Junior .NET Developer
70+ Junior .NET Developer Interview Questions and Answers
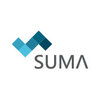
Asked in Suma Soft

Q. What is StringBuffer and where is it used?
StringBuffer is a mutable sequence of characters. It is used to efficiently manipulate strings.
StringBuffer is a class in Java that allows you to modify strings without creating a new object.
It is used when you need to concatenate a large number of strings efficiently.
StringBuffer is synchronized, so it is safe to use in multi-threaded environments.
It provides methods like append(), insert(), delete(), and reverse() to modify the string.
Example: StringBuffer sb = new StringBu...read more
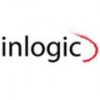
Asked in Inlogic Technologies

Q. What is the SQL query to retrieve the maximum salary from a database?
SQL query to retrieve the maximum salary from a database
Use the SELECT statement with the MAX function to retrieve the maximum salary
Specify the column name for the salary field in the table
Example: SELECT MAX(salary) FROM employees
Junior .NET Developer Interview Questions and Answers for Freshers
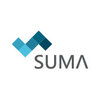
Asked in Suma Soft

Q. What is an ArrayList and where is it used?
ArrayList is a dynamic array that can store objects of any type. It is used to store and manipulate collections of data.
ArrayList is part of the System.Collections namespace in .NET.
It can store objects of any type, including value types and reference types.
It automatically resizes itself as elements are added or removed.
Elements can be accessed by their index.
It provides methods for adding, removing, and searching for elements.
Example: ArrayList names = new ArrayList(); name...read more
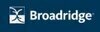
Asked in Broadridge Financial Solutions

Q. Coding round 1. Write a code for prime and odd numbers
Code for prime and odd numbers
For prime numbers, check if the number is divisible by any number less than itself
For odd numbers, check if the number is not divisible by 2
Use loops and conditional statements to implement the checks
Consider edge cases such as negative numbers and 0
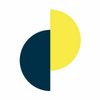
Asked in Casepoint

Q. How can you identify and delete duplicate rows in a SQL table?
Identifying and deleting duplicate rows in a SQL table can be done using SQL queries.
Use the GROUP BY clause along with the COUNT() function to identify duplicate rows.
Use the HAVING clause to filter out rows with a count greater than 1.
Use the DELETE statement with a subquery to delete duplicate rows.
Example: SELECT column1, column2, COUNT(*) FROM table_name GROUP BY column1, column2 HAVING COUNT(*) > 1;
Example: DELETE FROM table_name WHERE id NOT IN (SELECT MIN(id) FROM tab...read more
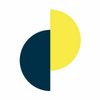
Asked in Casepoint

Q. How would you implement a recursive query in SQL? Provide an example.
To implement a recursive query in SQL, use Common Table Expressions (CTE) with a recursive part and a termination condition.
Use a Common Table Expression (CTE) to define the recursive part of the query.
Include a base case in the CTE to stop the recursion.
Reference the CTE in the main query to retrieve the results.
Example: WITH RecursiveCTE AS (SELECT * FROM TableName WHERE condition UNION ALL SELECT * FROM RecursiveCTE WHERE condition)
Junior .NET Developer Jobs
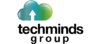
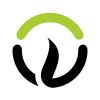
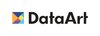
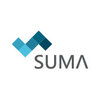
Asked in Suma Soft

Q. What are generics and how do you declare them?
Generics in .NET allow you to create reusable code that can work with different types.
Generics are declared using the 'generic' keyword followed by the type parameter(s) in angle brackets.
Example: 'public class MyGenericClass<T> {}'
You can use multiple type parameters separated by commas.
Example: 'public class MyGenericClass<T1, T2> {}'
Generics provide type safety and eliminate the need for casting.
Example: 'List<string> myList = new List<string>();'
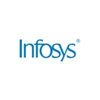
Asked in Infosys

Q. What are the key concepts of Object-Oriented Programming (OOP)?
Key concepts of OOP include encapsulation, inheritance, polymorphism, and abstraction.
Encapsulation: Bundling data and methods that operate on the data into a single unit (class). Example: Class with private fields and public methods.
Inheritance: Creating new classes based on existing classes, inheriting their attributes and behaviors. Example: Subclass extending a superclass.
Polymorphism: Objects of different classes can be treated as objects of a common superclass. Example:...read more
Share interview questions and help millions of jobseekers 🌟
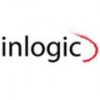
Asked in Inlogic Technologies

Q. What is your understanding of normalization in SQL?
Normalization in SQL is the process of organizing data in a database to reduce redundancy and improve data integrity.
Normalization is a technique used to eliminate redundant data and ensure data integrity.
It involves breaking down a table into smaller tables and defining relationships between them.
There are different normal forms such as 1NF, 2NF, 3NF, and BCNF.
For example, in a database of students and courses, instead of storing student details in the course table, a separa...read more
Asked in Qizo Technologies & Solutions

Q. Do you know SQL, What Databases are you familiar with?
Yes, I know SQL and I am familiar with various databases.
I have experience in writing SQL queries for Microsoft SQL Server, MySQL, and Oracle databases.
I am familiar with database design, normalization, and indexing.
I have worked with Entity Framework and LINQ to SQL for database access in .NET applications.
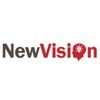
Asked in NewVision Software & Consultancy

Q. Find second highest salary,Oops concept what is asp.net core and difference.
Answering interview questions on finding second highest salary, OOPs concepts, and ASP.NET Core.
To find the second highest salary, we can use the SQL query 'SELECT MAX(salary) FROM employees WHERE salary < (SELECT MAX(salary) FROM employees)'.
OOPs concepts include inheritance, polymorphism, encapsulation, and abstraction.
ASP.NET Core is a cross-platform, open-source framework for building modern web applications.
The main difference between ASP.NET and ASP.NET Core is that ASP...read more
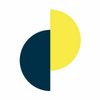
Asked in Casepoint

Q. What is the purpose of indexing in a database, and what are the different types of indexes?
Indexing in a database improves query performance by allowing faster retrieval of data.
Indexes are used to quickly locate data without having to search every row in a table.
Types of indexes include clustered, non-clustered, unique, and composite indexes.
Clustered indexes physically reorder the data in the table based on the index key.
Non-clustered indexes store a separate structure that contains the index key and a pointer to the actual data.
Unique indexes ensure that no two ...read more
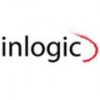
Asked in Inlogic Technologies

Q. What are the available access modifiers in programming?
Access modifiers control the visibility and accessibility of classes, methods, and variables in programming.
Public - accessible from anywhere
Private - accessible only within the same class
Protected - accessible within the same class and its subclasses
Internal - accessible within the same assembly
Protected Internal - accessible within the same assembly or subclasses
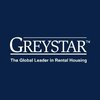
Asked in Greystar

Q. What is the difference between procedures and functions?
Procedures do not return a value, while functions return a value.
Procedures are used to perform an action, while functions are used to calculate and return a value.
Functions have a return type specified, while procedures do not.
Example: Procedure to display a message on the screen vs Function to calculate the sum of two numbers.
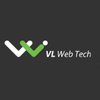
Asked in Venturelabour.com

Q. Why do we use interfaces and abstract methods?
Interfaces and abstract methods are used to achieve abstraction and provide a blueprint for classes to implement.
Interfaces allow for multiple inheritance and provide a contract for classes to adhere to.
Abstract methods define a method signature without implementation, allowing subclasses to provide their own implementation.
Interfaces and abstract methods promote code reusability and maintainability.
Interfaces can be used to achieve loose coupling and dependency injection.
Exa...read more
Asked in Emperor Solutions

Q. Why is the IsPostBack property used in the Page_Load event?
The isPostBack property is used in the Page_Load event to determine if the page is being loaded for the first time or if it is a postback.
The isPostBack property is a boolean property that indicates whether the page is being loaded for the first time or if it is a postback.
It is commonly used to differentiate between the initial page load and subsequent postbacks.
This property is useful when you want to perform certain actions only on the initial page load and not on postback...read more
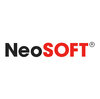
Asked in NeoSOFT

Q. What is dependency injection and what are its lifetimes?
Dependency injection is a design pattern where components are given their dependencies rather than creating them.
Dependency injection helps in making components loosely coupled, making it easier to test and maintain code.
There are three main lifetimes for dependencies: transient, scoped, and singleton.
Transient dependencies are created each time they are requested.
Scoped dependencies are created once per request.
Singleton dependencies are created only once and reused througho...read more
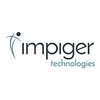
Asked in Impiger Technologies

Q. What class names would you use for a chess game using OOP concepts?
Class names for a chess game using OOP concepts include pieces, board, and game logic components.
ChessBoard: Represents the chessboard with an 8x8 grid.
ChessPiece: Base class for all chess pieces (e.g., Pawn, Rook, Knight).
Pawn: Inherits from ChessPiece, defines movement and capture rules.
Rook: Inherits from ChessPiece, defines vertical and horizontal movement.
Game: Manages the game state, player turns, and rules enforcement.
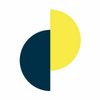
Asked in Casepoint

Q. Explain the difference between a correlated subquery and a nested subquery.
Correlated subquery refers to a subquery that depends on the outer query, while nested subquery is a subquery within another subquery.
Correlated subquery is executed for each row of the outer query, while nested subquery is executed only once.
Correlated subquery can reference columns from the outer query, while nested subquery cannot.
Example of correlated subquery: SELECT * FROM table1 WHERE column1 = (SELECT MAX(column2) FROM table2 WHERE table1.id = table2.id)
Example of nes...read more
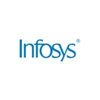
Asked in Infosys

Q. What is the difference between an abstract class and an interface?
Abstract classes can have both abstract and non-abstract methods, while interfaces can only have abstract methods.
Abstract classes can have constructors, fields, and properties, while interfaces cannot.
A class can inherit from only one abstract class, but can implement multiple interfaces.
Abstract classes are used when a class needs to provide a default implementation for some methods, while interfaces are used to define a contract for classes to implement.
Example: Abstract c...read more
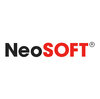
Asked in NeoSOFT

Q. what is CORS ? and we use this
CORS stands for Cross-Origin Resource Sharing, a security feature that allows servers to specify who can access their resources.
CORS is used to prevent web pages from making requests to a different domain than the one that served the original page.
It is implemented using HTTP headers like Access-Control-Allow-Origin.
CORS is commonly used in web development to enable secure cross-origin requests in browsers.
Example: If a frontend application on domain A wants to make a request...read more
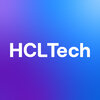
Asked in HCLTech

Q. What is the difference between connected and disconnected architecture in ADO.NET?
Connected architecture maintains a continuous connection to the database while disconnected architecture connects only when required.
Connected architecture is suitable for applications that require real-time data updates.
Disconnected architecture is suitable for applications that require occasional data updates.
Connected architecture uses DataReader while disconnected architecture uses DataSet.
Connected architecture is faster but consumes more resources than disconnected arch...read more
Asked in Paltech

Q. What is auto mapper,middleware, opps conceps
AutoMapper is a library for mapping objects, middleware is software that acts as a bridge between different systems, OOPs concepts are principles of object-oriented programming.
AutoMapper is a popular object-to-object mapping library in .NET that helps to eliminate repetitive mapping code.
Middleware in .NET is software that can handle requests and responses between a client and a server, providing additional functionalities like logging, authentication, etc.
OOPs concepts in ....read more
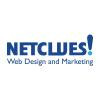
Asked in Netclues

Q. How can multiple inheritance be implemented in C#?
Multiple inheritance is not directly supported in C#, but can be achieved using interfaces.
C# does not support multiple inheritance of classes due to the Diamond Problem
Multiple inheritance can be achieved using interfaces by implementing multiple interfaces in a class
Example: public class MyClass : Interface1, Interface2 { }
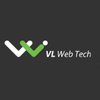
Asked in Venturelabour.com

Q. Why do we use TFS or GitHub?
TFS and GitHub are used for version control and collaboration in software development.
TFS and GitHub provide version control, allowing developers to track changes and collaborate on code.
They enable multiple developers to work on the same codebase simultaneously, avoiding conflicts.
They offer features like branching and merging, making it easier to manage different versions of code.
TFS and GitHub provide a centralized repository for code, ensuring its availability and backup....read more
Asked in Emperor Solutions

Q. What are DDL and DML queries?
DDL and DML are SQL queries used to manipulate databases. DDL is used to create, modify or delete database objects while DML is used to manipulate data.
DDL stands for Data Definition Language and is used to create, modify or delete database objects like tables, indexes, etc.
DML stands for Data Manipulation Language and is used to manipulate data in the database like inserting, updating or deleting records.
DDL queries include CREATE, ALTER and DROP statements while DML queries...read more
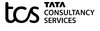
Asked in TCS

Q. Write code to reverse a string.
Code to reverse a string
Create an empty string variable to store the reversed string
Loop through the original string from the end to the beginning
Append each character to the empty string variable
Return the reversed string
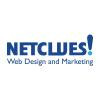
Asked in Netclues

Q. What are the advantages of .NET Core?
Dotnet Core offers improved performance, cross-platform compatibility, and open-source flexibility.
Improved performance compared to traditional .NET Framework
Cross-platform compatibility allows for development on Windows, macOS, and Linux
Open-source flexibility enables community contributions and faster updates
Support for microservices architecture and containerization
Enhanced security features and easier deployment options
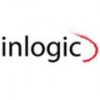
Asked in Inlogic Technologies

Q. What is the difference between substr() and charindex()?
substr() extracts a substring from a string based on start and length, while charindex() finds the position of a character or substring within a string.
substr() is used in SQL Server to extract a portion of a string based on the starting position and length.
charindex() is used in SQL Server to find the position of a specific character or substring within a string.
Example: SELECT SUBSTRING('Hello World', 1, 5) will return 'Hello'.
Example: SELECT CHARINDEX('o', 'Hello World') w...read more
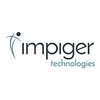
Asked in Impiger Technologies

Q. Write a database schema for a many-to-many relationship.
Schema for many to many relationship
Create a junction table to connect the two entities
Each record in the junction table represents a relationship between the two entities
Both entities have a many-to-many relationship with each other
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
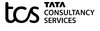
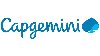
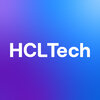
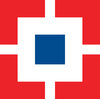
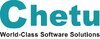
Top Interview Questions for Junior .NET Developer Related Skills
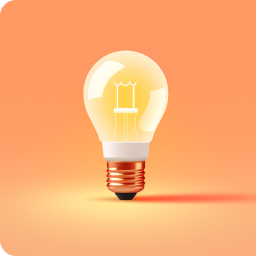
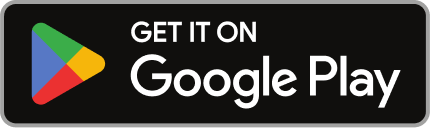
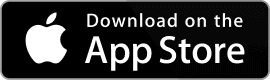
Reviews
Interviews
Salaries
Users
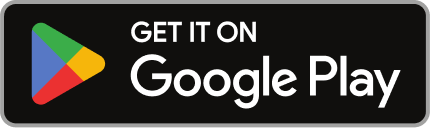
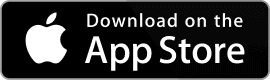