Senior Software Developer
800+ Senior Software Developer Interview Questions and Answers
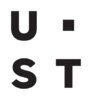
Asked in UST

Spring Boot offers basic annotations like @Controller, @RestController, @Service, @Repository, @Component.
@Controller - Used to mark a class as a Spring MVC controller.
@RestController - Combination of @Controller and @ResponseBody, used to create RESTful web services.
@Service - Used to mark a class as a service component in Spring.
@Repository - Used to mark a class as a data access component in Spring.
@Component - General-purpose stereotype annotation for any Spring-managed c...read more
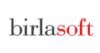
Asked in Birlasoft

React context is a built-in feature for passing data through the component tree, while React Redux is a library for managing global state in React applications.
React context is used for passing data down the component tree without having to pass props manually at every level.
React Redux is a library that provides a centralized store for managing global state in a React application.
React context is built into React, while React Redux is a separate library that needs to be inst...read more
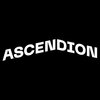
Asked in Ascendion

StringBuffer is synchronized and thread-safe, while StringBuilder is not synchronized and faster.
StringBuffer is synchronized, making it thread-safe for use in multi-threaded environments.
StringBuilder is not synchronized, making it faster but not thread-safe.
Use StringBuffer when thread safety is needed, and StringBuilder for better performance in single-threaded scenarios.
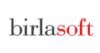
Asked in Birlasoft

Prototype chain in JavaScript is the mechanism by which objects inherit properties and methods from other objects.
In JavaScript, each object has a private property which holds a link to another object called its prototype.
If a property or method is not found on an object, JavaScript will look for it in the object's prototype, and so on up the chain.
This allows for inheritance in JavaScript, where objects can inherit properties and methods from their prototypes.
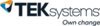
Asked in TEKsystems

Q. Write code to calculate the square of a series of numbers divisible by 3 using a lambda function.
Code to find square of series divisible by 3 using lambda function.
Create a list of numbers in the series
Use filter() function with lambda function to filter numbers divisible by 3
Use map() function with lambda function to find square of each number
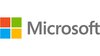
Asked in Microsoft Corporation

Q. Given a binary tree, return a doubly linked list of all the nodes at each level.
The function returns a doubly linked list of all the nodes at each level of a given binary tree.
Use a breadth-first search (BFS) algorithm to traverse the binary tree level by level.
Create a doubly linked list for each level and append the nodes to it.
Connect the doubly linked lists of each level to form the final result.
Senior Software Developer Jobs
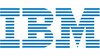
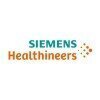
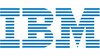

Asked in LearningMate Solutions

Q. Write regex patterns to extract the required information from a given text.
The answer provides regex patterns to extract specific information from a given text.
Use the appropriate regex pattern to match the desired information
Consider any variations or patterns in the text that may affect the regex
Test the regex patterns with sample texts to ensure accuracy
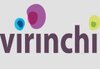
Asked in Virinchi Technologies

Q. What steps are needed to use a method from a different file in a Spring MVC project?
To use a method in a different file in a Spring MVC project, you need to create a bean for the class containing the method and then inject it where needed.
Create a bean for the class containing the method in the Spring configuration file
Use @Autowired annotation to inject the bean where the method needs to be used
Ensure the class containing the method is in the component scan path
Share interview questions and help millions of jobseekers 🌟
Asked in Naptico Services

Q. What is stored procedure, packages, functions and joins?
Stored procedures, packages, functions, and joins are all database concepts used to improve performance and functionality.
Stored procedures are precompiled SQL statements that can be executed multiple times.
Packages are a collection of related procedures, functions, and variables that can be used together.
Functions are similar to stored procedures but return a value.
Joins are used to combine data from multiple tables based on a common column.
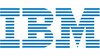
Asked in IBM

Q. 1. Why we go for microservice architecture 2. What is circuit breaker 3. What is service discovery 4.disadvantages of microservice 5. Disadvantage of stream api 6. Why we need default methods in functional inte...
read moreAnswers to questions related to microservice architecture, circuit breaker, service discovery, disadvantages of microservice, disadvantage of stream API, and default methods in functional interface.
Microservice architecture allows for scalability, flexibility, and easier maintenance of complex systems.
Circuit breaker is a design pattern used to prevent cascading failures in distributed systems.
Service discovery is the process of automatically detecting and locating services i...read more
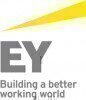
Asked in Ernst & Young

The @RestController annotation in Spring Boot is used to define a class as a RESTful controller.
Used to create RESTful web services in Spring Boot
Combines @Controller and @ResponseBody annotations
Eliminates the need to annotate every method with @ResponseBody
Returns data directly in the response body as JSON or XML
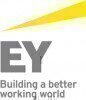
Asked in Ernst & Young

Hibernate provides several concurrency strategies like optimistic locking, pessimistic locking, and versioning.
Optimistic locking: Allows multiple transactions to read a row simultaneously, but only one can update it at a time.
Pessimistic locking: Locks the row for exclusive use by one transaction, preventing other transactions from accessing it.
Versioning: Uses a version number to track changes to an entity, allowing Hibernate to detect and resolve conflicts.
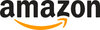
Asked in Amazon

Deep copy creates a new copy of an object with all nested objects also copied, while shallow copy creates a new copy of an object with references to nested objects.
Deep copy creates a new object and recursively copies all nested objects, resulting in a completely independent copy.
Shallow copy creates a new object but only copies references to nested objects, so changes in nested objects will reflect in both the original and copied objects.
Example: deep copy - copying an array...read more
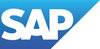
Asked in SAP

There are 204 squares on a chessboard.
The chessboard has 64 squares in total.
Each square can be divided into smaller squares, such as 1x1, 2x2, 3x3, etc.
The total number of squares can be calculated by adding the squares of all sizes together.
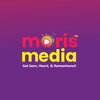
Asked in Moris Media

Q. What programming languages are you proficient in?
Proficient in Java, Python, and JavaScript.
Java
Python
JavaScript
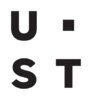
Asked in UST

Q. Fail safe fail fast Why is string immutable String buffer vs String builder Executor framework Lombok Transient Volatile Synchronized keyword Finally keyword Finalize Will finally execute if we return from try...
read moreQuestions related to Java concepts and frameworks
Fail safe fail fast - used in concurrent programming to handle exceptions and ensure thread safety
String is immutable to ensure thread safety and prevent unintended changes to the string
String buffer vs String builder - both are used to manipulate strings, but string builder is faster and not thread-safe
Executor framework - used for asynchronous task execution and thread pooling
Lombok Transient Volatile Synchronized keyword Fin...read more
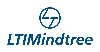
Asked in LTIMindtree

Override and virtual are keywords in C# used for method overriding and polymorphism.
Override keyword is used to provide a new implementation for a method in a derived class that is already defined in a base class.
Virtual keyword is used to allow a method to be overridden in a derived class.
Override keyword is used in the derived class to indicate that a method overrides a base class method.
Virtual keyword is used in the base class to indicate that a method can be overridden i...read more
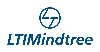
Asked in LTIMindtree

CTE is a temporary result set that can be referenced within a SELECT, INSERT, UPDATE, or DELETE statement in SQL.
CTEs help improve readability and maintainability of complex SQL queries.
They can be recursive, allowing for hierarchical data querying.
CTEs are defined using the WITH keyword followed by the CTE name and query.
Example: WITH cte_name AS (SELECT * FROM table_name) SELECT * FROM cte_name;
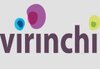
Asked in Virinchi Technologies

Q. What are the different annotations used in a Spring Boot project, and can you explain each one?
Annotations used in Spring Boot projects with explanations
1. @RestController - Used to define a controller and automatically includes @ResponseBody in all methods.
2. @RequestMapping - Maps HTTP requests to handler methods of MVC and REST controllers.
3. @Autowired - Used for automatic dependency injection.
4. @Component - Indicates that a class is a Spring component.
5. @Service - Indicates that a class is a service component in the business layer.
6. @Repository - Indicates that...read more
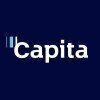
Asked in Capita

Independent microservices communicate through APIs, messaging queues, or event-driven architecture.
Use RESTful APIs for synchronous communication between microservices
Implement messaging queues like RabbitMQ or Kafka for asynchronous communication
Leverage event-driven architecture with tools like Apache Kafka or AWS SNS/SQS
Consider gRPC for high-performance communication between microservices
Asked in LogicValley Technologies

Q. How would you handle millions of records in your application?
Use efficient data structures and algorithms to handle large data sets.
Use indexing and partitioning to break down data into manageable chunks.
Implement caching and lazy loading to reduce memory usage.
Use parallel processing and distributed computing to improve performance.
Optimize database queries and use NoSQL databases for scalability.
Consider using data compression and encryption for security and storage efficiency.
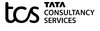
Asked in TCS

Q. What is the difference between a function and a stored procedure?
Functions return a value while stored procedures do not.
Functions can be used in SQL statements while stored procedures cannot.
Functions can be called from within stored procedures.
Functions can be used in views while stored procedures cannot.
Functions can have input parameters while stored procedures can have both input and output parameters.
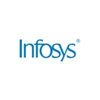
Asked in Infosys

Q. What is the difference between an abstract class and an interface?
Abstract class can have implementation while interface cannot.
Abstract class can have constructors while interface cannot.
A class can implement multiple interfaces but can only inherit from one abstract class.
Abstract class can have non-abstract methods while interface can only have abstract methods.
Abstract class can have instance variables while interface cannot.
Example of abstract class: public abstract class Animal { public void eat() { System.out.println('Eating'); } }
Ex...read more
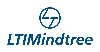
Asked in LTIMindtree

Memory for a string in Java is allocated on the heap, with a reference stored on the stack.
Strings in Java are immutable, so once created they cannot be changed.
String objects are stored on the heap, while references to them are stored on the stack.
String literals are stored in a special memory area called the String Pool.
String objects can also be created using the 'new' keyword.
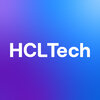
Asked in HCLTech

Q. What is the use of method references instead of lambda expressions in Java 8?
Method references provide a more concise way to refer to methods by name instead of using lambda expressions.
Method references can make code more readable and maintainable by reducing boilerplate code.
They can be used to refer to static methods, instance methods, and constructors.
Example: list.forEach(System.out::println) is equivalent to list.forEach(item -> System.out.println(item)).

Asked in LearningMate Solutions

Q. Describe your approach to debugging Python OOP code without using a code editor.
Debugging Python OOP code involves identifying and fixing errors in class definitions and method implementations.
Check for syntax errors: Ensure all colons, parentheses, and indentation are correct.
Verify class inheritance: Ensure the parent class is correctly referenced in the child class.
Inspect method definitions: Ensure methods are defined with 'self' as the first parameter.
Look for attribute initialization: Ensure all attributes are initialized in the __init__ method.
Che...read more
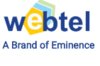
Asked in Webtel Electrosoft

Q. What is Multithreading? What is the use of background worker?
Multithreading is the concurrent execution of multiple threads to achieve parallelism and improve performance.
Multithreading allows multiple threads to run concurrently within a single process.
It enables parallel execution of tasks, improving performance and responsiveness.
Background worker is a component that simplifies multithreading by providing a dedicated thread for executing time-consuming tasks in the background.
It allows the main thread to remain responsive and not ge...read more
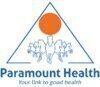
Asked in Paramount Health Services Tpa

Q. What is the difference between a string and a string builder?
String is immutable while StringBuilder is mutable.
String is a sequence of characters that cannot be modified once created.
StringBuilder is a dynamic object that can be modified and manipulated.
String concatenation creates a new string object while StringBuilder modifies the existing object.
String is thread-safe while StringBuilder is not.
Use String for small strings and StringBuilder for large strings or frequent manipulations.
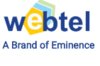
Asked in Webtel Electrosoft

Q. How do you create a file and append text to it?
To create a file and append text, use file handling functions in the programming language.
Open the file in append mode
Write the text to the file
Close the file
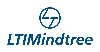
Asked in LTIMindtree

Types of hashmaps in Java include HashMap, LinkedHashMap, and TreeMap.
HashMap: basic implementation, no order guaranteed
LinkedHashMap: maintains insertion order
TreeMap: sorted by natural order or custom comparator
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
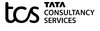
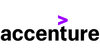
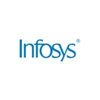
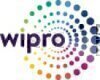
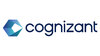
Top Interview Questions for Senior Software Developer Related Skills
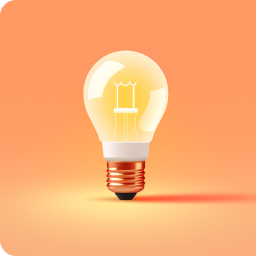
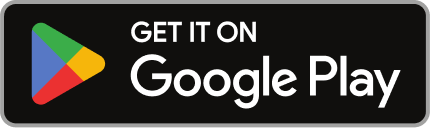
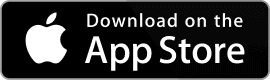
Reviews
Interviews
Salaries
Users
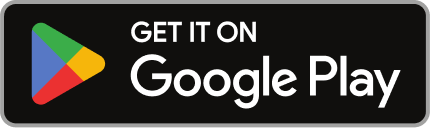
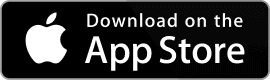