Software Engineer
8000+ Software Engineer Interview Questions and Answers
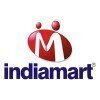
Asked in Indiamart Intermesh

Q. Prime Numbers within a Range
Given an integer N, determine and print all the prime numbers between 2 and N, inclusive.
Input:
Integer N
Output:
Prime numbers printed on separate lines
Example:
Input:
N = 10
Out...read more
Generate and print all prime numbers between 2 and N, inclusive.
Iterate from 2 to N and check if each number is prime
Use a helper function to determine if a number is prime
Print each prime number on a new line
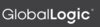
Asked in GlobalLogic

Q. Reverse Array Elements
Given an array containing 'N' elements, the task is to reverse the order of all array elements and display the reversed array.
Explanation:
The elements of the given array need to be rear...read more
Reverse the order of elements in an array and display the reversed array.
Create a function that takes an array as input
Use a loop to iterate through the array and swap elements at opposite ends
Return the reversed array
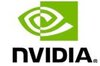
Asked in Nvidia

Q. Check Word Presence in String
Given a string S
and a list wordList
containing N
distinct words, determine if each word in wordList
is present in S
. Return a boolean array where the value at index 'i' indicates ...read more
Given a string and a list of words, determine if each word in the list is present in the string and return a boolean array indicating their presence.
Iterate through each word in the word list and check if it is present in the string.
Use a boolean array to store the presence of each word in the string.
Remember that the presence of a word is case sensitive.
Do not use built-in string-matching methods.
Return the boolean array without printing it.
Asked in Tower Research Capital LLC

Q. Dance Team Pairing Challenge
Imagine you are helping Ninja, a dance coach, who needs to form dance pairs from the available boys and girls in a studio. Given the number of boys N
, the number of girls M
, and the...read more
The challenge involves forming dance pairs from available boys and girls based on potential pairings to maximize the number of pairs.
Parse the input to get the number of test cases, boys, girls, and potential pairings.
Iterate through the potential pairings and form pairs based on the given indexes.
Output '1' if a set of maximum possible pairs is returned, else output '0'.
There can be multiple valid configurations of pairs.
Example: For input '2 2 2' and pairings '1 1' and '2 2...read more
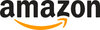
Asked in Amazon

Q. Maximum Subarray Sum Problem Statement
Given an array arr
of length N
consisting of integers, find the sum of the subarray (including empty subarray) with the maximum sum among all subarrays.
Explanation:
A sub...read more
Find the sum of the subarray with the maximum sum among all subarrays in an array of integers.
Iterate through the array and keep track of the maximum sum subarray seen so far.
Use Kadane's algorithm to efficiently find the maximum subarray sum.
Consider the case where all elements in the array are negative.
Handle the case where the array contains only one element.
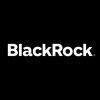
Asked in Blackrock

Q. Merge Two Sorted Arrays Problem Statement
Given two sorted integer arrays ARR1
and ARR2
of size M and N, respectively, merge them into ARR1
as one sorted array. Assume that ARR1
has a size of M + N to hold all ...read more
Merge two sorted arrays into one sorted array in place.
Iterate from the end of both arrays and compare elements to merge in place
Use two pointers to keep track of the current position in each array
Handle cases where one array is fully merged before the other
Software Engineer Jobs
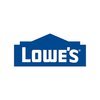


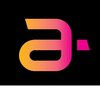
Asked in Amdocs

Q. Reverse Stack with Recursion
Reverse a given stack of integers using recursion. You must accomplish this without utilizing extra space beyond the internal stack space used by recursion. Additionally, you must r...read more
Reverse a given stack of integers using recursion without using extra space or loops.
Use recursion to pop all elements from the original stack and store them in function call stack
Once the stack is empty, push the elements back in reverse order using recursion
Use the top(), pop(), and push() methods to manipulate the stack
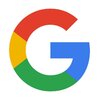
Asked in Google

Q. Sudoku Solver
Given a 9x9 Sudoku board, your task is to fill the empty slots and return the completed Sudoku solution.
A Sudoku is a grid composed of nine 3x3 smaller grids. The challenge is to fill in the numb...read more
Implement a Sudoku solver to fill empty slots in a 9x9 grid with numbers 1-9 satisfying constraints.
Create a recursive function to solve the Sudoku puzzle by trying out different numbers in empty slots.
Use backtracking to backtrack and try a different number if a conflict is encountered.
Ensure each number appears only once per row, column, and 3x3 grid.
Return the completed Sudoku grid as the output.
Share interview questions and help millions of jobseekers 🌟
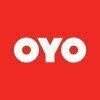
Asked in Oyo Rooms

Q. Water Jug Problem Statement
You have two water jugs with capacities X
and Y
liters respectively, both initially empty. You also have an infinite water supply. The goal is to determine if it is possible to measu...read more
The Water Jug Problem involves determining if a specific amount of water can be measured using two jugs of different capacities.
Start by considering the constraints and limitations of the problem.
Think about how the operations allowed can be used to reach the target measurement.
Consider different scenarios and test cases to come up with a solution.
Implement a function that takes the capacities of the jugs and the target measurement as input and returns True or False based on ...read more
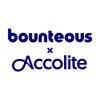
Asked in Bounteous x Accolite

Q. Total time: 110 mins 1. Find missing and duplicate numbers from given array(algo, code, optimization, dry run, complexity analysis) 2. Flatten Binary tree to Linked List Conversion (algo, code, optimization, dr...
read moreInterview questions for Software Engineer position
Array manipulation and linked list operations
Tree data structure and balancing techniques
Database concepts and differences between SQL and NoSQL
Web development frameworks and protocols
Concepts of Deadlock and Race Condition
Use of pointers in function oriented programming and their absence in Java
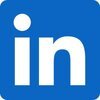
Asked in LinkedIn

Q. Generate All Parentheses Combinations
Given an integer N
, your task is to create all possible valid parentheses configurations that are well-formed using N
pairs. A sequence of parentheses is considered well-fo...read more
Generate all possible valid parentheses configurations with N pairs.
Use backtracking to generate all possible combinations of parentheses.
Keep track of the number of open and close parentheses used.
Add '(' if there are remaining open parentheses, and add ')' if there are remaining close parentheses.
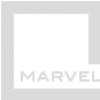
Asked in Marvel Realtors

Q. In a two-player game, players take turns placing coins on a round table without overlapping. The player who cannot place a coin loses. Assuming both players have an infinite supply of coins, and you go first, w...
read moreTo win the coin placement game, start by placing a coin in the center and mirror your opponent's moves.
Place your first coin in the center of the table.
After your opponent places a coin, identify the position relative to the center.
Reflect their move across the center point to maintain symmetry.
This strategy ensures that you always have a valid move until the table is full.

Asked in UnitedHealth

Q. Bubble Sort Problem Statement
Sort the given unsorted array consisting of N non-negative integers in non-decreasing order using the Bubble Sort algorithm.
Input:
The first line contains an integer 'T' represent...read more
Bubble Sort is used to sort an array of non-negative integers in non-decreasing order.
Iterate through the array and compare adjacent elements, swapping them if they are in the wrong order.
Repeat this process until the array is sorted.
Time complexity of Bubble Sort is O(n^2) in worst case.
Space complexity of Bubble Sort is O(1) as it sorts the array in place.
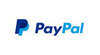
Asked in PayPal

Q. Maximum Difference Problem Statement
Given an array ARR
of N
elements, your task is to determine the maximum difference between any two elements in ARR
.
If the maximum difference is even, print EVEN
. If the max...read more
Find the maximum difference between any two elements in an array and determine if it is even or odd.
Iterate through the array to find the maximum and minimum elements.
Calculate the difference between the maximum and minimum elements.
Check if the difference is even or odd and return the result.
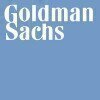
Asked in Goldman Sachs

On an Island, there is an airport that has an unlimited number of identical air-planes. Each air-plane has a fuel capacity to allow it to fly exactly 1/2 way around the world, along a great circle. The pl...read more
Minimum number of airplanes needed to get one airplane all the way around the world and return safely to the airport.
To achieve this, we need 3 airplanes in total.
The first airplane flies halfway around the world, then transfers half of its fuel to the second airplane.
The first airplane then returns to the airport, while the second airplane continues to fly the remaining half of the journey.
When the second airplane reaches the destination, it transfers all its fuel to the thi...read more
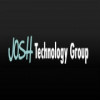
Asked in Josh Technology Group

Q. Railway Seat Berth Determination
Given a railway seat number represented as an integer, determine if it is a valid seat number and identify its berth type. Possible berth types include lower berth, middle berth...read more
Given a railway seat number, determine if it is valid and identify its berth type.
Parse input integer 't' for number of test cases
For each test case, check if seat number is valid (1 <= N <= 100)
Identify berth type based on seat number and output the result
Possible berth types are Lower, Middle, Upper, Side Lower, and Side Upper
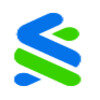
Asked in Standard Chartered

Q. Ways To Make Coin Change
Given an infinite supply of coins of varying denominations, determine the total number of ways to make change for a specified value using these coins. If it's not possible to make the c...read more
The task is to determine the total number of ways to make change for a specified value using given denominations.
Create a dynamic programming table to store the number of ways to make change for each value up to the target value.
Iterate through each denomination and update the table accordingly based on the current denomination.
The final value in the table will represent the total number of ways to make change for the target value.
Consider edge cases such as when the target v...read more
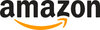
Asked in Amazon

Q. Minimum Number of Platforms Needed Problem Statement
You are given the arrival and departure times of N trains at a railway station for a particular day. Your task is to determine the minimum number of platform...read more
The task is to determine the minimum number of platforms needed at a railway station based on arrival and departure times of trains.
Sort the arrival and departure times in ascending order.
Use two pointers to track overlapping schedules.
Increment platform count when a new train arrives before the previous one departs.
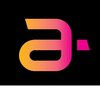
Asked in Amdocs

Q. Palindrome String Validation
Determine if a given string 'S' is a palindrome, considering only alphanumeric characters and ignoring spaces and symbols.
Note:
The string 'S' should be evaluated in a case-insensi...read more
Validate if a given string is a palindrome after removing special characters, spaces, and converting to lowercase.
Remove special characters and spaces from the input string
Convert the string to lowercase
Check if the modified string is equal to its reverse to determine if it's a palindrome
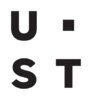
Asked in UST

Q. String Compression Task
Develop an algorithm that compresses a string by replacing consecutive duplicate characters with the character followed by the count of its repetitions, if that count exceeds 1.
Example:...read more
Develop an algorithm to compress a string by replacing consecutive duplicate characters with the character followed by the count of its repetitions.
Iterate through the input string while keeping track of consecutive character counts.
Replace consecutive duplicate characters with the character followed by the count if count exceeds 1.
Ensure the count does not exceed 9 for each character.
Return the compressed string as output.
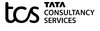
Asked in TCS

Q. Write a program for Fibonacci series for n terms where n is the user input.
Program for Fibonacci series for n terms with user input.
Take user input for n
Initialize variables for first two terms of Fibonacci series
Use a loop to generate the series up to n terms
Print the series
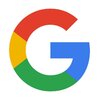
Asked in Google

Q. If your Wi-Fi router is not working, what steps would you take to fix it?
I will troubleshoot the router by checking the power supply, resetting the router, and checking the network settings.
Check if the router is properly plugged in and receiving power
Reset the router by turning it off and on again
Check the network settings and make sure they are correct
Try connecting to the router with a different device
If all else fails, contact the internet service provider for assistance
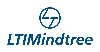
Asked in LTIMindtree

Q. There are 3 wheels, each capable of traveling 5 km. To move forward, you must use 2 wheels at a time. What is the maximum distance you can travel?
Maximum distance that can be traveled using 3 wheels, each with a 5km limit and requiring 2 wheels to move forward.
Using all 3 wheels, we can move forward a total of 10kms (5kms using 2 wheels at a time)
After using the first 2 wheels to travel 5kms, we can switch to the remaining wheel and travel another 5kms
Alternatively, we can use 2 wheels to travel 5kms, then switch to the remaining wheel and use another 2 wheels to travel another 5kms, for a total of 10kms
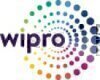
Asked in Wipro

Q. What is the difference between a compiler and an interpreter?
A compiler translates the entire program into machine code before execution, while an interpreter translates and executes the program line by line.
A compiler converts the source code into an executable file, while an interpreter executes the code directly.
Compilers typically produce faster and more efficient code, while interpreters provide faster development and debugging.
Examples of compilers include GCC, Clang, and Visual C++, while examples of interpreters include Python,...read more
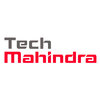
Asked in Tech Mahindra

Q. Character Frequency in Order of Occurrence
For the given string S
of length N
, determine the frequency of each character from 'a' to 'z' appearing within S
.
Input:
The first input line contains an integer 'T' r...read more
The task is to determine the frequency of each character from 'a' to 'z' in a given string.
Create an array of size 26 to store the frequency of each character from 'a' to 'z'.
Iterate through the input string and increment the corresponding index in the array for each character encountered.
Output the array of frequencies for each test case.
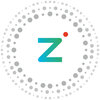
Asked in Zenoti

Q. Given a string of digits, find the length of the longest substring composed of the same digit. For example, given the string 01241111XXXXXPHONE_NUMBER, the output should be 4 because 1111 is the longest substri...
read moreFind the length of longest substring composed of same digit within a string of digits.
Iterate through the string and keep track of the current digit and its count
Update the maximum count whenever a new digit is encountered
Return the maximum count
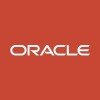
Asked in Oracle

Q. Greatest Common Divisor Problem Statement
You are tasked with finding the greatest common divisor (GCD) of two given numbers 'X' and 'Y'. The GCD is defined as the largest integer that divides both of the given...read more
Find the greatest common divisor (GCD) of two given numbers 'X' and 'Y'.
Implement a function to calculate the GCD of two numbers using Euclidean algorithm
Iteratively find the remainder of dividing the larger number by the smaller number until the remainder is 0
The last non-zero remainder is the GCD of the two numbers
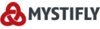
Asked in Mystifly Consulting

Q. On a dark night, 4 people want to cross a bridge and they have only one torch. At a time at max 2 people can cross the bridge. The time required for each individual to cross the bridge is 1, 2, 5, and 10 minute...
read more4 people with different crossing times need to cross a bridge with one torch. Only 2 can cross at a time. What's the minimum time?
The person with the longest time should always be accompanied by someone else
The two fastest people should cross together to save time
The torch needs to be brought back to the starting side every time two people cross
The total time taken will be the sum of all individual crossing times
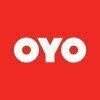
Asked in Oyo Rooms

Q. Boolean Matrix Transformation Challenge
Given a 2-dimensional boolean matrix mat
of size N x M, your task is to modify the matrix such that if any element is 1, set its entire row and column to 1. Specifically,...read more
Modify a boolean matrix such that if any element is 1, set its entire row and column to 1.
Iterate through the matrix and mark rows and columns to be modified
Use additional arrays to keep track of rows and columns to be modified
Update the matrix in-place based on the marked rows and columns
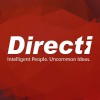
Asked in Directi

Q. Given an n x n matrix, where every row and column is sorted in increasing order, how do you decide whether a given number x is in the matrix with linear time complexity?
Algorithm to find if a number is present in a sorted n x n matrix with linear time complexity.
Start with the top right element
Compare the element with x
If equal, return its position
If e < x, move down (if out of bounds, return false)
If e > x, move left (if out of bounds, return false)
Repeat till element is found or returned false
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
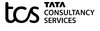
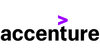
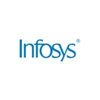
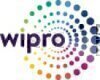
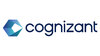
Top Interview Questions for Software Engineer Related Skills
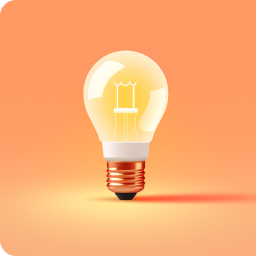
Calculate your in-hand salary
Confused about how your in-hand salary is calculated? Enter your annual salary (CTC) and get your in-hand salary
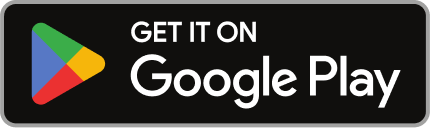
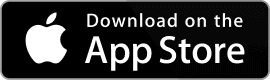
Reviews
Interviews
Salaries
Users
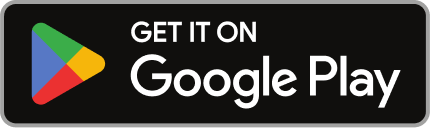
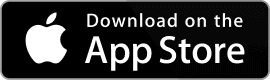