Software Development Engineer Intern
100+ Software Development Engineer Intern Interview Questions and Answers
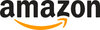
Asked in Amazon

Q. Say you're dealing with really long integers. They're too long to fit into a regular datatype, so linked lists are used to store them, with each node of the list containing one digit. Now the problem is, given...
read moreGiven two linked lists representing long integers, perform addition operation.
Traverse both linked lists simultaneously, starting from the head.
Perform addition of corresponding digits and keep track of carry.
Create a new linked list to store the result.
Handle cases where one list is longer than the other.
Handle the final carry if any.
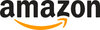
Asked in Amazon

Q. Given a singly-linked, non-circular linked list, swap the kth node with the kth last node in the list. Note that the length of the list is not known.
Swap kth node with kth last node in a singly-linked, non-circular linked list.
Traverse the linked list to find its length or use two pointers to find kth and kth last nodes.
Swap the nodes and update the pointers accordingly.
Handle edge cases such as k being out of bounds or kth and kth last nodes being the same.
Consider using recursion to traverse the linked list.
Software Development Engineer Intern Interview Questions and Answers for Freshers
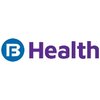
Asked in Bajaj Finserv Health

Q. What is the angle between the minute and hour hand at 1:15?
The angle between the minute and hour hand at 1:15 is 7.5 degrees.
At 1:15, the minute hand is at 3 and the hour hand is slightly past 1
Calculate the angles covered by each hand from 12 o'clock position
Subtract the smaller angle from the larger angle to get the angle between the hands
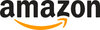
Asked in Amazon

Q. Given two linked lists representing two long numbers, add the numbers and store the result in a third linked list.
Add two long numbers and store the result in a third linked list.
Create a linked list to store the result
Traverse both input linked lists simultaneously, adding corresponding digits
Handle carry over from addition
If one linked list is longer than the other, handle remaining digits
Return the resulting linked list
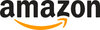
Asked in Amazon

Q. Given a Binary Search Tree, print the kth last node in inorder traversal of the tree
To print the kth last node in inorder traversal of a Binary Search Tree, we can use a modified inorder traversal algorithm.
Perform an inorder traversal of the BST, keeping track of the nodes visited in a stack.
Once the traversal is complete, pop k elements from the stack to get the kth last node.
Print the value of the kth last node.
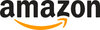
Asked in Amazon

Q. What are hamming distance and hamming weights? Write code to calculate hamming distance between 2 numbers.
Hamming distance is the number of differing bits between two binary strings. Hamming weight is the number of 1s in a binary string.
Hamming distance is used in error detection and correction codes.
Hamming weight is also known as population count or popcount.
To calculate hamming distance, XOR two numbers and count the number of 1s in the result.
Example: Hamming distance between 1010 and 1110 is 1.
Example: Hamming weight of 1010 is 2.
Software Development Engineer Intern Jobs

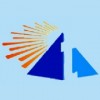
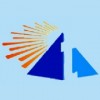
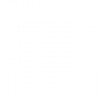
Asked in Jiffy Software

Q. Given a string, print each character of your name separated by a comma.
The task is to print each character of a name separated by commas, enhancing readability and formatting.
Use a loop to iterate through each character of the string.
Join the characters using a comma as a separator.
Example: For the name 'John', the output should be 'J,o,h,n'.
In Python, this can be done using: ','.join(name).
Asked in JTG E-Business Software

Q. What is the problem statement for the "Subsequence" question from LeetCode, which is categorized as medium to hard level and focuses on arrays?
Find the length of the longest increasing subsequence in an array of integers.
The problem involves finding the length of the longest increasing subsequence in an array of integers.
The subsequence does not have to be contiguous, but the elements must be in increasing order.
Dynamic programming can be used to solve this problem efficiently.
Example: Input: [10, 9, 2, 5, 3, 7, 101, 18] Output: 4 (The longest increasing subsequence is [2, 3, 7, 101])
Share interview questions and help millions of jobseekers 🌟
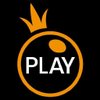
Asked in Pragmatic Play

Q. How does an internet webpage work and get the right content to be displayed?
An internet webpage works by sending a request to a server, which then processes the request and sends back the necessary content to be displayed.
When a user enters a URL in a web browser, the browser sends a request to a server hosting the webpage.
The server processes the request, retrieves the necessary files (HTML, CSS, JavaScript, images, etc.), and sends them back to the browser.
The browser then renders the content received from the server and displays it to the user.
Web...read more
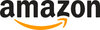
Asked in Amazon

Q. List some sorting algorithms. Why time complexity of merge sort is O(nlogn)
Sorting algorithms include bubble sort, insertion sort, selection sort, quick sort, merge sort, etc. Merge sort has O(nlogn) time complexity.
Sorting algorithms are used to arrange data in a specific order.
Merge sort is a divide and conquer algorithm that divides the input array into two halves, sorts each half, and then merges the sorted halves.
The time complexity of merge sort is O(nlogn) because it divides the input array into halves recursively until the base case is reach...read more
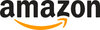
Asked in Amazon

Q. What data structure do you know? Advantages and disadvantages of singly and doubly linked lists
Singly and doubly linked lists are linear data structures used to store and manipulate data.
Singly linked lists use less memory than doubly linked lists
Doubly linked lists allow for traversal in both directions
Singly linked lists are faster for insertion and deletion at the beginning of the list
Doubly linked lists are faster for insertion and deletion in the middle of the list
Both have O(n) time complexity for searching
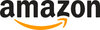
Asked in Amazon

Q. Given an array, what is the sum of the products of all subsequences?
The sum of the products of all subsequences in an array of strings.
Calculate the product of all elements in the array
Calculate the product of all possible subsequences
Sum up all the products of subsequences
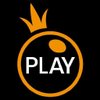
Asked in Pragmatic Play

Q. Describe a coding question where you had to swap two strings without using a third variable.
Swap two strings in an array without using a third variable through arithmetic operations.
Use XOR operation: str1 = str1 XOR str2; str2 = str1 XOR str2; str1 = str1 XOR str2.
For example, if str1 = 'hello' and str2 = 'world', after swapping, str1 = 'world' and str2 = 'hello'.
This method works for numeric values but can be adapted for strings by converting them to their ASCII values.
Another approach is to concatenate: str1 = str1 + str2; str2 = str1.substring(0, str1.length - s...read more
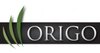
Asked in Origo Commodities

Q. What is the Many-Many Relationship in SQL? Explain with an example.
Many-Many Relationship in SQL is a type of relationship where each record in one table can be related to multiple records in another table.
Many-Many Relationship requires a junction table to connect the two tables
It is used when multiple records in one table can be related to multiple records in another table
Example: A student can enroll in multiple courses and a course can have multiple students enrolled, so a junction table 'Enrollment' will have student_id and course_id co...read more
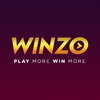
Asked in WinZO

Q. Describe different ways to traverse a tree.
Tree traversal involves visiting all nodes in a specific order, such as in-order, pre-order, or post-order.
In-order traversal visits nodes in the order: left, root, right. Example: For a tree with root 1, left child 2, right child 3, the order is 2, 1, 3.
Pre-order traversal visits nodes in the order: root, left, right. Example: For the same tree, the order is 1, 2, 3.
Post-order traversal visits nodes in the order: left, right, root. Example: For the same tree, the order is 2,...read more
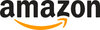
Asked in Amazon

Q. Write a program to copy a linked list to another linked list using pointers.
Copy a linked list to a new linked list using pointers, maintaining the same order and structure.
Define a new head for the destination list.
Iterate through the source list using a pointer.
For each node in the source list, create a new node in the destination list.
Copy the data from the source node to the new node.
Update the next pointer of the new node to point to the next node in the source list.
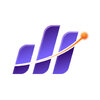
Asked in Bluestock ™

Q. If you were provided with a solution to a problem, how would you apply it to a real-world scenario?
Applying a provided solution involves understanding, adapting, and implementing it effectively in a real-world context.
Understand the problem: Analyze the context and requirements of the real-world scenario.
Adapt the solution: Modify the provided solution to fit the specific needs of the scenario.
Implement the solution: Develop a plan for integrating the solution into existing systems or processes.
Test and iterate: Evaluate the effectiveness of the solution and make necessary...read more
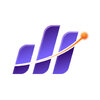
Asked in Bluestock ™

Q. Given a directed graph, how can I count the total number of nodes?
To count nodes in a directed graph, traverse the graph using DFS or BFS and maintain a count of visited nodes.
Use Depth-First Search (DFS) or Breadth-First Search (BFS) to traverse the graph.
Initialize a counter to zero before starting the traversal.
For each node visited, increment the counter.
Ensure to mark nodes as visited to avoid counting them multiple times.
Example: For a graph with nodes A, B, C, and edges A->B, A->C, the count will be 3.
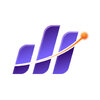
Asked in Bluestock ™

Q. What is a topological sort, and how is it used in graph algorithms?
Topological sort is an ordering of vertices in a directed acyclic graph (DAG) where each directed edge u -> v implies u comes before v.
Used to schedule tasks based on dependencies, e.g., course prerequisites.
Implemented using Depth-First Search (DFS) or Kahn's algorithm.
Example: For tasks A, B, C where A must be done before B and C, a valid order is A, B, C or A, C, B.
Topological sort is only applicable to Directed Acyclic Graphs (DAGs).
It helps in resolving dependencies in b...read more
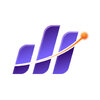
Asked in Bluestock ™

Q. What is the problem statement for Binary Search Trees (BST) in Data Structures and Algorithms (DSA)?
Binary Search Trees (BST) are data structures that maintain sorted data for efficient search, insertion, and deletion operations.
BST allows for O(log n) average time complexity for search, insert, and delete operations.
Each node has at most two children, with the left child containing values less than the parent and the right child containing values greater.
Example: Inserting 10, 5, and 15 into a BST results in a root node of 10, with 5 as the left child and 15 as the right c...read more
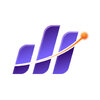
Asked in Bluestock ™

Q. What is your understanding of Figma in relation to front-end development?
Figma is a collaborative design tool that streamlines the front-end development process by providing design-to-code workflows.
Figma allows designers and developers to collaborate in real-time, ensuring alignment on design specifications.
Design systems created in Figma can be easily translated into front-end components, improving consistency.
Figma's export features enable developers to extract CSS, SVG, and other assets directly from designs, speeding up implementation.
Plugins...read more
Asked in Omniful

Q. What is the concept of polymorphism in programming?
Polymorphism is the ability of a function or method to behave differently based on the object it is acting upon.
Polymorphism allows objects of different classes to be treated as objects of a common superclass.
There are two types of polymorphism: compile-time (method overloading) and runtime (method overriding).
Example: Inheritance in object-oriented programming languages like Java allows for polymorphism.
Example: A method 'draw()' can be implemented differently in classes Cir...read more
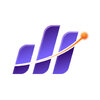
Asked in Bluestock ™

Q. What kind of real-time work is conducted at Bluestock?
Bluestock conducts real-time data analysis and monitoring to enhance user experience and optimize performance.
Real-time analytics for user engagement metrics, helping to tailor content and features.
Monitoring system performance to ensure minimal downtime and quick response to issues.
Implementing A/B testing in real-time to evaluate user preferences and improve product offerings.
Utilizing machine learning algorithms to provide personalized recommendations based on user behavio...read more
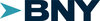
Asked in BNY

Q. What is addressing mode and its types?
Addressing mode is a technique used in computer architecture to specify how to calculate the effective address of an operand.
Addressing mode determines how the CPU accesses data from memory
Types of addressing modes include direct, indirect, indexed, and register
Examples: direct addressing mode - MOV A, 1000H, indirect addressing mode - MOV A, [BX], indexed addressing mode - MOV A, [BX+SI], register addressing mode - MOV A, BX
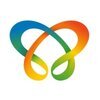
Asked in Capillary Technologies

Q. what is insertion sort and tell me implementation ?
Insertion sort is a simple sorting algorithm that builds the final sorted array one item at a time.
Iterate through the array starting from the second element.
Compare each element with the elements before it and insert it in the correct position.
Repeat until all elements are sorted.
Example: [5, 2, 4, 6, 1, 3] -> [2, 4, 5, 6, 1, 3] -> [2, 4, 5, 6, 1, 3] -> [1, 2, 4, 5, 6, 3] -> [1, 2, 3, 4, 5, 6]
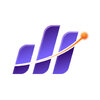
Asked in Bluestock ™

Q. Which programming language is better, Python or Java?
Python and Java are both powerful languages, each with unique strengths suited for different applications and developer preferences.
Ease of Learning: Python has a simpler syntax, making it more accessible for beginners. For example, 'print("Hello, World!")' in Python vs. 'System.out.println("Hello, World!");' in Java.
Performance: Java generally offers better performance due to its compiled nature and Just-In-Time (JIT) compilation, making it suitable for high-performance appl...read more
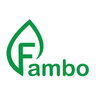
Asked in Fambo

Q. What are React Hooks, and how does each one function?
React Hooks are functions that let you use state and lifecycle features in functional components.
useState: Allows you to add state to functional components. Example: const [count, setCount] = useState(0);
useEffect: Enables side effects in functional components. Example: useEffect(() => { document.title = `Count: ${count}`; }, [count]);
useContext: Provides a way to pass data through the component tree without props drilling. Example: const value = useContext(MyContext);
useRedu...read more
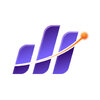
Asked in Bluestock ™

Q. Why wasn't the Jibble application added to my project?
The Jibble application wasn't added due to project scope, integration challenges, and prioritization of core features.
Project Scope: The project had defined objectives that did not include time tracking features.
Integration Challenges: Jibble may not have easily integrated with existing tools like Slack or Jira.
Prioritization: Focus was on core functionalities, such as user experience and performance, rather than additional tools.
Resource Constraints: Limited time and team re...read more
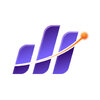
Asked in Bluestock ™

Q. Can you provide details about your web development projects?
I developed several web applications, focusing on user experience and responsive design using modern frameworks.
Created a personal portfolio website using React to showcase my projects and skills.
Developed an e-commerce site with Node.js and Express, implementing payment processing with Stripe.
Built a blog platform using Django, allowing users to create and manage their posts with a rich text editor.
Implemented a real-time chat application using Socket.io and Vue.js for seaml...read more
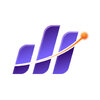
Asked in Bluestock ™

Q. What strategies can be employed to improve communication skills?
Improving communication skills involves practice, feedback, and various strategies to enhance clarity and effectiveness in interactions.
Practice active listening: Focus on the speaker, avoid interruptions, and summarize their points to ensure understanding.
Engage in public speaking: Join groups like Toastmasters to build confidence and improve verbal communication.
Seek feedback: Ask peers for constructive criticism on your communication style and areas for improvement.
Read an...read more
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
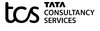
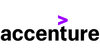
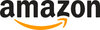
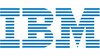
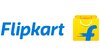
Top Interview Questions for Software Development Engineer Intern Related Skills
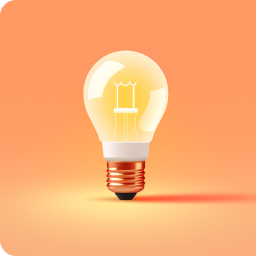
Calculate your in-hand salary
Confused about how your in-hand salary is calculated? Enter your annual salary (CTC) and get your in-hand salary
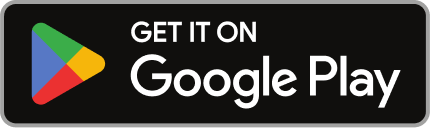
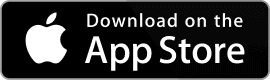
Reviews
Interviews
Salaries
Users
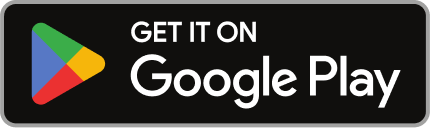
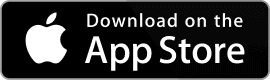