Senior Java Developer
400+ Senior Java Developer Interview Questions and Answers
Asked in Smartavya Analytica

Q. What is Dependency Injection? What are it's types (Constructor Based, Setter Based, Field Injection).
Dependency Injection is a design pattern where the dependencies of an object are provided externally rather than created within the object itself.
Types of Dependency Injection: Constructor Based, Setter Based, Field Injection
Constructor Based: Dependencies are provided through the constructor of the class
Setter Based: Dependencies are provided through setter methods
Field Injection: Dependencies are directly assigned to fields of the class
Asked in Smartavya Analytica

Q. What is Springboot? difference between Spring and Springboot. What is difference between @Component and @ComponentScan
Springboot is a framework that simplifies the development of Java applications by providing pre-configured settings and conventions.
Springboot is built on top of the Spring framework, providing a more streamlined way to build Spring applications.
Springboot eliminates the need for XML configuration files and allows for easier setup of dependencies.
Difference between @Component and @ComponentScan: @Component is used to mark a class as a Spring component, while @ComponentScan is...read more
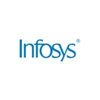
Asked in Infosys

Q. A backend query yields 500 results, but the UI requires sorting and displaying only 10 results per page. How would you help the UI achieve this?
Implement pagination in UI by limiting results to 10 per page.
Implement pagination logic in UI to display 10 results per page.
Use backend query parameters like 'offset' and 'limit' to fetch specific results.
Utilize front-end frameworks like React or Angular for efficient pagination implementation.
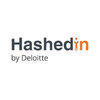
Asked in HashedIn by Deloitte

Q. Monolith vs Microservices difference? which is better and why?
Monolith is a single, large application while microservices are smaller, independent services. Microservices are better for scalability and flexibility.
Monolith is a single, large application where all components are tightly coupled. Microservices are smaller, independent services that communicate with each other through APIs.
Monoliths are easier to develop and deploy initially but become harder to maintain and scale as they grow. Microservices allow for better scalability an...read more
Asked in Smartavya Analytica

Q. Garbage Collection in Java? what is the process of GC? how can we explicitly invoke Garbage Collector?
Garbage Collection in Java is the process of automatically reclaiming memory by destroying unused objects.
Garbage Collection is a process in Java where the JVM automatically reclaims memory by destroying objects that are no longer referenced.
The process of GC involves marking objects that are still in use, sweeping and deleting objects that are no longer in use, and compacting the memory to reduce fragmentation.
We can explicitly invoke Garbage Collector in Java using System.g...read more
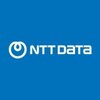
Asked in NTT Data

Q. How does Spring Boot determine which data source to use for application execution when multiple data sources are available and no specific profile has been defined?
Spring Boot uses @Primary annotation to determine which data source to use when multiple are available.
Spring Boot uses the @Primary annotation to specify the primary bean to be used when multiple beans of the same type are present.
If no specific profile is defined, Spring Boot will use the bean marked with @Primary as the default data source.
Developers can also use @Qualifier annotation to specify which bean to use if @Primary is not defined.
Senior Java Developer Jobs
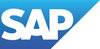
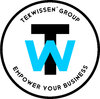
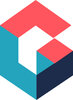
Asked in Caspex Corp

Q. Your application is experiencing memory leaks and frequent garbage collection processes impacting its performance. How would you identify and diagnose memory-related issues in a Java application?
Identify and diagnose memory leaks in Java using profiling tools, heap dumps, and code analysis.
Use a profiling tool like VisualVM or YourKit to monitor memory usage and identify memory leaks.
Analyze heap dumps with tools like Eclipse MAT to find objects that are not being garbage collected.
Check for common memory leak patterns, such as static collections holding references to objects.
Review code for improper use of listeners or callbacks that may prevent objects from being g...read more
Asked in Tandem Crystals

Q. Find the complexity of the given algorithms? How do you optimise this further for performance and scalability?
Complexity analysis and optimization of algorithms for performance and scalability.
Determine the time complexity (Big O notation) and space complexity of the algorithm.
Identify bottlenecks and optimize code by reducing unnecessary operations or improving data structures.
Use efficient algorithms and data structures like hash maps, binary search trees, and dynamic programming.
Parallelize tasks to leverage multi-core processors for improved performance.
Consider scalability by de...read more
Share interview questions and help millions of jobseekers 🌟
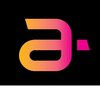
Asked in Amdocs

Garbage collector in Java is a built-in mechanism that automatically manages memory by reclaiming unused objects.
Garbage collector runs in the background to reclaim memory from objects that are no longer in use.
It helps prevent memory leaks and optimize memory usage.
Examples of garbage collectors in Java include Serial, Parallel, CMS, G1, and Z Garbage Collectors.
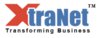
Asked in Xtranet Technologies

Q. What is a singleton, how do you create a singleton class, and what are the benefits?
Singleton is a design pattern that restricts the instantiation of a class to one object.
To create a singleton class, make the constructor private, create a static method to get the instance of the class, and create a private static variable to hold the instance.
Benefits of singleton include ensuring only one instance of the class exists, providing a global point of access to the instance, and reducing the number of objects created in the system.
Example: java.lang.Runtime is a...read more
Asked in Transforma

Q. BELOW SQL QUESTIONS: 1.TRUNCATED VS DELETE VS DROP 2.WHAT IS COMPOSITE KEY 3.Write query to find customer details whos average between 1000 and 2000 customer and order different table link with customer_id
SQL questions on Truncate, Delete, Drop, Composite Key and Query to find customer details
Truncate removes all data from a table, Delete removes specific data, Drop removes the entire table
Composite key is a combination of two or more columns that uniquely identifies a row in a table
SELECT * FROM customer c JOIN order o ON c.customer_id = o.customer_id WHERE AVG(o.amount) BETWEEN 1000 AND 2000
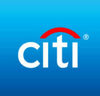
Asked in Citicorp

Q. How will you handle an out-of-memory exception?
Handle out-of-memory exception by analyzing heap dump and optimizing code.
Analyze heap dump to identify memory leaks
Optimize code to reduce memory usage
Increase heap size if necessary
Use memory profiling tools like JProfiler or VisualVM
Avoid creating unnecessary objects
Use caching to reduce object creation
Implement garbage collection strategies
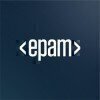
Asked in EPAM Systems

Q. what is equals and hashcode and does Object class implement equals method ?
equals and hashcode are methods in Java used for comparing objects and generating hash codes respectively. Object class does implement equals method.
equals method is used to compare two objects for equality. It is overridden in most classes to provide custom comparison logic.
hashcode method is used to generate a unique integer value for an object. It is used in hash-based collections like HashMap.
Object class does implement equals method, but it uses reference equality (==) f...read more
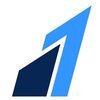
Asked in Razorpay

Q. What is the software development process at your company?
The system or process of a software company involves various stages from planning to deployment.
The process starts with requirement gathering and analysis.
Then comes the design and development phase.
Testing and quality assurance is an important part of the process.
Deployment and maintenance are the final stages.
Agile and DevOps methodologies are commonly used.
Tools like JIRA, Git, Jenkins, etc. are used for project management and automation.
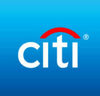
Asked in Citicorp

Q. What is the difference between shallow and deep comparison in Strings? Provide a code example.
Shallow and deep comparison in Strings with code example
Shallow comparison checks if two String variables refer to the same object in memory
Deep comparison checks if two String variables have the same sequence of characters
Shallow comparison can be done using the '==' operator
Deep comparison can be done using the 'equals()' method
Example: String str1 = 'hello'; String str2 = 'hello'; str1 == str2; //shallow comparison returns true
Example: String str1 = 'hello'; String str2 = ...read more
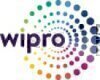
Asked in Wipro

Q. Internal architecture of Spring boot, how enable autoconfiguration works
Spring Boot uses autoconfiguration to automatically configure the Spring application based on dependencies and properties.
Spring Boot autoconfiguration is achieved through @EnableAutoConfiguration annotation
Autoconfiguration classes are located in the org.springframework.boot.autoconfigure package
Autoconfiguration classes are conditionally applied based on the presence of specific classes or properties
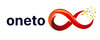
Asked in Onetoinfinite

Q. Write simple ms architecture layer to fetch emp list? Explain about monolithic and MS archtecture? Write Singleton class? And why the variable is static? How to return list of emp where salary > 1000 in java? A...
read moreAnswering questions related to Java development and architecture
Monolithic architecture is a traditional approach where all components of an application are tightly coupled into a single unit
Microservices architecture is a modern approach where an application is divided into smaller, loosely coupled services
Singleton class is a design pattern that restricts the instantiation of a class to a single object
Static variables in Singleton class are shared among all instances and ca...read more
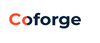
Asked in Coforge

Q. Singleton class in Java Why string is immutable in Java ? Difference between ArrayList & LinkedList What is IOC in Spring Find 3rd largest integer in integer array using streams in java. Java 8 nee features Sor...
read moreA set of interview questions for a Senior Java Developer position.
Singleton class in Java is a class that allows only one instance of itself to be created.
String is immutable in Java because it ensures thread safety and allows for efficient memory management.
ArrayList and LinkedList are both implementations of the List interface, but differ in their underlying data structure and performance characteristics.
IOC (Inversion of Control) in Spring is a design principle that allows...read more
Asked in Smartavya Analytica

Q. How are constructor or setter-based injections used in the traditional Spring framework?
Constructor or Setter based injection is used in Spring framework to inject dependencies into a bean.
Constructor injection involves passing dependencies as arguments to the constructor of a class.
Setter injection involves setting dependencies using setter methods.
Constructor injection is preferred for mandatory dependencies, while setter injection is used for optional dependencies.
Example: Constructor injection - public class MyClass { private Dependency dependency; public My...read more
Asked in Smartavya Analytica

Q. What is Metaspace and how was it improved in Java 11?
Meta space is a memory space in Java that stores metadata about classes and methods.
Meta space replaces the permanent generation in Java 8 and earlier versions.
In Java 11, meta space is improved by introducing a new flag called 'UseContainerSupport' which allows the JVM to use container-specific memory limits.
This improvement helps in better managing memory usage in containerized environments.
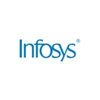
Asked in Infosys

Q. If I only have an OS and JDK, how would you set up a Spring-Boot project on my laptop?
To set up a Spring-Boot project with only OS and JDK, you need to install Maven and then create a new project using Spring Initializr.
Install Apache Maven to manage dependencies and build the project
Use Spring Initializr to generate a new Spring Boot project with required dependencies
Import the project into your IDE and start coding
Asked in Aera Software Technology

Q. Multiply ele E.g:[1,8,7,3,2] => 1 = 8*7*3*2 [336,42,48,112,168] If 1 then you should multiply all except 1 i.e 8*7*3*2=336
The question asks to multiply all elements in an array except for a given number.
Iterate through the array and calculate the product of all elements except the given number.
Use a variable to keep track of the product.
If the given number is 1, multiply all other elements.
Return the resulting array.
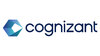
Asked in Cognizant

Q. What is the use of static and final keywords, and when would you use static methods?
Static methods can be accessed without creating an instance of the class, while final keyword makes the method unchangeable.
Static methods belong to the class itself, not to any specific instance
Final keyword ensures that the method cannot be overridden in subclasses
Static methods are commonly used for utility methods that do not require access to instance variables
Example: Math class in Java has static methods like Math.max() and Math.min()
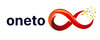
Asked in Onetoinfinite

Q. What is default server in springboot application? And how can we configure Other server? HashSet set = new HashSet(); for(int i=0; i<100; i++){ set.add(i); set.remove(i-1); } sop(set.size()); What is the o/p of...
read moreThe default server in a Spring Boot application is Tomcat. Other servers can be configured by adding dependencies and modifying the configuration.
The default server in Spring Boot is Tomcat, but other servers like Jetty or Undertow can be used.
To configure a different server, you need to exclude the default server dependency and add the desired server dependency in the pom.xml file.
You also need to modify the application.properties or application.yml file to specify the serve...read more
Asked in Caspex Corp

Q. Can we override or replace the embedded Tomcat server in Spring Boot?
Yes, we can override or replace the embedded tomcat server in Spring Boot.
You can override the embedded Tomcat server by excluding the Tomcat dependency in the pom.xml file and adding a different embedded server dependency like Jetty or Undertow.
You can also customize the embedded server configuration by creating a bean of type EmbeddedServletContainerCustomizer in a @Configuration class.
For example, to replace Tomcat with Jetty, exclude the Tomcat dependency and add the Jett...read more
Asked in Caspex Corp

Q. How does Amazon S3 provide high availability and low latency?
Amazon S3 provides high availability and low latency through data replication and global edge locations.
Amazon S3 replicates data across multiple availability zones within a region to ensure high availability.
Global edge locations cache frequently accessed data closer to users, reducing latency.
Amazon S3 uses a content delivery network (CDN) to deliver content quickly to users worldwide.
Asked in Caspex Corp

Q. What are Recovery Time Objective and Recovery Point Objective in AWS?
Recovery Time Objective (RTO) and Recovery Point Objective (RPO) are key metrics in disaster recovery planning in AWS.
RTO is the maximum acceptable downtime for restoring services after a disaster.
RPO is the maximum acceptable data loss in case of a disaster.
RTO and RPO help in determining the appropriate backup and recovery strategies.
For example, if RTO is 4 hours and RPO is 1 hour, it means services should be restored within 4 hours with a maximum data loss of 1 hour.
Asked in Caspex Corp

Q. What is Metaspace and how is it different from PermGen?
Meta space is a memory space in Java 8 and later versions that replaces PermGen for storing class metadata.
Meta space is a part of the native memory and is used to store class metadata, such as class structures and method information.
Unlike PermGen, meta space is not part of the Java heap and is not subject to the same memory limits.
Meta space automatically resizes based on the application's demand, unlike PermGen which had a fixed size.
Meta space can be monitored and tuned u...read more
Asked in Caspex Corp

Q. What is your understanding of stopping and terminating an EC2 instance?
Stopping an EC2 instance puts it into a stopped state, while terminating an EC2 instance permanently deletes it.
Stopping an EC2 instance preserves the data on the instance's EBS volumes
Terminating an EC2 instance deletes the instance and all associated data
Stopped instances can be started again, while terminated instances cannot be recovered
Stopping an instance does not incur charges, but terminating an instance does
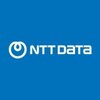
Asked in NTT Data

Q. What is one problem that interfaces solve that abstract classes do not?
Interfaces allow multiple inheritance, abstract classes do not.
Interfaces can be implemented by multiple classes, allowing for multiple inheritance.
Abstract classes can only be extended by one class, limiting inheritance.
Interfaces promote loose coupling and flexibility in design.
Abstract classes provide a common base implementation for subclasses.
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
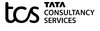
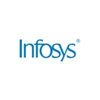
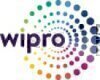
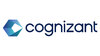
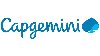
Top Interview Questions for Senior Java Developer Related Skills
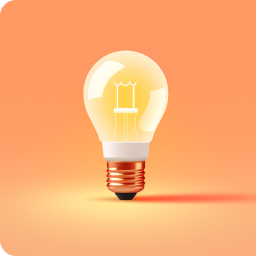
Calculate your in-hand salary
Confused about how your in-hand salary is calculated? Enter your annual salary (CTC) and get your in-hand salary
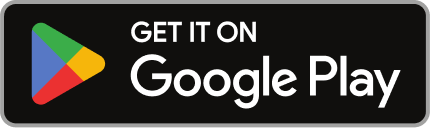
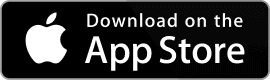
Reviews
Interviews
Salaries
Users
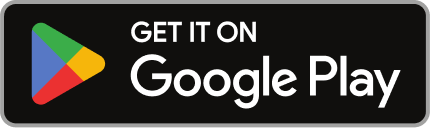
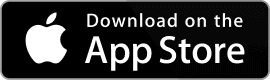