Senior Software Engineer 2
200+ Senior Software Engineer 2 Interview Questions and Answers
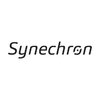
Asked in Synechron

Q. What microservices patterns are you aware ? let's assume that there is a microservice based architecture and service A is calling service B which in turn service C. If service b fails, how will you manage trans...
read moreUse compensating transactions and distributed tracing for managing transaction and logging in case of service B failure.
Implement compensating transactions to rollback changes made by service B in case of failure.
Use distributed tracing to track the flow of requests and identify where the failure occurred.
Implement retry mechanisms to handle transient failures in service B.
Use circuit breakers to prevent cascading failures in the system.
Implement centralized logging to captur...read more
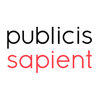
Asked in Publicis Sapient

Q. Given a scenario, draw a system diagram and explain the reasoning behind your design choices.
Design a system diagram for a scalable e-commerce platform with user management and payment processing.
Use microservices architecture for scalability and maintainability.
Implement a user management service for authentication and authorization.
Integrate a payment gateway service for secure transactions (e.g., Stripe, PayPal).
Utilize a database service for storing user data and transaction history.
Incorporate a caching layer (e.g., Redis) to improve performance.
Deploy services ...read more
Senior Software Engineer 2 Interview Questions and Answers for Freshers
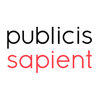
Asked in Publicis Sapient

Q. Draw a system diagram for an e-commerce system that allows a customer to place an order and retrieve their order history.
System diagram for e-commerce order placement and history retrieval
Frontend: Customer interface for browsing products, adding to cart, and placing orders
Backend: Order processing system to handle order placement, payment processing, and order fulfillment
Database: Store customer information, order details, and order history for retrieval
APIs: Communication between frontend, backend, and database for seamless order processing
Analytics: Track order history and customer behavior ...read more
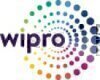
Asked in Wipro

Q. What are the key features of Java 8 that facilitate coding with streams?
Java 8 features like lambda expressions and functional interfaces make coding with streams easier and more efficient.
Lambda expressions allow for concise and readable code when working with streams.
Functional interfaces like Predicate, Function, and Consumer can be used with streams to perform operations on elements.
Stream API provides methods like map, filter, and reduce for processing collections of data in a declarative way.
Parallel streams enable concurrent processing of ...read more
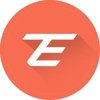
Asked in ZeMoSo Technologies

Q. Explain the internal workings of Node.js and how it processes asynchronous requests.
Node.js is a runtime environment that executes JavaScript code outside of a web browser, using an event-driven, non-blocking I/O model.
Node.js uses the V8 JavaScript engine to execute code.
It uses an event-driven architecture to handle asynchronous operations.
Node.js uses a single-threaded event loop to process multiple requests concurrently.
Callbacks are used to handle asynchronous operations in Node.js.
Node.js provides modules like 'fs' for file system operations and 'http'...read more
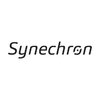
Asked in Synechron

Q. What is the internal implementation of hashmap? Let's assume that you want to store duplicate keys in the hashmap, how can we achieve the same in hashmap ?
HashMap internally uses an array of linked lists to store key-value pairs. To store duplicate keys, we can use a custom implementation of HashMap.
HashMap internally uses an array of linked lists to handle collisions.
To store duplicate keys, we can create a custom HashMap implementation that allows multiple values for the same key.
One approach is to use a HashMap with values as lists, where each key can have multiple values associated with it.
Senior Software Engineer 2 Jobs
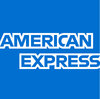
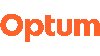
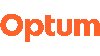
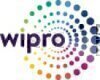
Asked in Wipro

Q. What are the different isolation levels in transactions and how do propagation types work?
Isolation levels in transactions determine the degree to which transactions are isolated from each other. Propagation types define how transactions are propagated between different components.
Isolation levels include READ UNCOMMITTED, READ COMMITTED, REPEATABLE READ, and SERIALIZABLE.
Propagation types include REQUIRED, REQUIRES_NEW, SUPPORTS, MANDATORY, and NEVER.
Isolation levels control the visibility of changes made by other transactions before the current transaction is co...read more
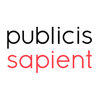
Asked in Publicis Sapient

Q. What is the need for the @Service annotation?
The @Service annotation is used in Spring framework to indicate that a class is a service component.
Used to mark a class as a service component in Spring framework
Helps in auto-detection and auto-configuration of Spring beans
Facilitates dependency injection and inversion of control
Share interview questions and help millions of jobseekers 🌟
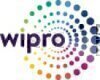
Asked in Wipro

Q. What is the purpose of Optional in Java 8, and which methods are commonly used with it?
Optional in Java 8 is used to represent a value that may or may not be present, reducing the chances of NullPointerException.
Optional is used to avoid NullPointerException by explicitly stating whether a value is present or not.
Commonly used methods with Optional include isPresent(), get(), orElse(), orElseGet(), and map().
Example: Optional<String> optionalString = Optional.ofNullable("Hello");
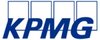
Asked in KPMG India

Q. 1. Program to get a response as Excel column name for integer input. Input 1-> A, 2-> B, 3->C, ...26->Z,27->AA, 28->AB, 29->AC..52->AZ. 2. Find first no-repeating character in String. 3. Given a list of Name ,...
read moreProgram to convert integer to Excel column name, find first non-repeating character in a string, and create a map of last names with count of names.
For converting integer to Excel column name, you can use a recursive function to map the remainder of the division by 26 to the corresponding letter.
To find the first non-repeating character in a string, you can iterate through the string and use a HashMap to store the count of each character.
For creating a map of last names with ...read more
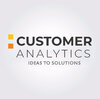
Asked in Customer Analytics

Q. What is your level of expertise as a full stack developer with MSBI, and what advanced options do you consider when responding to questions?
As a full stack developer with MSBI, I leverage data integration and analytics to build robust applications across the stack.
Data Integration: I utilize SQL Server Integration Services (SSIS) for ETL processes, ensuring seamless data flow between systems.
Data Analysis: Using SQL Server Analysis Services (SSAS), I create multidimensional models for complex data analysis, enhancing decision-making.
Reporting: I implement SQL Server Reporting Services (SSRS) to generate interacti...read more
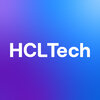
Asked in HCLTech

Q. Write pseudo code to arrange a random list of numbers in ascending order.
Pseudo code to sort a random list of numbers in ascending order
Loop through the list and compare adjacent elements
If the left element is greater than the right element, swap them
Repeat until no swaps are needed

Asked in BOLT India

Q. Given an array of numbers that represents the stock price for each day, on which days should you buy and sell to achieve the maximum profit?
Identify optimal buy and sell days from stock prices to maximize profit using a strategic approach.
Buy on the lowest price day and sell on the highest price day after that. For example, if prices are [7, 1, 5, 3, 6, 4], buy at 1 and sell at 6.
Track the minimum price seen so far as you iterate through the array. Update the maximum profit whenever you find a higher price after the minimum.
Return the days (indices) for buying and selling. In the previous example, buy on day 1 (p...read more
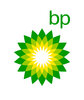
Asked in bp

Q. Implement LRU cache with expiration, priority, and recentness-based eviction policy.
Implement LRU cache with expiration, priority, and recentness based eviction policy.
Use a doubly linked list to keep track of the order of elements based on recentness.
Use a hashmap to store key-value pairs for quick access.
Implement a priority queue to handle eviction based on priority.
Set expiration time for each element and remove expired elements during access or eviction.
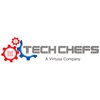
Asked in TechChefs Software

Q. Multithreading in Python is not true multithreading due to the GIL. Explain why.
Python's GIL limits true parallelism in multi-threading, causing threads to execute sequentially rather than concurrently.
The Global Interpreter Lock (GIL) allows only one thread to execute Python bytecode at a time.
This means that CPU-bound threads cannot run in parallel, leading to performance bottlenecks.
I/O-bound threads can benefit from multi-threading, as they can release the GIL while waiting for I/O operations.
Example: In a web scraping application, multiple threads c...read more
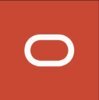
Asked in Oracle Financial Services Software

Q. Design a parking lot as part of the system design round.
Design a parking lot system with features like ticketing, payment, and space availability tracking.
Create a class for ParkingLot with attributes like total number of spaces, available spaces, and ticketing system.
Implement methods for issuing tickets, calculating parking fees, and updating space availability.
Consider implementing a payment system for customers to pay for parking.
Include features like tracking available spaces, handling multiple levels or sections in the parki...read more
Asked in BuyStars

Q. Given an array of integers temperatures represents the daily temperatures, return an array answer such that answer[i] is the number of days you have to wait after the ith day to get a warmer temperature. If the...
read moreFind the number of days until a warmer temperature for each day in a list of daily temperatures.
Use a stack to keep track of indices of temperatures.
Iterate through the temperature array from left to right.
For each temperature, pop from the stack until the current temperature is warmer.
The difference in indices gives the number of days until a warmer temperature.
Example: For temperatures [73, 74, 75, 71, 69, 72, 76, 73], the output is [1, 1, 4, 2, 1, 1, 0, 0].
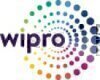
Asked in Wipro

Q. What is multiple inheritance, and how is it achieved?
Multiple inheritance is a feature in object-oriented programming where a class can inherit attributes and methods from more than one parent class.
Allows a class to inherit from multiple parent classes, combining their attributes and methods
Can lead to the diamond problem where ambiguity arises if two parent classes have a method with the same name
Achieved in languages like C++ using virtual inheritance or interfaces in languages like Java
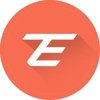
Asked in ZeMoSo Technologies

Q. Discuss HTTP Response Status Codes and how they can be customized based on business requirements.
Response status codes indicate the outcome of an HTTP request, customizable to meet specific business needs.
200 OK: Standard response for successful requests.
201 Created: Used when a new resource is created, e.g., after a POST request.
400 Bad Request: Indicates that the server cannot process the request due to client error.
401 Unauthorized: Indicates that authentication is required and has failed or not been provided.
404 Not Found: Used when the requested resource could not b...read more
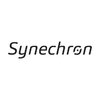
Asked in Synechron

Q. What is the Singleton pattern ? in how many ways can this pattern be broken ?
Singleton pattern ensures a class has only one instance and provides a global point of access to it.
Singleton pattern can be implemented by making the constructor private and providing a static method to access the instance.
The pattern can be broken by using reflection to access the private constructor and create multiple instances.
Another way to break the Singleton pattern is by using multiple class loaders in Java.
Thread synchronization issues can also lead to breaking the ...read more
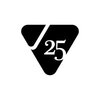
Asked in ValueLabs

Q. Can you describe a migration project that you have completed in the past?
Led a successful migration of a legacy system to a cloud-based architecture, enhancing performance and scalability.
Conducted a thorough assessment of the existing legacy system to identify dependencies and potential challenges.
Developed a detailed migration plan, including timelines, resource allocation, and risk management strategies.
Utilized AWS services to migrate databases, ensuring data integrity and minimal downtime during the transition.
Implemented automated testing to...read more
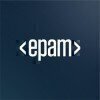
Asked in EPAM Systems

Q. Create a shell script to create 100 users on 5 different servers.
Create a Shell script to add 100 users on 5 different servers.
Use a loop to create 100 users on each server.
Utilize the 'useradd' command to add users.
Ensure unique usernames for each user on each server.
Consider using SSH for remote server access.
Test the script on a single server before running on all servers.
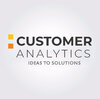
Asked in Customer Analytics

Q. What are your skills in advanced database queries and performance optimization?
I possess strong skills in advanced database queries and performance optimization, ensuring efficient data retrieval and management.
Proficient in writing complex SQL queries, including JOINs, subqueries, and CTEs for data aggregation.
Experience with indexing strategies to improve query performance, such as creating composite indexes for multi-column searches.
Utilized query optimization techniques like EXPLAIN plans to analyze and refine slow-running queries.
Implemented cachin...read more
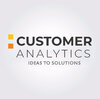
Asked in Customer Analytics

Q. What technologies did you use, and what complexities did you resolve?
I utilized various technologies to solve complex problems in software development, enhancing performance and user experience.
Microservices Architecture: Implemented a microservices architecture using Spring Boot, which improved scalability and allowed independent deployment of services.
Database Optimization: Resolved performance issues by optimizing SQL queries and implementing indexing strategies in PostgreSQL, reducing query time by 40%.
API Development: Developed RESTful AP...read more
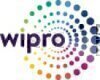
Asked in Wipro

Q. How does inter-service communication work in microservices?
Inter-service communication in microservices involves using protocols like HTTP, gRPC, or messaging queues.
Microservices communicate with each other through APIs using protocols like HTTP or gRPC.
Message queues like RabbitMQ or Kafka can be used for asynchronous communication between services.
Service discovery tools like Consul or Eureka help services locate each other dynamically.
API gateways can be used to manage and secure communication between services.
Event-driven archit...read more
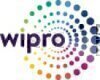
Asked in Wipro

Q. What are the different design patterns used in microservice architecture?
Design patterns in microservice architecture help in solving common design problems and improving scalability, maintainability, and flexibility.
Service Registry pattern - used for service discovery and registration
Circuit Breaker pattern - used for fault tolerance and resilience
API Gateway pattern - used for routing and load balancing
Saga pattern - used for managing distributed transactions
Event Sourcing pattern - used for capturing all changes to an application state as a se...read more
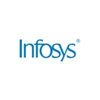
Asked in Infosys

Q. What are the different types of dependency injection?
Types of dependency injection include constructor injection, setter injection, and interface injection.
Constructor injection: Dependencies are provided through a class's constructor.
Setter injection: Dependencies are provided through setter methods.
Interface injection: Dependencies are provided through an interface method.
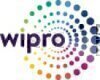
Asked in Wipro

Q. What is the difference between RestTemplate and Feign Client?
RestTemplate is a synchronous client for making HTTP requests, while Feign Client is a declarative HTTP client that simplifies making API calls.
RestTemplate is part of the Spring framework and requires manual configuration for each request.
Feign Client is a declarative client that uses annotations to define API endpoints and parameters.
RestTemplate is synchronous, blocking the calling thread until the response is received.
Feign Client is asynchronous by default, allowing for ...read more
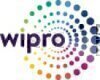
Asked in Wipro

Q. What is transaction management in Spring Boot applications?
Transaction management in Spring Boot ensures data integrity by managing database transactions.
Spring Boot uses @Transactional annotation to manage transactions
It ensures that all operations within a transaction are completed successfully or rolled back if an error occurs
Transactions can be managed programmatically using TransactionTemplate
Supports different transaction isolation levels like READ_COMMITTED, REPEATABLE_READ, etc.
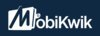
Asked in Mobikwik

Q. Design a system that can handle 1 million transactions per second.
Design a system to handle 10 lacs transactions per second.
Use distributed systems and load balancing to handle the high volume of transactions.
Implement caching mechanisms to reduce database load.
Use high-performance hardware and optimize code for speed.
Consider using a NoSQL database for faster read/write operations.
Implement fault-tolerant mechanisms to ensure system reliability.
Use asynchronous processing to handle requests quickly.
Consider using a message queue to handle ...read more
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
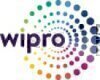
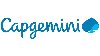
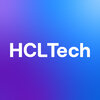
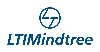
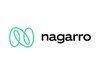
Top Interview Questions for Senior Software Engineer 2 Related Skills
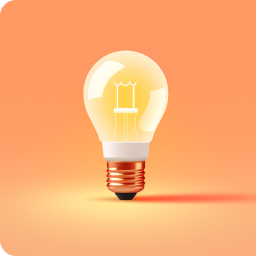
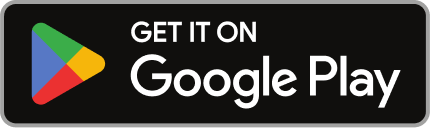
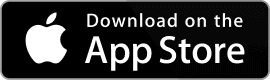
Reviews
Interviews
Salaries
Users
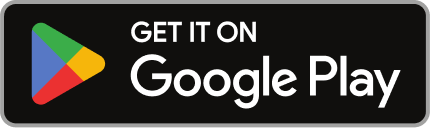
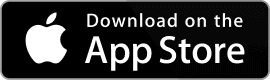