Node JS Developer
200+ Node JS Developer Interview Questions and Answers

Asked in Celebal Technologies

Q. What are the main modules of Node.js? Explain in detail.
The main modules of Node.js are HTTP, File System, Path, and Events.
HTTP module allows Node.js to transfer data over the HyperText Transfer Protocol (HTTP).
File System module enables interaction with the file system, allowing reading, writing, and manipulating files.
Path module provides utilities for working with file and directory paths.
Events module allows for event-driven programming, enabling the creation and handling of custom events.

Asked in Lattice Innovations

Q. What are joins in mysql ? what is middleware ? what is JWT ? Difference between POST, PUT and GET ? Difference between RDBMS and No-sql DBMS ? And a lot.... Every question was related to my assignment and conce...
read moreQuestions related to backend development concepts and technologies.
Joins in MySQL are used to combine data from two or more tables based on a related column.
Middleware is software that acts as a bridge between different applications or systems.
JWT (JSON Web Token) is a standard for securely transmitting information between parties as a JSON object.
POST is used to submit data to be processed, PUT is used to update existing data, and GET is used to retrieve data.
RDBMS (Relation...read more
Node JS Developer Interview Questions and Answers for Freshers

Asked in Celebal Technologies

Q. 1. What is Node.js? Describe the inner workings of Node.js
Node.js is a JavaScript runtime built on Chrome's V8 JavaScript engine, used for server-side and networking applications.
Node.js is an open-source, cross-platform runtime environment for executing JavaScript code outside of a browser.
It uses an event-driven, non-blocking I/O model that makes it lightweight and efficient.
Node.js allows developers to build scalable and high-performance applications.
It provides a rich set of built-in modules and libraries for various functionali...read more
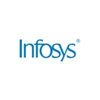
Asked in Infosys

Q. How do you deploy your Node.js application?
Nodejs application can be deployed using various tools like Heroku, AWS, DigitalOcean, etc.
Use a cloud platform like Heroku, AWS, DigitalOcean, etc.
Create a production build of the application
Configure environment variables
Use a process manager like PM2 to manage the application
Use a reverse proxy like Nginx to handle incoming requests
Set up SSL/TLS certificates for secure communication
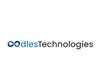
Asked in OodlesTechnologies

Q. What are closures??promises??callback??asynchrnous programming??and these questions.
Closures, promises, callbacks, and asynchronous programming are all important concepts in Node JS development.
Closures are functions that have access to variables in their outer scope.
Promises are objects that represent the eventual completion or failure of an asynchronous operation.
Callbacks are functions that are passed as arguments to other functions and are executed when the other function completes.
Asynchronous programming allows multiple tasks to be executed concurrentl...read more
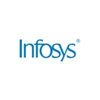
Asked in Infosys

Q. Do you follow agile or any other SDLC model?
Yes, I follow agile methodology for software development.
I believe in iterative development and continuous feedback.
I prioritize customer satisfaction and collaboration with the team.
I use tools like Jira and Trello to manage tasks and sprints.
I also follow the Scrum framework for daily stand-ups, sprint planning, and retrospectives.
Node JS Developer Jobs
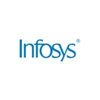
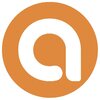
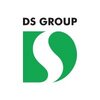
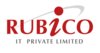
Asked in RubicoIT

Q. What are streams and its type, write a code using the any of the stream.
Streams are objects that allow reading or writing data continuously. There are four types of streams: Readable, Writable, Duplex, and Transform.
Streams are used to handle large amounts of data efficiently.
They can be used for reading data from a source or writing data to a destination.
Streams can be piped together to create a data flow.
Example: Reading a file using a Readable stream and writing it to another file using a Writable stream.
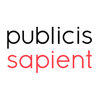
Asked in Publicis Sapient

Q. What is the difference between cluster and thread?
Cluster is a group of processes that share the same resources while thread is a lightweight process that shares the same memory.
Cluster is used for scaling and load balancing while thread is used for improving performance.
Cluster can run on multiple machines while thread runs within a single process.
Cluster requires inter-process communication while thread does not.
Examples of cluster include PM2 and Node.js cluster module while examples of thread include worker threads in No...read more
Share interview questions and help millions of jobseekers 🌟
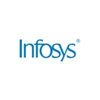
Asked in Infosys

Q. What is an error-first callback function?
Error first callback function is a convention in Node.js where the first parameter of a callback function is reserved for an error object.
The error object is passed as the first argument to the callback function
If there is no error, the error object will be null or undefined
If there is an error, the error object will contain information about the error
This convention allows for consistent error handling in asynchronous operations
Example: fs.readFile(path, 'utf8', function(err...read more

Asked in Xelpmoc Design & Tech

Q. If you have a large CSV data file, how would you process it?
Use Node.js streams to efficiently process large CSV data.
Use the 'fs' module to create a read stream for the CSV file.
Use a CSV parsing library like 'csv-parser' to parse the data row by row.
Process each row asynchronously to avoid blocking the event loop.
Use a database like MongoDB or PostgreSQL to store the processed data if needed.
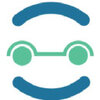
Asked in Enmovil

Q. What are the differences between var, let, and const in JavaScript?
var, let, and const are used to declare variables in JavaScript with different scoping and mutability.
var has function scope and can be redeclared and reassigned
let has block scope and can be reassigned but not redeclared
const has block scope and cannot be reassigned or redeclared
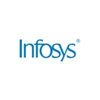
Asked in Infosys

Q. What would be the output of the setTimeout JavaScript functions?
The setTimeout function in JavaScript is used to schedule a task to be executed after a specified delay.
The setTimeout function takes two parameters: a callback function and a delay time in milliseconds.
The callback function is executed after the specified delay.
The setTimeout function returns a unique identifier (timer ID) that can be used to cancel the execution of the callback function using the clearTimeout function.
The output of the setTimeout function itself is not sign...read more
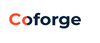
Asked in Coforge

Q. What is the Collection Framework?
Collection framework is a unified architecture for representing and manipulating collections of objects in Java.
It provides interfaces (such as List, Set, Queue) and classes (such as ArrayList, HashSet, PriorityQueue) to store and manipulate collections of objects.
It allows for easy insertion, deletion, and retrieval of elements from collections.
It provides algorithms for searching, sorting, and manipulating collections.
It promotes code reuse and modularity by providing a com...read more
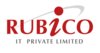
Asked in RubicoIT

Q. Given two code snippets, identify their output. The code includes the concept of the 'this' keyword and its behavior with arrow functions and normal functions.
The code demonstrates the behavior of 'this' keyword with arrow functions and normal functions.
Arrow functions do not bind their own 'this' value, it inherits 'this' from the surrounding scope.
Normal functions have their own 'this' value, which is determined by how the function is called.
Arrow functions are useful when you want to preserve the value of 'this' from the surrounding context.
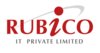
Asked in RubicoIT

Q. Write a JavaScript function that takes input from a user, calculates the sum of the inputs, and returns the result.
This code takes user input, calculates the sum, and prints the result using JavaScript.
Use prompt() to take user input in a browser environment.
Convert input strings to numbers using parseInt() or parseFloat().
Use a loop to allow multiple inputs until a specific condition is met.
Example: let sum = 0; while (true) { let input = prompt('Enter a number (or type exit):'); if (input === 'exit') break; sum += parseFloat(input); } console.log('Total Sum:', sum);
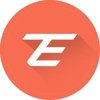
Asked in ZeMoSo Technologies

Q. Which tech stack would you prefer for designing Swiggy, and why?
For designing Swiggy, I would choose a tech stack that ensures scalability, performance, and real-time data processing.
Node.js: Ideal for handling multiple requests simultaneously, making it suitable for a food delivery platform with high traffic.
Express.js: A minimal and flexible Node.js web application framework that provides a robust set of features for web and mobile applications.
MongoDB: A NoSQL database that allows for flexible data storage and quick retrieval, essentia...read more
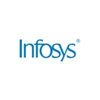
Asked in Infosys

Q. What is the MongoDB code to connect from a NodeJS backend?
To connect to MongoDB from NodeJS backend, use the 'mongodb' package and the 'MongoClient' class.
Install the 'mongodb' package using npm: npm install mongodb
Require the 'mongodb' package in your NodeJS file: const MongoClient = require('mongodb').MongoClient
Create a connection URL with the appropriate MongoDB server details: const url = 'mongodb://localhost:27017/mydatabase'
Use the 'MongoClient' class to connect to the MongoDB server: MongoClient.connect(url, function(err, db...read more
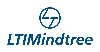
Asked in LTIMindtree

Q. When did you used and where did you used redis cache
I have used Redis cache in multiple projects to improve performance and reduce database load.
Used Redis cache to store frequently accessed data for faster retrieval
Implemented Redis cache in a Node.js application to cache API responses
Utilized Redis cache to store session data for improved user experience
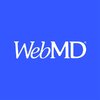
Asked in WebMD

Q. How does Node.js differentiate and handle synchronous and asynchronous tasks?
Node.js uses event-driven architecture to handle asynchronous tasks while synchronous tasks are executed in a blocking manner.
Node.js uses event loop to handle asynchronous tasks by offloading them to the system, allowing other tasks to continue without waiting.
Synchronous tasks are executed in a blocking manner, meaning the program waits for each task to complete before moving on to the next one.
Node.js provides non-blocking I/O operations which allow multiple tasks to be ex...read more
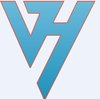
Asked in Virtual Height IT Services

Q. What are the spread and rest operators in JavaScript?
Spread operator allows an iterable to be expanded in places where zero or more arguments or elements are expected. Rest operator collects all the remaining elements into an array.
Spread operator is denoted by three dots (...) and is used to expand elements of an iterable like an array or object.
Rest operator is also denoted by three dots (...) and is used to collect multiple elements into a single array.
Spread operator example: const arr1 = [1, 2, 3]; const arr2 = [...arr1, 4...read more
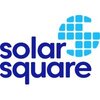
Asked in SolarSquare Energy

Q. What would be an appropriate database schema for a meeting booking application?
A meeting booking application requires a schema to manage users, meetings, and availability efficiently.
Users Table: Stores user information (e.g., user_id, name, email).
Meetings Table: Contains meeting details (e.g., meeting_id, title, description, start_time, end_time).
Availability Table: Tracks user availability (e.g., user_id, date, start_time, end_time).
Participants Table: Links users to meetings (e.g., meeting_id, user_id, status).
Notifications Table: Manages reminders ...read more
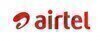
Asked in Bharti Airtel

Q. Why do we need async/await when we have callbacks and promises?
Async await simplifies asynchronous code by allowing it to be written in a synchronous style.
Async await makes asynchronous code easier to read and write compared to callbacks and promises.
It allows developers to write asynchronous code in a more synchronous manner, making it easier to understand and maintain.
Async await also helps in error handling by allowing try-catch blocks to be used with asynchronous code.
It eliminates the need for chaining multiple .then() methods when...read more
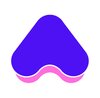
Asked in Appening

Q. How would you design a scalable Node.js application to handle 100,000 concurrent connections?
Designing a scalable Node.js app for 100,000 concurrent connections requires efficient architecture and resource management.
Use a load balancer (e.g., Nginx) to distribute traffic across multiple Node.js instances.
Implement clustering with Node.js's built-in cluster module to utilize multi-core systems.
Leverage asynchronous programming to handle I/O operations without blocking the event loop.
Utilize a message broker (e.g., RabbitMQ, Kafka) for decoupling services and managing...read more
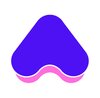
Asked in Appening

Q. What is the event loop? Describe how it handles CPU-bound tasks and I/O bound tasks.
The event loop is a core concept in Node.js that manages asynchronous operations and handles tasks efficiently.
The event loop allows Node.js to perform non-blocking I/O operations despite being single-threaded.
I/O-bound tasks (like file reading) are handled asynchronously, allowing other operations to continue.
CPU-bound tasks (like heavy computations) can block the event loop, causing delays in handling I/O tasks.
Example of I/O-bound: Reading a file using fs.readFile() allows...read more
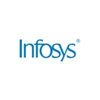
Asked in Infosys

Q. Write a SQL command to get the TOP 3 list in a data set?
SQL command to get the TOP 3 list in a data set
Use the SELECT statement to retrieve data from the table
Use the ORDER BY clause to sort the data in descending order
Use the LIMIT clause to limit the result set to 3 rows
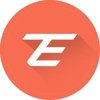
Asked in ZeMoSo Technologies

Q. What changes would you make to the middleware to mitigate a DDoS attack?
Implementing middleware changes can help mitigate DDoS attacks by controlling traffic and enhancing security measures.
Rate Limiting: Implement rate limiting to restrict the number of requests a user can make in a given time frame, e.g., 100 requests per minute.
IP Blacklisting: Maintain a list of known malicious IP addresses and block requests from them to prevent attacks from those sources.
Request Throttling: Introduce throttling mechanisms to slow down the response for users...read more
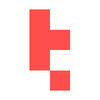
Asked in Thinkwik

Q. What is your experience with implementing task management APIs using Node.js?
I have extensive experience in building task management APIs using Node.js, focusing on scalability and performance.
Utilized Express.js to create RESTful APIs for task management, allowing CRUD operations for tasks.
Implemented JWT authentication for secure access to the API endpoints.
Used MongoDB as the database to store task data, leveraging Mongoose for schema definition and data validation.
Integrated third-party services like Slack for notifications when tasks are updated ...read more
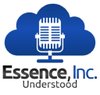
Asked in Essence Software Solutions

Q. Describe a looping logic without using built-in methods that can be shuffled every time.
Explaining loop logic in JavaScript without using built-in methods.
Use 'for' loops to iterate through arrays: for (let i = 0; i < arr.length; i++) { console.log(arr[i]); }
Implement 'while' loops for conditional iteration: let i = 0; while (i < arr.length) { console.log(arr[i]); i++; }
Utilize 'do...while' loops for at least one execution: let i = 0; do { console.log(arr[i]); i++; } while (i < arr.length);
Nested loops can be used for multi-dimensional arrays: for (let i = 0; i ...read more
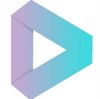
Asked in DigiPrima Technologies

Q. What is the role and responsibilities of a web developer?
A web developer is responsible for designing, coding, and maintaining websites and web applications.
Designing and developing websites and web applications using programming languages such as HTML, CSS, and JavaScript
Collaborating with designers and other team members to ensure the website meets the client's requirements
Testing and debugging websites to ensure they are functioning correctly across different browsers and devices
Updating and maintaining websites to keep them cur...read more
Asked in Venus Associates

Q. Which are the most popular Node.js frameworks you have used?
Popular Node.js frameworks include Express, Koa, NestJS, and Hapi, each offering unique features for web development.
Express: Minimalist framework, great for building RESTful APIs quickly.
Koa: Created by the same team as Express, it uses async/await for better error handling.
NestJS: A progressive framework for building efficient, reliable, and scalable server-side applications.
Hapi: Focuses on configuration-driven development, ideal for building robust applications.
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
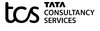
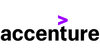
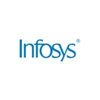
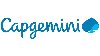
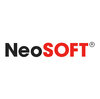
Top Interview Questions for Node JS Developer Related Skills
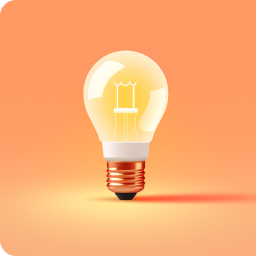
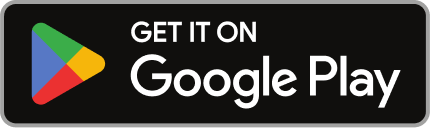
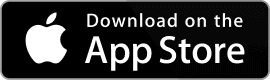
Reviews
Interviews
Salaries
Users
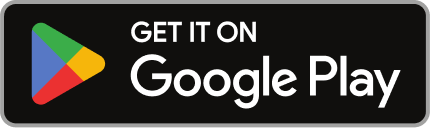
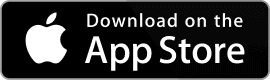