Top 30 C# Interview Questions and Answers
Updated 7 Jul 2025
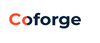
Asked in Coforge

Q. Why override the Equals method?
Overriding Equals method helps to compare objects based on their values rather than their references.
Default implementation of Equals method compares object references
Overriding Equals method allows custom comparison based on object values
Helps in im...read more

Asked in UnitedHealth

ViewData, ViewBag, and TempData are ways to pass data between controllers and views in ASP.NET MVC.
ViewData is a dictionary object used to pass data from controller to view. It requires typecasting.
ViewBag is a dynamic property used to pass data from...read more
Asked in Scaffolded Edtech

Q. How many projects have you completed using C#?
I have completed 5 projects in C# including a web application, a desktop application, and a game.
Developed a web application using ASP.NET MVC
Created a desktop application for data management
Designed a game using Unity and C# scripting
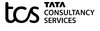
Asked in TCS

Q. What is the difference between .NET and C#?
C# is a programming language while .NET is a framework that supports multiple languages including C#.
C# is a programming language developed by Microsoft.
.NET is a framework developed by Microsoft that supports multiple languages including C#.
C# is us...read more
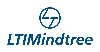
Asked in LTIMindtree

Q. How do you handle errors in C#?
Error handling in c# involves using try-catch blocks to handle exceptions and ensure graceful handling of errors.
Use try-catch blocks to catch exceptions and handle errors gracefully.
Use specific exception types to catch specific errors.
Use finally b...read more
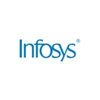
Asked in Infosys

Q. Baics Of C#
C# is a modern, object-oriented programming language developed by Microsoft.
C# is used to develop Windows desktop applications, games, mobile apps, and web applications.
It is strongly typed and supports both value and reference types.
C# uses a syntax...read more
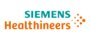
Asked in Siemens Healthineers

Q. What is the difference between == and .Equals() in C#?
The '==' operator checks if two values are equal, while 'Equal' is a method that checks if two objects are equal.
The '==' operator is used to compare values of primitive types like int, float, etc.
The 'Equal' method is used to compare objects of refe...read more
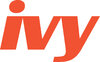
Asked in ivy

Q. Write a program using C#.
A C# program to demonstrate basic concepts like variables, loops, and conditionals.
Use 'int' for integers: int number = 5;
Implement loops: for (int i = 0; i < 5; i++) { Console.WriteLine(i); }
Use conditionals: if (number > 0) { Console.WriteLine('Pos...read more
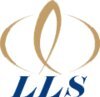
Asked in Lakshmi Life Sciences

Q. Have you used indexers?
Yes, I have used indexer extensively in my previous projects.
I have used indexer to access elements in arrays and lists.
I have also used it to access properties and methods of objects.
Indexer has helped me to write more concise and readable code.
For ...read more
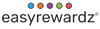
Asked in EasyRewardz Software Services

Q. What is the purpose of the yield keyword?
Yield keyword is used in C# to return a sequence of values one at a time.
Yield keyword is used in iterator methods to return each element one by one.
It allows the method to return multiple values without needing to store them all in memory.
Yield retu...read more
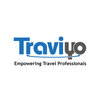
Asked in TraviYo

Q. What are the differences between Transient, AddScoped, and AddSingleton?
Transient creates a new instance every time it is requested, Scoped creates a new instance per scope, Singleton creates a single instance for the entire application.
Transient: Creates a new instance every time it is requested.
AddScoped: Creates a new...read more
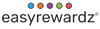
Asked in EasyRewardz Software Services

Q. What is the lock keyword?
Lock keyword is used in C# to synchronize access to a shared resource by allowing only one thread to enter a critical section at a time.
Used to prevent multiple threads from accessing a shared resource simultaneously
Helps in avoiding race conditions ...read more
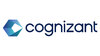
Asked in Cognizant

Q. What are the basic C# concepts?
Basic C# concepts include data types, variables, loops, conditional statements, classes, and methods.
Data types in C# include int, string, bool, double, etc.
Variables are used to store data and can be declared using var keyword.
Loops like for, while,...read more
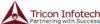
Asked in Tricon Infotech

Q. What are indexers in C#?
Indexers in C# allow objects to be indexed like arrays, providing a way to access elements using an index value.
Indexers are defined using 'this' keyword followed by square brackets containing the index parameters.
They can be used to get or set the v...read more
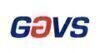
Asked in GAVS Technologies

Q. What are manageable codes in .NET?
Manageable codes in dot net are codes that are easy to understand, maintain, and debug.
Well-structured and organized code
Proper commenting and documentation
Consistent naming conventions
Modular design with reusable components
Avoiding complex nested lo...read more
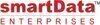
Asked in smartData Enterprises

Q. What is the difference between configure and configure services?
The difference between configure and configure services is that configure is a general term for setting up or adjusting something, while configure services specifically refers to setting up or adjusting services.
Configure: General term for setting up...read more
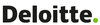
Asked in Deloitte

Q. What are const and readonly?
const and readonly are keywords used in programming to define variables that cannot be modified.
const is used to declare a constant variable that cannot be changed after initialization
readonly is used to declare a variable that can only be assigned a...read more
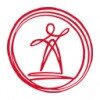
Asked in Prime Focus Technologies

Q. Explain the basics of C#.
C# is a programming language developed by Microsoft for building a wide range of applications on the .NET framework.
C# is an object-oriented language with features like classes, inheritance, and polymorphism.
It is strongly typed, meaning variables mu...read more
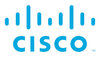
Asked in Cisco

Q. What is a namespace in C#?
Namespace in C# is a way to organize and group related classes, interfaces, structs, enums, and delegates.
Namespaces help in avoiding naming conflicts by providing a way to uniquely identify classes.
They also help in organizing code and making it mor...read more
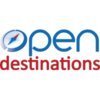
Asked in Open Destinations

Q. Tell me about the latest C# 10 features.
C# 10 introduces features like file-scoped namespaces, global using directives, and interpolated string handlers.
File-scoped namespaces allow defining namespaces at the file level instead of wrapping everything in a namespace block.
Global using direc...read more
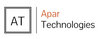
Asked in Apar Technologies

Q. How proficient are you in C#?
Proficient in coding in C# with experience in developing various applications.
Experienced in using C# for developing web applications, desktop applications, and APIs.
Familiar with object-oriented programming concepts and design patterns in C#.
Knowled...read more
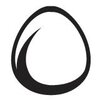
Asked in ContractPodAi

Q. What is the 'using' keyword in C#?
The 'using' keyword in C# is used to define a scope in which an object is created and used, and automatically disposes of the object when the scope is exited.
Used for managing resources like files, database connections, etc.
Ensures that the object is...read more
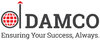
Asked in Damco Solutions

Q. Which version of Visual C# have you worked on?
I work on Visual C# 2019.
I primarily work on Visual C# 2019 for my software development projects.
I have experience with Visual C# 2017 and earlier versions as well.
Visual C# 2019 offers new features and improvements over previous versions.
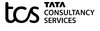
Asked in TCS

Q. What is C# and what are its uses?
C# is a programming language developed by Microsoft for building a wide range of applications on the .NET framework.
C# is widely used for developing desktop applications, web applications, mobile apps, games, and more.
It is an object-oriented languag...read more
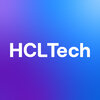
Asked in HCLTech

Q. What is the difference between constant and readonly?
Constants are compile-time constants, while readonly variables can be assigned a value at runtime but cannot be changed after initialization.
Constants are declared using the 'const' keyword, while readonly variables are declared using the 'readonly' ...read more
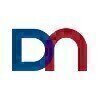
Asked in Diebold Nixdorf

Q. How would you rate your proficiency in C#?
I was rated highly in C# language due to my strong understanding of object-oriented programming principles and experience with various C# frameworks.
Strong understanding of object-oriented programming principles
Experience with various C# frameworks s...read more
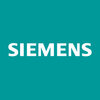
Asked in Siemens

Q. What is new in C# 10?
C# 10 introduces new features like file-scoped namespaces, global using directives, and interpolated string handlers.
File-scoped namespaces allow defining namespaces at the file level instead of wrapping everything in a namespace block.
Global using d...read more
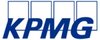
Asked in KPMG India

Q. What is the difference between .ToString() and Convert.ToString()?
Convert.ToString() is a static method that handles null values, while .ToString() is an instance method that may throw a NullReferenceException.
Convert.ToString() is a static method that can handle null values and return an empty string if the input ...read more
Asked in Wealden Computing Services

Q. What is the difference between var and dynamic keywords?
var and dynamic are both used for type inference in C#, but var is statically typed while dynamic is dynamically typed.
var is used to declare a variable with an inferred type at compile-time.
dynamic is used to declare a variable with a type that is r...read more
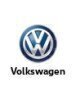
Asked in Volkswagen

Q. What are the differences between ref and out parameters?
ref and out are keywords in C# used for passing arguments to methods by reference.
ref keyword is used to pass arguments by reference, allowing the method to modify the value of the argument
out keyword is similar to ref, but the argument does not have...read more
Top Interview Questions for Related Skills
Interview Experiences of Popular Companies
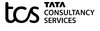
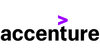
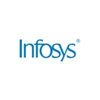
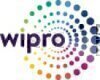
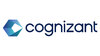
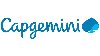
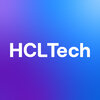
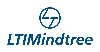
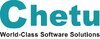

Interview Questions of C# Related Designations
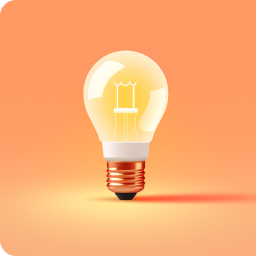
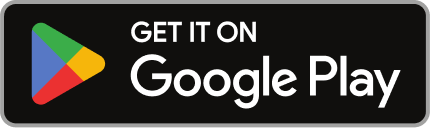
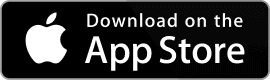
Reviews
Interviews
Salaries
Users
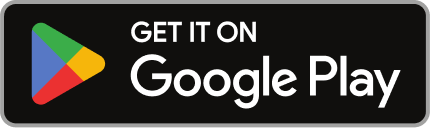
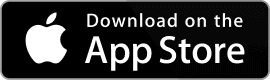