Principal Software Engineer
100+ Principal Software Engineer Interview Questions and Answers
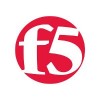
Asked in F5 Networks

Q. Codng question:For the given stream of integers, calculate the avg,print top 10 elements and bottom 10 elements. It was not clearly mentioned on the problem. After poking more on the problem only provided the d...
read moreCalculate average, top 10 and bottom 10 elements of a given stream of integers.
Create a variable to store the sum of integers and another variable to store the count of integers.
Use a loop to read the integers from the stream and update the sum and count variables.
Calculate the average by dividing the sum by the count.
Sort the integers in ascending order and print the first 10 elements for bottom 10.
Sort the integers in descending order and print the first 10 elements for top...read more
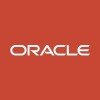
Asked in Oracle

Q. Can static variables be accessed from a non-static method? Explain with an example.
Static variables can be accessed from non-static methods using an object reference or by making the variable non-static.
Static variables can be accessed from non-static methods by creating an object of the class containing the static variable.
Alternatively, the static variable can be made non-static to be accessed directly from a non-static method.
Attempting to access a static variable directly from a non-static method will result in a compilation error.
Principal Software Engineer Interview Questions and Answers for Freshers
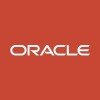
Asked in Oracle

Q. Implement a linked list with add, display, insert at end, and delete operations.
Implementation of Linked List with add, display, insert at end and delete operations.
Create a Node class with data and next pointer
Create a LinkedList class with head pointer
Add method to add a new node at the end of the list
Display method to print all nodes in the list
Insert at end method to insert a new node at the end of the list
Delete method to delete a node from the list
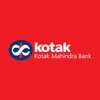
Asked in Kotak Mahindra Bank

Q. Can you describe your experience with previous projects that involved scalable design for data-intensive applications?
I have extensive experience designing scalable data-intensive applications, focusing on performance, reliability, and maintainability.
Microservices Architecture: In a recent project, I designed a microservices architecture for a financial application, allowing independent scaling of services based on load.
Database Sharding: Implemented sharding in a large-scale e-commerce platform to distribute data across multiple databases, improving query performance and reducing latency.
C...read more
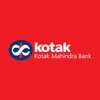
Asked in Kotak Mahindra Bank

Q. What is the process of migrating from on-premises systems to the cloud, and what challenges do you believe are imperative to consider during this process?
Migrating to the cloud involves planning, execution, and addressing challenges like security, compliance, and data integrity.
Assessment and Planning: Evaluate existing on-premises systems to determine which applications and data are suitable for migration. For example, legacy systems may require refactoring.
Choosing the Right Cloud Model: Decide between IaaS, PaaS, or SaaS based on business needs. For instance, using SaaS for CRM can reduce maintenance overhead.
Data Migration...read more
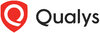
Asked in Qualys

Q. Why looking for change ? What kind of work is there in qualys?
I am looking for change to explore new challenges and opportunities. Qualys offers a variety of work in cybersecurity and cloud security.
Seeking new challenges and opportunities to grow professionally
Interested in cybersecurity and cloud security
Excited about the diverse range of projects at Qualys
Principal Software Engineer Jobs
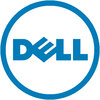
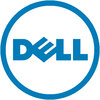
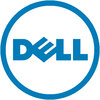
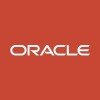
Asked in Oracle

Q. String a = "Something"; String b = new String("Something"); How many objects are created?
Two objects created - one in the string pool and one in the heap.
String 'a' is created in the string pool, while String 'b' is created in the heap.
String pool is a special area in the heap memory where strings are stored to increase reusability and save memory.
Using 'new' keyword always creates a new object in the heap, even if the content is the same as an existing string in the pool.
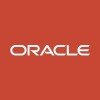
Asked in Oracle

Q. String a = "Something" a.concat(" New") What will be garbage collected?
Only the original string 'Something' will be garbage collected.
The concat method in Java does not modify the original string, but instead returns a new string with the concatenated value.
In this case, 'a' remains as 'Something' and a new string 'Something New' is created, but not assigned to any variable.
Since the new string is not stored in any variable, it will not be garbage collected.
Share interview questions and help millions of jobseekers 🌟
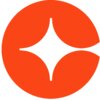
Asked in Cornerstone OnDemand

Q. How can caching be used practically? What are the advantages and drawbacks of using caching?
Caching improves performance by storing frequently accessed data, reducing latency and load on resources.
1. Performance Improvement: Caching reduces data retrieval time. For example, a web application can cache database query results.
2. Reduced Load: By serving cached data, the load on backend systems decreases, allowing them to handle more requests.
3. Cost Efficiency: Caching can lower operational costs by reducing the need for expensive database queries or API calls.
4. Scal...read more
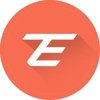
Asked in ZeMoSo Technologies

Q. What are the features of the Date and Time API in Java 8?
Java 8 Date and Time API provides improved date and time handling capabilities.
Introduction of new classes like LocalDate, LocalTime, LocalDateTime, ZonedDateTime, OffsetTime, OffsetDateTime, and Instant for better date and time manipulation
Support for time zones and offsets
Ability to perform date and time calculations easily
Enhanced formatting and parsing capabilities
Integration with existing date and time classes like java.util.Date and java.util.Calendar
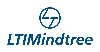
Asked in LTIMindtree

Q. What are some strategies to improve the performance of an Angular application?
Some strategies to improve Angular application performance
Use lazy loading for modules to reduce initial load time
Optimize change detection by using OnPush strategy
Minimize the use of two-way data binding
Implement server-side rendering for faster initial page load
Use AOT (Ahead-of-Time) compilation to reduce bundle size
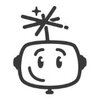
Asked in Wagepoint India

Q. When to Use Array and Hashset and Hashmap
Arrays are used for ordered collections, HashSet for unique elements, and HashMap for key-value pairs.
Use arrays when you need to store elements in a specific order and access them by index.
Use HashSet when you need to store unique elements and do not care about the order.
Use HashMap when you need to store key-value pairs and quickly retrieve values based on keys.
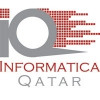
Asked in Informatica

Q. Given an array of numbers, find the length of the longest subsequence that is first increasing and then decreasing.
Use dynamic programming to find the longest increasing decreasing subsequence in an array.
Use dynamic programming to keep track of the length of the longest increasing subsequence ending at each index.
Similarly, keep track of the length of the longest decreasing subsequence starting at each index.
Combine the two to find the longest increasing decreasing subsequence.
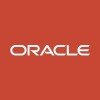
Asked in Oracle

Q. Write code to merge and remove duplicates from two sorted arrays using Collections.
Merge and remove duplicates from two sorted arrays without using Collections
Use two pointers to iterate through both arrays simultaneously
Compare elements at each pointer and add the smaller one to the result array
Skip duplicates by checking if the current element is equal to the previous element
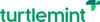
Asked in Turtlemint

Q. How do you handle locking in a distributed environment?
Locking in distributed systems ensures data consistency and prevents conflicts during concurrent operations.
Use distributed locks with tools like ZooKeeper or etcd to manage access to shared resources.
Implement optimistic locking by using versioning to detect conflicts before committing changes.
Consider using a consensus algorithm like Paxos or Raft to ensure agreement on the state of resources.
Leverage database features like row-level locking or transactions to maintain cons...read more
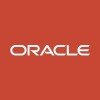
Asked in Oracle

Q. Implement two stacks using a single array without using extra space.
Implement two stacks using a single array without extra space.
Divide the array into two halves and use one half for each stack.
Keep track of the top of each stack separately.
Handle stack overflow and underflow conditions.
Example: Implementing two stacks for undo and redo operations in a text editor.
Asked in OneFin

Q. Why didn't you follow the superior design?
I chose a different design approach based on specific project requirements and constraints.
Considered project requirements and constraints when deciding on design approach
Different designs may have different trade-offs and considerations
Example: Chose a design that prioritized scalability over simplicity due to anticipated growth in user base
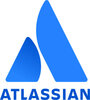
Asked in Atlassian

Q. Design a web crawler.
Design a scalable web crawler to efficiently index web pages and extract relevant data.
Define the scope: Determine which websites to crawl and what data to extract (e.g., text, images).
Use a distributed architecture: Implement multiple crawler instances to handle large-scale crawling.
Implement a queue system: Use a message queue (like RabbitMQ) to manage URLs to be crawled.
Respect robots.txt: Ensure the crawler adheres to the rules set by websites regarding crawling.
Handle du...read more
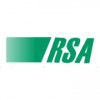
Asked in RSA

Q. How would you improve the performance of a page that takes a long time to load data?
Optimize page performance by reducing load times through various strategies like caching, lazy loading, and efficient queries.
Implement caching strategies (e.g., Redis or Memcached) to store frequently accessed data.
Use lazy loading for images and other resources to load them only when they are in the viewport.
Optimize database queries by using indexing and avoiding N+1 query problems.
Minimize HTTP requests by combining CSS and JavaScript files.
Utilize Content Delivery Networ...read more
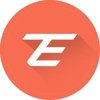
Asked in ZeMoSo Technologies

Q. Describe the high-level system design for a movie booking application.
A movie booking app system design involves user authentication, movie selection, seat reservation, payment processing, and booking confirmation.
User authentication: Implement login/signup functionality for users.
Movie selection: Display list of movies with details like showtimes, ratings, and genres.
Seat reservation: Allow users to select seats for chosen movie showtime.
Payment processing: Integrate payment gateway for secure transactions.
Booking confirmation: Send confirmati...read more
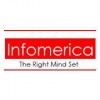
Asked in Infomerica

Q. joins and merge in sql and python
Joins and merges are used to combine data from multiple tables in SQL and Python.
Joins in SQL are used to combine rows from two or more tables based on a related column between them.
Types of joins in SQL include INNER JOIN, LEFT JOIN, RIGHT JOIN, and FULL JOIN.
Merging in Python is done using the pandas library, where two DataFrames are combined based on a common column.
Example: SQL - SELECT * FROM table1 INNER JOIN table2 ON table1.id = table2.id
Example: Python - merged_df = ...read more
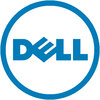
Asked in Dell

Q. How do you handle critical application incidents?
I handle critical application incidents by following a structured incident response process.
Quickly assess the severity and impact of the incident
Notify relevant stakeholders and form an incident response team
Identify the root cause of the issue and implement a temporary fix if needed
Communicate updates and progress to stakeholders regularly
Conduct a post-incident review to learn from the incident and prevent future occurrences

Asked in Calix

Q. Given a problem statement, what are the ways to come up with technical solutions to solve it?
Technical solutions are derived by analyzing the problem, researching existing solutions, brainstorming with team, prototyping, and testing.
Analyze the problem statement thoroughly to understand the requirements and constraints.
Research existing solutions and technologies to see if any can be leveraged or modified.
Brainstorm with team members to gather different perspectives and ideas.
Prototype the solution to quickly test and iterate on different approaches.
Test the solution...read more
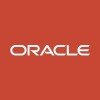
Asked in Oracle

Q. What is the interview process at Oracle?
The interview process at Oracle typically involves multiple stages, including technical assessments and behavioral interviews.
Initial screening: A recruiter reviews your resume and conducts a brief phone interview to assess your fit for the role.
Technical interview: You may face coding challenges or system design questions, such as designing a scalable application.
Behavioral interview: Expect questions about teamwork and conflict resolution, like describing a time you overcam...read more
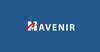
Asked in Mavenir Systems

Q. What is the use of the 'Volatile' keyword in C#?
The 'Volatile' keyword in C# is used to indicate that a field might be modified by multiple threads that are executing at the same time.
Ensures that any read or write operation on the variable is done directly from the main memory and not from the CPU cache.
Useful when working with multithreaded applications to prevent unexpected behavior due to caching.
Example: 'volatile int count = 0;'
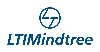
Asked in LTIMindtree

Q. How do you ensure the architectural design and development process is followed?
I ensure adherence to architectural design through structured processes, documentation, and regular reviews.
Establish clear architectural guidelines and principles that align with business goals.
Conduct regular design reviews to ensure compliance with architectural standards, such as using peer reviews in code development.
Utilize architectural documentation tools like C4 model diagrams to visualize and communicate the architecture effectively.
Implement automated testing and C...read more
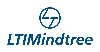
Asked in LTIMindtree

Q. How do you ensure the architecture aligns with industry best practices and the domain?
I ensure architecture aligns with industry standards through continuous learning, collaboration, and leveraging best practices.
Stay updated with industry trends by attending conferences and webinars, such as AWS re:Invent or Google I/O.
Engage in code reviews and architectural discussions with peers to gather diverse perspectives and insights.
Utilize established design patterns and frameworks, like Microservices or Event-Driven Architecture, to enhance scalability and maintain...read more
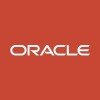
Asked in Oracle

Q. What do you know about Oracle?
Oracle is a multinational computer technology corporation that specializes in developing and marketing database software and technology.
Oracle is known for its flagship product, the Oracle Database, which is widely used in enterprise applications.
It also offers a range of other software products, including middleware, application development tools, and cloud services.
Oracle has a strong presence in the enterprise market and is a major competitor to other database vendors like...read more
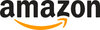
Asked in Amazon

Q. What is regression testing?
Regression testing is the process of retesting modified software to ensure that previously working functionalities still work as expected.
Regression testing is performed after making changes to software to identify any new defects or issues introduced.
It helps ensure that existing functionalities are not affected by the changes made.
Regression testing can be done manually or using automated testing tools.
It involves re-executing test cases that cover the affected areas of the...read more
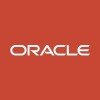
Asked in Oracle

Q. Write code to emulate the Producer/Consumer Problem.
Emulate Producer/Consumer Problem using code
Create a shared buffer between producer and consumer
Use synchronization mechanisms like mutex or semaphore to control access to the buffer
Implement producer and consumer functions to add and remove items from the buffer respectively
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
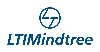
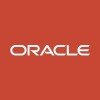
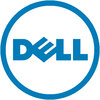
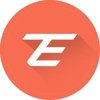
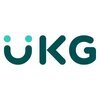
Top Interview Questions for Principal Software Engineer Related Skills
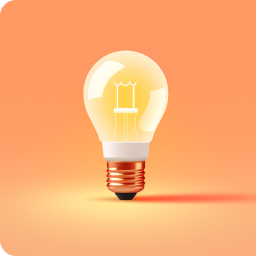
Calculate your in-hand salary
Confused about how your in-hand salary is calculated? Enter your annual salary (CTC) and get your in-hand salary
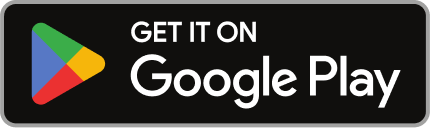
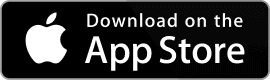
Reviews
Interviews
Salaries
Users
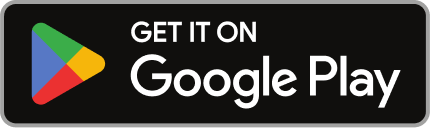
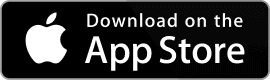