Lead Software Engineer
200+ Lead Software Engineer Interview Questions and Answers
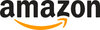
Asked in Amazon

Q. Square Root with Decimal Precision Problem Statement
You are provided with two integers, 'N' and 'D'. Your objective is to determine the square root of the number 'N' with a precision up to 'D' decimal places. ...read more
Implement a function to find square root of a number with specified decimal precision.
Implement a function that takes two integers N and D as input and returns the square root of N with precision up to D decimal places.
Ensure that the discrepancy between the computed result and the correct value is less than 10^(-D).
Handle multiple test cases efficiently within the given constraints.
Consider using mathematical algorithms like Newton's method for square root calculation.
Test t...read more
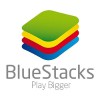
Asked in BlueStacks

Q. Vertical Order Traversal Problem Statement
You are given a binary tree, and the task is to perform a vertical order traversal of the values of the nodes in the tree.
For a node at position ('X', 'Y'), the posit...read more
Perform vertical order traversal of a binary tree based on decreasing 'Y' coordinates.
Implement a function to perform vertical order traversal of a binary tree
Nodes are added in order from top to bottom based on decreasing 'Y' coordinates
Handle cases where two nodes have the same position by adding the node that appears first on the left
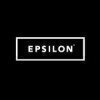
Asked in Epsilon

Q. If we have two tables with the same schema, where one table has indexes and the other does not, will there be any performance difference when inserting data?
Indexes can slow down insert performance due to the overhead of maintaining the index.
Inserting data into the table without indexes will be faster than inserting into the table with indexes.
The more indexes a table has, the slower the insert performance will be.
However, indexes can improve query performance by allowing the database to quickly find the data being searched for.
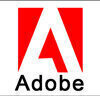
Asked in Adobe

Q. Power Calculation Problem Statement
Given a number x
and an exponent n
, compute xn
. Accept x
and n
as input from the user, and display the result.
Note:
You can assume that 00 = 1
.
Input:
Two integers separated...read more
Calculate x raised to the power of n, accepting x and n as input and displaying the result.
Accept two integers x and n as input
Compute x^n and display the result
Handle special case 0^0 = 1
Ensure x is between 0 and 8, and n is between 0 and 9
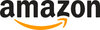
Asked in Amazon

Q. Longest Unique Substring Problem Statement
Given a string input of length 'n', your task is to determine the length of the longest substring that contains no repeating characters.
Explanation:
A substring is a ...read more
Find the length of the longest substring with unique characters in a given string.
Use a sliding window approach to keep track of the longest substring without repeating characters.
Use a hashmap to store the index of each character in the string.
Update the start index of the window when a repeating character is encountered.
Calculate the maximum length of the window as you iterate through the string.
Return the maximum length as the result.
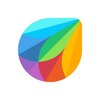
Asked in Freshworks

Q. Zig-Zag Conversion Problem Statement
You are given a string S
and an integer ROW
. Your task is to convert the string into a zig-zag pattern on a given number of rows. After the conversion, output the string row...read more
Convert a given string into a zig-zag pattern on a specified number of rows and output the result row-wise.
Iterate through the string and place characters in the zig-zag pattern based on the row number
Keep track of the direction of movement (up or down) to determine the row placement
Combine characters from each row to get the final result
Lead Software Engineer Jobs



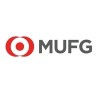
Asked in MUFG

Q. Regarding functional interfaces, what are the real-time use cases for static and default methods?
Static and default methods in functional interfaces provide utility methods and default implementations for interface methods in real-time applications.
Static methods in functional interfaces can be used for utility methods that are not tied to a specific instance of the interface.
Default methods in functional interfaces provide default implementations for interface methods, allowing for backward compatibility when new methods are added to the interface.
In real-time applicati...read more
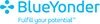
Asked in Blue Yonder

Q. What is pessimistic locking and optimistic locking?
Pessimistic locking is when a resource is locked for a long time, while optimistic locking is when a resource is locked only when it is being modified.
Pessimistic locking involves locking a resource for a long time to prevent other users from accessing it.
Optimistic locking involves locking a resource only when it is being modified, allowing other users to access it in the meantime.
Pessimistic locking is useful when conflicts are likely to occur, while optimistic locking is u...read more
Share interview questions and help millions of jobseekers 🌟
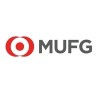
Asked in MUFG

Q. How do you apply multiple primary keys to an entity in Hibernate?
Use @IdClass annotation to apply multiple primary keys to an entity in Hibernate
Create a separate class for composite primary key
Use @IdClass annotation on the entity class to reference the composite primary key class
Define the primary key fields in both the entity class and the composite primary key class
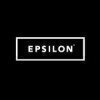
Asked in Epsilon

Q. What is angular? Which language does it use? What is typescript?
Angular is a popular front-end web application framework that uses TypeScript as its primary language.
Angular is developed and maintained by Google.
It is used for building dynamic, single-page web applications.
It uses TypeScript, a superset of JavaScript, which adds features like static typing and class-based object-oriented programming.
Angular provides a range of features like data binding, dependency injection, and component-based architecture.
Some popular websites built wi...read more
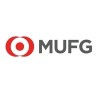
Asked in MUFG

Q. How do you ensure a bean is used before it is destroyed?
Use a bean before it is destroyed by implementing a pre-destroy method
Implement a method annotated with @PreDestroy in the bean class
This method will be called before the bean is destroyed
Perform any necessary cleanup or final operations in this method
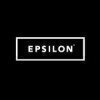
Asked in Epsilon

Q. How to handle load balancing? What is load balancing?
Load balancing is the process of distributing network traffic across multiple servers to avoid overloading a single server.
Load balancing helps to improve the performance, availability, and scalability of applications.
It can be achieved through hardware or software solutions.
Examples of load balancing algorithms include round-robin, least connections, and IP hash.
Load balancing can also be combined with other techniques such as caching and compression to further optimize perf...read more
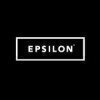
Asked in Epsilon

Q. What is a Factory design pattern? How do you implement that?
Factory design pattern is a creational pattern that provides an interface for creating objects in a superclass, but allows subclasses to alter the type of objects that will be created.
Factory pattern is used when we have a superclass with multiple subclasses and based on input, we need to return one of the subclass.
It provides a way to delegate the instantiation logic to child classes.
Example: java.util.Calendar, java.util.ResourceBundle, java.text.NumberFormat, etc.
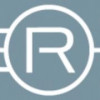
Asked in Radiometer Medical

Q. What tools and languages are you familiar with?
I am familiar with a variety of programming languages and tools.
Languages: Java, Python, C++, JavaScript, SQL
Tools: Git, JIRA, Jenkins, Eclipse, Visual Studio
Frameworks: Spring, React, Angular, Node.js
Databases: MySQL, MongoDB, Oracle
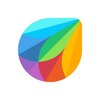
Asked in Freshworks

The architecture of our current project is a microservices-based system with a combination of RESTful APIs and message queues.
Utilizes microservices architecture for scalability and flexibility
Uses RESTful APIs for communication between services
Incorporates message queues for asynchronous processing
Each microservice is responsible for a specific domain or functionality
Data is stored in a combination of relational and NoSQL databases
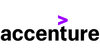
Asked in Accenture

Q. If Java and .Net were used in the same environment, what are their key differences and similarities?
Java and .NET are both powerful platforms for software development, each with unique features and ecosystems.
Java is platform-independent due to the Java Virtual Machine (JVM), while .NET is primarily Windows-based but has expanded with .NET Core.
Java uses a syntax similar to C++, while .NET languages like C# have a syntax that is more modern and user-friendly.
Java has a strong emphasis on object-oriented programming, while .NET supports multiple programming paradigms, includ...read more
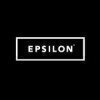
Asked in Epsilon

Q. What is routing? Types of routing
Routing is the process of selecting a path for network traffic to travel from one network to another.
Routing is done by routers in a network.
Types of routing include static routing, dynamic routing, and default routing.
Static routing involves manually configuring the routes.
Dynamic routing uses protocols to automatically update the routing table.
Default routing is used when there is no specific route for a packet.
Examples of routing protocols include OSPF, BGP, and RIP.
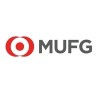
Asked in MUFG

Q. Stream API: What are intermediate and terminal operators?
Intermediate operators transform or filter the stream elements, while terminal operators produce a result or side effect.
Intermediate operators include filter(), map(), sorted(), distinct(), etc.
Terminal operators include forEach(), collect(), reduce(), count(), etc.
Intermediate operators are lazy and do not execute until a terminal operator is called.
Terminal operators trigger the stream processing and produce a result or side effect.
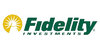
Asked in Fidelity Investments

Q. 1. Explain protractor framework 2. What is package lock file ? 3. Difference between === and == in JS 4. A javascript program to reverse a number
Answers to technical questions for Lead Software Engineer position
Protractor is an end-to-end testing framework for AngularJS applications
Package lock file is used to lock the version of dependencies installed in a project
=== checks for both value and type equality while == checks for value equality only
function reverseNumber(num) { return parseInt(num.toString().split('').reverse().join('')) }
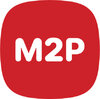
Asked in M2P Fintech

Q. What is the mean by thread, how many ways we can create thread?
A thread is a lightweight sub-process that allows concurrent execution within a process. Threads can be created in multiple ways.
Threads can be created by extending the Thread class in Java.
Threads can be created by implementing the Runnable interface in Java.
Threads can be created using thread pools in Java.
Threads can be created using asynchronous functions in JavaScript.
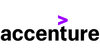
Asked in Accenture

Q. Good condition or bad condition on what does it depend.
Condition can depend on various factors such as environment, genetics, lifestyle, and medical history.
Condition can depend on environmental factors like pollution, climate, and access to healthcare.
Genetics play a role in determining one's predisposition to certain conditions.
Lifestyle choices such as diet, exercise, and stress management can impact one's overall health.
Medical history, including past illnesses and treatments, can also influence current condition.
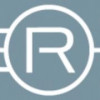
Asked in Radiometer Medical

Q. How would you model a product to derive both functional and non-functional test cases?
Modeling a product involves defining test cases for both functional and non-functional requirements to ensure quality and performance.
Identify functional requirements: e.g., user authentication, data processing.
Define non-functional requirements: e.g., performance, security, usability.
Create test cases for functional aspects: e.g., test login with valid/invalid credentials.
Develop non-functional test cases: e.g., load testing for 1000 concurrent users.
Consider edge cases: e.g...read more
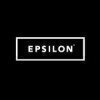
Asked in Epsilon

Q. What is the CORS issue? Where does it occur?
CORS (Cross-Origin Resource Sharing) is a security feature that restricts web pages from making requests to a different domain.
CORS issue occurs when a web page tries to access resources from a different domain
It is a security feature implemented by web browsers to prevent cross-site scripting attacks
CORS issue can be resolved by configuring the server to allow cross-origin requests or by using JSONP
It can occur in AJAX requests, web fonts, images, and videos
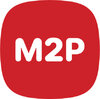
Asked in M2P Fintech

Q. What is the difference between synchronous and asynchronous calls?
Sync call blocks the execution until the response is received, while asynchronous call allows the program to continue executing without waiting for the response.
Sync call blocks the program execution until the response is received
Asynchronous call allows the program to continue executing without waiting for the response
Sync calls are easier to understand and debug, but can lead to performance issues if used excessively
Asynchronous calls are more efficient for handling multipl...read more
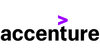
Asked in Accenture

Q. Do you prefer 5+5 or 5*5 and why?
I prefer 5*5 as it is a standard mathematical operation for multiplication.
Multiplication is a fundamental arithmetic operation.
5*5 is more commonly used in mathematical calculations.
5+5 is a simple addition operation.
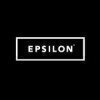
Asked in Epsilon

Q. What is caching? Types of caching
Caching is the process of storing frequently accessed data in a temporary storage area for faster access.
Caching reduces the time and resources required to access data.
Types of caching include browser caching, server caching, and database caching.
Browser caching stores web page resources like images, stylesheets, and scripts on the user's device.
Server caching stores frequently accessed data on the server to reduce database queries.
Database caching stores frequently accessed ...read more
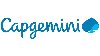
Asked in Capgemini

Q. 1) Explain AIDL, HIDL ? 2) Android Boot Up Sequence? 3) Android Architecture in detail? 4) Android Components Framework? 5) Android Activity Lifecycle?
AIDL and HIDL are communication interfaces used in Android development. Android Boot Up Sequence, Architecture, Components Framework, and Activity Lifecycle are key concepts in Android development.
AIDL (Android Interface Definition Language) is used for inter-process communication in Android. It allows different applications to communicate with each other.
HIDL (Hardware Interface Definition Language) is used for communication between Android system services and hardware compo...read more
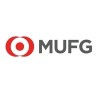
Asked in MUFG

Q. How can you use a POJO as a key in a HashMap?
Use the POJO's attributes that define equals() and hashCode() methods as the key in the Hashmap.
Ensure the POJO class overrides equals() and hashCode() methods.
Create an instance of the POJO and use it as the key in the Hashmap.
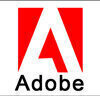
Asked in Adobe

Q. What features should be added to a pen to make it the best pen on the market?
The best pen in the market should have a comfortable grip, smooth writing experience, long-lasting ink, and a stylish design.
Comfortable grip for extended use
Smooth writing experience for effortless writing
Long-lasting ink to avoid frequent refills
Stylish design to appeal to customers
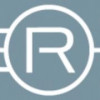
Asked in Radiometer Medical

Q. Give an overview of STLC and detail the process followed for development.
STLC outlines the phases of software testing, ensuring quality and efficiency in the development lifecycle.
1. Requirement Analysis: Understand testing requirements based on specifications.
2. Test Planning: Define the scope, resources, and schedule for testing activities.
3. Test Case Design: Create detailed test cases and scenarios based on requirements.
4. Test Environment Setup: Prepare the necessary hardware and software for testing.
5. Test Execution: Execute the test cases ...read more
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
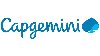
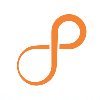
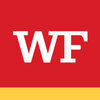
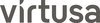
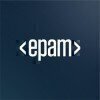
Top Interview Questions for Lead Software Engineer Related Skills
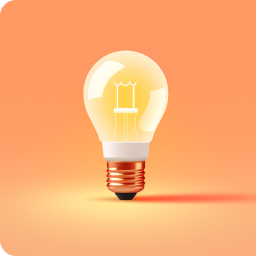
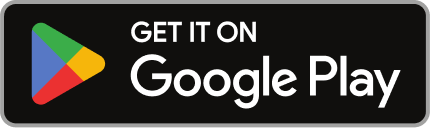
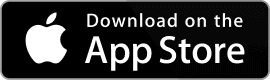
Reviews
Interviews
Salaries
Users
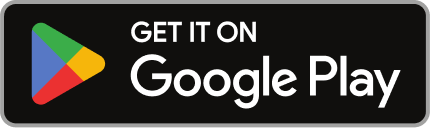
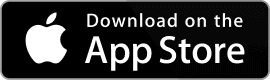