Golang Developer
90+ Golang Developer Interview Questions and Answers
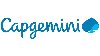
Asked in Capgemini

Q. can we return difference data type and how ,what architeture you worked in your project,does go have oops concepts
Yes, Go allows returning different data types. Go has OOP concepts and I have worked with microservices architecture.
Go allows returning different data types using interfaces.
I have worked with microservices architecture using Go and Docker.
Go has OOP concepts like structs and methods.
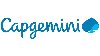
Asked in Capgemini

Q. difference between buffered channel and unbuffered channel,error handling methods,how you solve error
Buffered channels have a fixed capacity while unbuffered channels have no capacity limit.
Buffered channels allow sending multiple values without blocking until the buffer is full.
Unbuffered channels block until the sender and receiver are both ready to communicate.
Error handling methods include returning errors as values, using panic and recover, and logging errors.
Errors can be solved by identifying the root cause, implementing a fix, and testing the solution.
Golang Developer Interview Questions and Answers for Freshers
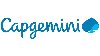
Asked in Capgemini

Q. why we use go,advantages of golang,does any other language support garbage collection,go routines why we use'multithreading
Go is a fast, efficient, and easy-to-learn programming language with built-in concurrency features.
Advantages of Go include its speed, simplicity, and concurrency features.
Garbage collection is supported by other languages such as Java, Python, and Ruby.
Go routines allow for easy and efficient concurrency without the need for traditional multithreading.
Go is used by companies such as Google, Uber, and Dropbox for its performance and scalability.
Asked in Seven Tech Solutions

Q. Does Golang support OOP concepts, and if so, how?
Go does not have traditional OOP concepts like classes and inheritance, but it does support some OOP principles.
Go supports encapsulation through the use of structs and methods.
Polymorphism can be achieved through interfaces.
Inheritance is not supported, but composition can be used instead.
Go also supports abstraction through the use of interfaces and packages.
Asked in Vizzve Services

Q. explain go path and go root.what is encapsulation.write a program on encapsulation
Explanation of Go path and Go root, and encapsulation with a program example.
Go path is an environment variable that specifies the location of Go source code and binaries.
Go root is the location where Go is installed on the system.
Encapsulation is the process of hiding implementation details and exposing only necessary information.
Example of encapsulation in Go can be creating a struct with private fields and public methods to access them.
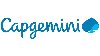
Asked in Capgemini

Q. do you know dockers and kubernets,what is docker used for, how you do unit testing how many looping concepts we have in go what is go path and go root
Questions on Docker, Kubernetes, unit testing, looping concepts, and Go path and root.
Docker is a containerization platform used for packaging and deploying applications. Kubernetes is a container orchestration tool used for managing containerized applications.
Unit testing in Go can be done using the built-in testing package and the 'go test' command.
Go has only one looping concept, the 'for' loop.
Go path is the location where Go packages are installed and Go root is the loca...read more
Golang Developer Jobs
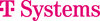
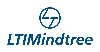
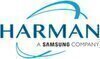
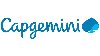
Asked in Capgemini

Q. define empty interface and empty struct,difference between array and slice,difference between function and method
Questions on Go programming language concepts
Empty interface is an interface with no methods. Empty struct is a struct with no fields.
Array has fixed size, slice is dynamic. Array is passed by value, slice is passed by reference.
Function is standalone, method is associated with a type. Method has a receiver, function does not.
Asked in Seven Tech Solutions

Q. How can you implement REST APIs in Golang?
REST API can be implemented in both Golang and other languages using HTTP methods and JSON data format.
In Golang, we can use third-party packages like Gorilla Mux and net/http to create REST APIs.
We can define routes and handlers for each HTTP method like GET, POST, PUT, DELETE.
We can use JSON encoding and decoding to send and receive data in REST API calls.
In other languages, we can use frameworks like Flask in Python, Express in Node.js to create REST APIs.
We can use tools ...read more
Share interview questions and help millions of jobseekers 🌟
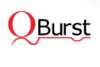
Asked in QBurst Technologies

Q. What is defer in GO ? If there are multiple defers in a function, what will be order of execution of these?
defer in Go is used to delay the execution of a function until the surrounding function returns.
Defer is used to ensure that a function call is performed at the end of the surrounding function, regardless of where the defer statement is located.
If there are multiple defers in a function, they will be executed in Last In, First Out (LIFO) order.
Example: func exampleFunc() { defer fmt.Println('First defer'); defer fmt.Println('Second defer'); } // Output: Second defer First def...read more
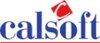
Asked in Calsoft

Q. What are the different types of channels in Go, and where can we use them?
Types of channels in Go and their use cases
Buffered channels: Allow multiple senders to send data without blocking until the buffer is full
Unbuffered channels: Synchronize goroutines by blocking sender until receiver is ready
Bidirectional channels: Allow both sending and receiving data
Receive-only channels: Restrict channel to only receive data
Send-only channels: Restrict channel to only send data
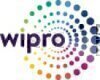
Asked in Wipro

Q. What is a channel, and what are the differences between buffered and unbuffered channels?
Channels are a way for goroutines to communicate. Buffered channels have a capacity while unbuffered channels do not.
Unbuffered channels block until a sender and receiver are ready to communicate
Buffered channels allow for asynchronous communication up to a certain capacity
Channels are typed, meaning they can only send and receive values of a specific type
Asked in Vizzve Services

Q. difference between pointer and slice,difference between slice and array,map,bridge patterns,abstrac pattern,singelton,explain 2 design patterns.explain channels.
Questions related to Go programming language concepts and design patterns.
Pointer is a variable that stores the memory address of another variable, while slice is a reference to an underlying array.
Arrays have a fixed size, while slices are dynamic and can grow or shrink.
Maps are key-value pairs, used to store and retrieve data based on a unique key.
Bridge pattern is used to separate an abstraction from its implementation, allowing them to vary independently.
Singleton pattern...read more
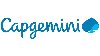
Asked in Capgemini

Q. what is complicity faced in your project write a program on sorting
Complicity faced in project: Handling concurrent requests and ensuring data consistency
Implemented mutex locks to prevent race conditions
Used channels to coordinate communication between goroutines
Ensured atomicity of operations on shared data
Implemented retry mechanisms to handle failed requests
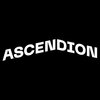
Asked in Ascendion

Q. How do you handle errors in your code?
I handle errors by using error handling mechanisms like try-catch blocks and returning error codes or messages.
Use try-catch blocks to catch and handle errors
Return error codes or messages to indicate the type of error
Implement error handling mechanisms like panic and recover in Go
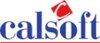
Asked in Calsoft

Q. how you create own package,explain your project
To create a package, define a new directory and add a file with package name and functions. My project is a web scraper.
Create a new directory with package name
Add a file with package name and functions
Import the package in main program
Use the functions in the package
Example: package name - scraper, functions - scrapeWebsite(url string) string
Example usage: import 'scraper', scraper.scrapeWebsite('https://example.com')
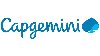
Asked in Capgemini

Q. what is channel,explains types of channel
A channel is a way for goroutines to communicate with each other and synchronize their execution.
Channels are typed and can only transmit values of that type.
There are two types of channels: buffered and unbuffered.
Unbuffered channels block until a sender and receiver are ready to communicate.
Buffered channels have a fixed capacity and can transmit values without blocking until the buffer is full.
Channels can be used to implement various synchronization patterns such as worke...read more
Asked in Securelayer7 Technologies

Q. What is the structure and implementation of a basic worker pool in Golang?
A worker pool in Golang efficiently manages concurrent tasks using goroutines and channels.
Define a worker function that processes tasks from a channel.
Create a pool of workers by launching multiple goroutines.
Use a channel to send tasks to workers and another to receive results.
Example: Use 'sync.WaitGroup' to wait for all workers to finish.
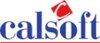
Asked in Calsoft

Q. What is concurrency and how can it be achieved using Go?
Concurrency is the ability to run multiple tasks simultaneously, achieving parallelism.
Go uses goroutines to achieve concurrency
Goroutines are lightweight threads managed by the Go runtime
Concurrency in Go is achieved using channels for communication between goroutines
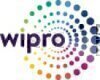
Asked in Wipro

Q. basics program on goroutines.expalin goroutine.explain parallisum and concurrency
Goroutines are lightweight threads of execution in Go that allow for concurrent programming.
Goroutines are created using the 'go' keyword followed by a function call.
Concurrency is the ability to run multiple tasks simultaneously, while parallelism is the ability to run multiple tasks at the same time.
Goroutines can communicate with each other using channels.
Example: go func() { fmt.Println('Hello, world!') }()
Example: c := make(chan int); go func() { c <- 1 }(); x := <-c; fm...read more
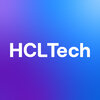
Asked in HCLTech

Q. What is GRPC? where it is used?
gRPC is a high-performance, open-source RPC framework developed by Google.
gRPC stands for Google Remote Procedure Call.
It uses HTTP/2 for transport, Protocol Buffers for serialization, and supports multiple programming languages.
gRPC is commonly used for building efficient and scalable microservices.
It allows for bidirectional streaming and authentication features.
Examples of companies using gRPC include Google, Netflix, and Square.
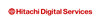
Asked in Hitachi Digital Services

Q. If the input is 3, the output should be 14. If the input is 4, the output should be 30. Explain the logic.
The output is calculated using a mathematical formula based on the input number.
The formula to calculate the output is: output = (input^2) + (input * 2)
For example, for input 3: output = (3^2) + (3 * 2) = 9 + 6 = 15
For example, for input 4: output = (4^2) + (4 * 2) = 16 + 8 = 24
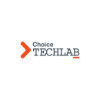
Asked in Choice Techlab

Q. How do you efficiently process large amounts of data?
Efficiently process large amounts of data by using parallel processing, optimizing algorithms, and utilizing data structures.
Utilize parallel processing techniques such as goroutines in Golang to process data concurrently.
Optimize algorithms to reduce time complexity and improve processing speed.
Use efficient data structures like maps, slices, and channels to store and manipulate data.
Consider using caching mechanisms to reduce the need for repeated data processing.
Implement ...read more
Asked in Seven Tech Solutions

Q. const variable and using and string replace
Const variables are immutable and can be used to declare values that won't change during runtime. String replace replaces a substring with another in a string.
Const variables are declared using the 'const' keyword and cannot be reassigned.
String replace can be used to replace a substring with another in a string.
Example: const pi = 3.14; str := 'Hello World'; newStr := strings.Replace(str, 'World', 'Golang', -1);
Example: const arr = [3]string{'apple', 'banana', 'orange'}; new...read more
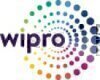
Asked in Wipro

Q. design pattersns explain and write a program on singelton
Singleton is a creational design pattern that ensures a class has only one instance and provides a global point of access to it.
Singleton pattern restricts the instantiation of a class to one object.
It is useful when exactly one object is needed to coordinate actions across the system.
Singleton pattern can be implemented using lazy initialization or eager initialization.
Example: Database connection, Logger, Configuration settings.
Singleton pattern can be implemented in Go usi...read more
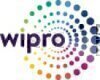
Asked in Wipro

Q. difference between microservice and monolithic you work an any payment
Microservices are small, independent services while monolithic is a single, large application.
Microservices are loosely coupled and can be developed and deployed independently.
Monolithic applications are tightly coupled and require a complete redeployment for any changes.
Microservices allow for better scalability and fault tolerance.
Monolithic applications are easier to develop and test.
Examples of microservices include Netflix, Amazon, and Uber.
Examples of monolithic applica...read more
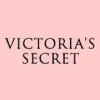
Asked in Victoria's Secret

Q. use case of defer in project and a logic of reverse array of string
The use case of defer in a project and a logic to reverse an array of strings.
Defer is used to ensure that a function call is performed later in a program's execution.
It is commonly used to close resources, unlock mutexes, or log the execution time.
To reverse an array of strings, iterate from both ends and swap the elements until the middle is reached.
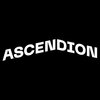
Asked in Ascendion

Q. How have you handled multi-threading?
I have handled multi threading by using goroutines and channels in Golang.
Used goroutines to run concurrent tasks
Used channels to communicate between goroutines
Avoided race conditions by using mutexes or sync package
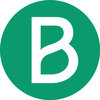
Asked in Brevo

Q. What is the process of implementing sharding in a database?
Sharding is a database architecture pattern that splits data across multiple servers to improve performance and scalability.
Identify the sharding key: Choose a key that will determine how data is distributed (e.g., user ID, geographic location).
Design the shard architecture: Decide on the number of shards and how they will be managed (e.g., horizontal vs. vertical sharding).
Implement data distribution: Write logic to route queries to the appropriate shard based on the shardin...read more
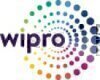
Asked in Wipro

Q. explain your project how do you work on unit testing
I have worked on various projects, including a web application for managing employee data.
I follow the Arrange-Act-Assert pattern for unit testing
I use testing frameworks like GoConvey and testify
I write test cases for both positive and negative scenarios
I use mocks and stubs to isolate dependencies
I aim for high code coverage to ensure thorough testing
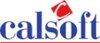
Asked in Calsoft

Q. program on interface,methods,write an implemention interface
Implementing an interface with methods in Go
Define an interface with method signatures
Create a struct that implements the interface methods
Use the 'implements' keyword to associate the struct with the interface
Call the interface methods on the struct instance
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
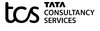
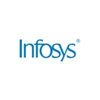
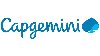
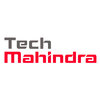
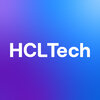
Top Interview Questions for Golang Developer Related Skills
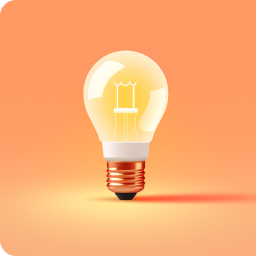
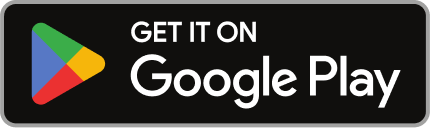
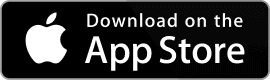
Reviews
Interviews
Salaries
Users
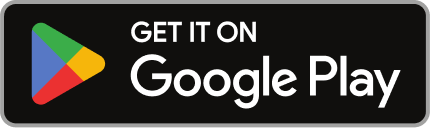
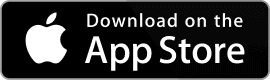