Software Engineer Intern
100+ Software Engineer Intern Interview Questions and Answers
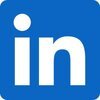
Asked in LinkedIn

Q. Check if Two Trees are Mirror
Given two arbitrary binary trees, your task is to determine whether these two trees are mirrors of each other.
Explanation:
Two trees are considered mirror of each other if:
- The r...read more
Check if two binary trees are mirrors of each other based on specific criteria.
Compare the roots of both trees.
Check if the left subtree of the first tree is the mirror of the right subtree of the second tree.
Verify if the right subtree of the first tree is the mirror of the left subtree of the second tree.
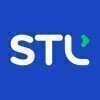
Asked in Sterlite Technologies

Q. Partition Array Minimizing Subset Sum Difference
Given an array containing N non-negative integers, your task is to partition this array into two subsets such that the absolute difference between their sums is ...read more
Partition array into two subsets to minimize absolute difference in sum.
Sort the array in non-decreasing order.
Calculate the total sum of all elements in the array.
Iterate through the array and find the subset sum closest to half of the total sum.
The minimum absolute difference will be the absolute difference between total sum and twice the subset sum.
Software Engineer Intern Interview Questions and Answers for Freshers
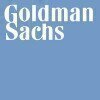
Asked in Goldman Sachs

Q. Zero Matrix Problem Statement
You are given a matrix MATRIX
of dimension N x M
. Your task is to make all the elements of row i
and column j
equal to 0
if any element in the ith
row or jth
column of the matrix i...read more
The task is to modify a given matrix such that if any element in a row or column is 0, then make all elements in that row and column 0.
Iterate through the matrix and keep track of rows and columns that contain 0s
After the first iteration, iterate through the tracked rows and columns and set all elements to 0
Handle the case where the first row or column contains 0 separately
To solve with O(1) space complexity, use the first row and first column of the matrix to track the rows ...read more
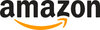
Asked in Amazon

Q. Connecting Ropes with Minimum Cost
You are given 'N' ropes, each of varying lengths. The task is to connect all ropes into one single rope. The cost of connecting two ropes is the sum of their lengths. Your obj...read more
The problem is to connect N ropes of different lengths into one rope with minimum cost.
Sort the array of rope lengths in ascending order.
Initialize a variable to keep track of the total cost.
While there are more than one rope remaining, take the two shortest ropes and connect them.
Add the cost of connecting the two ropes to the total cost.
Replace the two shortest ropes with the connected rope.
Repeat the above steps until only one rope remains.
Return the total cost.
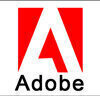
Asked in Adobe

Q. Search In Rotated Sorted Array Problem Statement
Given a rotated sorted array ARR
of size 'N' and an integer 'K', determine the index at which 'K' is present in the array.
Note:
1. If 'K' is not present in ARR,...read more
Given a rotated sorted array, find the index of a given integer 'K'.
Use binary search to efficiently find the index of 'K'.
Consider the rotation of the array while performing the search.
Handle cases where 'K' is not present in the array by returning -1.
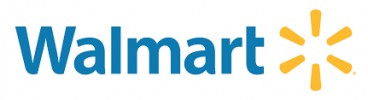
Asked in Walmart

Q. Similar Strings Problem Statement
Determine whether two given strings, A
and B
, both of length N
, are similar by returning a 1 if they are, otherwise return a 0.
Explanation:
String A
is similar to string B
if ...read more
The task is to determine if two strings are similar based on the given conditions.
Check if the strings are equal, if yes, return 1
Divide both strings into two parts and check if any combination satisfies the similarity condition
If none of the conditions hold true, return 0
Software Engineer Intern Jobs
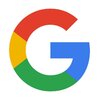
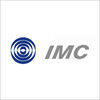
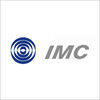
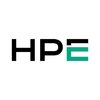
Asked in Hewlett Packard Enterprise

Q. Merge Sort Problem Statement
You are given a sequence of numbers, ARR
. Your task is to return a sorted sequence of ARR
in non-descending order using the Merge Sort algorithm.
Explanation:
The Merge Sort algorit...read more
Implement Merge Sort algorithm to sort a sequence of numbers in non-descending order.
Divide the input array into two halves recursively until each array has only one element.
Merge the sorted halves to produce a completely sorted array.
Time complexity of Merge Sort is O(n log n).
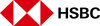
Asked in HSBC Group

Q. Find Slope Problem Statement
Given a linked list where each node represents coordinates on the Cartesian plane, your task is to determine the minimum and maximum slope between consecutive points.
Example:
Input...read more
Given a linked list of coordinates, find the starting nodes of segments with maximum and minimum slopes between consecutive points.
Traverse the linked list and calculate slopes between consecutive points
Identify the segments with maximum and minimum slopes
Return the starting nodes of these segments
Share interview questions and help millions of jobseekers 🌟
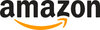
Asked in Amazon

Q. Word Presence in Sentence
Determine if a given word 'W' is present in the sentence 'S' as a complete word. The word should not merely be a substring of another word.
Input:
The first line contains an integer 'T...read more
Check if a given word is present in a sentence as a complete word, not a substring.
Split the sentence into words using spaces as delimiters.
Check if the given word matches any of the words in the sentence exactly.
Ensure the word is not part of a larger word in the sentence.
Output 'Yes' if the word is found as a complete word, 'No' otherwise.
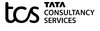
Asked in TCS

Q. Binary Palindrome Check
Given an integer N
, determine whether its binary representation is a palindrome.
Input:
The first line contains an integer 'T' representing the number of test cases.
The next 'T' lines e...read more
Check if the binary representation of a given integer is a palindrome.
Convert the integer to binary representation
Check if the binary representation is a palindrome by comparing it with its reverse
Return true if it is a palindrome, false otherwise
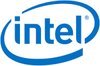
Asked in Intel

Q. Given a binary tree, find the maximum sum from root to leaf, where only the parent or the child can be included in the sum (no two level adjacent nodes will be included in the sum).
The maximum sum from root to leaf in a binary tree, where only parent or child can be included in the sum.
Use a recursive approach to traverse the binary tree.
At each node, calculate the maximum sum from its left and right child.
Compare the sums and return the maximum sum plus the value of the current node.
Repeat this process until reaching a leaf node.
Track the maximum sum encountered during the traversal.
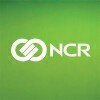
Asked in NCR Corporation

Q. What is Software Development Cycle??What are the phase in software development??
Software Development Cycle is a process of designing, creating, testing, and deploying software.
The phases of Software Development Cycle are Planning, Analysis, Design, Implementation, Testing, Deployment, and Maintenance.
Planning involves defining the project scope, goals, and requirements.
Analysis involves gathering and analyzing user requirements.
Design involves creating a detailed design of the software.
Implementation involves writing code and integrating different compon...read more
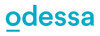
Asked in Odessa

Q. Best sorting algorithm and why and explain the logic
The best sorting algorithm is QuickSort due to its average case time complexity of O(nlogn) and low memory usage.
QuickSort is a divide and conquer algorithm that recursively partitions the array into smaller subarrays and sorts them.
It has an average case time complexity of O(nlogn) and a worst case time complexity of O(n^2).
QuickSort has low memory usage as it sorts the array in place, without requiring additional memory.
It is widely used in practice due to its efficiency an...read more
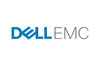
Asked in Dell EMC

Q. Describe your approach to architecting a product to solve problems in the storage industry.
Architecting a storage solution involves scalability, reliability, and performance optimization to meet industry demands.
Identify key requirements: Understand the specific needs of the storage industry, such as data volume, access speed, and security.
Choose the right architecture: Consider distributed systems for scalability, like using cloud storage solutions (e.g., AWS S3).
Implement redundancy: Use techniques like RAID or replication to ensure data durability and availabili...read more
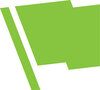
Asked in Unthinkable Solutions

Q. Describe a puzzle question involving five numbered fingers where you need to determine which finger corresponds to a given number in constant time complexity O(1).
Determine which finger corresponds to a number using a simple mapping technique for constant time retrieval.
Use an array to represent fingers: fingers = ['Thumb', 'Index', 'Middle', 'Ring', 'Pinky']
Access the finger by index: for number 1, return fingers[0] (Thumb), for 2, return fingers[1] (Index).
This approach ensures O(1) time complexity since array access is constant time.
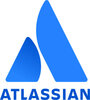
Asked in Atlassian

Q. Design a rate limiter where a user can make a maximum of 5 API calls per minute. If the API calls exceed this limit, return false; otherwise, return true. Consider variations for premium memberships.
Design a rate limiter allowing 5 API calls per minute, with variations for premium users.
Use a sliding window algorithm to track API calls within the last minute.
Store user call counts in a hash map with timestamps.
For premium users, allow a higher limit, e.g., 10 calls per minute.
Return false if the limit is exceeded, otherwise return true.
Consider edge cases like simultaneous requests at the limit.
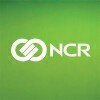
Asked in NCR Corporation

Q. What is waterfall model?? What are the phases in waterfall model??
Waterfall model is a linear sequential approach to software development.
Phases: Requirements gathering, Design, Implementation, Testing, Deployment, Maintenance
Each phase must be completed before moving to the next
No going back to previous phases
Documentation is important
Less flexible than Agile model
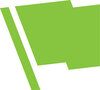
Asked in Unthinkable Solutions

Q. How would you design a store management system, including all relevant schema tables?
Design a store management system with relevant schema tables
Create a 'Store' table with attributes like store_id, name, location, etc.
Design a 'Product' table with attributes like product_id, name, price, quantity, etc.
Include a 'Sales' table to track sales transactions with attributes like sale_id, date, total_amount, etc.
Implement a 'Stock' table to manage product stock levels with attributes like stock_id, product_id, quantity_available, etc.
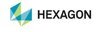
Asked in Hexagon Capability Center

Q. Find the output of the given psuedo code and other technical MCQs
Psuedo code output and technical MCQs for Software Engineer Intern position
Provide examples for technical MCQs
Psuedo code output needs to be calculated
Assess candidate's technical knowledge
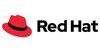
Asked in Red Hat

Q. Write a program to print all Prime Numbers up to the number provided as input using the Prime Sieve Algorithm.
Program to print all Prime Numbers up to the input number using Prime Sieve Algorithm.
Create a boolean array of size n+1 and initialize all values as true.
Loop through the array starting from 2 and mark all multiples of each prime number as false.
Print all the numbers that are still marked as true in the array.
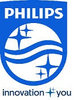
Asked in Philips Innovation Campus

Q. How would you rate your C++ skills, considering the majority of the work is in C++ and C#?
I would rate myself a 7/10 in C++, with solid understanding and practical experience in various projects.
Strong grasp of C++ fundamentals, including OOP concepts like inheritance and polymorphism.
Experience with STL (Standard Template Library) for efficient data handling, e.g., using vectors and maps.
Worked on a project involving multithreading, improving performance by implementing thread-safe data structures.
Familiar with memory management in C++, including pointers and dyn...read more
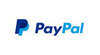
Asked in PayPal

Query to find the nth highest salary from a database
Use ORDER BY and LIMIT in the query
Consider handling ties if multiple employees have the same salary
Example: SELECT DISTINCT salary FROM employees ORDER BY salary DESC LIMIT n-1, 1
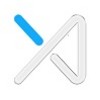
Asked in Arcesium

Q. Given a matrix, find the minimum weight path to reach the bottom right corner from the top left corner. You can only move down and right.
Minimum weight to reach bottom right corner in matrix starting from top left. You can move only down and right.
Use dynamic programming to solve the problem efficiently
Create a 2D array to store the minimum weight to reach each cell
The minimum weight to reach a cell is the minimum of the weight to reach the cell above and the cell to the left plus the weight of the current cell
The minimum weight to reach the bottom right corner is the value in the last cell of the 2D array
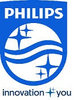
Asked in Philips Innovation Campus

Q. What are the four pillars of object-oriented programming? Explain in detail.
The 4 pillars of object oriented programming are encapsulation, inheritance, polymorphism, and abstraction.
Encapsulation: Bundling data and methods that operate on the data into a single unit.
Inheritance: Creating new classes based on existing classes, allowing for code reuse.
Polymorphism: The ability for objects of different classes to respond to the same message.
Abstraction: Hiding the complex implementation details and only showing the necessary features.
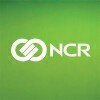
Asked in NCR Corporation

Access modifiers in Java control the visibility and accessibility of classes, methods, and variables.
There are four types of access modifiers in Java: public, private, protected, and default.
Public: accessible from any other class.
Private: accessible only within the same class.
Protected: accessible within the same package and subclasses.
Default: accessible only within the same package.
Example: public class MyClass { private int myVar; protected void myMethod() {}}
Asked in The Software Practice

Q. Describe your experience debugging a Tic Tac Toe game written in React within a Codesandbox environment.
Debugging a Tic Tac Toe game in React involves fixing state management and rendering issues.
Check state initialization: Ensure the game state is initialized correctly, e.g., `const [squares, setSquares] = useState(Array(9).fill(null));`.
Verify event handlers: Ensure the click handlers update the state properly, e.g., `handleClick(i) { const newSquares = squares.slice(); newSquares[i] = xIsNext ? 'X' : 'O'; setSquares(newSquares); }`.
Ensure rendering logic is correct: Check if...read more
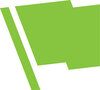
Asked in Unthinkable Solutions

Q. Write code for searching in a 2D matrix with a time complexity of O(mlogn).
Implement a binary search in each row of a sorted 2D matrix to achieve O(mlogn) time complexity.
Divide and conquer approach can be used to search in each row of the matrix.
Perform binary search on each row to find the target element efficiently.
Ensure the rows are sorted to apply binary search in each row.
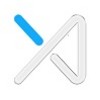
Asked in Arcesium

Q. Given a string, find the nearest index of a given character for each index in the string.
Find the nearest index of a character in a string for each index.
Create an array of strings to store the results.
Loop through each character in the string.
For each character, loop through the rest of the string to find the nearest index of the same character.
Store the result in the array.
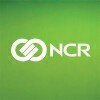
Asked in NCR Corporation

OOP concepts are fundamental principles in object-oriented programming that help in organizing and designing code.
Encapsulation: Bundling data and methods that operate on the data into a single unit (class).
Inheritance: Allowing a class to inherit properties and behavior from another class.
Polymorphism: The ability for objects of different classes to respond to the same message in different ways.
Abstraction: Hiding the complex implementation details and showing only the neces...read more
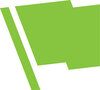
Asked in Unthinkable Solutions

Q. Design a category schema where a category can have multiple subcategories.
Design a category schema with multiple subcategories
Use a parent-child relationship to link categories and subcategories
Implement a tree data structure to represent the hierarchy
Each category can have multiple subcategories
Example: Category - Electronics, Subcategories - Computers, Mobile Phones, TVs
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
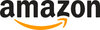

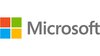
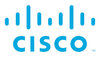
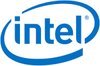
Top Interview Questions for Software Engineer Intern Related Skills
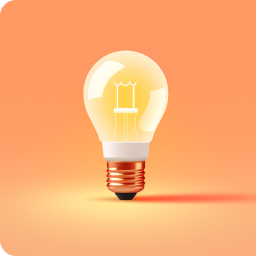
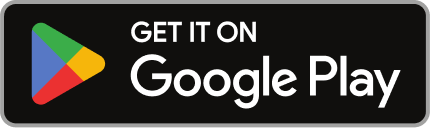
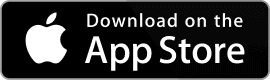
Reviews
Interviews
Salaries
Users
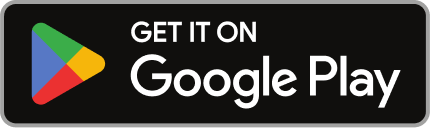
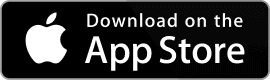