Top 250 Design Patterns Interview Questions and Answers
Updated 15 Jul 2025
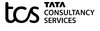
Asked in TCS

Q. What objects are generated after a TMG is created?
Objects generated after TMG creation in SAP ABAP
After creating a TMG (Table Maintenance Generator), a function group is generated.
The function group contains function modules for maintenance screens, list displays, and authorization checks.
A maintena...read more
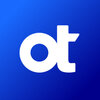
Asked in OpenText Technologies

Q. What is the difference between a factory and a service?
A factory is responsible for creating and managing objects, while a service is responsible for providing specific functionality or performing tasks.
A factory is used to create and initialize objects, often based on certain parameters or configuration...read more
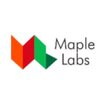
Asked in MapleLabs

Q. What is the singleton design pattern and how do you create a singleton class?
Singleton design pattern ensures only one instance of a class is created and provides global access to it.
Create a private constructor to prevent direct instantiation
Create a private static instance of the class
Provide a public static method to acces...read more
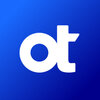
Asked in OpenText Technologies and 3 others

Q. What is the factory design pattern?
A factory is a design pattern that provides an interface for creating objects of a certain class.
A factory encapsulates the object creation logic and hides it from the client.
It allows the client to create objects without knowing the specific class o...read more
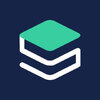
Asked in SquadStack

Designing a parking lot system involves layout planning, ticketing system, payment methods, security measures, and efficient traffic flow.
Layout planning to maximize space and accommodate different types of vehicles
Implementing a ticketing system for...read more
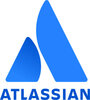
Asked in Atlassian

Design a snake game
Use a 2D array to represent the game board
Keep track of the snake's position and direction
Handle user input to change the snake's direction
Update the snake's position and check for collisions with walls or itself
Add food to the boa...read more
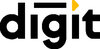
Asked in Digit Insurance

Q. What design patterns are available in Microservices?
Design patterns in Micro services help in solving common architectural challenges.
Service Registry pattern
Circuit Breaker pattern
Gateway pattern
Saga pattern
API Gateway pattern
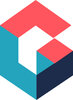
Asked in Genpact

Q. What is a singleton pattern and how do you implement it?
Singleton pattern restricts the instantiation of a class to a single instance and provides a global point of access to it.
Create a private constructor to restrict instantiation of the class
Create a private static instance of the class
Create a public ...read more
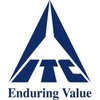
Asked in ITC

Q. How does the MVVM structure work?
MVVM is a design pattern that separates UI logic from business logic.
Model represents the data and business logic
View displays the UI and user interactions
ViewModel acts as a mediator between Model and View
ViewModel exposes data and commands to the V...read more
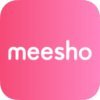
Asked in Meesho

Q. Design a cab booking system using object-oriented programming principles.
A cab booking system designed using OOP principles
Create classes for Cab, Customer, Driver, and Booking
Use inheritance and polymorphism to handle different types of cabs and bookings
Implement methods for booking a cab, assigning a driver, and calcula...read more
Design Patterns Jobs
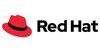
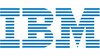
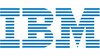
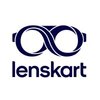
Asked in Lenskart

Q. LRU Cache Implementation Problem
Design and implement a Least Recently Used (LRU) cache data structure. This cache must support the following operations efficiently:
get(key)
: Return the value associated with the key if the key e...read more
Implement a Least Recently Used (LRU) cache data structure that supports get and put operations efficiently.
Design a data structure that maintains a cache with a specified capacity.
Implement get(key) function to return value associated with key or -1...read more
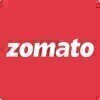
Asked in Eternal Limited

Q. LFU Cache Design Problem
Design and implement a Least Frequently Used (LFU) Cache with the following functionalities:
1. put(U__ID, value): Insert the value in the cache if the key ('U__ID') is not already present, or update the v...read more
Design and implement a Least Frequently Used (LFU) Cache with put and get functionalities, handling capacity and frequency of use.
Implement a LFU cache with put and get functions
Handle capacity and frequency of use for eviction
Return the value of key...read more
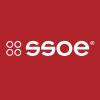
Asked in Ssoe Group

Q. Which Design Codes are you familiar with?
I am conversant with several design codes including ASME, API, and ANSI.
ASME
API
ANSI
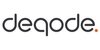
Asked in Deqode

Q. What are the different microservices patterns?
Microservices architecture involves designing applications as a collection of loosely coupled services, each focused on a specific business function.
Decomposition: Breaks down applications into smaller, manageable services (e.g., user service, order ...read more
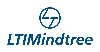
Asked in LTIMindtree

Q. Which design pattern would you use for a complex design?
Use the Strategy Pattern for complex designs to encapsulate algorithms and make them interchangeable.
Encapsulates algorithms: Allows you to define a family of algorithms, encapsulate each one, and make them interchangeable.
Promotes flexibility: Clien...read more

Asked in VMware Software

Q. What is the difference between MVC and Factory pattern?
MVC is a design pattern for structuring applications, while Factory is a creational pattern for object creation.
MVC stands for Model-View-Controller, which separates application logic into three interconnected components.
Factory pattern is used to cr...read more
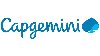
Asked in Capgemini

Q. What is the difference between architectural patterns and design patterns?
Architectural patterns define the overall structure and organization of a system, while design patterns provide solutions to specific design problems within the system.
Architectural patterns focus on high-level decisions about the system's structure,...read more
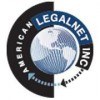
Asked in American Legalnet

Q. What is meant by the Repository Pattern?
Repository Pattern is a design pattern that separates data access logic from business logic.
It provides a way to access data from a data source without exposing the underlying database or data store.
It helps in achieving separation of concerns and ma...read more
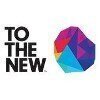
Asked in TO THE NEW

Q. Design a repository pattern using EF Core.
Repository pattern using EF core for data access layer
Create an interface for the repository with generic CRUD methods
Implement the repository interface with EF core for data access
Use dependency injection to inject the repository into services
Separa...read more
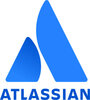
Asked in Atlassian

Q. Design the Snake Game (similar to the Nokia version).
Design a classic Snake game based on Nokia phones.
Use a 2D array to represent the game board.
Implement logic for snake movement and growth.
Include collision detection with walls and itself.
Add food items for the snake to eat and grow.
Display the game...read more
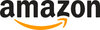
Asked in Amazon

Q. Design a lift system using OOP concepts.
Design a lift system using OOPs concepts.
Create a Lift class with methods like moveUp(), moveDown(), openDoor(), closeDoor()
Create a Floor class with methods like requestLift()
Use inheritance to create different types of lifts like passenger lift, ca...read more
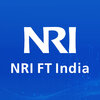

Q. Design a class that allows a maximum of three instances. After the third instance, subsequent calls should return one of the existing instances.
Design a class that limits maximum 3 instances and repeats them from 4th call.
Create a private static array to hold instances.
Create a private static counter to keep track of instance count.
Create a public static method to get instance of the class.
I...read more
Asked in Botree Communications

Q. Tell me about the Factory Design pattern.
Factory Design pattern is a creational design pattern that provides an interface for creating objects in a superclass, but allows subclasses to alter the type of objects that will be created.
Factory Design pattern is used to create objects without sp...read more
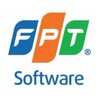
Asked in FPT Software

Q. Explain the API Gateway pattern.
An API Gateway pattern acts as a single entry point for managing and routing requests to various microservices.
Centralizes access to multiple microservices, simplifying client interactions.
Handles cross-cutting concerns like authentication, logging, ...read more
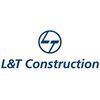
Asked in L&T Construction

Q. What design patterns are used in Angular?
The design pattern commonly used in Angular is the Observer pattern.
The Observer pattern is used to establish a one-to-many dependency between objects.
In Angular, Observables are used to implement the Observer pattern for handling asynchronous data s...read more
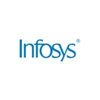
Asked in Infosys

Q. Explain the most commonly used design patterns in JavaScript.
The mostly used design patterns in JavaScript are Module, Observer, Singleton, Factory, and Prototype.
Module pattern helps to keep the code organized and maintainable.
Observer pattern is used for event handling and notification.
Singleton pattern rest...read more
Asked in Tower Research Capital LLC

Splitwise is a system for managing shared expenses among groups of people.
1. Allow users to create groups and add members to track shared expenses.
2. Implement features for adding expenses, specifying who paid and who owes.
3. Calculate balances for e...read more
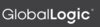
Asked in GlobalLogic

Q. Tell me about your understanding of SOLID principles.
SOLID principles are five design principles aimed at making software designs more understandable, flexible, and maintainable.
S - Single Responsibility Principle: A class should have one reason to change. Example: A User class should only handle user ...read more
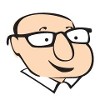
Asked in Brightly Software

Q. What is the difference between Static and Singleton?
Static is a keyword used to declare a variable or method that belongs to the class. Singleton is a design pattern that restricts the instantiation of a class to one object.
Static members are accessed using the class name, while Singleton objects are ...read more
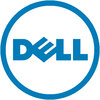
Asked in Dell

The design process for building a chatbot involves defining user goals, choosing a platform, designing conversation flow, implementing natural language processing, and testing for accuracy.
Define user goals and objectives for the chatbot
Choose a plat...read more
Top Interview Questions for Related Skills
Interview Experiences of Popular Companies
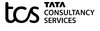
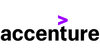
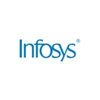
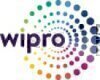
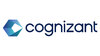
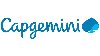
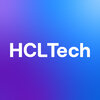
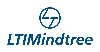
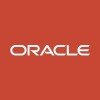
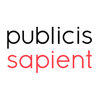
Interview Questions of Design Patterns Related Designations
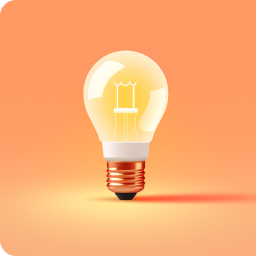
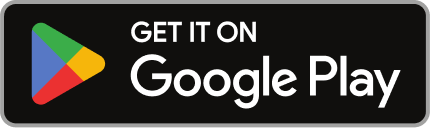
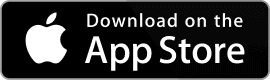
Reviews
Interviews
Salaries
Users
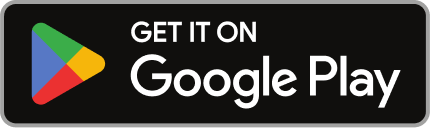
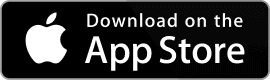