Senior Java Software Engineer
Senior Java Software Engineer Interview Questions and Answers
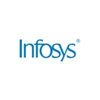
Asked in Infosys

Q. What is ResultSet in Java, and why should it be avoided?
ResultSet is an interface in Java used to retrieve data from a database. It should be avoided due to its performance issues.
ResultSet is used to retrieve data from a database in Java
It has performance issues and should be avoided if possible
Alternative options like JPA or Hibernate can be used instead
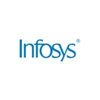
Asked in Infosys

Q. What are collections and why are they used in Java?
Collections are a group of classes and interfaces used to store and manipulate groups of objects in Java.
Collections provide a way to store and manipulate groups of objects in Java
They offer various data structures like List, Set, Map, etc.
Collections provide methods to add, remove, and search for elements in the data structures
They also offer algorithms for sorting and searching elements
Examples include ArrayList, HashSet, TreeMap, etc.
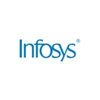
Asked in Infosys

Q. What is java and how it is platform independent
Java is a high-level programming language used to develop applications that can run on any platform.
Java code is compiled into bytecode that can run on any platform with a Java Virtual Machine (JVM)
JVM acts as an interpreter and translates bytecode into machine code
Java's platform independence is achieved through the 'Write Once, Run Anywhere' (WORA) principle
Java's standard library provides platform-independent APIs for common tasks
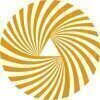
Asked in Altimetrik

Q. What annotations do you use in your current Spring Boot project?
Some annotations used in Spring Boot projects are @RestController, @Autowired, @RequestMapping, @Service, @Component, @Repository.
@RestController - Used to define RESTful web services.
@Autowired - Used for automatic dependency injection.
@RequestMapping - Used to map web requests to specific handler methods.
@Service - Used to indicate that a class is a service.
@Component - Used to indicate that a class is a Spring component.
@Repository - Used to indicate that a class is a repo...read more
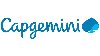
Asked in Capgemini

Q. What are the new features in Java 8?
Java 8 introduced several new features including lambda expressions, streams, and default methods.
Lambda expressions for functional programming
Streams for efficient processing of large data sets
Default methods for backward compatibility
Date and Time API for improved handling of date and time
Nashorn JavaScript engine for improved performance
Type annotations for improved type checking
Parallel array sorting for improved performance
Optional class for handling null values
Repeatabl...read more
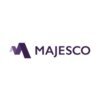
Asked in Majesco

Q. What is a static variable?
Static variable is a variable that belongs to the class and not to the instance of the class.
Static variables are declared using the 'static' keyword.
They are initialized only once, at the start of the program.
They can be accessed using the class name, without creating an instance of the class.
Changes made to a static variable are reflected across all instances of the class.
Example: 'public static int count = 0;'
Senior Java Software Engineer Jobs
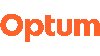
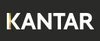
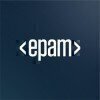
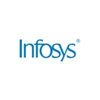
Asked in Infosys

Q. What is serialization in Java?
Serialization is the process of converting an object into a stream of bytes to store or transmit it.
Serialization is used to save and restore object states
Java provides Serializable interface to implement serialization
Serialization can be used for inter-process communication and distributed systems
Deserialization is the process of converting a stream of bytes back into an object
Serialization can be customized using readObject() and writeObject() methods
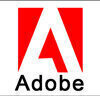
Asked in Adobe

Q. Design a parking lot system.
Design a parking lot system for efficient management of parking spaces.
Use object-oriented design principles to create classes for parking lot, parking space, vehicle, etc.
Implement algorithms for efficient parking space allocation and retrieval.
Incorporate features like ticketing system, payment processing, and monitoring.
Consider scalability and performance optimization for handling a large number of vehicles.
Include a user-friendly interface for drivers to easily navigate ...read more
Share interview questions and help millions of jobseekers 🌟
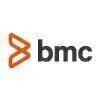
Asked in BMC Software

Q. Given a string, reverse the order of its characters and return the reversed string.
Reverse a given string
Create a char array from the input string
Use two pointers to swap characters from start and end of the array
Repeat until the pointers meet in the middle
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
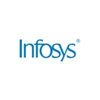
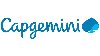
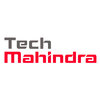
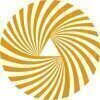
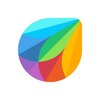
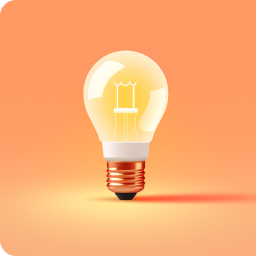
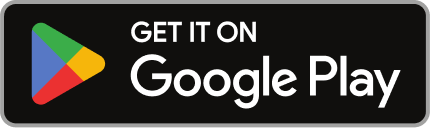
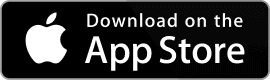
Reviews
Interviews
Salaries
Users
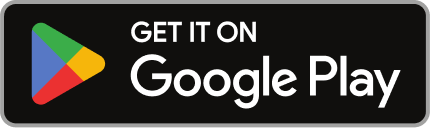
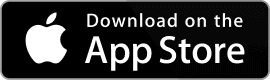