Senior Software Engineer
4500+ Senior Software Engineer Interview Questions and Answers
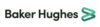
Asked in Baker Hughes

Q. How do you communicate between components in Angular?
Components in Angular can communicate with each other using @Input, @Output, and services.
Use @Input to pass data from parent to child component
Use @Output to emit events from child to parent component
Use services to share data between components that are not directly related
Use RxJS subjects to create a shared data stream between components
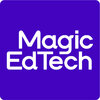
Asked in Magic Edtech

Q. How do you find the second largest number in a column in MySQL?
To find the second largest number in a column in MySQL, you can use the ORDER BY and LIMIT clauses.
Write a SELECT statement to retrieve the column values in descending order using ORDER BY.
Use the LIMIT clause to limit the result set to the second row.
The value in the second row will be the second largest number in the column.
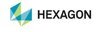
Asked in Hexagon Capability Center

Q. Q Reverse String Coding question Q find unique array value
Reverse a given string and find unique values in an array of strings.
Use the reverse() method to reverse the given string.
Use a Set to store unique values in the array.
Loop through the array and add each element to the Set.
Convert the Set back to an array to get the unique values.
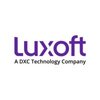
Asked in Luxoft

Q. What algorithm does the Java 8 Garbage Collector follow?
Java 8 Garbage collector follows the algorithm called G1 (Garbage-First).
G1 divides the heap into regions and collects garbage in small portions called 'garbage-first' regions.
It uses multiple parallel threads for garbage collection.
G1 aims to minimize pause times by dynamically adjusting the amount of garbage collected in each region.
It uses a combination of young and old generation collection to achieve better performance.
G1 also supports concurrent garbage collection, allo...read more
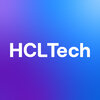
Asked in HCLTech

Q. What is the internal working of a HashMap?
HashMap is a data structure that stores key-value pairs and uses hashing to quickly retrieve values based on keys.
HashMap internally uses an array of linked lists to store key-value pairs.
When a key-value pair is added, the key is hashed to determine the index in the array where it will be stored.
If multiple keys hash to the same index, a linked list is used to handle collisions.
To retrieve a value, the key is hashed again to find the corresponding index and then the linked l...read more
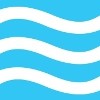
Asked in Headstream Technologies

Q. Can we use SQL Functions on the JOIN Clause?
Yes, SQL Functions can be used on the JOIN Clause.
SQL Functions can be used on the JOIN Clause to manipulate the data before joining.
Examples of SQL Functions that can be used on the JOIN Clause are CONCAT, SUBSTRING, and DATE_FORMAT.
Using SQL Functions on the JOIN Clause can improve query performance and reduce the amount of data transferred.
Senior Software Engineer Jobs
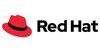
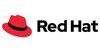
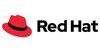
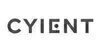
Asked in Cyient

Q. I have experienced in front-end developer 1.what are the challenges you faced in your project. 2.rela time example of subject and behaviour subject 3.how you call the multiple API using promises 4. disadvantage...
read moreAnswering questions related to front-end development challenges and techniques.
Challenges faced in front-end development projects include browser compatibility issues, performance optimization, and responsive design implementation.
Real-time examples of Subject and BehaviorSubject in Angular can be demonstrated by creating a chat application where Subject is used for broadcasting messages and BehaviorSubject is used for storing the current chat room.
Calling multiple APIs using...read more
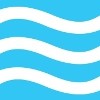
Asked in Headstream Technologies

Q. What are the SOLID principles, and can you explain them?
SOLID is a set of principles for object-oriented programming to make software more maintainable, scalable, and robust.
S - Single Responsibility Principle: A class should have only one reason to change.
O - Open-Closed Principle: A class should be open for extension but closed for modification.
L - Liskov Substitution Principle: Subtypes should be substitutable for their base types.
I - Interface Segregation Principle: A client should not be forced to depend on methods it does no...read more
Share interview questions and help millions of jobseekers 🌟
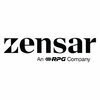
Asked in Zensar Technologies

Q. What are the things to keep in mind when you raise a Bug/defect?
Things to keep in mind when raising a bug/defect
Provide a clear and concise description of the bug
Include steps to reproduce the bug
Provide screenshots or videos if possible
Assign the bug to the appropriate team member
Set the priority and severity of the bug
Track the bug until it is resolved
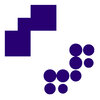
Asked in Tietoevry

Q. What is Function app? What is App service? What is key vault? Difference between key and secret? Basic questions related to Vm an networking in VM?
Function app is a serverless compute service that enables you to run code on-demand without having to manage infrastructure.
Function app is used to run code on-demand without managing infrastructure
It is a serverless compute service
It supports multiple languages and integrates with other Azure services
Examples of Function app include Azure Functions and Logic Apps
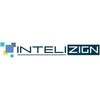
Asked in Intelizign Lifecycle Services

Q. Which database is used in Mendix?
Mendix uses a multi-model database called Mendix Data Hub.
Mendix Data Hub is a unified data layer that integrates with various databases.
It supports both SQL and NoSQL databases.
Examples of databases that can be used with Mendix include MySQL, PostgreSQL, MongoDB, and Oracle.
Mendix Data Hub provides a visual interface for managing data models and relationships.
It allows developers to easily connect and interact with data from different sources.
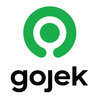
Asked in Go-Jek

Q. Write a Go program to find the kth minimum element from a given list of numbers.
Code in Go to find kth minimum element from a given list of numbers.
Sort the list of numbers in ascending order
Return the kth element from the sorted list
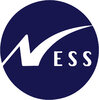
Asked in Ness Digital Engineering

Q. Write a program to find the number of repeating characters in a given string using collections.
Program to find the number of repeating characters in a given string using collections.
Use a HashMap to store the characters as keys and their count as values.
Iterate through the string and update the count in the HashMap for each character.
Finally, iterate through the HashMap and count the characters with a count greater than 1.
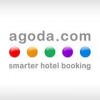
Asked in Agoda

Q. Given a list of products with multiple discounts applicable, how do you find the minimum cost required to buy all the products? Each product can have zero or more discounts, and there may be multiple discounts...
read moreCalculate the minimum cost to buy products with multiple applicable discounts using a hashmap and mathematical strategies.
Identify each product and its base price.
Collect all applicable discounts for each product using a hashmap.
Calculate the effective price for each product by applying the best discount.
Example: Product A costs $100 with discounts of 20% and $15 off. Best discount is $15 off, making it $85.
Sum the effective prices of all products to get the total minimum cos...read more
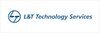
Asked in L&T Technology Services

Q. How would you pass a variable number of parameters to a function when the number and types of parameters are unknown?
Use variadic arguments in C++ to handle variable number of parameters in a function
Use variadic arguments in C++ to create a function that can accept any number of parameters
Example: void myFunction(int firstParam, ...)
Access the parameters using va_list and va_start macros
Example: va_list args; va_start(args, firstParam); int nextParam = va_arg(args, int);
End the argument list with va_end macro
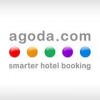
Asked in Agoda

Q. Return the minimum number of changes applied to a list of strings such that no two adjacent characters in the list are the same.
Minimize changes in a list of strings to ensure no two adjacent characters are the same using a greedy approach.
Iterate through each string in the list.
For each string, check adjacent characters.
If two adjacent characters are the same, change one of them.
Count the number of changes made.
Example: For ['aabb', 'ccdd'], change 'aabb' to 'abab' (1 change) and 'ccdd' to 'cdcd' (1 change), total changes = 2.
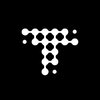
Asked in Turing

Q. Write a program to check whether a string is valid. The string consists of only three kinds of special characters: '()', '[]', and '{}'. Return true if all special characters are in the correct form (e.g., (){}...
read moreCheck if a string of special characters is valid with correct pairing and nesting.
Use a stack to track opening characters: '(', '[', '{'.
For each closing character: ')', ']', '}', pop from the stack and check for a match.
If the stack is empty at the end, the string is valid.
Examples: Valid - '(){}[]', '{()[]}', '{{[()]}}'; Invalid - '{[](}}', '{], ()[}'
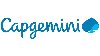
Asked in Capgemini

Q. Can you provide a program that prints the second largest number in both an array and a list, illustrating implementations using streams as well as traditional methods?
Program to find second largest number in an array and list using streams and traditional methods.
Use streams to find second largest number in array: Arrays.stream(array).distinct().sorted().skip(array.length - 2).findFirst().orElse(null)
Use traditional method to find second largest number in list: Sort the list in descending order and get the element at index 1
Ensure to handle edge cases like empty array/list or arrays/lists with less than 2 elements
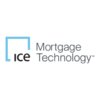
Asked in Ice Mortgage Technology

Q. Explain how you would troubleshoot a network bottleneck in your web API application hosted in a public cloud like Azure or AWS.
To troubleshoot a network bottleneck in a web API application hosted in a public cloud like Azure or AWS, one can analyze network traffic, monitor server performance, and optimize resource allocation.
Use network monitoring tools to analyze traffic patterns and identify potential bottlenecks.
Monitor server performance metrics such as CPU usage, memory usage, and disk I/O to pinpoint any resource constraints.
Optimize resource allocation by scaling up or out based on the identif...read more
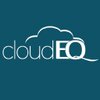
Asked in cloudEQ

Q. What are Action Filters? Why are they used?
Action Filters are attributes that can be applied to controller actions to perform pre/post processing.
Action Filters are used to modify the behavior of controller actions.
They can be used to perform authentication, logging, caching, etc.
Action Filters can be applied globally or to specific actions.
Examples include [Authorize] for authentication and [OutputCache] for caching.
Action Filters can also be created by the developer to perform custom processing.
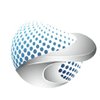
Asked in Apisero

Q. What are oAuth tokens? Can you explain there types ?
oAuth tokens are used for authentication and authorization purposes in web applications.
oAuth tokens are used to grant access to resources without sharing the user's credentials.
There are three types of oAuth tokens: access tokens, refresh tokens, and authorization codes.
Access tokens are short-lived tokens that are used to access protected resources.
Refresh tokens are long-lived tokens that are used to obtain new access tokens.
Authorization codes are used to obtain access to...read more
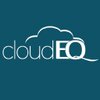
Asked in cloudEQ

Q. What is Interfaces? and Why these are used?
Interfaces are a way to define a contract between two objects, specifying the methods and properties that one object must implement.
Interfaces allow for loose coupling between objects, making it easier to change the implementation of one object without affecting others.
They promote code reusability by allowing multiple objects to implement the same interface.
Interfaces can be used to create mock objects for testing purposes.
Examples of interfaces in Java include Comparable, R...read more
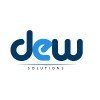
Asked in Dew Solutions

Q. Can you explain the fundamental concepts of JavaScript and React?
A brief overview of JavaScript and React fundamentals for a Senior Software Engineer role.
JavaScript is a versatile, high-level programming language used for web development.
React is a JavaScript library for building user interfaces, particularly single-page applications.
JS features include variables (let, const), functions (arrow functions), and asynchronous programming (promises, async/await).
React components can be class-based or functional, with hooks like useState and us...read more
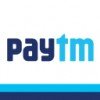
Asked in Paytm

Q. For infinite scrolling, how do you handle large datasets to ensure efficient and smooth loading while scrolling up and down?
To handle large size data for infinite scroll, use virtual scrolling, lazy loading, and optimize data fetching/rendering.
Implement virtual scrolling to render only the visible items on the screen, reducing memory usage and improving performance.
Use lazy loading to fetch more data as the user scrolls, avoiding loading all data at once.
Optimize data fetching and rendering by using efficient algorithms and data structures, and minimizing unnecessary operations.
Consider implement...read more
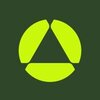
Asked in Advantmed

Q. Keep SQL procedures short, use multiple small procedure with specific functionality being used, avoid use of temp tables and Joins, use select statements wisely. do not use sub queries, do not use dynamic sql
To optimize SQL procedures, keep them short, use small procedures with specific functionality, avoid temp tables and joins, use select statements wisely, avoid subqueries and dynamic SQL.
Break down complex procedures into smaller ones with specific functionality
Avoid using temporary tables and joins whenever possible
Use select statements wisely to retrieve only the necessary data
Avoid subqueries and find alternative approaches
Do not use dynamic SQL to prevent potential securi...read more
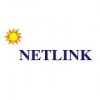
Asked in Netlink

Q. What is CDN in Computers/Information technology industry
CDN stands for Content Delivery Network. It is a distributed network of servers that deliver web content to users based on their geographic location.
CDN improves website performance by reducing latency and increasing page load speed.
CDN caches content in multiple locations, allowing users to access it from a server closest to them.
CDN helps handle high traffic loads and prevents server overload.
Popular CDN providers include Cloudflare, Akamai, and Amazon CloudFront.
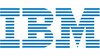
Asked in IBM

Q. Write a program to find the equilibrium index in an array, where the sum of the elements before the index is equal to the sum of the elements after the index.
Find the equilibrium index in an array where the sum of elements before equals the sum after the index.
Definition: An equilibrium index is an index in an array where the sum of elements on the left equals the sum on the right.
Example: In the array [1, 3, 5, 2, 2], the equilibrium index is 2 because 1+3 = 2+2.
Approach: Calculate the total sum of the array, then iterate through the array while maintaining a running sum of the left side.
Efficiency: This can be done in O(n) time ...read more
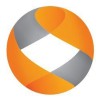
Asked in Maven Wave Partners

Q. What is the difference between ViewBag, ViewData, and TempData in MVC?
Difference between view bag, view data and Temp data in mvc
ViewBag is a dynamic object used to pass data from controller to view
ViewData is a dictionary object used to pass data from controller to view
TempData is used to pass data between controller actions or redirects
ViewBag and ViewData are short-lived while TempData is longer-lived

Asked in INSORCE

Q. Explain encapsulation What is overriding and over loading What is interface When to use interface When to use seald class What is virtual overide key word Which arte ctecture use in your application Do you know...
read moreExplaining encapsulation, overriding, overloading, interface, sealed class, virtual override keyword, and MVC architecture.
Encapsulation is the process of hiding implementation details and exposing only necessary information.
Overriding is providing a new implementation for a method in a subclass that is already defined in the parent class.
Overloading is defining multiple methods with the same name but different parameters.
Interface is a contract that defines a set of methods ...read more
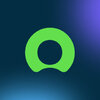
Asked in ServiceNow

Q. Given arrival and departure times of all trains that reach a railway station, find the minimum number of platforms required for the railway station so that no train waits.
Minimum 2 platforms required for a railway station.
At least 2 platforms are required for trains to arrive and depart simultaneously.
Additional platforms may be required based on the frequency of trains and passenger traffic.
Platforms should be long enough to accommodate the longest trains that will use the station.
Platforms should also have appropriate facilities for passengers, such as seating, shelter, and signage.
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
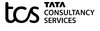
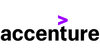
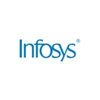
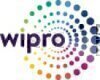
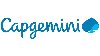
Top Interview Questions for Senior Software Engineer Related Skills
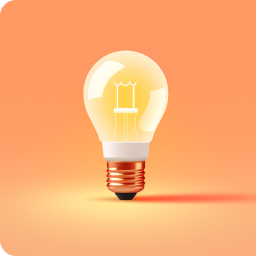
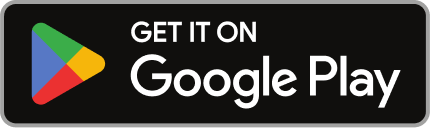
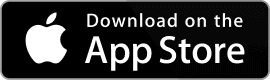
Reviews
Interviews
Salaries
Users
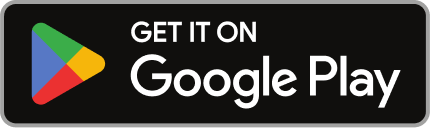
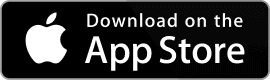