Python Developer
50+ Python Developer Interview Questions and Answers for Freshers
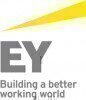
Asked in Ernst & Young

Q. How do you convert a .txt file to a .csv file using Python?
To convert a .txt file into a .csv file in Python, you can use the csv module.
Import the csv module
Open the .txt file in read mode
Open a new .csv file in write mode
Use csv.reader to read the .txt file line by line
Use csv.writer to write each line to the .csv file
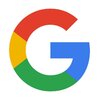
Asked in Google

Q. 6.What are local variables and global variables in Python? 7.When to use a tuple vs list vs dictionary in Python? 8.Explain some benefits of Python 9.What is Lambda Functions in Python? 10.What is a Negative In...
read moreLocal variables are variables that are defined within a function and can only be accessed within that function. Global variables are variables that are defined outside of any function and can be accessed throughout the program.
Local variables are created when a function is called and destroyed when the function completes.
Global variables can be accessed and modified by any function in the program.
Using local variables helps in encapsulation and prevents naming conflicts.
Globa...read more
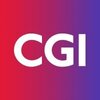
Asked in CGI Group

Q. What is the difference between Python arrays and lists?
Arrays are homogeneous data structures while lists are heterogeneous data structures in Python.
Arrays are fixed in size while lists are dynamic.
Arrays can only contain elements of the same data type while lists can contain elements of different data types.
Arrays are faster and more memory-efficient for numerical operations.
Lists have more built-in functions and are more versatile for general-purpose programming.
Example of array: import array as arr; a = arr.array('i', [1, 2, ...read more
Asked in Spectromax Technology Solutions

Q. If we decide to change the domain, should we dedicate 3-6 months to learning about the new domain to achieve our goals effectively?
Agree, taking time to learn the new domain is crucial for success.
Learning the new domain will help us understand the business requirements better.
It will also help us identify potential challenges and opportunities.
We can leverage our existing skills and knowledge to build better solutions.
Taking time to learn the new domain will also help us build credibility with stakeholders.
We can use this time to network and build relationships with experts in the new domain.
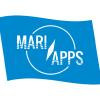
Asked in MariApps Marine Solutions

Q. define class and functions.why functions are written inside class.what is the role of a class
A class is a blueprint for creating objects that have properties and methods. Functions are written inside a class to define its behavior.
A class is a user-defined data type that encapsulates data and functions that operate on that data.
Functions written inside a class are called methods and are used to define the behavior of the class.
The role of a class is to provide a template for creating objects that share common properties and behavior.
Classes allow for code reusability...read more
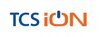
Asked in TCS iON

Q. What is the difference between lists and tuples in Python?
Lists are mutable while tuples are immutable in Python.
Lists use square brackets [] while tuples use parentheses ().
Elements in a list can be added, removed, or modified while tuples cannot be modified.
Lists are used for collections of homogeneous items while tuples are used for heterogeneous items.
Lists are generally used for large collections of data while tuples are used for smaller collections or for data that should not be changed.
Lists are slower than tuples in terms of...read more
Python Developer Jobs
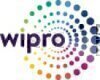

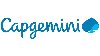
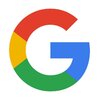
Asked in Google

Q. 11.What is the namespace in Python? 12.What is a dictionary in Python? 13.What is type conversion in Python? 14.What is the difference between Python Arrays and lists? 15.What are functions in Python?
Answers to common Python interview questions.
Namespace is a container for storing variables and functions.
Dictionary is a collection of key-value pairs.
Type conversion is the process of converting one data type to another.
Arrays are homogeneous while lists are heterogeneous.
Functions are blocks of code that perform a specific task.
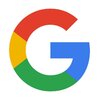
Asked in Google

Q. 1. What is Python? 2. What Are Python Advantages? 3. Why do you we use in python Function? 4. What is the break Statement? 5. What is tuple in python?
Python is a high-level, interpreted programming language known for its simplicity, readability, and versatility.
Python is used for web development, data analysis, artificial intelligence, and more.
Advantages of Python include its ease of use, large standard library, and community support.
Functions in Python are used to group related code and make it reusable.
The break statement is used to exit a loop prematurely.
A tuple is an ordered, immutable collection of elements.
Share interview questions and help millions of jobseekers 🌟
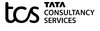
Asked in TCS

Q. What is Python?
Python is a high-level programming language known for its simplicity and readability.
Python is an interpreted language, which means it does not need to be compiled before running.
It has a large standard library that provides many useful modules and functions.
Python supports multiple programming paradigms, including procedural, object-oriented, and functional programming.
It is widely used in web development, data analysis, artificial intelligence, and scientific computing.
Pyth...read more
Asked in Six Phrase

Q. What is the difference between a list and a tuple in programming?
Lists are mutable, ordered collections; tuples are immutable, ordered collections in Python.
Mutability: Lists can be modified (e.g., list.append(4)), while tuples cannot (e.g., tuple[0] = 1 raises an error).
Syntax: Lists use square brackets (e.g., my_list = [1, 2, 3]), while tuples use parentheses (e.g., my_tuple = (1, 2, 3)).
Performance: Tuples are generally faster than lists for iteration due to their immutability.
Use Cases: Lists are used for collections of items that may ...read more
Asked in Adshi5.com

Q. What is the difference between the append and extend methods in Python lists?
append adds a single element, while extend adds multiple elements from an iterable to a list.
append(obj): Adds 'obj' as a single element to the end of the list. Example: lst.append(4) results in [1, 2, 3, 4].
extend(iterable): Adds each element from 'iterable' to the list. Example: lst.extend([4, 5]) results in [1, 2, 3, 4, 5].
Use append when you want to add one item; use extend when you want to merge another iterable into the list.
Both methods modify the original list in plac...read more
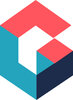
Asked in Genpact

Q. What is the difference between a tester and a developer?
Testers focus on finding defects while developers focus on creating software.
Testers are responsible for testing the software and finding defects.
Developers are responsible for creating the software and fixing defects.
Testers use test cases and scenarios to ensure the software meets requirements.
Developers use programming languages and tools to create software.
Testers work closely with developers to ensure defects are fixed.
Developers work closely with testers to understand a...read more
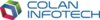
Asked in Colan Infotech

Q. Which data type is mutable in Python: list, tuple, string, int, or float?
Lists are mutable in Python.
Lists can be modified after creation, allowing for addition, removal, and modification of elements.
Tuples, strings, integers, and floats are immutable in Python.
Example: list_example = [1, 2, 3]; list_example[1] = 5 # modifies the list element at index 1 to 5.
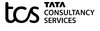
Asked in TCS

Q. What is the difference between a list and a tuple?
Lists are mutable while tuples are immutable.
Lists are enclosed in square brackets [], while tuples are enclosed in parentheses ().
Elements in a list can be added, removed or modified, while elements in a tuple cannot be modified.
Lists are used for collections of homogeneous items, while tuples are used for collections of heterogeneous items.
Lists are generally used for sequences that need to be modified frequently, while tuples are used for sequences that are accessed freque...read more
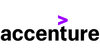
Asked in Accenture

Q. What are lambda functions in Python?
Lambda functions are anonymous functions that can be defined in a single line of code.
Lambda functions are defined using the lambda keyword.
They can take any number of arguments, but can only have one expression.
They are often used as arguments for higher-order functions.
Example: lambda x: x**2 defines a lambda function that squares its input.
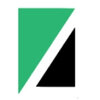
Asked in Zvolv

Q. Design a CRUD App which supports ASYNC and AWAIT Operations in FastAPI.
Design a CRUD App with AYSNC and AWAIT Operations in Fast API
Use FastAPI framework for building the CRUD operations
Implement async and await keywords for asynchronous operations
Utilize SQLAlchemy for database operations
Include endpoints for Create, Read, Update, and Delete operations
Asked in Spectromax Technology Solutions

Q. how to build our-self for technology needs?
To build ourselves for technology needs, we need to constantly learn and adapt to new technologies and trends.
Stay updated with the latest technologies and trends in the industry
Attend conferences, workshops, and webinars to learn new skills
Practice coding regularly and work on personal projects to improve skills
Collaborate with other developers and participate in open source projects
Read blogs, articles, and books related to technology
Be open to learning new programming lang...read more
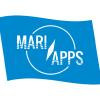
Asked in MariApps Marine Solutions

Q. How do you reverse a dictionary in Python?
Reverse a dictionary in Python
Create a new dictionary with values as keys and keys as values
Use dictionary comprehension to create the new dictionary
If there are duplicate values, use a list comprehension to create a list of keys
Use the built-in function 'reversed' to reverse the order of the keys or values
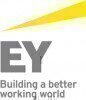
Asked in Ernst & Young

Q. List and tuple difference
Lists and tuples are both sequence data types in Python, but they have some key differences.
Lists are mutable, meaning their elements can be changed, added, or removed, while tuples are immutable.
Lists are defined using square brackets [], while tuples are defined using parentheses ().
Lists are typically used for collections of similar items, while tuples are used for heterogeneous data.
Lists have more built-in methods for manipulation, such as append(), extend(), and remove(...read more
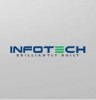
Asked in Infotech

Q. What is a while loop?
A while loop is a control flow statement that allows code to be executed repeatedly based on a condition.
The loop continues until the condition becomes false
The condition is checked before each iteration
The loop body must include a way to modify the condition to avoid an infinite loop
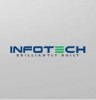
Asked in Infotech

Q. What is reang
reang is not a recognized term in Python development.
reang is not a Python keyword, module, or function.
There is no known reference or documentation for reang in Python.
It is possible that reang is a typo or a mispronunciation of another term.
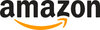
Asked in Amazon

Q. What is the Python programming language?
Python is a high-level programming language known for its simplicity and readability.
Python is an interpreted language, meaning it does not need to be compiled before running.
It has a large standard library that provides many pre-built functions and modules.
Python supports multiple programming paradigms, including procedural, object-oriented, and functional programming.
It is widely used in web development, data analysis, artificial intelligence, and scientific computing.
Pytho...read more
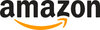
Asked in Amazon

Q. What is a set in Python?
A set is an unordered collection of unique elements in Python.
Sets are created using curly braces or the set() function.
Sets do not allow duplicate elements.
Sets can perform mathematical operations like union, intersection, difference, and symmetric difference.
Sets are mutable, meaning you can add or remove elements from a set.
Sets are useful for removing duplicates from a list or checking for membership of an element.
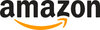
Asked in Amazon

Q. How many keywords are there in Python?
Python has 35 keywords.
Python has a set of reserved words that cannot be used as variable names or identifiers.
These keywords are used to define the syntax and structure of the Python language.
Examples of Python keywords include 'if', 'else', 'for', 'while', 'def', 'class', 'import', etc.
Asked in Spectromax Technology Solutions

Q. Can we change the domain ?
Yes, we can change the domain by updating the DNS records and configuring the web server accordingly.
Update the DNS records to point to the new domain
Configure the web server to recognize the new domain
Redirect the old domain to the new domain to avoid broken links
Update any links or references to the old domain in the codebase
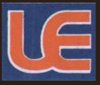
Asked in Unique Enterprises

Q. Nothing to worry Do you oopa concept? Do you know python?
Yes, I am familiar with object-oriented programming concepts and have experience with Python.
Yes, I am familiar with object-oriented programming concepts such as classes, objects, inheritance, and polymorphism.
I have experience using Python for various projects and tasks.
I understand the principles of encapsulation, abstraction, and inheritance in Python.
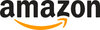
Asked in Amazon

Q. What are the benefits of using Python?
Python benefits include simplicity, versatility, and a large community support.
Python is easy to learn and read, making it a great language for beginners.
Python has a wide range of applications, from web development to data analysis and machine learning.
Python has a large and active community, providing extensive documentation, libraries, and frameworks.
Python's simplicity and readability contribute to faster development and easier maintenance of code.
Python supports multiple...read more
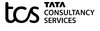
Asked in TCS

Q. What is a suite in Python?
A suite in Python is a group of statements that are executed together as a single block.
Suites are used in control structures like if, while, for, etc.
Suites are defined by their indentation level.
Suites can contain one or more statements.
Suites can also be empty.
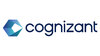
Asked in Cognizant

Q. Why is Python used in real-world applications?
Python is used in reality due to its simplicity, versatility, and wide range of applications.
Python is easy to learn and read, making it a popular choice for beginners.
It has a large standard library and many third-party modules, allowing for rapid development.
Python is used in web development, data analysis, machine learning, scientific computing, and more.
It is cross-platform and can run on various operating systems.
Python is also used in automation, scripting, and testing....read more
Asked in V-Count

Q. What is a for loop?
A for loop is a control flow statement that iterates over a sequence of elements and executes a block of code for each element.
A for loop is used to iterate over a sequence such as a list, tuple, or string.
It consists of an initialization, condition, and increment/decrement statement.
The loop continues until the condition is false.
The code block inside the loop is executed for each element in the sequence.
Example: for item in my_list: print(item)
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
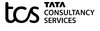
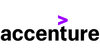
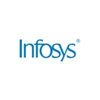
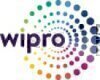
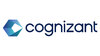
Top Interview Questions for Python Developer Related Skills
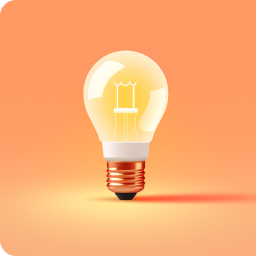
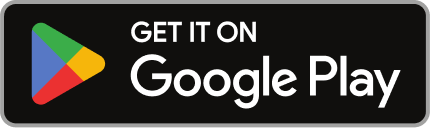
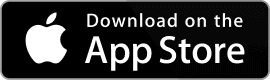
Reviews
Interviews
Salaries
Users
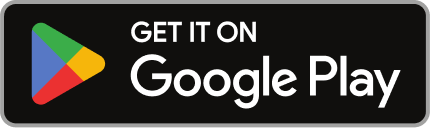
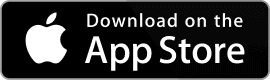