Software Engineer
8000+ Software Engineer Interview Questions and Answers
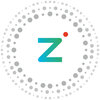
Asked in Zenoti

Q. An API is running slow, but the underlying stored procedure is optimized. How would you debug this API's performance, neglecting resolve and connect times?
To debug the slow behavior of the API, analyze network latency, check for database issues, monitor server performance, and review code for potential bottlenecks.
Analyze network latency to identify any issues with data transmission.
Check for database issues such as slow queries or insufficient indexing.
Monitor server performance to ensure it has enough resources and is not overloaded.
Review the code for potential bottlenecks, such as inefficient algorithms or excessive databas...read more
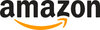
Asked in Amazon

Q. Loot Houses Problem Statement
A thief is planning to steal from several houses along a street. Each house has a certain amount of money stashed. However, the thief cannot loot two adjacent houses. Determine the...read more
Determine the maximum amount of money a thief can steal from houses without looting two consecutive houses.
Create an array 'dp' to store the maximum money that can be stolen up to the i-th house.
Iterate through the houses and update 'dp' based on whether the current house is stolen or not.
Return the maximum value in 'dp' as the answer.
Example: For input N=4 and values=[6, 7, 1, 30], the output should be 36.
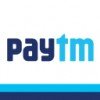
Asked in Paytm

Q. Generate Binary Strings with No Consecutive 1s
Given an integer K
, your task is to produce all binary strings of length 'K' that do not contain consecutive '1's.
Input:
The input begins with an integer 'T', the...read more
Generate all binary strings of length 'K' with no consecutive '1's in lexicographically increasing order.
Use backtracking to generate all possible binary strings without consecutive '1's.
Start with an empty string and recursively add '0' or '1' based on the condition.
Keep track of the current string and its length to ensure no consecutive '1's are added.
Sort the generated strings in lexicographically increasing order before outputting.
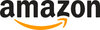
Asked in Amazon

Q. Merge Two Sorted Linked Lists Problem Statement
You are provided with two sorted linked lists. Your task is to merge them into a single sorted linked list and return the head of the combined linked list.
Input:...read more
Merge two sorted linked lists into a single sorted linked list with constant space complexity and linear time complexity.
Create a dummy node to start the merged list
Compare the values of the two linked lists and append the smaller value to the merged list
Move the pointer of the merged list and the pointer of the smaller value's linked list
Continue this process until one of the linked lists is fully traversed
Append the remaining elements of the other linked list to the merged ...read more
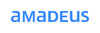
Asked in Amadeus

Q. Matrix Transformation Problem Statement
Given a 2-dimensional matrix of size NxN containing 0s and 1s, the task is to modify the matrix such that, if an element is 1, set its entire row and column to 1. In the ...read more
Modify a matrix by setting entire row and column to 1 if an element is 1, then count the number of 1s.
Iterate through the matrix to find elements with value 1
Use two arrays to keep track of rows and columns to be modified
Update the matrix by setting entire row and column to 1 if an element is 1
Count the number of 1s in the modified matrix
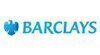
Asked in Barclays

Q. Sum of Two Elements Equals the Third
Determine if a given array contains a valid triplet of integers where two elements sum up to the third. Specifically, find indices i, j, and k such that i != j
, j != k
, and ...read more
Check if a given array contains a valid triplet where two elements sum up to the third.
Iterate through all possible triplets in the array and check if any of the conditions are satisfied.
Use nested loops to compare each element with every other element in the array.
Handle cases where the elements are not distinct by ensuring i != j, j != k, and i != k.
Software Engineer Jobs
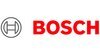

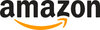

Asked in NXP Semiconductors

Q. City of Happy People Problem Statement
Imagine a city where the happiness of each resident is described by a numerical value. Ninja, who is visiting this city, is interested in forming groups of people such tha...read more
The problem is to find the number of ways to form a group of people such that the overall happiness of the group falls within a given range.
Iterate through all possible subsets of the given array/list
Calculate the sum of happiness values for each subset
Count the number of subsets whose sum falls within the given range
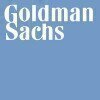
Asked in Goldman Sachs

Q. Merge Overlapping Intervals Problem Statement
Given a specified number of intervals, where each interval is represented by two integers denoting its boundaries, the task is to merge all overlapping intervals an...read more
Merge overlapping intervals and return sorted list of merged intervals.
Identify overlapping intervals based on start and end times
Merge overlapping intervals to form new intervals
Sort the merged intervals in ascending order of start times
Share interview questions and help millions of jobseekers 🌟
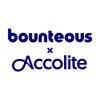
Asked in Bounteous x Accolite

Q. Topological Sort Problem Statement
You are given a directed acyclic graph (DAG). Your task is to perform topological sorting of the graph and return any valid ordering.
Explanation:
A directed acyclic graph is ...read more
Implement a function to perform topological sorting on a directed acyclic graph (DAG) and return any valid ordering.
Create a graph representation using adjacency list or matrix
Perform depth-first search (DFS) to find the topological ordering
Use a stack to keep track of the ordering
Return the ordering as an array of integers
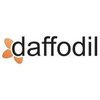
Asked in Daffodil Software

Q. Find the Second Largest Element
Given an array or list of integers 'ARR', identify the second largest element in 'ARR'.
If a second largest element does not exist, return -1.
Example:
Input:
ARR = [2, 4, 5, 6, ...read more
The task is to find the second largest element in an array of integers.
Iterate through the array and keep track of the largest and second largest elements.
Initialize the largest and second largest variables with the first two elements of the array.
Compare each element with the largest and second largest variables and update them accordingly.
Return the second largest element at the end.
Handle the case where no second largest element exists.
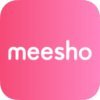
Asked in Meesho

Q. Idempotent Matrix Verification
Determine if a given N * N matrix is an idempotent matrix. A matrix is considered idempotent if it satisfies the following condition:
M * M = M
Input:
The first line contains a si...read more
Check if a given matrix is idempotent by verifying if M * M equals M.
Calculate the product of the matrix with itself and compare it with the original matrix.
If the product equals the original matrix, then it is idempotent.
Iterate through the matrix elements to perform the necessary calculations.
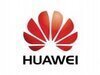
Asked in Huawei Technologies

Q. If there are 200 fishes in an aquarium, and 99% are red, how many fishes have to be removed to make the red fishes 98% of the aquarium?
To make the red fishes 98%, 50 fishes have to be removed from the aquarium.
Calculate 1% of 200 fishes to find out how many fishes represent 1%.
Multiply the result by 2 to find out how many fishes represent 2%.
Subtract the result from 200 to find out how many fishes represent 98%.
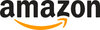
Asked in Amazon

Q. Best Time to Buy and Sell Stock Problem Statement
Given an array prices
representing the prices of a stock where each element indicates the price at a given minute, determine the maximum profit you can achieve ...read more
Find the maximum profit by buying and selling a stock once based on given prices.
Iterate through the prices array and keep track of the minimum price seen so far and the maximum profit achievable.
Calculate the profit by subtracting the current price from the minimum price and update the maximum profit if needed.
Return the maximum profit, ensuring it is not negative.
Example: prices = [2, 100, 150, 120], Buy at 2, sell at 150, profit = 150 - 2 = 148.
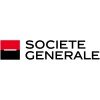

Q. Common Elements Between Array of Strings Problem Statement
Given two 1-dimensional arrays containing strings of lowercase alphabets, determine and return the elements that are common in both arrays, i.e., the s...read more
Given two arrays of strings, find and return the common elements in the order they appear in the second array.
Iterate through the strings in the second array and check if they exist in the first array.
Maintain a set to keep track of common elements to avoid duplicates.
Return the common elements as a single space-separated string in the order they appear in the second array.
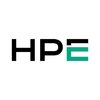
Asked in Hewlett Packard Enterprise

Q. Merge Sort Problem Statement
You are given a sequence of numbers, ARR
. Your task is to return a sorted sequence of ARR
in non-descending order using the Merge Sort algorithm.
Explanation:
The Merge Sort algorit...read more
Implement Merge Sort algorithm to sort a sequence of numbers in non-descending order.
Use Divide and Conquer approach to recursively divide the input array into two halves.
Merge the sorted halves to produce a completely sorted array.
Ensure the time complexity is O(n log n) for sorting.
Handle edge cases like empty array, single element array, etc.
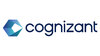
Asked in Cognizant

Q. Nth Fibonacci Number Problem Statement
Calculate the Nth term in the Fibonacci sequence, where the sequence is defined as follows: F(n) = F(n-1) + F(n-2)
, with initial conditions F(1) = F(2) = 1
.
Input:
The inp...read more
Calculate the Nth Fibonacci number efficiently using dynamic programming.
Use dynamic programming to store previously calculated Fibonacci numbers to avoid redundant calculations.
Start with base cases F(1) and F(2) as 1, then iterate to calculate subsequent Fibonacci numbers.
Return the Nth Fibonacci number as the final result.
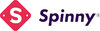
Asked in Spinny

Count Ways to Reach the Nth Stair
Given a number of stairs, starting from the 0th stair, calculate the number of distinct ways you can reach the Nth stair. You can climb either one step or two steps at a time.
The question is about calculating the number of distinct ways to reach the Nth stair by climbing one or two steps at a time.
Use dynamic programming to solve this problem efficiently.
Define a recursive function to calculate the number of ways to reach each stair.
Consider base cases for 0 and 1 stairs.
Use memoization to store intermediate results and avoid redundant calculations.
Handle large values of N by taking modulo 10^9+7 of the final result.
Example: For N = 3, the number ...read more
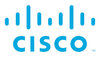
Asked in Cisco

Q. Covid Vaccination Distribution Problem
As the Government ramps up vaccination drives to combat the second wave of Covid-19, you are tasked with helping plan an effective vaccination schedule. Your goal is to ma...read more
Given constraints and rules, maximize vaccines administered on a specific day during a vaccination drive.
Iterate through each test case and calculate the maximum number of vaccines administered on the specified day.
Distribute the available vaccines evenly across the days while adhering to the rules.
Ensure that the sum of vaccines administered does not exceed the maximum allowed.
Maximize the vaccines administered on the specified day to meet the requirements.
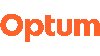
Asked in Optum Global Solutions

Q. Consonant Counting Problem Statement
Given a string STR
comprising uppercase and lowercase characters and spaces, your task is to count the number of consonants in the string.
A consonant is defined as an Engli...read more
Count the number of consonants in a given string containing uppercase and lowercase characters and spaces.
Iterate through each character in the string and check if it is a consonant (not a vowel).
Keep a count of the consonants encountered while iterating through the string.
Return the total count of consonants at the end.
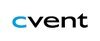
Asked in Cvent

Q. Detect Cycle in Undirected Graph Problem Statement
You are provided with an undirected graph composed of 'N' vertices and 'M' edges, where vertices are labeled from 1 to 'N'.
Your task is to determine if there ...read more
Detect cycle in an undirected graph by checking for a path that begins and ends at the same vertex.
Use Depth First Search (DFS) to traverse the graph and detect cycles.
Maintain a visited set to keep track of visited vertices and a parent pointer to avoid visiting the same vertex twice.
If a visited vertex is encountered that is not the parent of the current vertex, a cycle is detected.
Return 'Yes' if a cycle is found, 'No' otherwise.
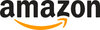
Asked in Amazon

Q. Find Row With Maximum 1's in a Sorted 2D Matrix
You are provided with a 2D matrix containing only the integers 0 or 1. The matrix has dimensions N x M, and each row is sorted in non-decreasing order. Your objec...read more
Find the row with the maximum number of 1's in a sorted 2D matrix.
Iterate through each row of the matrix and count the number of 1's in each row.
Keep track of the row index with the maximum number of 1's seen so far.
Return the index of the row with the maximum number of 1's.
If multiple rows have the same number of 1's, return the row with the smallest index.
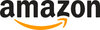
Asked in Amazon

Q. Longest Common Subsequence Problem Statement
Given two strings, S
and T
with respective lengths M
and N
, your task is to determine the length of their longest common subsequence.
A subsequence is a sequence tha...read more
The task is to find the length of the longest common subsequence between two given strings.
Use dynamic programming to solve this problem efficiently.
Create a 2D array to store the lengths of longest common subsequences of substrings.
Iterate through the strings to fill the array and find the length of the longest common subsequence.
Example: For strings 'abcde' and 'ace', the longest common subsequence is 'ace' with length 3.
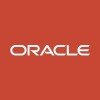
Asked in Oracle

Q. Merge K Sorted Arrays Problem Statement
Given 'K' different arrays that are individually sorted in ascending order, merge all these arrays into a single array that is also sorted in ascending order.
Input
The f...read more
Merge K sorted arrays into a single sorted array.
Iterate through all arrays and merge them into a single array.
Use a min heap to efficiently merge the arrays.
Implement a custom comparator function for the min heap.
Time complexity can be optimized to O(N log K) using min heap.

Asked in JPMorgan Chase & Co.

Q. Reverse Words in a String: Problem Statement
You are given a string of length N
. Your task is to reverse the string word by word. The input may contain multiple spaces between words and may have leading or trai...read more
Reverse words in a string word by word, removing leading/trailing spaces and extra spaces between words.
Split the input string by spaces to get individual words
Reverse the order of the words
Join the reversed words with a single space in between
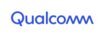
Asked in Qualcomm

Q. What is scheduling? List different types of scheduling
Scheduling is the process of allocating resources to tasks based on priority and availability.
Different types of scheduling include: preemptive and non-preemptive scheduling, round-robin scheduling, priority scheduling, and deadline scheduling.
Preemptive scheduling allows higher priority tasks to interrupt lower priority tasks, while non-preemptive scheduling does not.
Round-robin scheduling allocates a fixed time slice to each task in a cyclic manner.
Priority scheduling assig...read more
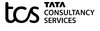
Asked in TCS

Q. Given a string S consisting of '*' and '#', find the maximum number of '*' or '#' to make it a valid string. The string is considered valid if the number of '*' and '#' are equal. The '*' and '#' can be at any...
read moreGiven a string of '*' and '#', find the maximum number of '*' or '#' to make it a valid string with equal count of both.
Count the number of '*' and '#' in the string
Return the minimum count of '*' and '#' as the maximum valid count
If the count of '*' and '#' is already equal, return that count as the maximum valid count
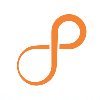
Asked in Persistent Systems

Q. Index of First Occurrence Problem Statement
Given two strings A
and B
, determine the index of the first occurrence of A
in B
. If A
is not present in B
, return -1.
Example:
Input:
A = "bc", B = "abcddbc"
Output:...read more
Find the index of the first occurrence of string A in string B.
Iterate through string B and check if a substring of length equal to A matches A.
Return the index of the first occurrence of A in B, or -1 if not found.
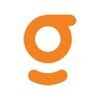
Asked in Grey Orange

Q. Print All Permutations of a String
Given an input string STR
, generate and print all possible permutations of the string.
Input:
str
Output:
All permutations of the input string, each on a new line.
Example:
In...read more
Generate and print all possible permutations of a given input string.
Use recursion to generate all permutations by swapping characters in the string.
Maintain a visited array to keep track of characters already used in a particular permutation.
Print each permutation as it is generated.
Handle duplicate characters by skipping swapping if the character is already in the current position.
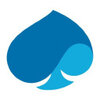
Asked in Capgemini Engineering

Q. Reverse a String Problem Statement
Given a string STR
containing characters from [a-z], [A-Z], [0-9], and special characters, determine the reverse of the string.
Input:
The input starts with a single integer '...read more
Reverse a given string containing characters from [a-z], [A-Z], [0-9], and special characters.
Iterate through the characters of the string from end to start and append them to a new string to get the reversed string.
Use built-in functions like reverse() or StringBuilder in languages like Java for efficient reversal.
Handle special characters and numbers along with alphabets while reversing the string.
Ensure to print each reversed string on a separate line as per the output for...read more
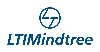
Asked in LTIMindtree

Q. Prime Numbers Identification
Given a positive integer N
, your task is to identify all prime numbers less than or equal to N
.
Explanation:
A prime number is a natural number greater than 1 that has no positive d...read more
Identify all prime numbers less than or equal to a given positive integer N.
Iterate from 2 to N and check if each number is prime
Use the Sieve of Eratosthenes algorithm for efficient prime number identification
Optimize by only checking up to the square root of N for divisors
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
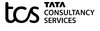
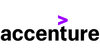
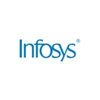
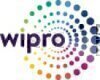
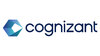
Top Interview Questions for Software Engineer Related Skills
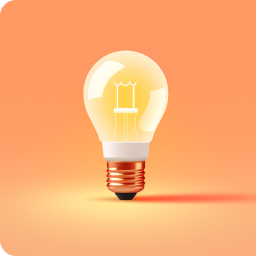
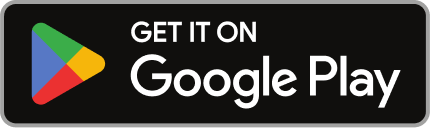
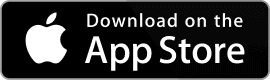
Reviews
Interviews
Salaries
Users
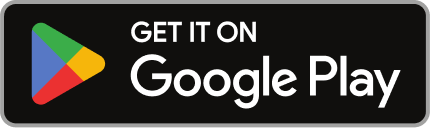
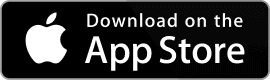