Software Engineering Specialist
90+ Software Engineering Specialist Interview Questions and Answers
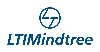
Asked in LTIMindtree

Q. Explain Python Data Structures and advantages and some differences in each
Python data structures are containers that hold data in an organized manner, each with its own advantages and differences.
Python lists are versatile and can hold different data types.
Tuples are immutable and can be used as keys in dictionaries.
Sets are unordered and eliminate duplicate elements.
Dictionaries store data in key-value pairs for efficient retrieval.
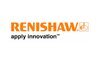
Asked in Renishaw

Q. find if point is inside shape if there are multiple shapes intersecting any better solution? how will you store if there are multiple points on a single line with better data structure
Determine if a point is inside multiple intersecting shapes using efficient data structures.
Use a spatial data structure like a QuadTree or R-tree to store shapes for efficient querying.
For point-in-shape tests, use bounding boxes to quickly eliminate shapes that cannot contain the point.
For multiple points on a line, consider using a sorted list or a balanced tree structure to maintain order and allow efficient searching.
Example: In a QuadTree, each node can represent a regi...read more
Software Engineering Specialist Interview Questions and Answers for Freshers
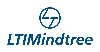
Asked in LTIMindtree

Q. List - Stores all types of elements Array - Stores one type of element and in sequential memory blocks hence faster access
An array stores one type of element in sequential memory blocks for faster access.
Arrays are useful for storing and accessing data in a specific order.
They can be used to store numbers, characters, or other data types.
Arrays can be multidimensional, allowing for more complex data structures.
Accessing elements in an array is done using an index, starting at 0.
Arrays can be resized dynamically in some programming languages.
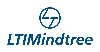
Asked in LTIMindtree

Q. What are analytical functions, what is the difference between rank and dens_rank, different types of joins, what is the difference between view and materialized view, different types of refresh methods in Mview...
read moreAnalytical functions are used to perform calculations across a set of rows. Rank assigns a unique rank to each row, while dens_rank assigns consecutive ranks.
Analytical functions are used to perform calculations across a set of rows in a table.
Rank function assigns a unique rank to each row based on the specified order.
Dens_rank function assigns consecutive ranks to rows, leaving no gaps between ranks.
Types of joins include inner join, outer join, left join, right join, etc.
A...read more
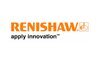
Asked in Renishaw

Q. DLL vs standard library how does DLL works? how executable know which DLL to load and does it unload them after
DLLs are dynamic libraries that allow executables to share code and resources, loaded at runtime.
DLL stands for Dynamic Link Library, which contains code and data that can be used by multiple programs.
An executable specifies which DLLs to load through import tables in the PE (Portable Executable) format.
Windows uses a process called 'binding' to resolve DLL dependencies at runtime, ensuring the correct version is loaded.
DLLs can be loaded explicitly using functions like LoadL...read more
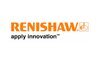
Asked in Renishaw

Q. Given a grid and a small group size to mesh that grid, where there are multiple points on the grid, find which group has the maximum points.
Identify the group in a grid with the highest concentration of points based on a specified group size.
Grid Representation: The grid can be represented as a 2D array where each cell contains a point or is empty.
Group Size: Define the size of the group (e.g., 2x2, 3x3) to analyze the grid for maximum points.
Sliding Window Technique: Use a sliding window approach to traverse the grid and calculate the number of points in each group.
Example: In a 5x5 grid with points at (0,0), (0...read more
Software Engineering Specialist Jobs
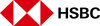
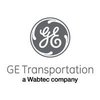
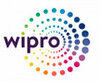
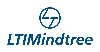
Asked in LTIMindtree

Q. What is the word count problem, and how can it be solved using Java 8 streams and the collect method?
The word count problem involves counting the frequency of words in a given text.
Use Java 8 streams to split the text into words, map each word to a key-value pair with word as key and count as value, and then collect the results using groupingBy and counting collectors.
Example: Stream.of(text.split("\\s+")).collect(Collectors.groupingBy(Function.identity(), Collectors.counting()));
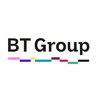
Asked in BT Group

Q. Design a system that processes a large volume of records daily, considering scalability, performance, and cost efficiency.
Design a scalable, high-performance, and cost-efficient system for processing large volumes of records daily.
Utilize distributed computing to handle high volume of records efficiently
Implement data partitioning to distribute workload evenly across multiple nodes
Use caching mechanisms to improve performance and reduce latency
Optimize data storage by choosing appropriate database technologies like NoSQL or columnar databases
Implement auto-scaling to dynamically adjust resources...read more
Share interview questions and help millions of jobseekers 🌟
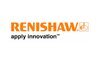
Asked in Renishaw

Q. Explain runtime polymorphism when a child pointer points to a parent object, both with and without virtual functions. How do you expose methods across DLLs?
Explains runtime polymorphism with virtual functions and DLL method exposure.
Runtime polymorphism allows a child class to override methods of a parent class.
Using virtual functions enables dynamic binding, allowing child class methods to be called through parent pointers.
Without virtual functions, the parent class method is called, leading to potential loss of child class functionality.
Example: If 'Base' has a virtual method 'show()', and 'Derived' overrides it, calling 'show...read more
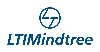
Asked in LTIMindtree

Q. What are map, filter, and reduce in JavaScript?
Map, filter and reduce are higher-order functions in JavaScript used to manipulate arrays.
Map transforms each element of an array into a new array based on a provided function.
Filter creates a new array with all elements that pass a test implemented by a provided function.
Reduce applies a function to each element of an array and reduces the array to a single value.
These functions are commonly used in functional programming and can make code more concise and readable.
Example: ...read more
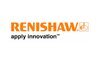
Asked in Renishaw

Q. what's abstraction and why we need it? How to achieve it
Abstraction simplifies complex systems by hiding unnecessary details, allowing focus on high-level functionality.
Abstraction helps manage complexity by reducing the amount of information to process.
In programming, abstraction can be achieved through interfaces and abstract classes.
Example: A car's dashboard provides controls (steering, pedals) without revealing engine mechanics.
In software design, using APIs allows developers to interact with services without knowing their in...read more
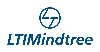
Asked in LTIMindtree

Q. Explain the implementation of Generators and Decorators in Python.
Generators and decorators are powerful features in Python for creating iterable objects and modifying functions respectively.
Generators are functions that use the yield keyword to return a sequence of values lazily.
They are memory efficient as they generate values on the fly instead of storing them in memory.
Decorators are functions that modify the behavior of other functions without changing their source code.
They are used to add functionality to functions, such as logging, ...read more
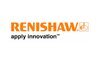
Asked in Renishaw

Q. Why do we need to pass an object by reference in a copy constructor? Why is it kept as constant?
Passing objects by reference in copy constructors avoids unnecessary copying and maintains const correctness.
1. Efficiency: Passing by reference avoids the overhead of copying large objects, improving performance.
2. Const correctness: Keeping the parameter as const ensures the original object is not modified during the copy process.
3. Example: In a class 'MyClass', a copy constructor can be defined as 'MyClass(const MyClass &obj)' to pass by reference.
4. Memory management: He...read more
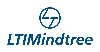
Asked in LTIMindtree

Q. Features of Tosca. Api scan why used. Authentication how to validate
Tosca is a test automation tool that supports API testing, enhancing efficiency and accuracy in software testing processes.
API Scanning: Tosca can automatically scan APIs to identify endpoints, methods, and parameters, streamlining the testing process.
Authentication Validation: Tosca supports various authentication methods (e.g., OAuth, Basic Auth) to ensure secure access to APIs during testing.
Test Case Design: Users can create test cases for API interactions, validating res...read more
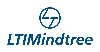
Asked in LTIMindtree

Q. What does API integration with a CI/CD pipeline mean? Explain in two lines.
API with CI/CD pipeline integration automates the deployment and testing of APIs, ensuring faster and more reliable software delivery.
Automated Testing: CI/CD pipelines can run automated tests on APIs to ensure functionality and performance before deployment.
Continuous Deployment: Changes to the API can be automatically deployed to production, reducing manual intervention and speeding up release cycles.
Version Control: Integration with version control systems allows tracking ...read more
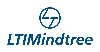
Asked in LTIMindtree

Q. What are Spring Boot annotations and how do they relate to object scopes?
Spring Boot annotations are used to simplify the development process by providing metadata to the Spring framework.
Annotations like @Component, @Service, @Repository are used to define object scopes in Spring Boot.
Annotations like @Scope can be used to specify the scope of a bean, such as singleton or prototype.
Annotations like @RequestScope, @SessionScope are used to define object scopes based on HTTP request or session.
Annotations like @Configuration, @Bean are used to conf...read more
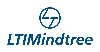
Asked in LTIMindtree

Q. How is Axios different from the Fetch API?
Axios is a third-party library that provides more features and flexibility than the fetch API.
Axios allows for easy cancellation of requests.
Axios has built-in support for handling request and response interceptors.
Axios can handle request and response transformations.
Axios has a larger community and more documentation than fetch API.
Fetch API is built into modern browsers and requires no additional installation or setup.
Fetch API has a simpler syntax than Axios.
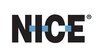
Asked in NICE

Q. Create a React component to fetch data.
Create a React component to fetch and display data from an API using hooks.
Use the useEffect hook to fetch data when the component mounts.
Utilize the useState hook to manage the fetched data and loading state.
Handle errors gracefully using try-catch within the fetch function.
Example: const [data, setData] = useState([]);
Example: useEffect(() => { fetchData(); }, []);
Example: async function fetchData() { const response = await fetch(url); setData(await response.json()); }
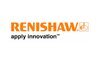
Asked in Renishaw

Q. What is the difference between overloading and overriding in programming?
Overloading allows multiple methods with the same name but different parameters; overriding replaces a method in a subclass.
Overloading occurs within the same class, while overriding involves a subclass and its superclass.
Example of overloading: A method 'add(int a, int b)' and 'add(double a, double b)'.
Example of overriding: A subclass 'Dog' overrides a method 'sound()' from superclass 'Animal'.
Overloading is resolved at compile time (static polymorphism), while overriding i...read more
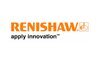
Asked in Renishaw

Q. What's copy constructor? What's shallow & deep copy
A copy constructor creates a new object as a copy of an existing object, enabling shallow or deep copying of data.
A copy constructor is a special constructor in C++ used to create a new object as a copy of an existing object.
Shallow copy duplicates the object's immediate values, but not the objects they point to, leading to shared references.
Deep copy duplicates all objects and values, creating entirely independent copies, preventing shared references.
Example of shallow copy:...read more
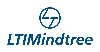
Asked in LTIMindtree

Q. Synchronisation why used. Execution list how to approve
Synchronization is essential in software to manage concurrent processes and ensure data consistency across threads.
Data Consistency: Synchronization prevents data corruption by ensuring that only one thread can access shared resources at a time.
Thread Safety: Using synchronization mechanisms like locks or semaphores ensures that critical sections of code are executed by one thread at a time.
Deadlock Prevention: Proper synchronization strategies help avoid deadlocks, where two...read more
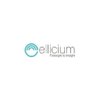
Asked in Ellicium Solutions

Q. What is the difference between a list and an array?
List is dynamic and can be modified while array is fixed in size and cannot be modified.
List can grow or shrink dynamically while array has a fixed size.
List can contain elements of different data types while array can only contain elements of the same data type.
List uses more memory than array due to its dynamic nature.
Array is faster than list for accessing elements by index.
Example: List - [1, 'hello', True], Array - [1, 2, 3]
Example: List - ['apple', 'banana', 'orange'], ...read more
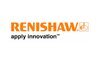
Asked in Renishaw

Q. Different types of constructor? How does move constructor works?
Constructors initialize objects; types include default, parameterized, copy, and move constructors.
Default Constructor: Initializes objects with default values. Example: `MyClass obj;`
Parameterized Constructor: Initializes objects with specific values. Example: `MyClass obj(5);`
Copy Constructor: Creates a new object as a copy of an existing object. Example: `MyClass obj2 = obj1;`
Move Constructor: Transfers resources from a temporary object to a new object, avoiding deep copie...read more
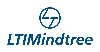
Asked in LTIMindtree

Q. How can you implement database concepts using Python?
Python provides various libraries and modules to interact with databases, such as SQLite, MySQL, and PostgreSQL.
Python's standard library includes the sqlite3 module for working with SQLite databases.
For MySQL, the popular library is mysql-connector-python, which provides an interface to interact with MySQL databases.
psycopg2 is a widely used library for connecting to PostgreSQL databases in Python.
ORM (Object-Relational Mapping) libraries like SQLAlchemy can be used to abstr...read more
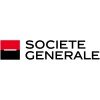

Q. How to work in pressurized environment. Handling priority production bugs
To work in a pressurized environment and handle priority production bugs, one must prioritize tasks, communicate effectively, stay calm under pressure, and seek help when needed.
Prioritize tasks based on their impact on the production environment
Communicate effectively with team members and stakeholders to understand the urgency and impact of the bugs
Stay calm under pressure and avoid making hasty decisions
Break down complex problems into smaller, manageable tasks
Seek help fr...read more
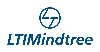
Asked in LTIMindtree

Q. Can you provide examples of when to use subqueries?
Subqueries enhance SQL queries by allowing nested queries for complex data retrieval.
Subqueries can be used in SELECT statements to filter results. Example: SELECT * FROM employees WHERE salary > (SELECT AVG(salary) FROM employees);
They can also be used in WHERE clauses to compare values. Example: SELECT name FROM departments WHERE id IN (SELECT department_id FROM employees);
Subqueries can be used in INSERT statements to add data based on existing data. Example: INSERT INTO n...read more
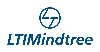
Asked in LTIMindtree

Q. Any one Object Oriented Database
MongoDB is an open-source document database that provides high performance, scalability, and flexibility.
MongoDB is a NoSQL database that stores data in flexible, JSON-like documents.
It supports a rich query language and provides features like indexing, replication, and sharding.
MongoDB is widely used in web applications, big data processing, and real-time analytics.
Example: MongoDB is used by companies like Uber, Airbnb, and LinkedIn.
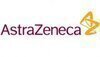
Asked in AstraZeneca

Q. What is MDM?
MDM stands for Master Data Management. It is a process that ensures the consistency and accuracy of an organization's critical data.
MDM is a method of managing and organizing data to create a single, reliable source of truth.
It involves creating and maintaining a central repository of master data, which includes information about customers, products, suppliers, etc.
MDM helps in eliminating data duplication, improving data quality, and providing a unified view of data across d...read more
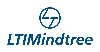
Asked in LTIMindtree

Q. What is @Qualifier, @value in springboot, transient, volatile
Annotations and keywords used in Spring Boot and Java
The @Qualifier annotation is used in Spring to specify which bean should be autowired when multiple beans of the same type are present
The @Value annotation is used in Spring to inject values from properties files or environment variables into Spring beans
The transient keyword in Java is used to indicate that a field should not be serialized when the object is converted to a byte stream
The volatile keyword in Java is used to...read more
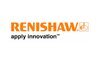
Asked in Renishaw

Q. Can you overload the new and delete operators?
Yes, you can overload new and delete operators in C++ for custom memory management.
Overloading allows custom allocation strategies.
Example: Custom new operator can log memory allocations.
Example: Custom delete operator can track deallocations.
Syntax: void* operator new(size_t size) and void operator delete(void* pointer).
Can be useful for debugging memory leaks or optimizing performance.
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
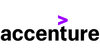
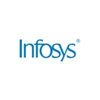
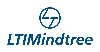
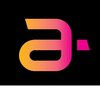
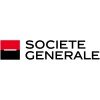
Top Interview Questions for Software Engineering Specialist Related Skills
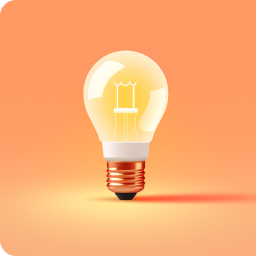
Calculate your in-hand salary
Confused about how your in-hand salary is calculated? Enter your annual salary (CTC) and get your in-hand salary
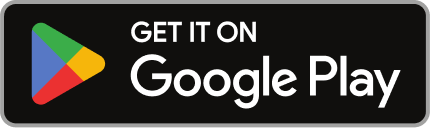
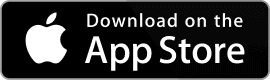
Reviews
Interviews
Salaries
Users
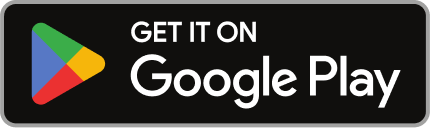
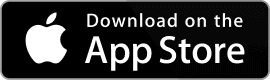