Senior Java Developer
400+ Senior Java Developer Interview Questions and Answers
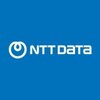
Asked in NTT Data

Q. What is one problem that interfaces solve that abstract classes do not?
Interfaces allow multiple inheritance, abstract classes do not.
Interfaces can be implemented by multiple classes, allowing for multiple inheritance.
Abstract classes can only be extended by one class, limiting inheritance.
Interfaces promote loose coupling and flexibility in design.
Abstract classes provide a common base implementation for subclasses.
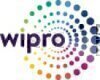
Asked in Wipro

Q. How do you allocate heap memory to the JVM, and what values do you pass?
To accumulate heap to the JVM, specify the initial and maximum heap size using -Xms and -Xmx flags respectively.
Use -Xms flag to specify the initial heap size, e.g. -Xms512m for 512 MB.
Use -Xmx flag to specify the maximum heap size, e.g. -Xmx1024m for 1 GB.
Example: java -Xms512m -Xmx1024m YourClassName
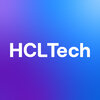
Asked in HCLTech

Q. Draw a low-level design for implementing a 'notify me' feature when an item is back in stock in an e-commerce application.
Implementation of 'notify me if item is back in stock' feature in an ecommerce application
Create a database table to store user notifications for out-of-stock items
Implement a service to check item availability and send notifications to subscribed users
Provide a user interface for users to subscribe to notifications for specific items
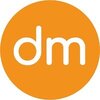
Asked in DataMetica

Q. 1.Calculate age without without using any inbuilt function 2.Department wise highest salary 3.Factorial number
Answering interview questions for Senior Java Developer
To calculate age, subtract birth year from current year
To find department wise highest salary, group by department and find max salary
To find factorial number, use a loop to multiply numbers from 1 to n
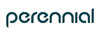
Asked in Perennial Systems

Q. with stream API, find employees with salary greater than 50000 and arrange it in descending order. using streams find average of numbers in a list
Use stream API to filter employees by salary and find average of numbers in a list.
Filter employees with salary > 50000 and sort in descending order using stream API
Calculate average of numbers in a list using stream API
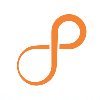
Asked in Persistent Systems

Q. Given an array, find all the numbers whose sum is 11 using streams only.
Use Java Streams to find pairs in an array that sum to 11.
Use IntStream.range to iterate through the array indices.
For each element, check if there's a complement (11 - current element) in the array.
Use a Set to store seen numbers for efficient lookup.
Example: For array [1, 10, 2, 9, 3, 8], pairs are (10, 1), (9, 2), (8, 3).
Senior Java Developer Jobs
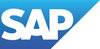
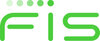
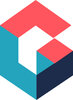
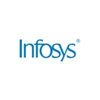
Asked in Infosys

Q. Can you perform all three operations (Get, Supply, Delete data) using a single API endpoint?
Yes, by implementing a RESTful API with different HTTP methods for each operation.
Implement a RESTful API with different endpoints for each operation: GET for retrieving data, POST for supplying data, and DELETE for deleting data.
For example, GET /data to retrieve data, POST /data to supply data, and DELETE /data/{id} to delete data by ID.
Use proper HTTP methods and status codes to handle each operation effectively.
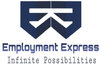
Asked in Employment Express

Q. What is the difference between ArrayList and LinkedList?
ArrayList is implemented as a resizable array while LinkedList is implemented as a doubly linked list.
ArrayList provides constant time for accessing elements while LinkedList provides constant time for adding or removing elements.
ArrayList is better for storing and accessing data while LinkedList is better for manipulating data.
ArrayList is faster for iterating through elements while LinkedList is faster for adding or removing elements in the middle of the list.
Share interview questions and help millions of jobseekers 🌟
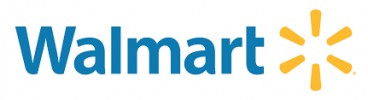
Asked in Walmart

Q. What are the common issues faced while deploying a service to AWS?
Common issues faced while deploying a service to AWS
Configuration errors leading to service downtime
Security misconfigurations exposing sensitive data
Performance issues due to improper resource allocation
Network connectivity problems affecting service availability
Lack of monitoring and logging causing difficulties in troubleshooting
Incompatibility issues with other AWS services or third-party integrations

Asked in Serosoft

Q. What is the difference between get and load in Hibernate?
get() method returns null if object is not found in database, while load() method throws ObjectNotFoundException.
get() method is eager loading while load() method is lazy loading.
get() method returns a fully initialized object while load() method returns a proxy object.
get() method is slower than load() method as it hits the database immediately.
load() method is faster than get() method as it returns a proxy object and hits the database only when required.
Asked in Caspex Corp

Q. Suppose your application experiences performance issues due to inefficient database queries and data access patterns. How would you analyze the database schema and query execution to identify performance bottle...
read moreAnalyzing database schema and query execution to identify performance issues
Review database schema for normalization and indexing
Analyze query execution plans for inefficiencies
Consider optimizing data access patterns and query structure
Use database profiling tools to identify bottlenecks
Implement caching mechanisms for frequently accessed data
Asked in Caspex Corp

Q. How do you enable debugging logs in a Spring Boot application?
Enable debugging log in Spring Boot application
Add 'logging.level.root=DEBUG' in application.properties file
Use '@Slf4j' annotation in the Java class to enable logging
Set 'logging.level.org.springframework=DEBUG' for Spring framework debugging
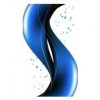
Asked in Saviynt

Q. Implement a custom HashMap, including efficient code to search for a key-value pair within a composite object (e.g., object within an object within a main object).
Implement a custom HashMap to efficiently search for a key value pair of a composite object.
Create a custom HashMap class with methods for adding key value pairs and searching for a specific key.
Implement a hash function to calculate the index of the key in the HashMap array.
For composite objects, override the hashCode and equals methods to ensure proper comparison and retrieval.
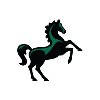
Asked in Lloyds Technology Centre

Q. In a microservice architecture, is it better to use multiple databases or a single database? Explain your reasoning.
Choosing between multiple databases or a single database in microservices depends on scalability, data isolation, and complexity.
Microservices with multiple databases allow for data isolation, enabling teams to choose the best database for their service's needs.
For example, a service handling user profiles might use a NoSQL database for flexibility, while a financial service might use a relational database for ACID compliance.
Using a single database can simplify data manageme...read more
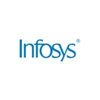
Asked in Infosys

Q. What is the difference between Spring Boot and Spring?
Spring is a framework for building Java applications, while Spring Boot is a tool that simplifies the setup and configuration of Spring applications.
Spring is a comprehensive framework that provides support for various functionalities like dependency injection, aspect-oriented programming, and more.
Spring Boot is an opinionated tool that simplifies the setup and configuration of Spring applications by providing defaults and auto-configuration.
Spring Boot includes embedded ser...read more
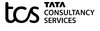
Asked in TCS

Q. Java 8 features and stream api example of distinct element in a list
Example of using Java 8 features and stream api to get distinct elements in a list.
Use the distinct() method of the Stream interface to get distinct elements in a list.
The distinct() method returns a stream consisting of the distinct elements of the original stream.
Example: List
list = Arrays.asList("apple", "banana", "apple", "orange"); list.stream().distinct().forEach(System.out::println); Output: apple banana orange
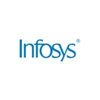
Asked in Infosys

Q. What are the Spring Boot annotations you commonly use?
Commonly used Spring Boot annotations include @RestController, @Autowired, @RequestMapping, @Service, @Component, @Repository.
@RestController - Used to define RESTful web services.
@Autowired - Used for automatic dependency injection.
@RequestMapping - Used to map web requests to specific handler methods.
@Service - Used to indicate that a class is a service component.
@Component - Used to indicate that a class is a Spring component.
@Repository - Used to indicate that a class is ...read more
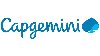
Asked in Capgemini

Q. What are the advantages of Spring Boot over Spring?
Spring Boot simplifies the development of Spring applications by providing out-of-the-box configurations and reducing boilerplate code.
Spring Boot provides a quick and easy way to set up a Spring application with minimal configuration.
It includes embedded servers like Tomcat, Jetty, or Undertow, eliminating the need for deploying WAR files.
Auto-configuration feature in Spring Boot automatically configures the application based on dependencies in the classpath.
Spring Boot Actu...read more
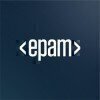
Asked in EPAM Systems

Q. What other principles, apart from SOLID, promote best coding practices?
Other principles for best coding practices include DRY, KISS, YAGNI, and design patterns.
DRY (Don't Repeat Yourself) - Avoid duplicating code by creating reusable functions or classes.
KISS (Keep It Simple, Stupid) - Write simple and easy-to-understand code rather than overcomplicating it.
YAGNI (You Aren't Gonna Need It) - Only implement functionality that is needed at the present moment, avoiding unnecessary features.
Design Patterns - Follow established design patterns like S...read more
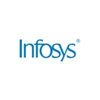
Asked in Infosys

Q. what about concurrent HashMap? spring framework IOC And DI
ConcurrentHashMap is a thread-safe implementation of the Map interface in Java.
ConcurrentHashMap allows multiple threads to read and write to the map concurrently without the need for external synchronization.
It is part of the java.util.concurrent package and provides better performance in multi-threaded environments compared to synchronized HashMap.
Spring framework supports the use of ConcurrentHashMap for managing beans in a concurrent environment.
Inversion of Control (IoC)...read more
Asked in Caspex Corp

Q. What is the difference between Monolithic SOA and Microservices architecture?
Monolithic SOE is a single large application while Microservices architecture breaks down the application into smaller, independent services.
Monolithic SOE is a single, self-contained application where all components are tightly coupled.
Microservices architecture breaks down the application into smaller, independent services that communicate through APIs.
Monolithic SOE is easier to develop and test but can be harder to scale and maintain.
Microservices architecture allows for ...read more
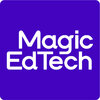
Asked in Magic Edtech

Q. Describe a situation where you were unable to implement a custom MongoDB query at the repository layer.
Creating custom MongoDB queries in the repository layer is essential for efficient data retrieval and manipulation.
Use MongoTemplate for custom queries: Example: mongoTemplate.find(query, YourEntity.class).
Utilize Query and Criteria classes to build dynamic queries: Example: Query query = new Query(Criteria.where('field').is(value)).
Implement custom repository interfaces for complex queries: Example: public interface YourRepository extends MongoRepository<YourEntity, String>,...read more
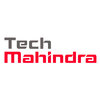
Asked in Tech Mahindra

Q. Write a Java program to print even numbers using one thread and odd numbers using another thread.
Program to print even and odd numbers using two threads in Java
Create two threads, one for even numbers and one for odd numbers
Use a loop to generate numbers and check if they are even or odd
Print the numbers using the respective threads
Use synchronization to ensure alternate printing of even and odd numbers
Asked in Aera Software Technology

Q. Write a function that removes characters before a '#' symbol based on the number of '#' symbols. For example, 'abc#d' should become 'abcd', and 'abc##d' should become 'ad'.
Write code to remove number character before hash based on count of hash
Count the number of hashes in the input string
Loop through the string and remove the character before the hash based on the count of hashes
Return the modified string
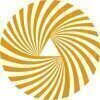
Asked in Altimetrik

Q. Can you explain the Java design patterns that you utilized in your project?
I utilized several design patterns in my projects to enhance code maintainability and scalability.
Singleton Pattern: Ensured a single instance of a configuration manager throughout the application.
Factory Pattern: Used to create different types of user objects based on user roles, promoting loose coupling.
Observer Pattern: Implemented for event handling, allowing multiple components to react to changes in the state of an object.
Strategy Pattern: Employed to define a family of...read more
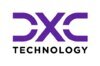
Asked in DXC Technology

Q. HOW TO CREATE OUR OWN IMMUTABLE CLASS? WHY IMMUTABLE CLASS
Immutable classes in Java are classes whose objects cannot be modified once they are created.
Make the class final to prevent inheritance
Make all fields private and final
Do not provide setter methods for fields
Ensure that any mutable objects within the class are also immutable
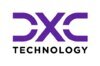
Asked in DXC Technology

Q. In which situation do we use @Primary and @Qualifier?
Use @Primary to specify a primary bean when multiple beans of the same type are present. Use @Qualifier to specify a specific bean when multiple beans of the same type are present.
Use @Primary to indicate the primary bean to be used when multiple beans of the same type are present in the Spring application context.
Use @Qualifier along with @Autowired to specify a specific bean to be injected when multiple beans of the same type are present.
Example: @Primary annotation can be ...read more
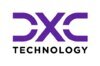
Asked in DXC Technology

Q. What is the difference between composition and aggregation?
Composition is a strong relationship where the child object cannot exist independently of the parent object, while aggregation is a weak relationship where the child object can exist independently.
Composition is a 'has-a' relationship, where the child object is a part of the parent object.
Aggregation is a 'has-a' relationship, where the child object is not a part of the parent object.
In composition, the child object is created and destroyed along with the parent object.
In agg...read more
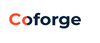
Asked in Coforge

Q. Different exceptions in Spring Boot
Spring Boot has various exceptions for different scenarios.
DataAccessException - for database access errors
HttpMessageNotReadableException - for errors in HTTP message conversion
MethodArgumentNotValidException - for validation errors in request parameters
NoSuchBeanDefinitionException - for errors in bean configuration
UnauthorizedException - for authentication and authorization errors
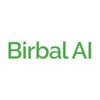
Asked in Birbal AI

Q. How do you optimize your database when handling large data?
Optimizing database for handling large data involves indexing, partitioning, denormalization, and using appropriate data types.
Use indexing on frequently queried columns to improve search performance.
Partition large tables to distribute data across multiple storage devices for faster access.
Denormalize data by reducing the number of joins needed for queries.
Use appropriate data types to minimize storage space and improve query performance.
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
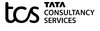
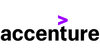
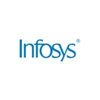
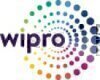
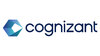
Top Interview Questions for Senior Java Developer Related Skills
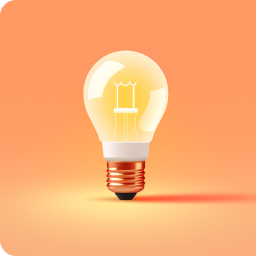
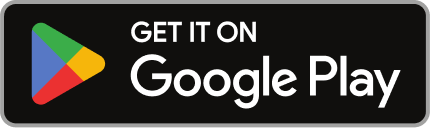
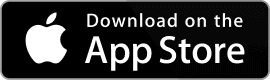
Reviews
Interviews
Salaries
Users
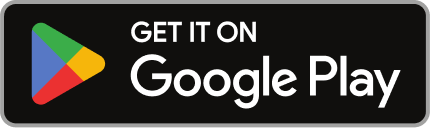
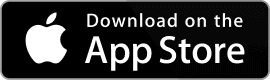