Senior Software Engineer
4500+ Senior Software Engineer Interview Questions and Answers
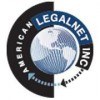
Asked in American Legalnet

Q. If a stored procedure has three SELECT statements and one statement is taking a long time to load, how would you investigate the cause?
Identify performance issues in a stored procedure with multiple select statements by analyzing execution plans and query performance.
Check the execution plan for the slow query using tools like SQL Server Management Studio.
Use SQL Server Profiler or Extended Events to monitor query performance and identify bottlenecks.
Analyze indexes on the tables involved in the slow query; missing or fragmented indexes can slow down performance.
Consider running the slow query in isolation t...read more
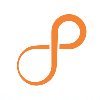
Asked in Persistent Systems

Q. what is callback hell, what is Promises?
Callback hell is a situation where nested callbacks make code unreadable. Promises are a solution to this problem.
Callback hell occurs when there are too many nested callbacks in asynchronous code
It makes the code difficult to read and maintain
Promises are a way to handle asynchronous operations without nested callbacks
Promises can be used to chain multiple asynchronous operations together
Promises have a resolve and reject function to handle success and failure respectively
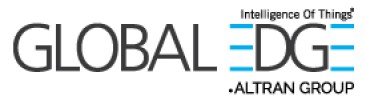
Asked in Global Edge Software

Q. What is pointer? Explain dangling pointer, null pointer, void pointer.
A pointer is a variable that stores the memory address of another variable. Dangling, null, and void pointers are types of pointers.
Dangling pointer: a pointer that points to a memory location that has been deallocated or freed
Null pointer: a pointer that does not point to any memory location
Void pointer: a pointer that has no specific data type and can point to any data type
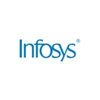
Asked in Infosys

Q. What is the difference between arraylist and linked list What are different types of annotations used in spring boot What is lambda expression?
ArrayList and LinkedList are both data structures used to store and manipulate collections of objects in Java.
ArrayList is implemented as a resizable array, while LinkedList is implemented as a doubly linked list.
ArrayList provides constant time access to elements using their index, while LinkedList provides constant time insertion and deletion of elements.
Spring Boot annotations include @Controller, @Service, @Repository, @Autowired, @RequestMapping, and more.
Lambda expressi...read more
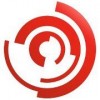
Asked in Wabtec

Q. Write a program to find the number of occurrences of each character in a given string.
Program to find the number of occurrences in a given string
Use a loop to iterate through the string and count the occurrences of each character
Store the count in a dictionary or hash table
Return the dictionary or hash table
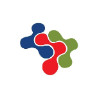
Asked in Vitrana

Q. What are the key concepts of Java 8, specifically regarding functional interfaces, streams, lambda expressions, and optional interfaces?
Java 8 introduced functional programming features like lambda expressions, streams, and optional interfaces for better code efficiency.
Functional Interfaces: Interfaces with a single abstract method, e.g., Runnable, Callable.
Lambda Expressions: Concise way to represent functional interfaces, e.g., (x, y) -> x + y.
Streams: A sequence of elements supporting sequential and parallel aggregate operations, e.g., list.stream().filter(x -> x > 10).
Optional Interface: A container for ...read more
Senior Software Engineer Jobs
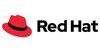
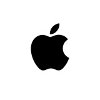

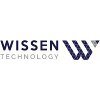
Asked in Wissen Technology

Q. Can we use a user-defined class as a key in a hashmap? If yes, explain how.
Yes, user-defined classes can be used as keys in a hashmap by overriding equals() and hashCode() methods.
Override equals() method to define equality based on class attributes.
Override hashCode() method to return a consistent hash code for the object.
Example: For a class 'Person', use 'name' and 'id' for equality and hash code.
Ensure that if two objects are equal, they must have the same hash code.
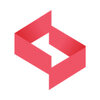
Asked in Simform

Q. what is sql server agent why can't we create objects of abstract class write extension method for abstract class SQL: SP vs Function, which one is faster, why? can I call SP from Function Microservice orchestra...
read moreSQL Server Agent is a job scheduling tool in SQL Server. Abstract classes cannot be instantiated. SPs are faster than functions. Microservice concepts. IEnumerable vs IQueryable.
SQL Server Agent is a job scheduling tool in SQL Server for automating tasks like backups, database maintenance, etc.
Abstract classes cannot be instantiated because they are incomplete and meant to be extended by subclasses.
Extension methods can be created for abstract classes to add new functionality...read more
Share interview questions and help millions of jobseekers 🌟
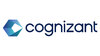
Asked in Cognizant

Q. What's is uses of cache memory in application and which cache you used in your application and why ??
Cache memory is used to store frequently accessed data for faster retrieval, improving application performance.
Cache memory reduces the time taken to access data by storing frequently used data closer to the processor.
It helps in improving the overall performance of the application by reducing latency.
Different types of cache memory include L1, L2, and L3 caches, each with varying sizes and speeds.
In my application, we used L1 cache for storing critical data that needed to be...read more
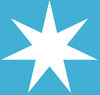
Asked in Maersk

Q. A user wants to upload multiple files at once, but the provided API only accepts one file at a time. How would you handle this scenario gracefully?
Implement a user-friendly solution for uploading multiple files to a single-file API.
1. Use a client-side script to handle multiple file selections and uploads sequentially.
2. Provide visual feedback to the user, such as a progress bar for each file upload.
3. Implement error handling to notify users of any failed uploads and allow retries.
4. Consider batching uploads by grouping files and sending them in a single request if the API supports it.
5. Use a temporary storage solut...read more
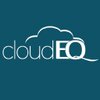
Asked in cloudEQ

Q. What is Self Join in SQL Server? Give an example.
Self Join is a way to join a table with itself using aliases.
It is useful when we need to compare records within the same table.
It requires the use of aliases to differentiate between the two instances of the same table.
Example: SELECT a.name, b.name FROM employees a, employees b WHERE a.manager_id = b.employee_id;
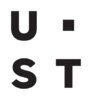
Asked in UST

N+1 SELECT problem in Hibernate occurs when a query results in N+1 database queries being executed instead of just one.
Occurs when a query fetches a collection of entities and then for each entity, another query is executed to fetch related entities individually
Can be resolved by using fetch joins or batch fetching to fetch all related entities in a single query
Example: Fetching a list of orders and then for each order, fetching the customer information separately
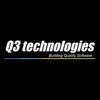
Asked in Q3 Technologies

Q. What are the core components of the .Net Framework?
The core components of .Net Framework include Common Language Runtime (CLR), Framework Class Library (FCL), and ASP.NET.
Common Language Runtime (CLR) provides the runtime environment for executing .NET applications.
Framework Class Library (FCL) is a collection of reusable classes, interfaces, and value types that provide access to system functionality.
ASP.NET is a web application framework for building dynamic web pages and web services using .NET technologies.
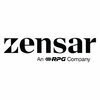
Asked in Zensar Technologies

Selenium supports various navigation commands to interact with web pages.
Some navigation commands supported by Selenium include get(), navigate().to(), navigate().back(), navigate().forward(), and navigate().refresh().
The get() command is used to open a webpage by providing the URL.
The navigate().to() command is used to navigate to a specific URL.
The navigate().back() command is used to navigate back to the previous page in the browser history.
The navigate().forward() command...read more
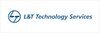
Asked in L&T Technology Services

A daemon in the context of Linux is a background process that runs continuously to perform specific tasks.
Daemons do not have a controlling terminal.
They typically start at system boot and run until the system is shut down.
Examples include the Apache web server daemon (httpd) and the SSH daemon (sshd).
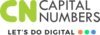
Asked in Capital Numbers Infotech

Q. What is soap. And what us differences between rest and soap
SOAP is a protocol for exchanging structured information in the implementation of web services. REST is an architectural style for building web services.
SOAP stands for Simple Object Access Protocol
SOAP uses XML for message exchange
SOAP requires more bandwidth and processing power than REST
REST uses HTTP for communication
REST is more flexible and scalable than SOAP
RESTful APIs are easier to implement and maintain than SOAP-based APIs
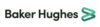
Asked in Baker Hughes

Abstract class is a class that cannot be instantiated and can have both abstract and non-abstract methods. Interface is a blueprint for a class and can only have abstract methods.
Abstract class can have constructors while interface cannot.
A class can implement multiple interfaces but can only extend one abstract class.
Abstract class can have instance variables while interface cannot.
Abstract class can provide default implementations for some methods while interface cannot.
Int...read more
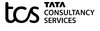
Asked in TCS

C is a procedural programming language while C++ is a multi-paradigm programming language with support for object-oriented programming.
C is a procedural programming language, focusing on functions and procedures.
C++ is a multi-paradigm programming language, supporting procedural, object-oriented, and generic programming.
C does not support classes and objects, while C++ does.
C++ has features like inheritance, polymorphism, and encapsulation which are not present in C.
C++ has a...read more
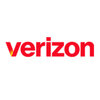
Asked in Verizon

Q. What is the event loop, and what types of tasks are in the queue?
Event loop is a mechanism that allows JavaScript to perform non-blocking I/O operations.
Event loop is responsible for handling asynchronous callbacks in JavaScript.
Tasks in the queue can be either microtasks or macrotasks.
Microtasks are executed before macrotasks.
Examples of macrotasks include setTimeout, setInterval, and I/O operations.
Examples of microtasks include Promise callbacks and mutation observer callbacks.
Asked in Alaan

Q. Design a caching service that can cache the results of a given method (e.g., sumOfArray). The method should only be executed once for a specific input, with subsequent calls for the same input returning the cac...
read moreImplement a caching service to store results of a method for specific inputs, avoiding redundant calculations.
Use a dictionary to store cached results, e.g., cache = {}.
Define a decorator function to wrap the target method, e.g., @cache_decorator.
Check if the input exists in the cache before executing the method.
If cached, return the cached result; otherwise, compute and store it.
Example: sumOfArray([1, 2, 3]) -> 6, cache it for future calls.
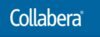
Asked in Collabera Technologies

Q. Have you ever implemented or worked with server-driven UIs?
Yes, I have experience implementing server driven UIs.
Implemented server driven UIs using JSON responses to dynamically update UI elements
Worked with frameworks like React and Angular to handle server driven UI updates
Used server driven UIs to efficiently manage and display large amounts of data
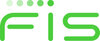
Asked in FIS

Q. How would you debug an application to solve a production defect that was raised?
I would start by reproducing the issue, analyzing logs, checking code changes, and using debugging tools.
Reproduce the issue to understand the exact scenario where the defect occurs.
Analyze logs to identify any error messages or warnings related to the defect.
Check recent code changes to see if any recent modifications could have caused the defect.
Use debugging tools like breakpoints, logging, and profiling to trace the issue in the code.
Collaborate with team members to brain...read more
Asked in TMotions Global

Q. Setup, Differences and Current project Code first Db vs Database First Db? and How to make changes from code side?
Code first and Database first are two approaches to create a database. Changes can be made from code side using migrations.
Code first approach involves creating the database schema using code and then generating the database from it.
Database first approach involves creating the database schema using a visual designer and then generating the code from it.
Migrations can be used to make changes to the database schema from code side.
Code first approach is more flexible and allows...read more
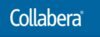
Asked in Collabera Technologies

Q. What is Core Data, and can you describe its operations with examples or scenarios?
Core Data is a framework provided by Apple for managing the model layer objects in an iOS application.
Core Data is used for storing, retrieving, and managing data in an iOS app.
It provides an object-oriented interface to work with data.
Operations include creating, reading, updating, and deleting data.
Example: Creating a new record in Core Data for a user profile.
Example: Fetching a list of items from Core Data to display in a table view.
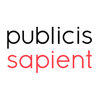
Asked in Publicis Sapient

Q. Create a login form and validate email input using Vanilla JS.
Create a Login form with email validation using Vanilla JS.
Create a form in HTML with input fields for email and password
Use JavaScript to validate the email input using regular expressions
Display error messages if the email input is not in the correct format
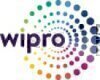
Asked in Wipro

Q. How to improve accuracy for an ICR project? Lifecycle of Abbyy Flexicapture project. The architecture of Abbyy Flexicapture distributed version. How to export via the database?
Improving accuracy for an ICR project involves training the system, optimizing image quality, and using advanced algorithms.
Train the system with a diverse set of data to improve recognition accuracy.
Optimize image quality by using high-resolution images and proper lighting.
Use advanced algorithms such as machine learning and natural language processing.
Regularly review and update the system to ensure accuracy over time.
Abbyy Flexicapture project lifecycle involves planning, ...read more
Asked in Ker InfoTech

Q. What are the different types of authentication, and how would you implement them?
Types of authentication include password-based, multi-factor, biometric, and token-based authentication.
Password-based authentication involves users entering a password to access a system.
Multi-factor authentication requires users to provide multiple forms of verification, such as a password and a code sent to their phone.
Biometric authentication uses unique physical characteristics like fingerprints or facial recognition to verify a user's identity.
Token-based authentication...read more
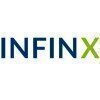
Asked in Infinx

Q. What is a join, and how many types of joins are available? Please provide examples.
Join is used to combine rows from two or more tables based on a related column between them.
Types of joins: Inner Join, Left Join, Right Join, Full Join
Inner Join: Returns rows when there is at least one match in both tables
Left Join: Returns all rows from the left table and the matched rows from the right table
Right Join: Returns all rows from the right table and the matched rows from the left table
Full Join: Returns rows when there is a match in one of the tables
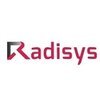
Asked in RadiSys

Q. How do you determine the number of processes running on a server?
Use command line tools like 'ps' or 'top' to find the number of running processes on a server.
Use 'ps aux' command to list all running processes and count the number of lines
Use 'top' command and look for the 'Tasks' section which shows the number of running processes
Use 'htop' command which provides a more interactive and detailed view of running processes
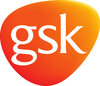
Asked in GlaxoSmithKline Pharmaceuticals

Q. How would you implement secure cloud deployments?
Secure cloud deployments can be implemented through various measures such as encryption, access control, and regular security audits.
Implement encryption for data at rest and in transit
Use access control mechanisms to restrict unauthorized access
Regularly conduct security audits to identify and address vulnerabilities
Implement multi-factor authentication for added security
Ensure compliance with industry standards and regulations
Use secure coding practices to prevent vulnerabi...read more
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
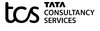
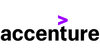
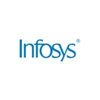
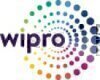
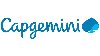
Top Interview Questions for Senior Software Engineer Related Skills
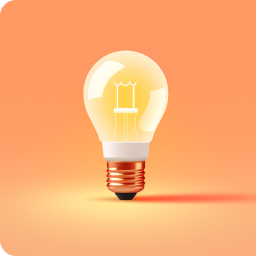
Calculate your in-hand salary
Confused about how your in-hand salary is calculated? Enter your annual salary (CTC) and get your in-hand salary
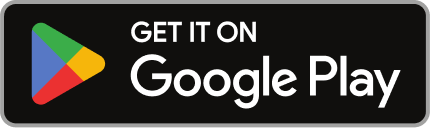
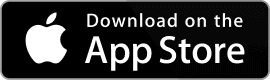
Reviews
Interviews
Salaries
Users
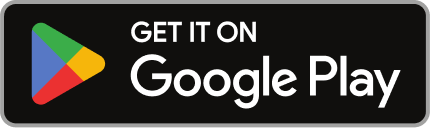
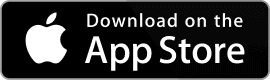