Technology Analyst
30+ Technology Analyst Interview Questions and Answers for Freshers

Asked in JPMorgan Chase & Co.

Q. Write an algorithm to find the square root of a number and determine if the number is a perfect square. Do not use built-in square root functions.
Implement logic to find the square root of a number and check if it's a perfect square without using built-in functions.
Use a loop to iterate from 1 to the number to find the square root.
Check if the square of the current number equals the input number.
If found, the number is a perfect square; otherwise, it's not.
Example: For input 16, output is 4 (perfect square). For input 20, output is 4.47 (not a perfect square).

Asked in JPMorgan Chase & Co.

Q. Can you draw and explain the different phases of SDLC (Software Development Life Cycle)?
SDLC consists of several phases including planning, analysis, design, implementation, testing, deployment, and maintenance.
Planning: Defining project goals, scope, and requirements.
Analysis: Gathering and analyzing user requirements.
Design: Creating a detailed blueprint of the software solution.
Implementation: Writing code and developing the software.
Testing: Conducting various tests to ensure software quality.
Deployment: Releasing the software for users.
Maintenance: Providin...read more
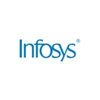
Asked in Infosys

Q. If your application experiences a memory leak in production, what steps will you take to identify and address the issue?
Identify and resolve memory leaks through systematic analysis and debugging techniques.
Monitor memory usage over time using tools like VisualVM or JProfiler to identify abnormal growth patterns.
Use profiling tools to analyze heap dumps and identify objects that are not being released, such as in Java applications.
Review code for common memory leak patterns, such as static collections holding references to objects or listeners not being removed.
Implement automated tests that s...read more
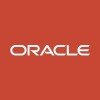
Asked in Oracle

Q. How can you divide a cake into 8 parts with only 3 cuts?
To divide a cake into 8 parts with only 3 cuts, make two perpendicular cuts to create 4 equal pieces, then cut each of those pieces in half.
Make a horizontal cut across the middle of the cake to create 2 equal halves.
Make a vertical cut through the middle of the cake to create 4 equal quarters.
Make a diagonal cut through each quarter to create 8 equal parts.
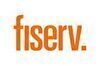
Asked in Fiserv

Q. How does memory management work in C++?
C++ memory management involves dynamic allocation, deallocation, and the use of pointers for efficient resource handling.
Memory allocation is done using 'new' keyword: int* arr = new int[10];
Memory deallocation is done using 'delete' keyword: delete[] arr;
C++ supports stack and heap memory management; stack is for static allocation, heap for dynamic.
Smart pointers (like std::unique_ptr) help manage memory automatically and prevent leaks.
Memory leaks occur when allocated memor...read more
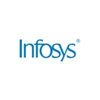
Asked in Infosys

Q. How do you write code in Lambda for EC2 provisioning?
Use AWS Lambda to automate EC2 provisioning by writing code in Python or Node.js.
Create a Lambda function in AWS console.
Write code in Python or Node.js to describe the EC2 instance to be provisioned.
Use AWS SDK to interact with EC2 API for provisioning.
Handle error cases and cleanup resources after provisioning.
Test the Lambda function to ensure it provisions EC2 instances correctly.
Technology Analyst Jobs

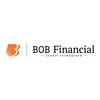
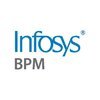
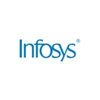
Asked in Infosys

Q. What is the importance of Spring Boot in application development?
Spring Boot simplifies Java application development with rapid setup, microservices support, and built-in features.
Rapid Development: Spring Boot reduces boilerplate code, allowing developers to create applications quickly. For example, using Spring Initializr.
Microservices Architecture: It supports building microservices, enabling scalable and maintainable applications. For instance, Netflix uses Spring Boot.
Embedded Server: Spring Boot applications can run on embedded serve...read more
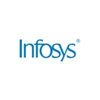
Asked in Infosys

Q. How was agile followed in the project?
Agile was followed in the project by implementing iterative development, continuous feedback, and collaboration.
The project used Scrum framework for agile implementation.
Sprints were planned and executed to deliver incremental value.
Daily stand-up meetings were held to discuss progress and address any issues.
Backlog grooming sessions were conducted to prioritize and refine user stories.
Continuous integration and automated testing were used to ensure code quality.
Regular retro...read more
Share interview questions and help millions of jobseekers 🌟
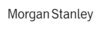
Asked in Morgan Stanley

Q. Given a number, print it in words.
A program to convert a given number into words.
Use a switch statement or if-else conditions to handle different cases
Break down the number into its individual digits and convert each digit into words
Handle special cases like numbers between 10 and 20
Consider adding a function to handle larger numbers with appropriate suffixes
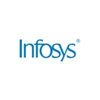
Asked in Infosys

Q. Write a Java program to filter employee objects from a list using streams.
Filter employee objects from a list using Java streams.
Use stream() method to convert the list to a stream.
Use filter() method to specify the condition for filtering employee objects.
Use collect() method to collect the filtered employee objects into a new list.
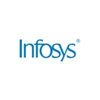
Asked in Infosys

Q. Write a template to create CloudFormation stacks.
Template for creating CloudFormation stacks
Define the resources to be created in the stack
Specify the properties for each resource
Set up dependencies between resources
Include any parameters or conditions needed for the stack
Use AWS CloudFormation Designer or AWS CLI to create the stack
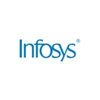
Asked in Infosys

Q. What is the importance of Generative AI?
Generative AI is crucial for innovation, creativity, and efficiency across various industries, transforming how we create and interact with technology.
Enhances creativity by generating new content, such as art, music, and writing. For example, tools like DALL-E create images from text prompts.
Improves efficiency in content creation, allowing businesses to produce marketing materials quickly and at scale.
Facilitates personalized experiences in applications like chatbots and vi...read more
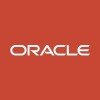
Asked in Oracle

Q. Explain your experience with MySQL procedures, triggers, and cursors.
SQL procedures, triggers, and cursors are essential for managing database operations and automating tasks.
Stored Procedures: Precompiled SQL code that can be executed with parameters. Example: `CREATE PROCEDURE GetEmployee @EmpID INT AS SELECT * FROM Employees WHERE EmployeeID = @EmpID;`
Triggers: Automated actions that occur in response to certain events on a table. Example: `CREATE TRIGGER trgAfterInsert ON Employees AFTER INSERT AS BEGIN INSERT INTO AuditLog (Action) VALUES...read more
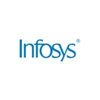
Asked in Infosys

Q. What are your three major accomplishments in the project?
Developed a mobile app, implemented a data analytics system, and led a successful software upgrade.
Developed a mobile app that increased user engagement by 30%.
Implemented a data analytics system that improved decision-making process and reduced costs by 20%.
Led a successful software upgrade project, ensuring minimal downtime and improved system performance.
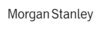
Asked in Morgan Stanley

Q. Explain the Bubble Sort algorithm.
Bubble Sort is a simple sorting algorithm that repeatedly steps through the list, compares adjacent elements and swaps them if they are in the wrong order.
Bubble Sort compares adjacent elements and swaps them if they are in the wrong order.
It continues this process until the entire list is sorted.
It is called Bubble Sort because smaller elements 'bubble' to the top of the list.
Bubble Sort has a time complexity of O(n^2) in the average and worst case scenarios.
Example: Given a...read more
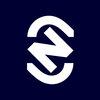
Asked in Nofinite

Q. What is software development?
Software development is the process of creating, designing, testing, and maintaining software applications.
Involves writing code to create software applications
Includes designing the user interface and user experience
Testing the software for bugs and errors
Maintaining and updating the software as needed
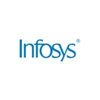
Asked in Infosys

Q. Write a Java program to sort a list.
Java program to sort a list of strings
Use Collections.sort() method to sort the list of strings
Create a list of strings and add elements to it
Call Collections.sort() method on the list to sort it
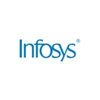
Asked in Infosys

Q. What is dependency injection?
Dependency Injection is a design pattern that allows objects to receive dependencies rather than creating them internally.
It helps to decouple the code and makes it more testable and maintainable.
It allows for easier swapping of dependencies without changing the code.
There are three types of dependency injection: constructor injection, setter injection, and interface injection.
Example: Instead of creating a database connection object inside a class, the object is passed as a ...read more
Asked in Thoughtline Technologies

Q. What are boxing and unboxing?
Boxing is the process of converting a value type to a reference type, while unboxing is the process of converting a reference type to a value type.
Boxing is done implicitly by the compiler when a value type is assigned to a reference type variable.
Unboxing requires an explicit cast from the reference type to the value type.
Boxing and unboxing can impact performance as they involve memory allocation and copying of data.
Example: int num = 10; object obj = num; // Boxing, conver...read more
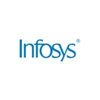
Asked in Infosys

Q. What are MVC and Razor?
MVC stands for Model-View-Controller, a software architectural pattern. Razor is a markup syntax used in ASP.NET MVC.
MVC is a design pattern that separates an application into three main components: Model, View, and Controller
Razor is a markup syntax used in ASP.NET MVC to create dynamic web pages
MVC helps in organizing code and separating concerns, making it easier to maintain and test applications
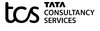
Asked in TCS

Q. What is garbage collection?
Garbage collection is a process in programming where the system automatically reclaims memory occupied by objects that are no longer in use.
Garbage collection helps prevent memory leaks by automatically freeing up memory that is no longer needed.
It is commonly used in languages like Java, C#, and Python.
Garbage collection can be either automatic or manual, with automatic being the most common approach.
Examples of garbage collection algorithms include reference counting and ma...read more
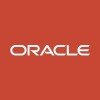
Asked in Oracle

Q. Explain all connections and terms
Connections and terms in technology analysis are essential for understanding the relationships between different components and concepts.
Connections refer to the relationships between different elements in a system or network.
Terms are the specific vocabulary used to describe concepts, tools, and processes in technology analysis.
Understanding connections and terms helps analysts make sense of data, identify patterns, and draw conclusions.
Examples: network topology, data encry...read more
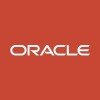
Asked in Oracle

Q. Prepare a DB schema for a university.
Design a database schema for a university
Create tables for students, courses, professors, departments, and enrollments
Establish relationships between tables using foreign keys
Include attributes such as student ID, course ID, professor ID, department ID, and enrollment date
Consider normalization to reduce redundancy and improve data integrity
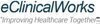
Asked in eClinicalWorks

Q. What is an immutable class?
An immutable class is a class whose instances cannot be modified after creation, ensuring data integrity and thread safety.
Immutable classes prevent changes to their state after creation, e.g., String in Java.
They often provide methods to return new instances with modified values, e.g., LocalDate in Java.
Immutable objects are inherently thread-safe, reducing synchronization issues.
Commonly used in functional programming and for creating value objects.
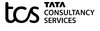
Asked in TCS

Q. Explain the concepts of OOPS.
Oops concepts are the fundamental concepts of object-oriented programming.
Encapsulation - binding data and functions together
Inheritance - creating new classes from existing ones
Polymorphism - ability of objects to take on multiple forms
Abstraction - hiding implementation details from users
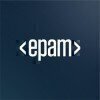
Asked in EPAM Systems

Q. interface vs abstract
Interface and abstract are both used for abstraction in object-oriented programming.
Interfaces define a contract that a class must implement.
Abstract classes provide a base implementation that can be extended by subclasses.
Interfaces can be implemented by multiple classes, while a class can only extend one abstract class.
Interfaces can only have abstract methods, while abstract classes can have both abstract and concrete methods.
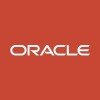
Asked in Oracle

Q. OOPS Concepts Implementation
OOPS concepts are implemented in object-oriented programming languages to improve code organization and reusability.
Encapsulation: bundling data and methods that operate on the data into a single unit (class)
Inheritance: allows a class to inherit properties and behavior from another class
Polymorphism: ability for objects to be treated as instances of their parent class or their own class
Abstraction: hiding the complex implementation details and showing only the necessary feat...read more
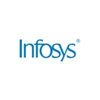
Asked in Infosys

Q. What is the cp command?
cp command is used in Unix and Linux operating systems to copy files and directories.
Used to copy files and directories from one location to another
Syntax: cp [options] source destination
Options include -r for recursive copying, -i for interactive mode, -v for verbose output
Example: cp file1.txt /path/to/directory/
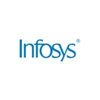
Asked in Infosys

Q. What is the mv command?
mv command is used in Unix and Linux operating systems to move files or directories from one location to another.
Used to rename files or directories by moving them to a new location
Syntax: mv [options] source destination
Example: mv file1.txt /path/to/new/location/
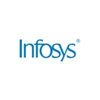
Asked in Infosys

Q. Write code to generate the Fibonacci series.
The Fibonacci series is a sequence where each number is the sum of the two preceding ones, starting from 0 and 1.
The series starts with 0 and 1: 0, 1, 1, 2, 3, 5, 8, 13, ...
The nth Fibonacci number can be calculated using recursion or iteration.
A common formula for Fibonacci is F(n) = F(n-1) + F(n-2).
Example: F(5) = F(4) + F(3) = 3 + 2 = 5.
Fibonacci numbers are used in algorithms, data structures, and nature.
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
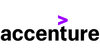
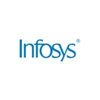
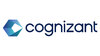
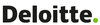
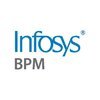
Top Interview Questions for Technology Analyst Related Skills
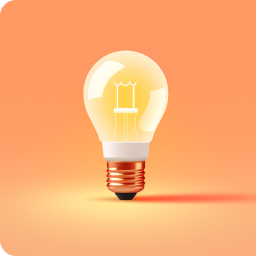
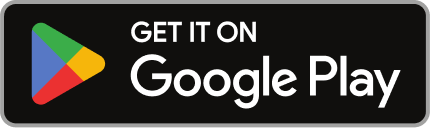
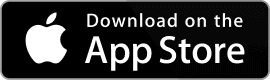
Reviews
Interviews
Salaries
Users
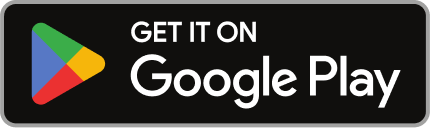
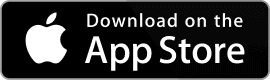