Node JS Developer
100+ Node JS Developer Interview Questions and Answers
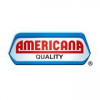
Asked in Americana Group

Q. What is Node.js, and how does it differ from other runtime environments?
Node.js is a runtime environment that allows you to run JavaScript code outside of a web browser.
Node.js is built on the V8 JavaScript engine from Google Chrome.
It uses an event-driven, non-blocking I/O model that makes it lightweight and efficient.
Node.js is commonly used for building server-side applications, APIs, and real-time web applications.
It has a large ecosystem of open-source libraries and frameworks, such as Express.js and Socket.io.
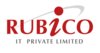
Asked in RubicoIT

Q. Given an array of numbers, write code to extract the even numbers into a new array.
Extract even numbers from an array and store them in a new array using JavaScript.
Use the filter() method to create a new array with even numbers. Example: [1, 2, 3, 4].filter(num => num % 2 === 0) returns [2, 4].
The filter() method iterates through each element and applies a condition. If the condition is true, the element is included in the new array.
You can also use a for loop to achieve the same result. Initialize an empty array and push even numbers into it.
Example using...read more
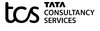
Asked in TCS

Q. What is encapsulation?
Encapsulation is the process of hiding internal implementation details and exposing only necessary information to the outside world.
Encapsulation helps in achieving data abstraction and data hiding.
It allows for better control over the data by preventing direct access to it.
Encapsulation promotes code reusability and maintainability.
In Node.js, encapsulation can be achieved using modules and closures.
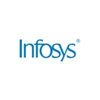
Asked in Infosys

Q. What are the differences between NodeJS and JavaScript?
Node.js is a runtime environment for executing JavaScript code outside of a web browser.
Node.js is built on the V8 JavaScript engine, while JavaScript is a programming language.
Node.js allows JavaScript to be run on the server-side, while JavaScript is primarily used for client-side scripting.
Node.js provides additional features and APIs for server-side development, such as file system access and networking capabilities.
JavaScript is used for web development, creating interac...read more
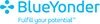
Asked in Blue Yonder

Q. What is the difference between NoSQL and SQL Database?
NoSQL databases are non-relational databases that do not require a fixed schema, while SQL databases are relational databases that use structured query language.
NoSQL databases are schema-less, allowing for flexible data models.
SQL databases use a fixed schema with tables and rows.
NoSQL databases are horizontally scalable, making them suitable for big data applications.
SQL databases are vertically scalable, meaning they can only handle increased load by increasing the power o...read more
Asked in NeoGenCode Technologies

Q. Write a program to get the index and its value from an array. For example, if arr = ["o", "s", "m", "b", "a"], the expected result is res = {0: "o", 1:"s", 2:"m", 3:"b", 4:"a"}.
This program converts an array of strings into an object with indices as keys and values from the array.
Use a loop to iterate through the array.
For each element, assign the index as the key and the element as the value in a new object.
Example: For arr = ['a', 'b', 'c'], the result will be {0: 'a', 1: 'b', 2: 'c'}.
Node JS Developer Jobs
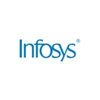
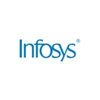
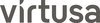
Asked in Venus Associates

Q. Is there any difference between JavaScript and Node.js?
JavaScript is a programming language, while Node.js is a runtime environment for executing JavaScript on the server side.
JavaScript is primarily used for client-side scripting in web browsers.
Node.js allows JavaScript to be run on the server, enabling backend development.
JavaScript runs in the browser's JavaScript engine (like V8 in Chrome).
Node.js uses the V8 engine to execute JavaScript code outside the browser.
JavaScript is event-driven and supports asynchronous programmin...read more
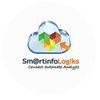
Asked in SmartinfoLogiks

Q. Describe the schema you would create for a form with a submit button that saves data to a database and a cancel button.
Create a form schema with submit and cancel buttons for database interaction.
Define the form fields: e.g., name, email, age.
Use a library like Mongoose for MongoDB to create a schema.
Implement a POST route to handle form submission.
Add a cancel button that redirects to a previous page or clears the form.
Ensure validation for form inputs before submission.
Share interview questions and help millions of jobseekers 🌟
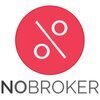
Asked in NoBroker

Q. How can a linked list be created without utilizing arrays?
A linked list can be created without utilizing arrays by defining a Node class with a value and a reference to the next node.
Define a Node class with a value and a reference to the next node
Create instances of the Node class and link them together by setting the next node reference
Implement methods to add, remove, and traverse the linked list
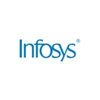
Asked in Infosys

Q. What is a callback function in NodeJS?
A callback function in NodeJS is a function that is passed as an argument to another function and is executed later.
Callback functions are commonly used in asynchronous programming in NodeJS.
They allow us to handle the result of an asynchronous operation once it is completed.
Callback functions can be defined inline or as separate named functions.
They are often used with functions like setTimeout, readFile, and database queries.
Callback functions can accept parameters to pass ...read more
Asked in Revyrie Global

Q. What do you know about NodeJS?
NodeJS is a server-side JavaScript runtime built on Chrome's V8 JavaScript engine.
NodeJS allows developers to build scalable and high-performance applications.
It uses an event-driven, non-blocking I/O model that makes it lightweight and efficient.
NodeJS has a large and active community with a vast number of open-source modules available.
It is commonly used for building web applications, APIs, real-time chat applications, and more.
NodeJS can be easily integrated with other tec...read more
Asked in SalesHandy

Q. What are the differences between public, private, and protected access modifiers?
Public, private, and protected are access modifiers used in object-oriented programming to control the visibility of class members.
Public: accessible from outside the class
Private: only accessible within the class
Protected: accessible within the class and its subclasses
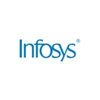
Asked in Infosys

Q. How have you implemented Docker and Kubernetes?
I implemented Docker and Kubernetes to containerize and orchestrate my NodeJS applications.
I used Docker to create containers for my NodeJS applications, ensuring consistency and portability.
I wrote Dockerfiles to define the environment and dependencies for each application.
I used Docker Compose to define and manage multi-container applications.
I used Kubernetes to orchestrate and manage the deployment, scaling, and monitoring of my NodeJS applications.
I created Kubernetes de...read more
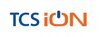
Asked in TCS iON

Q. What are the differences between MySQL and NoSQL?
MySql is a relational database management system while NoSql is a non-relational database management system.
MySql is table-based and uses structured query language (SQL) for querying data.
NoSql is document-based, key-value pairs, wide-column, or graph-based and does not require a fixed schema.
MySql is suitable for complex queries and transactions, while NoSql is better for large amounts of unstructured data and horizontal scalability.
Examples of MySql include Oracle, SQL Serv...read more
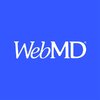
Asked in WebMD

Q. How can Node.js handle multiple threads?
Node.js is single-threaded, but can handle multiple threads using child processes or worker threads.
Use child processes to run multiple instances of Node.js
Use worker threads for CPU-intensive tasks
Leverage the cluster module to create a pool of worker processes
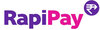
Asked in RapiPay

Q. Explain other sorting methods with examples, excluding built-in functions.
Other methods of sorting include bubble sort, selection sort, and insertion sort.
Bubble sort: repeatedly steps through the list, compares adjacent elements, and swaps them if they are in the wrong order.
Selection sort: repeatedly finds the minimum element from the unsorted part of the array and swaps it with the first unsorted element.
Insertion sort: builds the final sorted array one item at a time by inserting each element into its correct position.
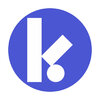
Asked in Krazio Cloud

Q. What is the design for a high throughput, fault-tolerant system?
Designing a high throughput, fault-tolerant system involves scalability, redundancy, and efficient resource management.
Use microservices architecture to isolate services and improve scalability.
Implement load balancing to distribute traffic evenly across servers.
Utilize message queues (e.g., RabbitMQ, Kafka) for asynchronous processing.
Incorporate database replication and sharding for data availability and performance.
Design for failure by using health checks and automatic fa...read more
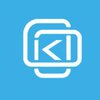
Asked in Klizo Solutions

Q. Why did you choose Node.js as your backend technology?
I chose Node.js for its performance, scalability, and the ability to use JavaScript across the stack.
Asynchronous and event-driven architecture allows handling multiple requests efficiently.
Single language (JavaScript) for both frontend and backend simplifies development and reduces context switching.
Rich ecosystem with npm provides access to a vast number of libraries and tools, speeding up development.
Great for building real-time applications like chat apps or collaborative...read more
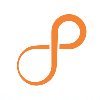
Asked in Persistent Systems

Q. Node.js is single-threaded, but how does it achieve concurrency?
NodeJs achieves multiple threading through event loop and asynchronous non-blocking I/O operations.
NodeJs uses event loop to handle multiple requests efficiently without blocking the main thread.
It utilizes asynchronous non-blocking I/O operations to perform tasks concurrently.
NodeJs can also create child processes to handle heavy computational tasks in parallel.
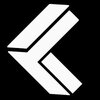
Asked in Antino

Q. What is current ctc and how much you are expecting
I am currently earning X amount and looking for a competitive salary based on my experience and skills.
My current CTC is X amount per annum
I am looking for a competitive salary based on market standards and my experience
I am open to negotiation based on the job role and responsibilities
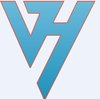
Asked in Virtual Height IT Services

Q. What do you mean by asynchronous nature?
Asynchronous nature in Node JS refers to the ability of the program to execute multiple tasks simultaneously without blocking the main thread.
Allows non-blocking I/O operations, improving performance and scalability
Uses event-driven architecture to handle multiple requests concurrently
Uses callbacks, promises, and async/await for handling asynchronous operations
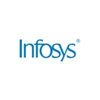
Asked in Infosys

Q. What's swagger and how to add authentication in swagger
Swagger is a tool for designing, building, and documenting APIs. Adding authentication in Swagger involves using security definitions and security requirements.
Swagger is a tool used for designing, building, and documenting APIs
To add authentication in Swagger, you can use security definitions to define authentication methods like API keys, OAuth, etc.
You can then specify security requirements in your Swagger documentation to enforce authentication for specific endpoints
For e...read more
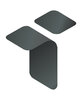
Asked in Technoladders Solutions

Q. What is the difference between functional components and class components?
Functional components are stateless and use functional syntax, while class components have state and lifecycle methods.
Functional components are simpler and easier to read/write.
Class components have access to lifecycle methods like componentDidMount.
Functional components do not have access to state or lifecycle methods.
Functional components can use hooks like useState and useEffect to manage state and side effects.
Example: Functional component - const MyComponent = () => { r...read more
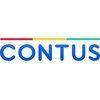
Asked in Contus

Q. Write a function that takes a date as input and prints all Saturdays and Sundays of that month.
This code prints all Saturdays and Sundays for the month of a given date input.
Use JavaScript's Date object to manipulate dates.
Extract the month and year from the input date.
Iterate through the days of the month to find Saturdays (6) and Sundays (0).
Push the found dates into an array and print them.
Example input: '2023-10-15' will yield Saturdays and Sundays of October 2023.
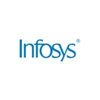
Asked in Infosys

Q. What is OAuth and how do you implement it?
OAuth is an open standard for authorization that allows users to grant access to their data without sharing their credentials.
OAuth is used to authenticate and authorize users in applications.
It allows users to grant limited access to their data to third-party applications.
OAuth uses tokens to grant access instead of sharing credentials.
The OAuth flow involves the client, server, and the authorization server.
Popular OAuth providers include Google, Facebook, and Twitter.
Asked in NeoGenCode Technologies

Q. Write a program to count the occurrences of each character in a string. For example, given the string 'aaabacbdac', the expected result is {'a': 5, 'b': 2, 'c': 2, 'd': 1}.
This program counts the occurrences of each character in a given string and returns the result as an object.
Use a loop to iterate through each character in the string.
Utilize an object to store character counts, initializing counts to zero.
For each character, increment its count in the object.
Example: For 'aaabacbdac', the counts would be: {'a': 5, 'b': 2, 'c': 2, 'd': 1}.
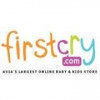
Asked in FirstCry

Q. What are some common problems encountered when using setTimeout() in JavaScript?
setTimeout() can lead to issues like callback hell, unexpected behavior, and timing inaccuracies in JavaScript.
setTimeout() executes code after a specified delay, but it doesn't guarantee exact timing.
Nested setTimeout() calls can lead to callback hell, making code hard to read and maintain.
Using setTimeout() in loops can cause unexpected behavior due to closure capturing the loop variable.
Example: for (let i = 0; i < 3; i++) { setTimeout(() => console.log(i), 1000); } // Out...read more
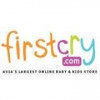
Asked in FirstCry

Q. What is Shadowing in JavaScript?
Shadowing is when a variable declared within a certain scope has the same name as a variable in an outer scope.
Occurs when a variable in an inner scope has the same name as a variable in an outer scope
The inner variable shadows the outer variable within the inner scope
Can cause unexpected behavior and bugs if not handled properly
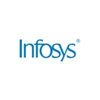
Asked in Infosys

Q. Describe the workflow of Node.js.
Nodejs is a JavaScript runtime built on Chrome's V8 JavaScript engine.
Nodejs uses an event-driven, non-blocking I/O model.
It is designed to build scalable network applications.
Nodejs uses modules to organize code.
It has a built-in package manager called npm.
Nodejs can be used for both server-side and client-side programming.
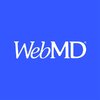
Asked in WebMD

Q. How do you deploy your application?
I deploy my application using containerization and continuous integration/continuous deployment (CI/CD) pipelines.
Utilize Docker to containerize the application for easy deployment and scalability
Use CI/CD pipelines such as Jenkins or GitLab CI to automate the deployment process
Deploy to cloud platforms like AWS, Azure, or Google Cloud for reliable hosting
Implement monitoring and logging tools like Prometheus and ELK stack for performance tracking
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
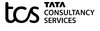
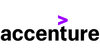
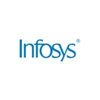
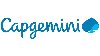
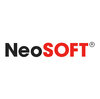
Top Interview Questions for Node JS Developer Related Skills
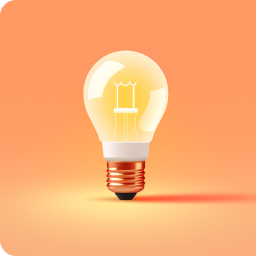
Calculate your in-hand salary
Confused about how your in-hand salary is calculated? Enter your annual salary (CTC) and get your in-hand salary
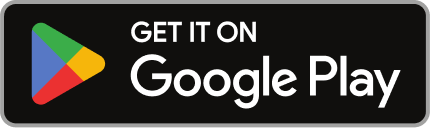
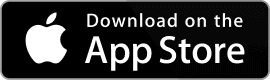
Reviews
Interviews
Salaries
Users
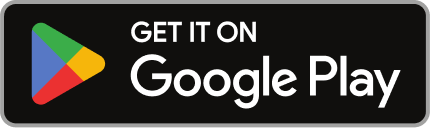
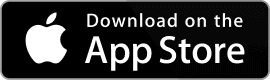