Software Engineer
1500+ Software Engineer Interview Questions and Answers for Freshers
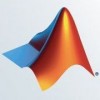
Asked in MathWorks

Q. Car Pooling Capacity Problem
You are a cab driver with a car that initially has 'C' empty seats. The car moves in a straight line towards the forward direction only. Your job is to determine if it is possible t...read more
Determine if it is possible to accommodate all passenger trips within a car's capacity without exceeding it at any point.
Iterate through each trip and keep track of the total number of passengers in the car at each point.
Check if the total number of passengers exceeds the car capacity at any point.
Return 'True' if all trips can be accommodated within the car capacity, otherwise return 'False'.
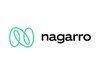
Asked in Nagarro

Q. Count Ways to Reach the N-th Stair Problem Statement
You are provided with a number of stairs, and initially, you are located at the 0th stair. You need to reach the Nth stair, and you can climb one or two step...read more
The problem involves finding the number of distinct ways to climb N stairs by taking 1 or 2 steps at a time.
Use dynamic programming to solve the problem efficiently.
The number of ways to reach the Nth stair is the sum of the number of ways to reach the (N-1)th stair and the (N-2)th stair.
Handle base cases for N=0 and N=1 separately.
Consider using modulo operation to avoid overflow for large values of N.
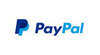
Asked in PayPal

Q. Kth Largest Element Problem
Given an array containing N
distinct positive integers and a number K
, determine the Kth largest element in the array.
Example:
Input:
N = 6, K = 3, array = [2, 1, 5, 6, 3, 8]
Output...read more
Find the Kth largest element in an array of distinct positive integers.
Sort the array in non-increasing order and return the Kth element.
Ensure all elements in the array are distinct.
Handle multiple test cases efficiently.

Asked in Tech Vedika

Q. Left Rotations of an Array
You are given an array consisting of N
elements and need to perform Q
queries on that array. Each query consists of an integer indicating the number of elements by which the array sho...read more
Perform left rotations on an array based on given queries.
Create a function that takes the array, number of elements, number of queries, and the queries as input.
For each query, rotate the array by the specified number of elements to the left.
Return the final array after each rotation query.
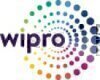
Asked in Wipro

Q. Determine Pythagoras' Position for Parallelogram Formation
Euclid, Pythagoras, Pascal, and Monte decide to gather in a park. Initially, Pascal, Monte, and Euclid choose three different spots. Pythagoras, arrivi...read more
Calculate Pythagoras' coordinates to form a parallelogram with Euclid, Pascal, and Monte.
Calculate the midpoint of the line segment between Euclid and Monte to get the coordinates of Pythagoras.
Use the formula: x = x3 + (x2 - x1), y = y3 + (y2 - y1) to find Pythagoras' coordinates.
Ensure that the coordinates of Pythagoras satisfy the conditions for forming a parallelogram.
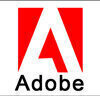
Asked in Adobe

Q. LCA in a Binary Search Tree
You are given a binary search tree (BST) containing N nodes. Additionally, you have references to two nodes, P and Q, within this BST.
Your task is to determine the Lowest Common Anc...read more
The task is to find the Lowest Common Ancestor (LCA) of two nodes in a Binary Search Tree (BST).
Traverse the BST from the root to find the LCA of nodes P and Q.
Compare the values of nodes P and Q with the current node's value to determine the LCA.
If both nodes are on the same side of the current node, move to that side; otherwise, the current node is the LCA.
Example: For input 2 3 and BST 1 2 3 4 -1 5 6 -1 7 -1 -1 -1 -1 -1 -1, the LCA is node 1.
Software Engineer Jobs
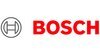

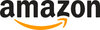

Asked in VMware Software

Q. Reverse the String Problem Statement
You are given a string STR
which contains alphabets, numbers, and special characters. Your task is to reverse the string.
Example:
Input:
STR = "abcde"
Output:
"edcba"
Input...read more
The task is to reverse a given string containing lowercase letters, uppercase letters, digits, and special characters.
Iterate through the string from the last character to the first character and append each character to a new string.
Alternatively, you can use built-in string reversal functions or methods available in your programming language.
To solve the follow-up question with O(1) space complexity, you can use a two-pointer approach. Initialize two pointers, one at the be...read more
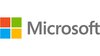
Asked in Microsoft Corporation

Q. You have a cuboid (m*n*p) where each block contains a metallic ball. X-rays are passed from the front face, resulting in a boolean matrix1 of size m*p, where elements are set if there is a black spot. Another m...
read moreYes, it is possible to get the accurate result from the given data.
The coordinates (i,j,k) where metallic balls are present can be determined by finding the set elements in both matrix1 and matrix2.
Additional data is not required as the given data is sufficient to determine the coordinates.
The coordinates can be obtained by iterating through the elements of matrix1 and matrix2 and checking for set elements.
Share interview questions and help millions of jobseekers 🌟
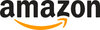
Asked in Amazon

Q. Connecting Ropes with Minimum Cost
You are given 'N' ropes, each of varying lengths. The task is to connect all ropes into one single rope. The cost of connecting two ropes is the sum of their lengths. Your obj...read more
Given 'N' ropes of varying lengths, find the minimum cost to connect all ropes into one single rope.
Sort the lengths of ropes in ascending order.
Keep connecting the two shortest ropes at each step.
Add the cost of connecting the two ropes to the total cost.
Repeat until all ropes are connected.
Return the total cost as the minimum cost to connect all ropes.

Asked in Visa

Q. Given two basketball game scenarios and a probability 'p' of making a basket, determine the condition where game 1 is preferable to game 2. In game 1, you have one trial to make a basket to win. In game 2, you...
read moreComparing 2 basketball game scenarios with different number of trials and baskets required to win
Calculate the probability of winning in each game scenario using binomial distribution formula
Compare the probabilities to determine which game scenario is preferable
In game1, the probability of winning is p. In game2, the probability of winning is the sum of probabilities of making 2 or 3 baskets
If p is high, game1 is preferable. If p is low, game2 is preferable
For example, if p ...read more
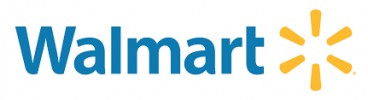
Asked in Walmart

Q. Kth Largest Element Problem Statement
Ninja enjoys working with numbers, and Alice challenges him to find the Kth largest value from a given list of numbers.
Input:
The first line contains an integer 'T', repre...read more
The task is to find the Kth largest element from a given list of numbers for each test case.
Read the number of test cases 'T'
For each test case, read the number of elements 'N' and the Kth largest number to find 'K'
Sort the array in descending order and output the Kth element
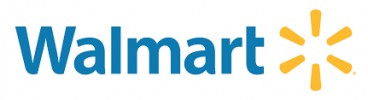
Asked in Walmart

Q. Minimum Cost to Buy Oranges Problem Statement
You are given a bag of capacity 'W' kg and a list 'cost' of costs for packets of oranges with different weights. Each element at the i-th position in the list indic...read more
Find the minimum cost to purchase a specific weight of oranges given the cost of different weight packets.
Iterate through the list of costs and find the minimum cost to achieve the desired weight
Keep track of the total cost while considering available packet weights
Return -1 if it is not possible to buy exactly the desired weight
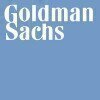
Asked in Goldman Sachs

Q. Minimum Umbrellas Problem
You are provided with ‘N’ types of umbrellas, where each umbrella type can shelter a certain number of people. Given an array UMBRELLA
that indicates the number of people each umbrella...read more
The problem involves determining the minimum number of umbrellas required to shelter a specific number of people based on the capacity of each umbrella.
Iterate through the array of umbrella capacities to find the combination that covers exactly 'M' people.
Keep track of the minimum number of umbrellas used to cover 'M' people.
If it is not possible to cover 'M' people exactly, return -1.
Example: For input [2, 3, 4] and M=5, the minimum number of umbrellas required is 2 (2-capac...read more
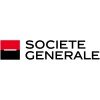

Q. Decimal to Octal Conversion Problem Statement
Convert a given decimal number into its equivalent octal representation.
Explanation:
The octal number system is a base-8 system, meaning each digit ranges from 0 t...read more
Convert decimal numbers to octal representation.
Iterate through each test case and use built-in functions to convert decimal to octal.
Ensure the output is in base-8 system with digits ranging from 0 to 7.
Handle constraints such as number of test cases and range of decimal numbers.
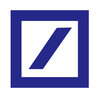
Asked in Deutsche Bank

Q. Longest Consecutive Sequence Problem Statement
You are provided with an unsorted array/list ARR
of N
integers. Your task is to determine the length of the longest consecutive sequence present in the array.
Expl...read more
The task is to find the length of the longest consecutive sequence in an unsorted array of integers.
Sort the array to bring consecutive numbers together
Iterate through the sorted array and keep track of the current consecutive sequence length
Update the maximum length if a longer sequence is found
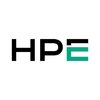
Asked in Hewlett Packard Enterprise

Q. Middle of a Linked List
You are given the head node of a singly linked list. Your task is to return a pointer pointing to the middle of the linked list.
If there is an odd number of elements, return the middle ...read more
Return the middle node of a singly linked list, considering odd and even number of elements.
Traverse the linked list with two pointers, one moving twice as fast as the other
When the fast pointer reaches the end, the slow pointer will be at the middle
Return the node pointed by the slow pointer
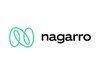
Asked in Nagarro

Q. Reverse Alternate K Nodes Problem Statement
You are given a singly linked list of integers along with a positive integer 'K'. The task is to modify the linked list by reversing every alternate 'K' nodes of the ...read more
Reverse every alternate K nodes in a singly linked list of integers.
Traverse the linked list in groups of K nodes and reverse every alternate group.
Handle cases where the number of remaining nodes is less than K.
Ensure to properly link the reversed groups to maintain the integrity of the linked list.
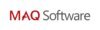
Asked in MAQ Software

Q. Print Series Problem Statement
Given two positive integers N
and K
, your task is to generate a series of numbers by subtracting K
from N
until the result is 0 or negative, then adding K
back until it reaches N
...read more
Generate a series of numbers by subtracting K from N until 0 or negative, then adding K back to reach N without using loops.
Create a recursive function to generate the series.
Subtract K from N until N is 0 or negative, then add K back until N is reached.
Return the series as an array of integers.
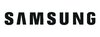
Asked in Samsung

Q. Triplets with Given Sum Problem
Given an array or list ARR
consisting of N
integers, your task is to identify all distinct triplets within the array that sum up to a specified number K
.
Explanation:
A triplet i...read more
The task is to identify all distinct triplets within an array that sum up to a specified number.
Iterate through the array and use nested loops to find all possible triplets.
Check if the sum of the triplet equals the specified number.
Print the valid triplets or return -1 if no triplet exists.
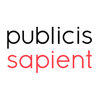
Asked in Publicis Sapient

Q. Cycle Detection in Undirected Graph Problem Statement
You are provided with an undirected graph containing 'N' vertices and 'M' edges. The vertices are numbered from 1 to 'N'. Your objective is to determine whe...read more
Detect cycles in an undirected graph with given vertices and edges.
Use Depth First Search (DFS) to traverse the graph and detect cycles.
Maintain a visited array to keep track of visited vertices and a parent array to keep track of the parent of each vertex.
If while traversing, you encounter a visited vertex that is not the parent of the current vertex, then a cycle exists.
Consider edge cases like disconnected graphs and self-loops.
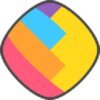
Asked in ShareChat

Q. Kruskal’s Minimum Spanning Tree Algorithm Problem Statement
You are given a connected undirected weighted graph. Your task is to determine the weight of the minimum spanning tree of this graph.
A minimum spanni...read more
The task is to determine the weight of the minimum spanning tree of a given connected undirected weighted graph.
Implement Kruskal's algorithm to find the minimum spanning tree weight.
Sort the edges based on their weights in non-decreasing order.
Iterate through the sorted edges and add them to the MST if they don't form a cycle.
Keep track of the total weight of the MST and return it as the output.
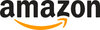
Asked in Amazon

Q. Maximum Subarray Sum Problem Statement
Given an array arr
of length N
consisting of integers, find the sum of the subarray (including empty subarray) with the maximum sum among all subarrays.
Explanation:
A sub...read more
Find the sum of the subarray with the maximum sum among all subarrays in an array of integers.
Iterate through the array and keep track of the maximum sum subarray seen so far.
At each index, decide whether to include the current element in the subarray or start a new subarray.
Update the maximum sum subarray if a new maximum is found.
Consider edge cases like all negative numbers in the array.
Example: For input arr = [-2, 1, -3, 4, -1], the maximum subarray sum is 4.
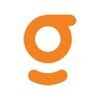
Asked in Grey Orange

Q. Rectangle Area Calculation
Given a list of rectangles, where each rectangle is represented by an array of four integers indicating its bottom-left and top-right corners, calculate the total area covered by all ...read more
Calculate total area covered by a list of rectangles on a plane.
Iterate through each rectangle and calculate its area
Check for overlapping areas between rectangles
Sum up the total area covered by all rectangles
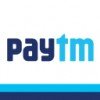
Asked in Paytm

Q. SpecialStack Design Problem
Design a stack that efficiently supports the getMin() operation in O(1) time with O(1) extra space. This stack should include the following operations: push(), pop(), top(), isEmpty(...read more
Design a stack that supports getMin() operation in O(1) time with O(1) extra space using inbuilt stack data structure.
Use two stacks - one to store the actual data and another to store the minimum value at each level.
When pushing a new element, check if it is smaller than the current minimum and update the minimum stack accordingly.
When popping an element, also pop the top element from the minimum stack if it matches the popped element.
For getMin() operation, simply return th...read more
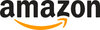
Asked in Amazon

Q. String Transformation Problem
Given a string (STR
) of length N
, you are tasked to create a new string through the following method:
Select the smallest character from the first K
characters of STR
, remove it fr...read more
Given a string and an integer K, create a new string by selecting the smallest character from the first K characters of the input string and repeating the process until the input string is empty.
Iterate through the input string, selecting the smallest character from the first K characters each time.
Remove the selected character from the input string and append it to the new string.
Continue this process until the input string is empty.
Return the final new string formed.
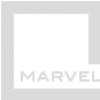
Asked in Marvel Realtors

Q. There are 2 processors each executing a separate program. Processor 1: int a=10; int *ptr = &a; ptr is written into file1; processor sleeps for 10sec; printf("%d",a); Processor 2: int *p; file1 is read and cont...
read moreThe output will be 5.
Processor 1 initializes variable 'a' with a value of 10 and creates a pointer 'ptr' pointing to 'a'.
Processor 1 writes the value of 'ptr' into 'file1' and then sleeps for 10 seconds.
Processor 2 reads the contents of 'file1' and saves it in pointer 'p'.
Processor 2 assigns the value 5 to the memory location pointed by 'p'.
When Processor 1 wakes up and executes the printf statement, it will print the updated value of 'a', which is 5.
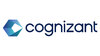
Asked in Cognizant

Q. two puzzles: 1. how can you cut a cake in 8 pieces in minimum number of cuts(answered) ,2.make 4 equilateral triangles using 6 matchsticks(answered)
1. Cut cake in 8 pieces in minimum cuts. 2. Make 4 equilateral triangles using 6 matchsticks.
1. Cut cake in half horizontally and vertically. Cut each quarter diagonally.
2. Use 3 matchsticks to form a triangle. Use the other 3 to connect the centers of each triangle.
Both puzzles require creative thinking and problem-solving skills.
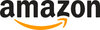
Asked in Amazon

Q. Unique Element In Sorted Array
Nobita wants to impress Shizuka by correctly guessing her lucky number. Shizuka provides a sorted list where every number appears twice, except for her lucky number, which appears...read more
Find the unique element in a sorted array where all other elements appear twice.
Iterate through the array and XOR all elements to find the unique element.
Use a hash set to keep track of elements and find the unique one.
Sort the array and check adjacent elements to find the unique one.
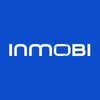
Asked in InMobi

Q. In a 10-floor office building with 300 employees per floor, 3000 employees arrive between 7-8 AM and wait for 3 lifts to go to their cubicles. People are experiencing long wait times. How would you optimize the...
read moreOptimize lift usage to reduce wait times for employees entering a 10-floor building.
Implement a smart scheduling system that prioritizes lifts based on the number of people waiting on each floor.
Introduce a destination dispatch system where employees select their floor before entering the lift, grouping them by destination.
Increase the number of lifts during peak hours or consider adding express lifts that service only certain floors.
Utilize real-time data to adjust lift allo...read more
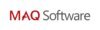
Asked in MAQ Software

Q. Remove Invalid Parentheses
Given a string containing only parentheses and letters, your goal is to remove the minimum number of invalid parentheses to make the input string valid and return all possible valid s...read more
Given a string with parentheses and letters, remove minimum invalid parentheses to make it valid and return all possible valid strings.
Use BFS to explore all possible valid strings by removing parentheses one by one
Keep track of visited strings to avoid duplicates
Return all unique valid strings obtained after removing minimum number of parentheses
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
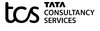
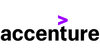
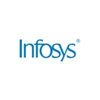
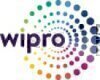
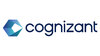
Top Interview Questions for Software Engineer Related Skills
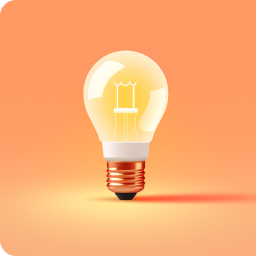
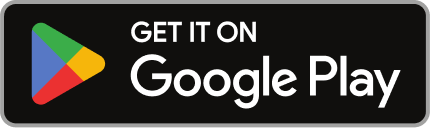
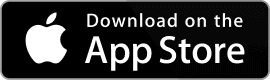
Reviews
Interviews
Salaries
Users
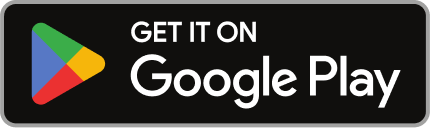
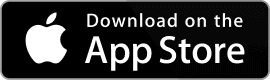