Associate Software Engineer
500+ Associate Software Engineer Interview Questions and Answers for Freshers
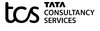
Asked in TCS

Q. Prime Time Again Problem Statement
You are given two integers DAY_HOURS
and PARTS
. Consider a day with DAY_HOURS
hours, which can be divided into PARTS
equal parts. Your task is to determine the total instances...read more
Calculate total instances of equivalent prime groups in a day divided into equal parts.
Divide the day into equal parts and find prime numbers in each part.
Identify prime groups where prime numbers occur at the same position in different parts.
Return the total number of equivalent prime groups found.
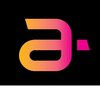
Asked in Amdocs

Q. Palindrome String Validation
Determine if a given string 'S' is a palindrome, considering only alphanumeric characters and ignoring spaces and symbols.
Note:
The string 'S' should be evaluated in a case-insensi...read more
Check if a given string is a palindrome after removing special characters, spaces, and converting to lowercase.
Remove special characters and spaces from the string
Convert the string to lowercase
Check if the modified string is a palindrome by comparing characters from start and end
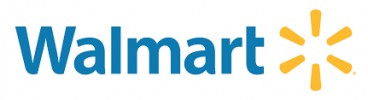
Asked in Walmart

Q. Bipartite Graph Problem Statement
Determine if a given graph is bipartite. A graph is bipartite if its vertices can be divided into two independent sets, 'U' and 'V', such that every edge ('u', 'v') connects a ...read more
The function checks whether a given graph is bipartite or not.
A bipartite graph can be divided into two independent sets such that every edge connects a vertex from one set to the other.
We can use graph coloring algorithm to check if the graph is bipartite.
Start by coloring the first vertex with one color and all its neighbors with the other color.
Continue coloring the remaining vertices, making sure that no adjacent vertices have the same color.
If at any point, we find that ...read more
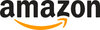
Asked in Amazon

Q. Minimum Number of Platforms Needed Problem Statement
You are given the arrival and departure times of N trains at a railway station for a particular day. Your task is to determine the minimum number of platform...read more
The task is to determine the minimum number of platforms needed at a railway station based on arrival and departure times of trains.
Sort the arrival and departure times in ascending order.
Use two pointers to keep track of overlapping schedules.
Increment platform count when a new train arrives before the previous one departs.
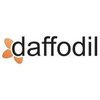
Asked in Daffodil Software

Q. Find the Longest Palindromic Substring
Given a string ‘S’ composed of lowercase English letters, your task is to identify the longest palindromic substring within ‘S’.
If there are multiple longest palindromic ...read more
Find the longest palindromic substring in a given string, returning the rightmost one if multiple substrings are of the same length.
Iterate through each character in the string and expand around it to check for palindromes
Keep track of the longest palindromic substring found so far
Return the rightmost longest palindromic substring
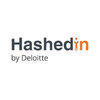
Asked in HashedIn by Deloitte

Q. Detect and Remove Loop in Linked List
For a given singly linked list, identify if a loop exists and remove it, adjusting the linked list in place. Return the modified linked list.
Expected Complexity:
Aim for a...read more
Detect and remove loop in a singly linked list in place with O(n) time complexity and O(1) space complexity.
Use Floyd's Cycle Detection Algorithm to identify the loop in the linked list.
Once the loop is detected, use two pointers approach to find the start of the loop.
Adjust the pointers to remove the loop and return the modified linked list.
Example: For input 5 2 and 1 2 3 4 5, output should be 1 2 3 4 5.
Associate Software Engineer Jobs
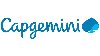
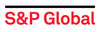
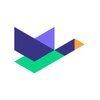
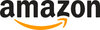
Asked in Amazon

Q. Implementing a Priority Queue Using Heap
Ninja has been tasked with implementing a priority queue using a heap data structure. However, he is currently busy preparing for a tournament and has requested your ass...read more
Implement a priority queue using a heap data structure by completing the provided functions: push(), pop(), getMaxElement(), and isEmpty().
Understand the operations: push() to insert element, pop() to remove largest element, getMaxElement() to return largest element, and isEmpty() to check if queue is empty.
Implement a heap data structure to maintain the priority queue.
Handle different types of queries based on the input provided.
Ensure correct output for each type 3 query.
Te...read more
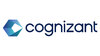
Asked in Cognizant

Q. Nth Fibonacci Number Problem Statement
Calculate the Nth term in the Fibonacci sequence, where the sequence is defined as follows: F(n) = F(n-1) + F(n-2)
, with initial conditions F(1) = F(2) = 1
.
Input:
The inp...read more
Calculate the Nth Fibonacci number efficiently using dynamic programming.
Use dynamic programming to store previously calculated Fibonacci numbers to avoid redundant calculations.
Start with base cases F(1) and F(2) as 1, then iterate to calculate subsequent Fibonacci numbers.
Return the Nth Fibonacci number as the final result.
Share interview questions and help millions of jobseekers 🌟
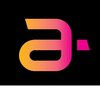
Asked in Amdocs

Q. Pythagorean Triplets Detection
Determine if an array contains a Pythagorean triplet by checking whether there are three integers x, y, and z such that x2 + y2 = z2 within the array.
Input:
The first line contai...read more
Detect if an array contains a Pythagorean triplet by checking if there are three integers x, y, and z such that x^2 + y^2 = z^2.
Iterate through all possible combinations of three integers in the array and check if x^2 + y^2 = z^2.
Use a nested loop to generate all possible combinations efficiently.
Return 'yes' if a Pythagorean triplet is found, otherwise return 'no'.
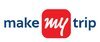
Asked in MakeMyTrip

Q. Sub Sort Problem Statement
You are given an integer array ARR
. Determine the length of the shortest contiguous subarray which, when sorted in ascending order, results in the entire array being sorted in ascendi...read more
The question asks to find the length of the shortest contiguous subarray that needs to be sorted in order to sort the whole array.
Iterate through the array and find the first element that is out of order from the left side.
Iterate through the array and find the first element that is out of order from the right side.
Find the minimum and maximum element within the subarray from the above steps.
Expand the subarray from both sides until all elements within the subarray are in the...read more
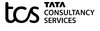
Asked in TCS

Q. Constellation Identification Problem
Given a matrix named UNIVERSE
with 3 rows and 'N' columns, filled with characters {#, *, .}, where:
- '*' represents stars.
- '.' represents empty space.
- '#' represents a separ...read more
The task is to identify constellations shaped like vowels within a matrix filled with characters {#, *, .}.
Iterate through the matrix to find 3x3 constellations shaped like vowels.
Check for vowels 'A', 'E', 'I', 'O', 'U' in each 3x3 constellation.
Print the vowels found in each constellation for each test case.
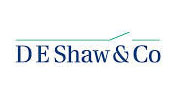
Asked in DE Shaw

Q. Buy and Sell Stock Problem Statement
Imagine you are Harshad Mehta's friend, and you have been given the stock prices of a particular company for the next 'N' days. You can perform up to two buy-and-sell transa...read more
The task is to determine the maximum profit that can be achieved by performing up to two buy-and-sell transactions on a given set of stock prices.
Iterate through the array of stock prices to find the maximum profit that can be achieved by buying and selling stocks.
Keep track of the maximum profit that can be achieved by considering all possible combinations of buy and sell transactions.
Ensure that you sell the stock before buying again to adhere to the constraints of the prob...read more
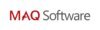
Asked in MAQ Software

Q. Remove String from Linked List Problem
You are provided with a singly linked list where each node contains a single character, along with a string 'STR'. Your task is to remove all occurrences of the string 'ST...read more
Remove all occurrences of a given string from a singly linked list by starting from the end of the list.
Traverse the linked list from the end to the beginning to efficiently remove the string occurrences.
Check for the string 'STR' in each node and remove it if found.
Continue checking for new occurrences of 'STR' after removal to ensure all instances are removed.
Return the head of the modified linked list after processing each test case.
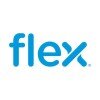
Asked in Flex

Q. Remove Vowels from a Given String
Given a string STR
of length N
, your task is to remove all the vowels present in that string and return the modified string.
Explanation:
English alphabets ‘a’, ‘e’, ‘i’, ‘o’, ...read more
Remove vowels from a given string while maintaining the relative position of other characters.
Iterate through each character in the string and check if it is a vowel (a, e, i, o, u).
If the character is not a vowel, add it to the modified string.
Return the modified string as the output.
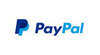
Asked in PayPal

Q. Replace Spaces in a String
Given a string STR
consisting of words separated by spaces, your task is to replace all spaces between words with the characters "@40".
Input:
The first line contains an integer ‘T’ d...read more
Replace spaces in a string with '@40'.
Iterate through the string and replace spaces with '@40'.
Return the modified string for each test case.
Handle multiple test cases by iterating through each one.
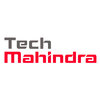
Asked in Tech Mahindra

Q. 1) What is NullPointerExceprion and give me a example?
NullPointerException is a runtime exception that occurs when a program tries to access or use an object reference that is null.
It is a common exception in Java programming.
It is thrown when a program attempts to use an object reference that has not been initialized.
It indicates that there is an attempt to access or invoke a method on an object that is null.
Example: String str = null; str.length();
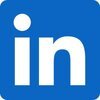
Asked in LinkedIn

Q. DFS Traversal Problem Statement
Given an undirected and disconnected graph G(V, E)
, where V
is the number of vertices and E
is the number of edges, the connections between vertices are provided in the 'GRAPH' m...read more
The question asks to print the DFS traversal of an undirected and disconnected graph.
Implement a Depth First Search (DFS) algorithm to traverse the graph.
Use a visited array to keep track of visited vertices.
For each unvisited vertex, start a DFS traversal and print the connected component.
Sort the vertices of each connected component in ascending order before printing.
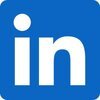
Asked in LinkedIn

Q. Check if Two Trees are Mirror
Given two arbitrary binary trees, your task is to determine whether these two trees are mirrors of each other.
Explanation:
Two trees are considered mirror of each other if:
- The r...read more
Check if two binary trees are mirrors of each other based on specific criteria.
Compare the roots of both trees.
Check if the left subtree of the first tree is the mirror of the right subtree of the second tree.
Verify if the right subtree of the first tree is the mirror of the left subtree of the second tree.
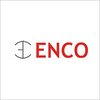
Asked in Enco Engineers Combine

Q. Colorful Knapsack Problem
You are given a set of 'N' stones, each with a specific weight and color. The goal is to fill a knapsack with exactly 'M' stones, choosing one stone of each color, so that the total we...read more
The goal is to fill a knapsack with exactly 'M' stones, one of each color, minimizing unused capacity.
Iterate through stones, keeping track of weights for each color
Sort stones by weight and color, then select one stone of each color
Calculate total weight for each combination and minimize unused capacity
If knapsack cannot be filled, return -1
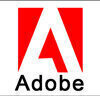
Asked in Adobe

Q. Maximum Sum Path in a Binary Tree Problem Statement
You are provided with a binary tree consisting of N
nodes where each node has an integer value. The task is to determine the maximum sum achievable by a simpl...read more
Find the maximum sum achievable by a simple path between any two nodes in a binary tree.
Traverse the binary tree to find all possible paths and calculate their sums.
Keep track of the maximum sum encountered during traversal.
Consider paths that may include the same node twice.
Implement a recursive function to explore all paths in the tree.
Optimize the solution to avoid redundant calculations.
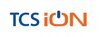
Asked in TCS iON

Q. Minimum Cash Flow Problem
Consider 'N' friends who have borrowed money from one another. They now wish to settle their debts by giving or taking cash among themselves in a way that minimizes the total cash flow...read more
The problem involves settling debts among friends by minimizing total cash flow.
Create a graph where nodes represent friends and edges represent debts.
Calculate the net amount each friend owes or is owed by summing up the debts and credits.
Use a recursive algorithm to settle debts by finding the maximum and minimum amounts owed.
Repeat the process until all debts are settled.
Output the total cash flow required to settle all debts.
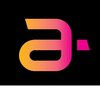
Asked in Amdocs

Q. Swap Two Numbers Problem Statement
Given two integers a
and b
, your task is to swap these numbers and output the swapped values.
Input:
The first line contains a single integer 't', representing the number of t...read more
Swap two numbers 'a' and 'b' and output the swapped values.
Create a temporary variable to store one of the numbers before swapping
Swap the values of 'a' and 'b' using the temporary variable
Output the swapped values as 'b' followed by 'a'
Example: If 'a' = 3 and 'b' = 4, after swapping 'a' will be 4 and 'b' will be 3
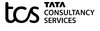
Asked in TCS

Q. Minimise Sum Problem Statement
You are given a matrix of 'N' rows and 'M' columns and a non-negative integer 'K'. Determine the minimum possible sum of all elements in each submatrix after performing at most 'K...read more
Given a matrix and a non-negative integer K, find the minimum possible sum of all elements in each submatrix after performing at most K decrements.
Iterate through all submatrices and find the minimum possible sum after performing decrements
Keep track of the number of decrements performed on each element
Use dynamic programming to optimize the solution
Ensure not to decrease any number below 0
Return the minimum possible sum modulo 10^9 + 7
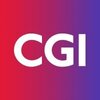
Asked in CGI Group

Q. Remove Vowels from a String
Given a string STR
of length N
, your task is to remove all the vowels from that string and return the modified string.
Input:
The first line of input contains an integer 'T' represen...read more
Remove all vowels from a given string and return the modified string.
Iterate through each character in the string and check if it is a vowel (a, e, i, o, u or A, E, I, O, U).
If the character is not a vowel, add it to a new string.
Return the new string with all vowels removed.
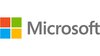
Asked in Microsoft Corporation

Q. Group Anagrams Problem Statement
Given an array or list of strings called inputStr
, your task is to return the strings grouped as anagrams. Each group should contain strings that are anagrams of one another.
An...read more
Group anagrams in an array of strings based on their characters.
Iterate through the array of strings and sort each string to create a key for grouping anagrams.
Use a hashmap to store the sorted string as key and the list of anagrams as values.
Return the values of the hashmap as the grouped anagrams.
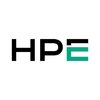
Asked in Hewlett Packard Enterprise

Q. Merge Sort Problem Statement
You are given a sequence of numbers, ARR
. Your task is to return a sorted sequence of ARR
in non-descending order using the Merge Sort algorithm.
Explanation:
The Merge Sort algorit...read more
Implement Merge Sort algorithm to sort a sequence of numbers in non-descending order.
Divide the input array into two halves recursively until each array has only one element.
Merge the sorted halves to produce a completely sorted array.
Time complexity of Merge Sort is O(n log n).
Example: Input: [3, 1, 4, 1, 5], Output: [1, 1, 3, 4, 5]
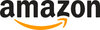
Asked in Amazon

Q. Rotting Oranges Problem Statement
You are given a grid containing oranges where each cell of the grid can contain one of the three integer values:
- 0 - representing an empty cell
- 1 - representing a fresh orange...read more
Find the minimum time required to rot all fresh oranges in a grid.
Iterate through the grid to find rotten oranges and their adjacent fresh oranges
Use BFS or DFS to simulate the rotting process and track the time taken
Return the minimum time taken to rot all fresh oranges or -1 if not possible
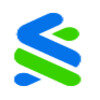
Asked in Standard Chartered

Q. Ways To Make Coin Change
Given an infinite supply of coins of varying denominations, determine the total number of ways to make change for a specified value using these coins. If it's not possible to make the c...read more
The task is to determine the total number of ways to make change for a specified value using given denominations.
Create a dynamic programming table to store the number of ways to make change for each value up to the target value.
Iterate through each denomination and update the table accordingly.
The final answer will be the value in the table at the target value.
Consider edge cases such as when the target value is 0 or when there are no denominations available.
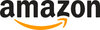
Asked in Amazon

Q. Postfix Expression Evaluation Problem Statement
Given a postfix expression, your task is to evaluate the expression. The operator will appear in the expression after the operands. The output for each expression...read more
Evaluate postfix expressions using a stack to compute results with modular arithmetic.
Use a stack to store operands as they are encountered.
When an operator is found, pop the required number of operands from the stack.
Perform the operation and push the result back onto the stack.
Ensure to apply modulo (10^9 + 7) to the results.
Example: For '3 4 +', push 3 and 4, then pop and compute 3 + 4 = 7.
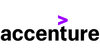
Asked in Accenture

Q. Arrange 10 coconuts in five lines such that each line contains 4 coconuts.
Arrange 10 coconuts in 5 lines with 4 coconuts in each line.
Arrange 4 coconuts in a straight line and place the remaining 6 coconuts in the gaps between them.
Place the first 4 coconuts in a line, then place the next 4 coconuts in a line below it, and so on.
The arrangement would look like this: C C C C C C C C C C C C C C C C C C C C
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
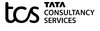
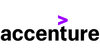
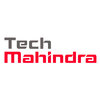
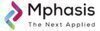
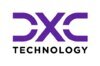
Top Interview Questions for Associate Software Engineer Related Skills
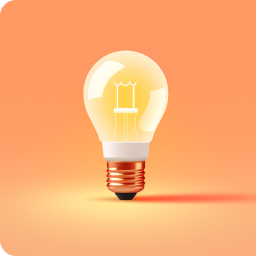
Calculate your in-hand salary
Confused about how your in-hand salary is calculated? Enter your annual salary (CTC) and get your in-hand salary
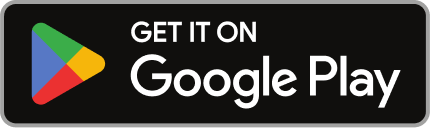
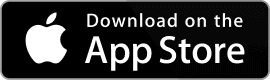
Reviews
Interviews
Salaries
Users
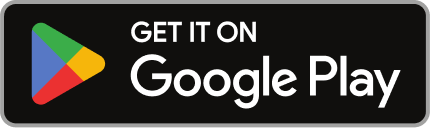
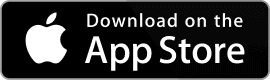