Senior Software Developer
700+ Senior Software Developer Interview Questions and Answers
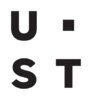
Asked in UST

Lambda expressions in Java 8 are used to provide a concise way to represent a single method interface.
Lambda expressions are used to provide implementation of functional interfaces.
They enable you to treat functionality as a method argument, or code as data.
Syntax of lambda expressions is (argument) -> (body).
Example: (int a, int b) -> a + b
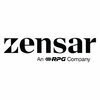
Asked in Zensar Technologies

ETL process involves extracting data from various sources, transforming it to fit the data warehouse schema, and loading it into the warehouse.
Extract: Data is extracted from different sources such as databases, files, APIs, etc.
Transform: Data is cleaned, filtered, aggregated, and transformed to match the data warehouse schema.
Load: Transformed data is loaded into the data warehouse for analysis and reporting.
Example: Extracting sales data from a CRM system, transforming it ...read more
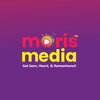
Asked in Moris Media

Q. How do you approach problem-solving in software development?
I approach problem-solving in software development by breaking down the issue, researching possible solutions, and collaborating with team members.
Identify the problem and gather all relevant information
Break down the problem into smaller, manageable tasks
Research possible solutions and consider different approaches
Collaborate with team members to brainstorm ideas and gather feedback
Implement and test the solution, making adjustments as needed
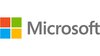
Asked in Microsoft Corporation

Q. Given two words, find the similarity between them. You have to develop your own sense of similarity here. Normalize length of LCS by the total length of the string.
The question asks to find the similarity between two words by developing our own sense of similarity.
Normalize the length of the Longest Common Subsequence (LCS) by dividing it by the total length of the string.
Implement a function that calculates the LCS between two words.
Divide the length of the LCS by the sum of the lengths of the two words.
Return the normalized similarity score as the result.
Asked in Naptico Services

Q. What is the architectural pattern for MVC and why is it segregated in this way?
MVC architecture pattern segregates an application into Model, View, and Controller components.
Model: represents the data and business logic
View: represents the user interface
Controller: handles user input and updates the model and view accordingly
This pattern helps in separating concerns and makes the code more maintainable and scalable
Examples: Ruby on Rails, ASP.NET MVC, Spring MVC
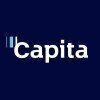
Asked in Capita

MVC components include Model (data), View (UI), and Controller (logic) for organizing code in a software application.
Model: Represents data and business logic, interacts with the database. Example: User model storing user information.
View: Represents the UI, displays data to the user. Example: HTML/CSS templates for displaying user profile.
Controller: Handles user input, updates the model, and selects the view to display. Example: User controller handling user registration.
Senior Software Developer Jobs
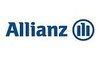
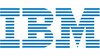
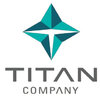
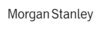
Asked in Morgan Stanley

A hash map in Java is a data structure that stores key-value pairs and uses hashing to efficiently retrieve values based on keys.
Hash map uses an array to store key-value pairs.
Keys are hashed to determine the index where the value will be stored.
Collision resolution techniques like chaining or open addressing are used to handle hash collisions.
Example: HashMap<String, Integer> map = new HashMap<>(); map.put("key", 123); int value = map.get("key");
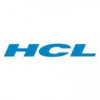
Asked in HCL Group

Q. How can you check if a string is not null without using string != null?
Checking if a string is not null without using string != null.
Use string.IsNullOrEmpty() method
Use string.IsNullOrWhiteSpace() method
Use string.Compare() method to compare with an empty string
Use string.Length property to check if length is greater than 0
Share interview questions and help millions of jobseekers 🌟
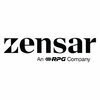
Asked in Zensar Technologies

Fact Table contains quantitative data and measures, while Dimension Table contains descriptive attributes.
Fact Table contains numerical data that can be aggregated, such as sales revenue or quantity sold
Dimension Table contains descriptive attributes for analysis, such as product name or customer details
Fact Table typically has a many-to-one relationship with Dimension Table
Fact Table is usually normalized, while Dimension Table is denormalized for faster query performance
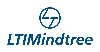
Asked in LTIMindtree

Intermediate operations return a new stream and allow further operations, while terminal operations produce a result or side-effect.
Intermediate operations include filter(), map(), sorted(), etc.
Terminal operations include forEach(), collect(), reduce(), etc.
Intermediate operations are lazy and only executed when a terminal operation is called.
Terminal operations are eager and trigger the stream to process the data.
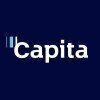
Asked in Capita

PUT is used to update or replace an existing resource, while POST is used to create a new resource.
PUT is idempotent, meaning multiple identical requests will have the same effect as a single request
POST is not idempotent, meaning multiple identical requests may have different effects
PUT requests are used to update an existing resource with a new representation, while POST requests are used to create a new resource
PUT requests are typically used for updating specific fields o...read more
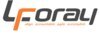
Asked in Foray Software

Q. What technology will be used in upcoming projects?
The upcoming projects will utilize a combination of technologies including Java, Python, and React.
Java
Python
React
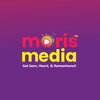
Asked in Moris Media

Q. Are you comfortable working with databases and SQL?
Yes, I am comfortable working with databases and SQL.
Proficient in writing complex SQL queries for data retrieval and manipulation
Experience with database design, normalization, and optimization
Familiarity with stored procedures, triggers, and views
Knowledge of indexing and query performance tuning
Examples: SELECT * FROM table WHERE condition; CREATE TABLE table_name (column1 datatype, column2 datatype, ...);
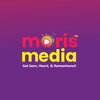
Asked in Moris Media

Q. Are you familiar with software testing methodologies and tools?
Yes, I am familiar with software testing methodologies and tools.
I have experience with both manual and automated testing
I am familiar with Agile testing methodologies such as Scrum and Kanban
I have used tools like Selenium, JUnit, and TestNG for testing
I understand the importance of regression testing and performance testing
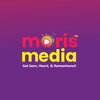
Asked in Moris Media

Q. Can you explain the concept of scalability in software architecture?
Scalability in software architecture refers to the ability of a system to handle increasing amounts of work or its potential to accommodate growth.
Scalability involves designing a system in a way that allows it to easily expand in terms of processing power, storage capacity, or network bandwidth.
Horizontal scalability involves adding more machines to distribute the load, while vertical scalability involves upgrading existing resources.
Examples of scalable architectures includ...read more
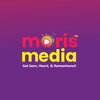
Asked in Moris Media

Q. Do you have experience with version control systems like Git?
Yes, I have extensive experience with version control systems like Git.
I have been using Git for version control in all my projects for the past 5 years.
I am proficient in creating branches, merging code, resolving conflicts, and performing code reviews using Git.
I have experience with popular Git hosting services like GitHub, GitLab, and Bitbucket.
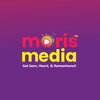
Asked in Moris Media

Q. Have you worked on both backend and frontend development?
Yes, I have experience in both backend and frontend development.
I have worked on backend development using languages like Java, Python, and Node.js to build APIs and handle server-side logic.
I have experience in frontend development using technologies like HTML, CSS, and JavaScript to create user interfaces and interactive web applications.
I have used frameworks like React and Angular for frontend development and frameworks like Spring and Express for backend development.
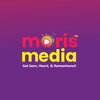
Asked in Moris Media

Q. Have you worked with any specific frameworks or libraries?
Yes, I have worked with several frameworks and libraries including React, Angular, and Node.js.
Worked with React for building user interfaces
Experience with Angular for front-end development
Utilized Node.js for server-side scripting
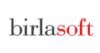
Asked in Birlasoft

A state in React is a JavaScript object that stores data relevant to a component and can be updated over time.
State is initialized in a component's constructor using this.state = {}
State can be updated using this.setState() method
State changes trigger re-rendering of the component
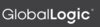
Asked in GlobalLogic

INNER JOIN returns rows when there is at least one match in both tables, while OUTER JOIN returns all rows from both tables.
INNER JOIN only returns rows that have matching values in both tables
OUTER JOIN returns all rows from both tables, filling in NULLs for unmatched rows
Types of OUTER JOIN include LEFT JOIN, RIGHT JOIN, and FULL JOIN
Example: SELECT * FROM table1 INNER JOIN table2 ON table1.id = table2.id
Example: SELECT * FROM table1 LEFT JOIN table2 ON table1.id = table2.i...read more
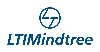
Asked in LTIMindtree

MVC architecture separates concerns, improves code organization, promotes reusability, and enhances maintainability.
Separates concerns: MVC separates the application into three main components - Model, View, and Controller, allowing for easier management of code and logic.
Improves code organization: Each component in MVC has a specific role, making it easier to organize and maintain code.
Promotes reusability: With clear separation of concerns, components can be reused in diff...read more
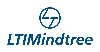
Asked in LTIMindtree

RANK() assigns a unique rank to each row based on the specified column, while ROW_NUMBER() assigns a unique sequential row number to each row.
RANK() may have gaps in the ranking if there are ties, while ROW_NUMBER() will not have any gaps.
RANK() will assign the same rank to rows with the same value, while ROW_NUMBER() will assign a unique row number to each row.
ROW_NUMBER() is used to generate a unique sequential number for each row in a result set, while RANK() is used to as...read more
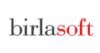
Asked in Birlasoft

Slice is a method that extracts a portion of an array without modifying the original array, while splice is a method that adds or removes elements from an array.
Slice returns a shallow copy of a portion of an array based on start and end index parameters.
Splice can add new elements to an array or remove existing elements by specifying the start index and number of elements to remove.
Example: const arr = [1, 2, 3, 4, 5]; arr.slice(1, 3) will return [2, 3], while arr.splice(1, ...read more
Asked in LogicValley Technologies

Q. Skip and skipkeep and what is difference between linq and sql?
Skip, SkipWhile, and SkipLast are LINQ methods used to skip elements in a sequence.
Skip(n) skips the first n elements in a sequence.
SkipWhile(predicate) skips elements in a sequence while the predicate is true.
SkipLast(n) skips the last n elements in a sequence.
LINQ is a language integrated query that allows querying data from different data sources.
SQL is a database management system that uses Structured Query Language to manage data.
LINQ is used to query data from different...read more
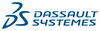
Asked in Dassault Systemes

Q. What is the minimum number of weighings required to identify the person with a different weight among nine individuals, where eight have equal weight and one has a different weight, using a balance scale?
To find the individual with a different weight among nine, only three weighings are needed using a balance scale.
First Weighing: Divide the nine individuals into three groups of three and weigh two groups against each other.
Second Weighing: Depending on the result, take the group that was heavier or lighter and divide it into three individuals, weighing two of them.
Third Weighing: If the second weighing is equal, the third individual is the one with the different weight; if n...read more
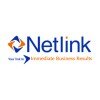
Asked in Netlink Software

Q. Rate your technical skills from 1 to 5, where 1 is the least and 5 is the most proficient.
4
Extensive experience in software development
Strong knowledge of programming languages and frameworks
Proficient in problem-solving and debugging
Ability to design and implement complex software systems
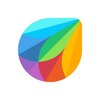
Asked in Freshworks

Threading is a way for a program to execute multiple tasks concurrently. Different scheduling algorithms determine the order in which threads are executed.
Threading allows multiple tasks to run concurrently within a single process.
Scheduling algorithms determine the order in which threads are executed, such as First-Come-First-Served (FCFS), Round Robin, Priority-Based Scheduling, etc.
FCFS schedules threads based on their arrival time, Round Robin assigns a fixed time slice t...read more
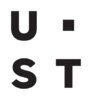
Asked in UST

N+1 SELECT problem in Hibernate occurs when a query results in N+1 database queries being executed instead of just one.
Occurs when a query fetches a collection of entities and then for each entity, another query is executed to fetch related entities individually
Can be resolved by using fetch joins or batch fetching to fetch all related entities in a single query
Example: Fetching a list of orders and then for each order, fetching the customer details separately
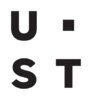
Asked in UST

SOLID principles are a set of five design principles in object-oriented programming to make software designs more understandable, flexible, and maintainable.
S - Single Responsibility Principle: A class should have only one reason to change.
O - Open/Closed Principle: Software entities should be open for extension but closed for modification.
L - Liskov Substitution Principle: Objects of a superclass should be replaceable with objects of its subclasses without affecting the func...read more
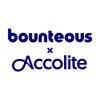
Asked in Bounteous x Accolite

Q. Given an array of integers, find the maximum value in each contiguous subarray of size k.
Find maximum for each contiguous subarray of size k from an array of size n.
Iterate through the array and keep track of maximum for each subarray of size k
Use a sliding window approach to efficiently calculate maximum for each subarray
Time complexity: O(n)
Example: arr = [10, 5, 2, 7, 1, 9, 4], k = 3, output = [10, 7, 7, 9, 9]
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
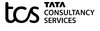
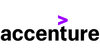
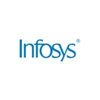
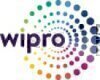
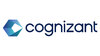
Top Interview Questions for Senior Software Developer Related Skills
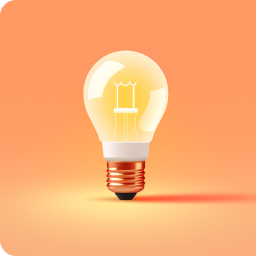
Calculate your in-hand salary
Confused about how your in-hand salary is calculated? Enter your annual salary (CTC) and get your in-hand salary
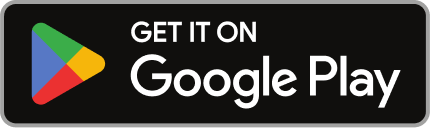
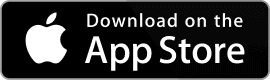
Reviews
Interviews
Salaries
Users
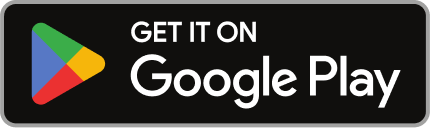
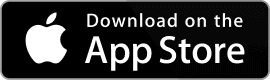