXX methods

Q. What are the implicit objects in JSP servlets?
Implicit objects in JSP servlets are predefined objects that can be accessed without any declaration or initialization.
Implicit objects are automatically available in JSP servlets without the need for explicit declaration or initialization.
These objects provide access to various functionalities and information related to the JSP environment.
Some commonly used implicit objects include request, response, session, application, out, pageContext, and exception.
For example, the req...read more
Asked in Asite Solutions

Q. What is java? What is oops? Difference between hashmap and hashset
Java is a high-level programming language known for its portability and object-oriented programming support. OOPs stands for Object-Oriented Programming. HashMap and HashSet are both data structures in Java, but HashMap is used to store key-value pairs while HashSet is used to store unique elements.
Java is a high-level programming language used for developing applications and software.
OOPs (Object-Oriented Programming) is a programming paradigm based on the concept of objects...read more
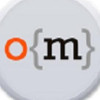
Asked in Orange Mantra

Q. In Tr what is exception handling.oops, difference between abstraction and encapsulation about project.
The question is about exception handling, OOPs, and the difference between abstraction and encapsulation in a project.
Exception handling is a mechanism to handle runtime errors and prevent program termination.
Abstraction is the process of hiding implementation details and showing only necessary information to the user.
Encapsulation is the process of binding data and methods together in a single unit.
In a project, abstraction can be achieved by using interfaces and abstract cl...read more
Asked in Asite Solutions

Q. Can we use a try block without a catch block?
Yes, we can use try block without catch block to handle exceptions using finally block.
Try block can be used without catch block if there is a finally block to handle exceptions.
Finally block is executed whether an exception is thrown or not.
Example: try { // code that may throw exception } finally { // code to be executed regardless of exception }
Asked in Acutech Solutions

Q. What is an object in Java?
An object in Java is an instance of a class that encapsulates data and behavior.
Objects have state and behavior
They are created from classes
They can be used to represent real-world entities or concepts
Objects can interact with each other through method calls
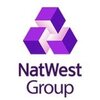
Asked in NatWest Group

Q. What are the differences between HashMap and Hashtable?
HashMap and Hashtable are both used to store key-value pairs, but there are some differences.
HashMap is not synchronized, while Hashtable is synchronized.
HashMap allows null values and one null key, while Hashtable does not allow null values or keys.
HashMap is generally preferred over Hashtable for non-thread-safe applications.
HashMap is faster than Hashtable as it is not synchronized.
Example: HashMap
map = new HashMap<>();

Q. What is the difference between an Interface and a Class?
An interface is a blueprint for classes, defining methods that must be implemented. A class is a blueprint for objects.
An interface can only have abstract methods, while a class can have both abstract and concrete methods.
A class can be instantiated to create objects, while an interface cannot be instantiated.
A class can extend only one class, but it can implement multiple interfaces.
Interfaces are used to achieve multiple inheritance in Java.
Classes can have fields, construc...read more
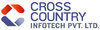
Asked in Cross Country Infotech

Q. What are the different types of Access Modifiers in Java?
Access modifiers in Java control the visibility and accessibility of classes, methods, and variables.
There are four types of access modifiers in Java: public, private, protected, and default.
Public access modifier allows unrestricted access from any class or package.
Private access modifier restricts access to only within the same class.
Protected access modifier allows access within the same class, package, and subclasses.
Default access modifier (no keyword) restricts access t...read more
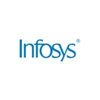
Asked in Infosys

Q. What is the difference between an Abstract class and an Interface?
Abstract class is a class that cannot be instantiated and can have both abstract and non-abstract methods. Interface is a blueprint for classes to implement.
Abstract class can have constructors, while interface cannot.
A class can extend only one abstract class, but can implement multiple interfaces.
Abstract class can have instance variables, while interface cannot.
Abstract class can provide default implementation for methods, while interface cannot.
Interfaces are used to achi...read more

Asked in Tech Vedika

Q. Explain the concept of method overloading in Java, including how to create multiple methods in a class with the same name but different argument types and lengths.
Method overloading allows creating multiple methods with the same name but different arguments.
Method signature must differ in number, type, or order of parameters
Return type can be different but not the only difference
Example: public void print(int num), public void print(String str), public void print(int[] arr)
Overloading improves code readability and reusability
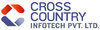
Asked in Cross Country Infotech

Q. What is the difference between composition and inheritance?
Composition is a way to combine objects to create complex structures, while inheritance is a way to create new classes based on existing ones.
Composition is a 'has-a' relationship, where an object contains other objects as its parts.
Inheritance is an 'is-a' relationship, where a subclass inherits properties and behaviors from its superclass.
Composition allows for greater flexibility and modularity in design, as objects can be easily swapped out or added.
Inheritance can lead t...read more
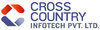
Asked in Cross Country Infotech

Q. Can a subclass access private methods of a parent class?
No, subclass cannot access private methods of the parent class.
Private methods in a class are only accessible within that class.
Subclasses can only access public and protected methods of the parent class.
Private methods are not inherited by subclasses.

Asked in HighRadius

Q. What are the different types of collections?
Different types of collections in Java include List, Set, Queue, and Map.
List: Ordered collection that allows duplicate elements (e.g. ArrayList, LinkedList)
Set: Unordered collection that does not allow duplicate elements (e.g. HashSet, TreeSet)
Queue: Collection that holds elements prior to processing (e.g. LinkedList, PriorityQueue)
Map: Collection that maps unique keys to values (e.g. HashMap, TreeMap)
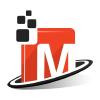
Asked in MobiTrail

Q. What is super keyword ? What is inheritance ? What is abstract and why it is used? And many more questions was asked......?
Answers to common Java interview questions on super keyword, inheritance, and abstract classes.
The super keyword is used to call a method or constructor in the parent class.
Inheritance is a mechanism in which one class acquires the properties and methods of another class.
Abstract classes are classes that cannot be instantiated and are used as a base for other classes to inherit from.
Abstract methods are methods that do not have a body and must be implemented by the subclass.
P...read more
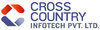
Asked in Cross Country Infotech

Q. Can a subclass inherit the properties of a parent class if the parent class is not loaded?
No, a subclass cannot inherit the properties of a parent class if the parent class is not loaded.
In Java, inheritance allows a subclass to inherit the properties and methods of its parent class.
However, for a subclass to inherit the properties of its parent class, the parent class must be loaded.
If the parent class is not loaded, the subclass will not have access to its properties.
To ensure that a subclass can inherit the properties of its parent class, the parent class must ...read more
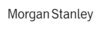
Asked in Morgan Stanley

Q. What is the difference between HashMap and HashSet?
HashMap is a key-value pair collection while HashSet is a set of unique elements.
HashMap allows duplicate values but not duplicate keys
HashSet does not allow duplicate values
HashMap implements Map interface while HashSet implements Set interface
HashMap uses put() method to add elements while HashSet uses add() method
Example: HashMap<String, Integer> map = new HashMap<>(); HashSet<String> set = new HashSet<>();
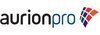
Asked in Aurionpro Solutions

Q. What is Hibernate, and how can we use it with Spring Boot?
Hibernate is an ORM framework for Java that simplifies database interactions, and it integrates seamlessly with Spring Boot.
Hibernate is an Object-Relational Mapping (ORM) tool that maps Java objects to database tables.
It provides a framework for managing database operations using Java objects, reducing boilerplate code.
Spring Boot simplifies the configuration of Hibernate through application properties.
Example: Use @Entity annotation to define a model class, and @Repository ...read more
Asked in Asite Solutions

Q. How do you define a Thread in Java?
Thread in Java is a lightweight sub-process that executes a set of instructions independently.
Thread can be defined by extending the Thread class or implementing the Runnable interface.
Thread class provides various methods to control the execution of threads like start(), sleep(), join(), etc.
Runnable interface has only one method run() which needs to be implemented.
Threads can be created and started using the start() method.
Example: public class MyThread extends Thread { pub...read more
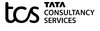
Asked in TCS

Q. What is the difference between throw and throws?
throw is used to explicitly throw an exception, while throws is used to declare that a method may throw an exception.
throw is used within a method to throw an exception explicitly
throws is used in the method signature to declare that the method may throw an exception
throw is followed by an instance of an exception class
throws is followed by the list of exception classes that the method may throw
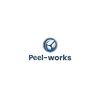
Asked in Peel-works

Q. Explain the internal implementation of HashSet. Since we only put values in HashSet, how does HashSet internally use HashMap?
HashSet uses HashMap internally to store unique elements with null values allowed.
HashSet is backed by a HashMap, where each element is stored as a key.
The value associated with each key in HashMap is a constant (usually 'PRESENT').
HashSet allows only unique elements; duplicates are ignored based on the hash code.
Example: Adding 'A' and 'B' to HashSet results in two entries in HashMap.
HashSet allows one null value, which is stored as a key in HashMap.
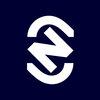
Asked in Nofinite

Q. What is HTML?
HTML stands for HyperText Markup Language, used for creating and structuring web pages.
HTML is a markup language used to create the structure of web pages.
It consists of elements enclosed in tags, such as <html>, <head>, <body>.
Attributes can be added to elements to provide additional information or functionality, like <img src='image.jpg'>.
HTML is the foundation of web development and is often used in conjunction with CSS and JavaScript.
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
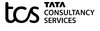
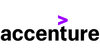
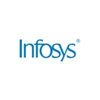
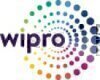
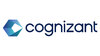
Top Interview Questions for Java Developer Related Skills
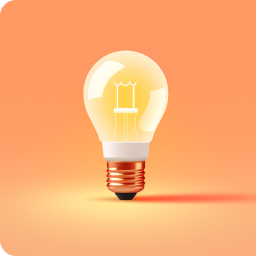
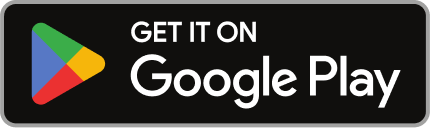
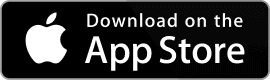
Reviews
Interviews
Salaries
Users
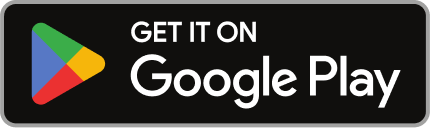
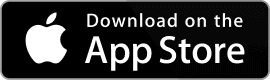