Software Trainee
100+ Software Trainee Interview Questions and Answers
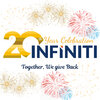
Asked in Infiniti Software Solutions

Q. How can you create a pattern by taking user input?
You can create patterns using loops and user input to define dimensions and characters for the pattern.
Use nested loops: Outer loop for rows, inner loop for columns.
Get user input for the number of rows and columns.
Use characters like '*', '#', or user-defined characters to form the pattern.
Example: For a right triangle pattern, use nested loops to print '*' based on the current row number.
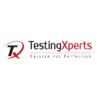
Asked in TestingXperts

Q. Explain OOPS concepts and why we use them.
OOPS concepts are fundamental principles in object-oriented programming that help in organizing and managing code efficiently.
Encapsulation: Bundling data and methods that operate on the data into a single unit (class). Example: Class Car with properties like make, model, and methods like start(), stop().
Inheritance: Allows a class to inherit properties and behavior from another class. Example: Class SUV inheriting from class Car.
Polymorphism: Ability to present the same inte...read more
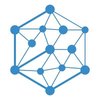
Asked in Infomaze Elite

Q. Do you have any experience with basic SQL queries (CRUD operations, JOINs, Views, etc.)?
I have a solid understanding of basic SQL queries, including CRUD operations, JOINs, and Views.
CRUD operations: Create (INSERT), Read (SELECT), Update (UPDATE), Delete (DELETE). Example: SELECT * FROM users;
JOINs: Used to combine rows from two or more tables based on a related column. Example: SELECT * FROM orders JOIN customers ON orders.customer_id = customers.id;
Views: Virtual tables created by a query. Example: CREATE VIEW active_users AS SELECT * FROM users WHERE status ...read more
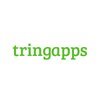
Asked in Tringapps Research Labs

Q. Given a problem, share your screen while solving it.
I will demonstrate problem-solving skills by sharing my screen while solving a given problem.
Explain my thought process while solving the problem
Use relevant tools or software to demonstrate the solution
Engage with the interviewer to clarify any doubts or seek feedback
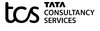
Asked in TCS

Q. What is the difference between a class and an object?
Class is a blueprint or template for creating objects, while object is an instance of a class.
A class defines the properties and behaviors of objects, while an object is an instance of a class.
A class can have multiple objects, but an object belongs to only one class.
A class can have static methods and variables, while objects cannot.
An object can access the properties and methods of its class, but not of other classes.
Example: Class - Car, Object - Honda Civic.
Example: Class...read more
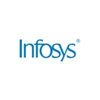
Asked in Infosys

Q. What is the difference between a stack and a queue?
Stacks and queues are both data structures, but they differ in how elements are added and removed.
Stack follows Last In First Out (LIFO) principle; the last element added is the first to be removed. Example: Undo feature in text editors.
Queue follows First In First Out (FIFO) principle; the first element added is the first to be removed. Example: Print job scheduling.
Stacks use operations like push (add) and pop (remove), while queues use enqueue (add) and dequeue (remove).
St...read more
Software Trainee Jobs
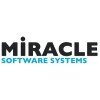
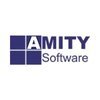
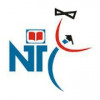
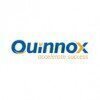
Asked in Quinnox

Q. What is your understanding of mainframe technology?
Mainframe technology refers to powerful computing systems used for large-scale data processing and critical applications.
Mainframes are known for their high reliability and availability, often used in banking systems for transaction processing.
They support multiple operating systems and can run thousands of applications simultaneously, such as IBM z/OS.
Mainframes excel in handling large volumes of data, making them ideal for industries like finance, healthcare, and government...read more
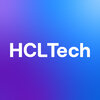
Asked in HCLTech

Q. How can we achieve multiple inheritance in Java?
Multiple inheritance cannot be achieved directly in Java, but it can be simulated using interfaces or abstract classes.
Java does not support multiple inheritance of classes
Multiple inheritance can be achieved using interfaces or abstract classes
Interfaces allow a class to inherit from multiple interfaces
Abstract classes can provide partial implementation and can be extended by a single class
Share interview questions and help millions of jobseekers 🌟
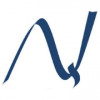
Asked in Neoquant Solutions

Q. What is OOPS, Exception, threads,static class,Array, collection
OOPS is a programming paradigm based on objects, Exception is an event that disrupts normal flow of program, threads are parallel execution units, static class cannot be instantiated, Array is a data structure to store multiple elements, Collection is a group of objects.
OOPS - Object-oriented programming paradigm
Exception - Event that disrupts normal flow of program
Threads - Parallel execution units
Static class - Cannot be instantiated
Array - Data structure to store multiple ...read more
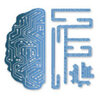
Asked in Feynn Labs

Q. What is the difference between linear and logistic regression?
Linear regression is used for predicting continuous values, while logistic regression is used for predicting binary outcomes.
Linear regression is used when the dependent variable is continuous and has a linear relationship with the independent variable.
Logistic regression is used when the dependent variable is binary or categorical and the relationship between the independent variables and the outcome is non-linear.
Linear regression predicts the value of a continuous outcome ...read more
Asked in Appinlay

Q. What are the data types in Javascript?
JavaScript has several data types including number, string, boolean, object, null, and undefined.
Number: represents numeric values, e.g. 10, 3.14
String: represents textual data, e.g. 'hello', '123'
Boolean: represents true or false values
Object: represents a collection of key-value pairs
Null: represents the intentional absence of any object value
Undefined: represents an uninitialized variable
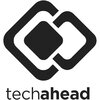
Asked in Techahead Software

Q. What are access specifiers? What is their significance in OOPs?
Access specifiers control the visibility of class members in OOP, enhancing encapsulation and data protection.
Public: Members are accessible from anywhere. Example: 'public int age;' allows access from any class.
Private: Members are accessible only within the class. Example: 'private String name;' restricts access to the class itself.
Protected: Members are accessible within the class and its subclasses. Example: 'protected void display();' allows access in derived classes.
Def...read more
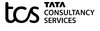
Asked in TCS

Q. What is inheritance, and what are its types?
Inheritance is a concept in object-oriented programming where a class inherits properties and behaviors from another class.
Types of inheritance: single inheritance, multiple inheritance, multilevel inheritance, hierarchical inheritance
Single inheritance: a class inherits from only one parent class
Multiple inheritance: a class inherits from multiple parent classes
Multilevel inheritance: a class inherits from a parent class, which in turn inherits from another parent class
Hiera...read more
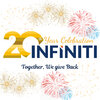
Asked in Infiniti Software Solutions

Q. What are the steps to create a form using HTML?
Creating a form in HTML involves defining the structure, input elements, and submission methods for user data collection.
1. Start with the <form> tag: This defines the form element. Example: <form action='submit.php' method='post'>.
2. Add input elements: Use <input>, <textarea>, <select>, etc., to collect user data. Example: <input type='text' name='username'>.
3. Label your inputs: Use <label> tags for accessibility. Example: <label for='username'>Username:</label>.
4. Include...read more
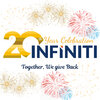
Asked in Infiniti Software Solutions

Q. What is a garbage collector in programming?
A garbage collector automatically manages memory by reclaiming unused objects, preventing memory leaks in programming languages.
Garbage collection helps in automatic memory management.
It identifies and disposes of objects that are no longer needed.
Languages like Java and C# use garbage collectors to manage memory.
Example: In Java, the garbage collector runs in the background to free memory.
It reduces the risk of memory leaks and improves application stability.
Asked in Railworld India

Q. What is the difference between JVM and JRE?
JVM is the Java Virtual Machine that executes Java bytecode, while JRE is the Java Runtime Environment that provides libraries and components for execution.
JVM (Java Virtual Machine) is responsible for executing Java bytecode.
JRE (Java Runtime Environment) includes JVM along with libraries and other components needed to run Java applications.
JVM is platform-independent, allowing Java programs to run on any device with a compatible JVM.
JRE is platform-specific; it must be inst...read more
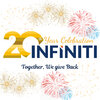
Asked in Infiniti Software Solutions

Q. What are the differences between a LinkedList and an ArrayList?
Linkedlist is a data structure where elements are stored in nodes with pointers to the next node. Arraylist is a dynamic array that can grow or shrink in size.
Linkedlist allows for efficient insertion and deletion of elements anywhere in the list, while Arraylist is faster for accessing elements by index.
Linkedlist uses more memory due to the overhead of storing pointers, while Arraylist uses contiguous memory for elements.
Example: Linkedlist - 1 -> 2 -> 3 -> 4, Arraylist - [...read more
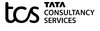
Asked in TCS

Q. What is encapsulation?
Encapsulation is the process of hiding internal details and providing a public interface for accessing and manipulating data.
Encapsulation is a fundamental principle of object-oriented programming.
It helps in achieving data abstraction and data hiding.
By encapsulating data and methods within a class, we can control access to them.
Encapsulation improves code maintainability and reusability.
Example: A class with private variables and public methods to access and modify those va...read more
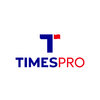
Asked in TimesPro

Q. What is the code to reverse a string?
Reversing a string involves rearranging its characters in the opposite order, which can be done using various programming techniques.
Using Python: reversed_string = original_string[::-1]
Using Java: String reversed = new StringBuilder(original).reverse().toString();
Using C++: std::reverse(original.begin(), original.end());
Using JavaScript: let reversed = original.split('').reverse().join('');
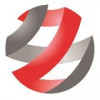
Asked in Softsquare

Q. What is Salesforce? products of salesforce
Salesforce is a cloud-based customer relationship management (CRM) platform that helps businesses manage their sales, customer service, marketing, and more.
Salesforce offers a wide range of products including Sales Cloud, Service Cloud, Marketing Cloud, and Commerce Cloud.
Sales Cloud helps businesses track customer information and interactions.
Service Cloud allows businesses to provide excellent customer service and support.
Marketing Cloud helps businesses create personalized...read more
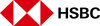
Asked in HSBC Group

Q. What is C++ and how is it used for OOP concepts?
C++ is a programming language used for object-oriented programming (OOP) concepts.
C++ supports OOP concepts like encapsulation, inheritance, and polymorphism.
Classes and objects are the building blocks of C++ OOP.
C++ allows for data abstraction and data hiding through access specifiers.
Virtual functions and templates are also important features of C++ OOP.
Example: A class 'Car' can have properties like 'make', 'model', and 'year', and methods like 'start' and 'stop'.
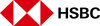
Asked in HSBC Group

Q. What is puthon and how compiler works in the laptop
Python is a high-level programming language used for web development, data analysis, artificial intelligence, and more. The compiler translates Python code into machine-readable code.
Python is an interpreted language, meaning that the code is executed line by line without the need for compilation before execution.
Python code is compiled into bytecode, which is then executed by the Python interpreter.
Python has a dynamic type system, which means that the type of a variable is ...read more
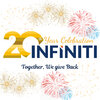
Asked in Infiniti Software Solutions

Q. Explain the code you solved in the previous round.
The code implements a sorting algorithm to arrange an array of integers in ascending order.
The algorithm used is QuickSort, which is efficient for large datasets.
It works by selecting a 'pivot' element and partitioning the array into two halves.
Elements less than the pivot go to the left, and those greater go to the right.
This process is recursively applied to the sub-arrays until sorted.
For example, sorting the array [3, 6, 8, 10, 1, 2, 1] results in [1, 1, 2, 3, 6, 8, 10].
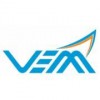
Asked in Vem Technologies

Q. Explain storage classes in C.
Storage classes in C define the scope, lifetime, and visibility of variables and functions.
1. Automatic Storage Class: Variables declared inside a function, e.g., 'int main() { int a; }'.
2. Static Storage Class: Retains value between function calls, e.g., 'static int count = 0;'.
3. External Storage Class: Variables declared outside any function, accessible globally, e.g., 'int globalVar;'.
4. Register Storage Class: Suggests to store variable in CPU registers for faster access...read more
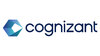
Asked in Cognizant

Q. What is diff between c and c++ Any code can u type now
C++ is an extension of C with object-oriented programming features.
C++ supports object-oriented programming while C does not.
C++ has classes and objects while C does not.
C++ has function overloading and operator overloading while C does not.
C++ has exception handling while C does not.
C++ supports namespaces while C does not.
C++ has a standard template library (STL) while C does not.
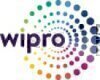
Asked in Wipro

Q. Tell me about variables and their types.
Variables are containers that store data values. There are different types of variables in programming.
Variables are declared using keywords like var, let, const.
Variables can store different types of data such as numbers, strings, booleans, objects, arrays, etc.
Variables can be global or local depending on where they are declared.
Variables can be reassigned with new values.
Examples: var age = 25; let name = 'John'; const PI = 3.14;
Asked in Code Vyasa

Q. What is the difference between MySQL and MongoDB?
MySQL is a relational database management system, while MongoDB is a NoSQL database management system.
MySQL is a relational database, meaning it stores data in tables with rows and columns.
MongoDB is a NoSQL database, storing data in collections of JSON-like documents.
MySQL uses structured query language (SQL) for querying data.
MongoDB uses a query language that is similar to JSON.
MySQL is ACID compliant, ensuring data integrity.
MongoDB is not ACID compliant but offers high s...read more
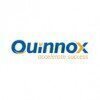
Asked in Quinnox

Q. What is your experience with mainframe technology?
I have foundational knowledge of mainframe technology, focusing on its architecture, programming languages, and applications in enterprise systems.
Familiar with COBOL, a primary language used in mainframe applications.
Understanding of JCL (Job Control Language) for job scheduling and management.
Experience with DB2 for database management on mainframes.
Knowledge of CICS (Customer Information Control System) for transaction processing.
Exposure to mainframe environments like IBM...read more
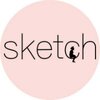
Asked in Sketch

Q. Write a React code with two boxes, where the sum of the values in the boxes must be greater than 3.5.
Implement a React component with two input boxes that only allows values above 3.5.
Use controlled components to manage input values.
Implement validation logic to check if the input is above 3.5.
Provide user feedback if the input is invalid.
Example: Use state to store input values and update them on change.
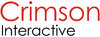
Asked in Crimson Interactive

Q. Extention methods in C#, Dependency injection
Extension methods add functionality to existing types. Dependency injection is a design pattern for managing object dependencies.
Extension methods allow adding new methods to existing types without modifying the original type
Dependency injection is a technique for providing objects with their dependencies
Dependency injection can be achieved through constructor injection, property injection, or method injection
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
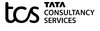
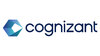
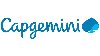
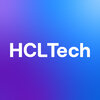
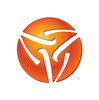
Top Interview Questions for Software Trainee Related Skills
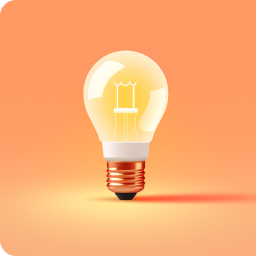
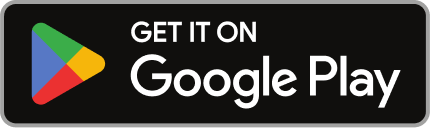
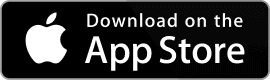
Reviews
Interviews
Salaries
Users
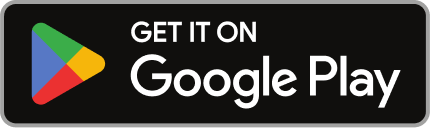
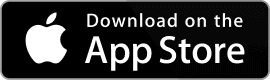