Senior Software Engineer
4500+ Senior Software Engineer Interview Questions and Answers
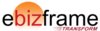
Asked in Eastern Software Solutions Pvt. Ltd

Q. Types of Trigger in Oracle forms? how to sequence of Trigger fire in oracle Forms.
Types of Trigger in Oracle forms and their sequence of firing.
Types of triggers include Key Triggers, Mouse Triggers, and Timer Triggers.
Key Triggers fire when a key is pressed or released.
Mouse Triggers fire when the mouse is clicked or moved.
Timer Triggers fire at a specified interval.
The sequence of trigger firing is Pre-Form, Pre-Block, Pre-Record, Post-Record, Post-Block, and Post-Form.

Asked in Fareportal

The default access modifier in C# is 'private'.
Default access modifier is applied if no access modifier is specified for a class member
It restricts the access to the member within the same class
Example: private int myVariable = 10;
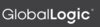
Asked in GlobalLogic

INNER JOIN returns rows when there is at least one match in both tables, while OUTER JOIN returns all rows from both tables.
INNER JOIN only returns rows that have matching values in both tables
OUTER JOIN returns all rows from both tables, even if there are no matches
Types of OUTER JOIN include LEFT JOIN, RIGHT JOIN, and FULL JOIN
Example: INNER JOIN would return only the customers who have made a purchase, while OUTER JOIN would return all customers with or without a purchase
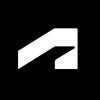
Asked in Autodesk

Q. Write a program that takes a string and a number of occurrences as input. Find a character with the same occurrence count in a single iteration.
Program to find a character with same occurrences count in a string in a single iteration.
Iterate through the string and keep track of character occurrences in a hashmap.
Check for characters with the same occurrence count in the hashmap.
Return the first character found with the same occurrence count.
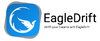
Asked in EagleDrift Technologies

Q. What are indexes in SQL and why are they important?
Indexes in SQL are data structures that improve the speed of data retrieval operations by allowing quick access to specific rows in a table.
Indexes are used to quickly locate rows in a table without having to search the entire table.
They are important because they can significantly improve the performance of SELECT queries by reducing the time taken to retrieve data.
Indexes can be created on one or more columns in a table to speed up search operations.
Examples of indexes incl...read more
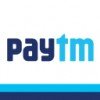
Asked in Paytm

Q. What are the best and worst solutions for the coding problem "Longest Substring Without Repeating Characters," including a detailed explanation of each solution along with their time and space complexity?
The Longest Substring Without Repeating Characters problem involves finding the length of the longest substring without any repeating characters.
Best solution: Sliding Window approach with HashSet to track unique characters. Time complexity O(n), space complexity O(min(n, m)) where n is the length of the string and m is the size of the character set.
Worst solution: Brute force approach checking all substrings for uniqueness. Time complexity O(n^3), space complexity O(min(n, m...read more
Senior Software Engineer Jobs
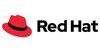
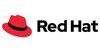
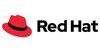
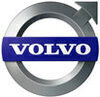
Asked in Volvo

Q. Find all even number from list with some limit.(like if in list there are 5 even number we have to fetch only 4 even number). > What is the Architecture currently we r following in Microservice > what is API Ga...
read moreTo find all even numbers from a list with a specified limit, iterate through the list and add even numbers until the limit is reached.
Iterate through the list of numbers
Check if each number is even
Add the even numbers to a new list until the limit is reached
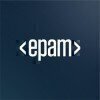
Asked in EPAM Systems

Q. Given a matrix, when you encounter a 0, make all the elements in the corresponding row and column to 0.
Given a matrix, replace rows and columns with 0 when encountering a 0.
Iterate through the matrix and store the row and column indices of 0s in separate sets.
Iterate through the sets and update the corresponding rows and columns to 0.
Share interview questions and help millions of jobseekers 🌟
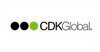
Asked in CDK Global

Q. What is the program using Stream API to find odd numbers, square those odd numbers, and then calculate their sum?
Using Stream API to find odd numbers, square them, and calculate their sum.
Use Stream API filter to find odd numbers
Use map to square the odd numbers
Use reduce to calculate the sum
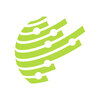
Asked in kipi.ai

Q. What are views, why are they used, and what are the different types of views?
Views in databases are virtual tables that display data from one or more tables based on a query.
Views are used to simplify complex queries by storing them as a virtual table.
They can hide the complexity of underlying tables and provide a layer of security by restricting access to certain columns.
Types of views include simple views, complex views, materialized views, and indexed views.
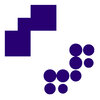
Asked in Tietoevry

Q. 1. What is design pattern and it's types and solid patterns.
Design patterns are reusable solutions to common software design problems. They include creational, structural, and behavioral patterns.
Design patterns are reusable solutions to common software design problems.
They provide proven solutions to recurring design problems.
Design patterns can be categorized into creational, structural, and behavioral patterns.
Creational patterns deal with object creation mechanisms.
Structural patterns focus on class and object composition.
Behavior...read more
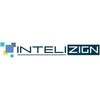
Asked in Intelizign Lifecycle Services

Q. What are the different activities available in Microflow?
Microflows are used in Mendix to define the logic and flow of an application. They consist of various activities.
Create object activity - creates a new object in the database
Change object activity - modifies the attributes of an existing object
Commit activity - saves changes made to objects in the database
Retrieve activity - retrieves objects from the database based on specified criteria
Loop activity - iterates over a list of objects or a range of numbers
Exclusive split activ...read more
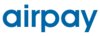
Asked in Airpay Payment Services

Q. what is an event loop, The second highest from the table
An event loop is a programming construct that waits for and dispatches events or messages in a program.
Event loop is commonly used in asynchronous programming to handle tasks like I/O operations without blocking the main thread.
It continuously checks for events in a queue and processes them one by one.
Examples of event loops include Node.js event loop for handling asynchronous operations in JavaScript.
Event loop helps in managing the flow of events and tasks in a program effi...read more

Asked in Nokia Networks

Q. What is the difference between a global and a static variable?
Global variables can be accessed from any part of the program while static variables are limited to the scope they are declared in.
Global variables are declared outside of any function while static variables are declared inside a function with the static keyword.
Global variables can be modified by any part of the program while static variables retain their value even after the function they are declared in has finished executing.
Global variables can lead to naming conflicts a...read more
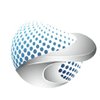
Asked in Apisero

Q. What is the difference between DROP, TRUNCATE, and DELETE commands?
Drop command removes a table from the database, truncate command removes all rows from a table, and delete command removes specific rows from a table.
Drop command removes the table structure and all associated data
Truncate command removes all rows from a table but keeps the table structure
Delete command removes specific rows from a table based on a condition
Drop and truncate are faster than delete as they do not generate logs
Delete can be rolled back, while drop and truncate ...read more
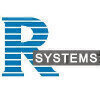
Asked in R Systems International

Q. What is dependency injection, and can you provide a use case where it would be beneficial?
Dependency injection is a design pattern where components are provided with their dependencies rather than creating them internally.
Dependency injection helps in achieving loose coupling between components.
It makes components easier to test by allowing for easier mocking of dependencies.
Use cases include injecting database connections, logging services, and external API clients into components.
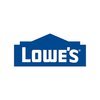
Asked in Lowe's

Q. Sprint groom, Maximum story points in sprint, what is the role of scrum master, refresh token, Synthetic events, webpack, reduction, redux saga vs thunk, what pattern thunk follows, hooks
Answering questions related to sprint grooming, Scrum Master role, refresh tokens, Synthetic events, webpack, redux saga vs thunk, and hooks for Senior Software Engineer position.
Sprint grooming involves prioritizing and estimating user stories for the upcoming sprint.
Scrum Master facilitates the Scrum process and ensures the team follows the Agile principles.
Refresh tokens are used to obtain new access tokens after the old ones expire.
Synthetic events are artificially create...read more
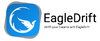
Asked in EagleDrift Technologies

Q. Are you aware of Spark? Do you have any experience in Spark?
Yes, I am aware of Spark and have experience working with it.
I have used Spark for processing large datasets in distributed computing environments.
I am familiar with Spark's core concepts such as Resilient Distributed Datasets (RDDs) and transformations/actions.
I have experience writing Spark applications using languages like Scala or Python.
I have worked with Spark SQL for querying structured data and Spark Streaming for real-time data processing.
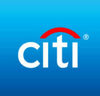
Asked in Citicorp

Q. Can I write a try-catch block inside a catch block?
Yes, it is possible to write a try catch block inside a catch block.
This is called nested try-catch block.
It is useful when we want to handle different types of exceptions in different ways.
Example: try { //code } catch (ExceptionType1 e1) { try { //code } catch (ExceptionType2 e2) { //code } } }
It is important to avoid excessive nesting as it can make the code difficult to read and maintain.
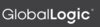
Asked in GlobalLogic

The lifecycle of a Docker container involves creation, running, pausing, restarting, and stopping.
1. Creation: A Docker container is created from a Docker image using the 'docker run' command.
2. Running: The container is started and runs the specified application or service.
3. Pausing: The container can be paused using the 'docker pause' command, which temporarily halts its processes.
4. Restarting: The container can be restarted using the 'docker restart' command, which stops...read more
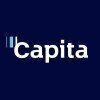
Asked in Capita

ArrayList is non-synchronized and faster, while Vector is synchronized and slower.
ArrayList is not synchronized, while Vector is synchronized.
ArrayList is faster than Vector due to lack of synchronization.
Vector is thread-safe, while ArrayList is not.
Example: ArrayList<String> list = new ArrayList<>(); Vector<String> vector = new Vector<>();
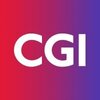
Asked in CGI Group

Hadoop is an open-source framework for distributed storage and processing of large data sets.
Core components include Hadoop Distributed File System (HDFS), Yet Another Resource Negotiator (YARN), and MapReduce.
HDFS is responsible for storing data across multiple machines in a Hadoop cluster.
YARN manages resources and schedules tasks across the cluster.
MapReduce is a programming model for processing and generating large data sets.
Other components like Hadoop Common, Hadoop Map...read more
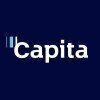
Asked in Capita

Spring Actuator is a set of production-ready features to help monitor and manage your application.
Provides insights into application's health, metrics, and other useful information
Enables monitoring and managing of application through HTTP endpoints
Can be used to check application's health, view metrics, and even perform custom actions
Helps in identifying and troubleshooting issues in production environment
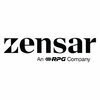
Asked in Zensar Technologies

ETL process involves extracting data from various sources, transforming it to fit the data warehouse schema, and loading it into the warehouse.
Extract: Data is extracted from different sources such as databases, files, APIs, etc.
Transform: Data is cleaned, filtered, aggregated, and transformed to match the data warehouse schema.
Load: Transformed data is loaded into the data warehouse for analysis and reporting.
Example: Extracting customer information from a CRM system, transf...read more
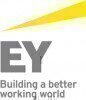
Asked in Ernst & Young

The @RestController annotation in Spring Boot is used to define a class as a RESTful controller.
Used to create RESTful web services in Spring Boot
Combines @Controller and @ResponseBody annotations
Eliminates the need to annotate each method with @ResponseBody

Asked in Bosch Global Software Technologies

STLC is a process followed to ensure high quality software by testing at every stage of development.
STLC consists of phases like requirement analysis, test planning, test design, test execution, and test closure.
Each phase has specific activities and deliverables to ensure the software meets quality standards.
Examples of testing types in STLC include unit testing, integration testing, system testing, and acceptance testing.
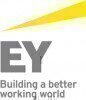
Asked in Ernst & Young

Microservice architecture is an architectural style that structures an application as a collection of loosely coupled services.
Microservices are small, independent services that work together to form a complete application.
Each microservice is responsible for a specific business function and can be developed, deployed, and scaled independently.
Communication between microservices is typically done through APIs.
Microservices allow for better scalability, flexibility, and resili...read more
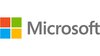
Asked in Microsoft Corporation

Q. Design elevators - How would you design how elevators work. How do you add different roles etc.
Designing elevators and adding different roles.
Design a system that can handle multiple elevators and floors
Implement a scheduling algorithm to optimize elevator usage
Add roles such as maintenance, emergency, and security
Incorporate safety features such as emergency stop buttons and sensors
Consider accessibility for individuals with disabilities
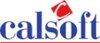
Asked in Calsoft

Q. How is memory managed in Python?
Python uses automatic memory management through garbage collection.
Python uses reference counting to keep track of memory usage.
When an object's reference count drops to zero, it is deleted.
Python also uses a garbage collector to handle circular references.
Memory allocation is handled by the Python memory manager.
Python provides tools like the 'gc' module for managing memory usage.

Asked in Bosch Global Software Technologies

Best practices in test automation include proper planning, selecting the right tools, creating reusable test scripts, maintaining test data, and continuous integration.
Proper planning is essential to identify test cases, prioritize them, and determine the scope of automation.
Selecting the right tools based on the project requirements and team expertise can improve efficiency and effectiveness.
Creating reusable test scripts helps save time and effort in maintaining and updatin...read more
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
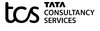
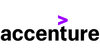
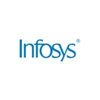
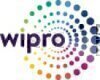
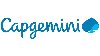
Top Interview Questions for Senior Software Engineer Related Skills
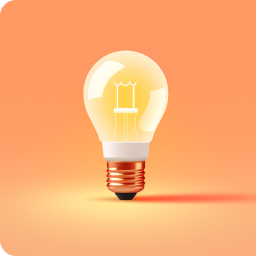
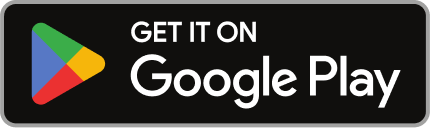
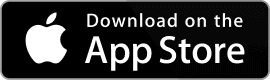
Reviews
Interviews
Salaries
Users
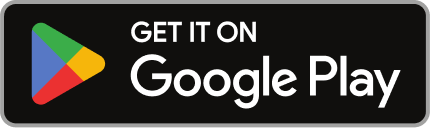
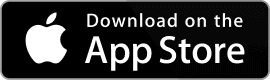