Python Software Developer
200+ Python Software Developer Interview Questions and Answers
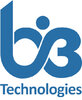
Asked in Bi3 Technologies

Q. Given a list of numbers, find all pairs of numbers whose sum equals a given target value. For example, given the list [1, 3, 5, 7, 6, 8] and a target of 9, the answer would be (8, 1) and (6, 3).
Iterate through the list and check for pairs that sum up to the target value.
Iterate through the list and for each element, check if the difference between the target and the element is present in a dictionary.
If the difference is present in the dictionary, then we have found a pair that sums up to the target.
If the difference is not present, add the current element to the dictionary.
Return the pairs that sum up to the target.
Asked in Aviva Global Services

Q. What is the list comprehension? Find the second largest in a list? L = [2,3,6,6,5] What is the lambda?
List comprehension is a concise way to create lists in Python. Lambda is an anonymous function. Find the second largest in a list.
List comprehension is a compact way to create lists in Python, using a single line of code.
Lambda functions are small, anonymous functions defined with the lambda keyword.
To find the second largest in a list, you can sort the list and then access the second last element.
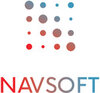
Asked in Navigators Software

Q. What is the difference between SQL and No-SQL databases?
SQL databases are relational databases with structured data and predefined schema, while No-SQL databases are non-relational databases with flexible schema and unstructured data.
SQL databases use structured query language for defining and manipulating data, while No-SQL databases use various query languages like JSON or XML.
SQL databases have predefined schema, which means the structure of the data must be defined before inserting data, while No-SQL databases have dynamic sch...read more
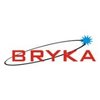
Asked in Bryka Electrosystems & Software

Q. What is the goal of ML in Robotics and Aircraft part design?
ML enhances robotics and aircraft design by optimizing performance, safety, and efficiency through data-driven insights.
Predictive maintenance: ML algorithms analyze data from sensors to predict equipment failures before they occur.
Design optimization: ML can optimize the shape and materials of aircraft components for better aerodynamics and weight reduction.
Autonomous navigation: ML enables robots and drones to navigate complex environments using computer vision and sensor f...read more
Asked in K DigitalCurry

Q. How can repeated characters be removed from an array?
Remove repeated characters from an array of strings by using set or dictionary to track occurrences.
Use a set to track seen characters: Iterate through each string and add unique characters to a new string.
Example: For ['apple', 'banana'], result is ['aple', 'ban'] after removing duplicates.
Use list comprehension: Create a new list with characters that haven't been seen yet.
Example: For ['hello', 'world'], result is ['helo', 'wrld'].
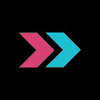
Asked in Techouts

Q. Explain the test codes you wrote and the logic behind them.
I implemented various algorithms to solve specific problems, focusing on efficiency and readability.
Used a binary search algorithm for efficient data retrieval in sorted arrays, reducing time complexity to O(log n).
Implemented a sorting function using quicksort, which is efficient for large datasets with an average time complexity of O(n log n).
Created a function to validate user input, ensuring data integrity and preventing errors in subsequent processing.
Utilized list compr...read more
Python Software Developer Jobs
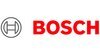
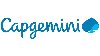
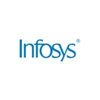
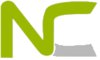
Asked in Northcorp Software

Q. If a number is divisible by 2 (number % 2 == 0), it is even; otherwise, it is odd.
A number is even if it is divisible by 2, otherwise it is odd.
Use the modulo operator (%) to check if a number is divisible by 2.
If number % 2 == 0, then the number is even.
For example, 4 % 2 == 0, so 4 is even; 5 % 2 != 0, so 5 is odd.
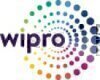
Asked in Wipro

Q. What is Python? What are the benefits of using Python What is a dynamically typed language? What is an Interpreted language? What is PEP 8 and why is it important?
Python is a high-level, interpreted, dynamically typed programming language known for its simplicity and readability.
Python is a high-level programming language used for web development, data analysis, artificial intelligence, and more.
Benefits of using Python include its simplicity, readability, extensive libraries, and community support.
A dynamically typed language is one where variable types are determined at runtime, allowing for flexibility but potentially leading to err...read more
Share interview questions and help millions of jobseekers 🌟
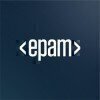
Asked in EPAM Systems

Q. What are decorators in Python?
Decorators in Python are functions that modify the behavior of other functions or methods.
Decorators are denoted by the @ symbol followed by the decorator function name.
They are commonly used for adding functionality to existing functions without modifying their code.
Decorators can be used for logging, timing, authentication, and more.
Example: @staticmethod decorator in Python is used to define a method that is not bound to a class instance.

Asked in MNC AUTOMATION

Q. How many types of comments are there in Python?
There are two types of Python comments: single-line comments and multi-line comments.
Single-line comments start with a hash symbol (#) and continue until the end of the line. Example: # This is a single-line comment
Multi-line comments are enclosed within triple quotes (''' or "). Example: ''' This is a multi-line comment '''
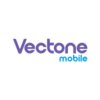
Asked in Vectone Mobile

Q. Write a program to reverse a string without using slicing.
Reverse a string using a loop instead of slicing to demonstrate understanding of string manipulation in Python.
Use a loop to iterate through the string from the last character to the first.
Initialize an empty string to store the reversed result.
Concatenate each character to the result string during the loop.
Example: For input 'hello', the output should be 'olleh'.
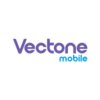
Asked in Vectone Mobile

Q. What is inheritance in Python, and what are its types?
Inheritance in Python allows a class to inherit attributes and methods from another class.
Types of inheritance in Python include single inheritance, multiple inheritance, and multilevel inheritance.
Single inheritance: a class inherits from only one base class.
Multiple inheritance: a class inherits from multiple base classes.
Multilevel inheritance: a class inherits from a derived class, which in turn inherits from another class.
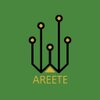
Asked in Areete Business Solutions

Q. How do you approach problem-solving in software development projects?
I approach problem-solving methodically, breaking down issues and collaborating with others to find effective solutions.
Define the problem clearly: Understand the requirements and constraints before diving into coding.
Break it down: Decompose the problem into smaller, manageable tasks. For example, if building a web app, start with user authentication.
Research and gather information: Look for existing solutions or libraries that can help. For instance, using Django for rapid ...read more
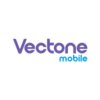
Asked in Vectone Mobile

Q. Given a string, extract the vowels from it.
Extracting vowels from a string involves identifying and collecting characters that are vowels (a, e, i, o, u).
Use a list comprehension to iterate through the string and check if each character is a vowel.
Example: For the string 'hello', the vowels are ['e', 'o'].
Consider both uppercase and lowercase vowels for comprehensive extraction.
Example: For the string 'Python', the vowels are ['o'].
Return the result as a list of vowels.
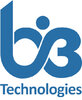
Asked in Bi3 Technologies

Q. Sort the given numbers in a list and count the number of inversions for each number
Sort a list of numbers and count inversions for each number
Sort the list of numbers using a sorting algorithm like merge sort or bubble sort
For each number, count the number of elements that are greater than it but appear before it in the sorted list
Return the list of numbers with their corresponding inversion counts
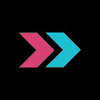
Asked in Techouts

Q. Explain OOPS concepts by writing Python code examples for class, object, inheritance, polymorphism, and abstraction.
OOP in Python includes concepts like classes, objects, inheritance, polymorphism, and abstraction for structured programming.
Class: A blueprint for creating objects. Example: class Dog: pass
Object: An instance of a class. Example: my_dog = Dog()
Inheritance: A way to form new classes using classes that have already been defined. Example: class Puppy(Dog): pass
Polymorphism: The ability to present the same interface for different data types. Example: def make_sound(animal): anim...read more
Asked in Droidal

Q. What are the different data types available in Python?
Python supports various data types, including numeric, sequence, mapping, set, and boolean types, each serving different purposes.
Numeric Types: Includes integers (e.g., 5), floats (e.g., 3.14), and complex numbers (e.g., 2 + 3j).
Sequence Types: Lists (e.g., [1, 2, 3]), tuples (e.g., (1, 2, 3)), and strings (e.g., 'Hello') are used to store ordered collections.
Mapping Type: The dictionary (e.g., {'key': 'value'}) is a collection of key-value pairs, allowing for fast lookups.
S...read more
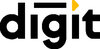
Asked in Digit Insurance

Q. What are the different date structures available in Python?
Python offers various date structures for handling dates and times, including datetime, date, time, and timedelta classes.
datetime: The main class for manipulating dates and times, allowing for operations like addition and subtraction. Example: datetime.now()
date: Represents a date (year, month, day) without time. Example: date(2023, 10, 5)
time: Represents a time (hour, minute, second, microsecond) without a date. Example: time(14, 30, 0)
timedelta: Represents the difference b...read more
Asked in Corestack

Q. How is memory management done in Python?
Python uses automatic memory management through a combination of reference counting and garbage collection.
Python uses reference counting to keep track of the number of references to an object.
When the reference count reaches zero, the object is immediately deallocated.
Python also employs a garbage collector to handle cyclic references and objects with circular dependencies.
The garbage collector identifies and collects unreachable objects, freeing up memory.
Python's garbage c...read more
Asked in Team Engine

Q. What is monkey patching?
Monkey patching is a technique in Python where existing code or modules are modified at runtime.
Monkey patching involves dynamically changing or extending the behavior of a module or class at runtime.
It can be used to fix bugs, add new functionality, or temporarily change the behavior of existing code.
Monkey patching can be a powerful tool but should be used carefully to avoid unexpected side effects.
Example: Adding a new method to an existing class by directly modifying the ...read more
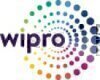
Asked in Wipro

Q. What is Python? What are the benefits of using Python What is a dynamically typed language?
Python is a high-level programming language known for its simplicity and readability. It is dynamically typed, allowing for flexibility and faster development.
Python is a high-level programming language used for web development, data analysis, artificial intelligence, and more.
It is known for its simplicity and readability, making it easier for developers to write and maintain code.
Python is dynamically typed, meaning variables do not need to be declared with a specific data ...read more
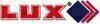
Asked in LUX Industries

Q. What are the data types in Python?
Data types in Python define the type of data that a variable can hold.
Python has several built-in data types such as int, float, str, list, tuple, dict, set, bool, etc.
Each data type has specific characteristics and operations that can be performed on it.
Examples: int for integers, float for floating-point numbers, str for strings, list for lists of elements.
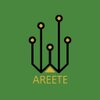
Asked in Areete Business Solutions

Q. Can you describe your experience with Python and how it relates to the role requirements?
I have extensive experience in Python, focusing on web development, data analysis, and automation, aligning well with the role's needs.
Developed RESTful APIs using Flask and Django, enhancing application performance and scalability.
Implemented data analysis scripts with Pandas and NumPy, leading to actionable insights for business decisions.
Automated repetitive tasks using Python scripts, saving the team significant time and reducing errors.
Contributed to open-source Python p...read more
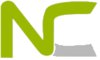
Asked in Northcorp Software

Q. Write a short program to check if a number is even or odd?
A simple program to determine if a number is even or odd.
Use the modulo operator (%) to check if the number divided by 2 leaves a remainder
If the remainder is 0, the number is even. Otherwise, it is odd
Example: num = 6, 6 % 2 = 0 (even); num = 7, 7 % 2 = 1 (odd)
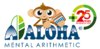
Asked in ALOHA

Q. Write a Python program to check if a string is a palindrome.
A program to check if a given string is a palindrome in Python.
Create a function that takes a string as input.
Use string slicing to reverse the input string.
Compare the reversed string with the original string to check for palindrome.
Return True if the string is a palindrome, False otherwise.
Asked in Bigworks

Q. You can handle the pressure? You can do the work under pressure
Yes, I can handle pressure and work effectively under tight deadlines.
I thrive in high-pressure situations and have a proven track record of delivering quality work under tight deadlines.
I prioritize tasks effectively and remain calm and focused when faced with challenging situations.
I have experience working on projects with tight deadlines, such as developing a critical feature before a product launch.
I am able to adapt quickly to changing priorities and can efficiently man...read more
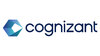
Asked in Cognizant

Q. What is a one-line function?
A one-line function is a function written in a single line of code, typically used for simple operations.
One-line functions are concise and easy to read, often used for simple tasks like mathematical operations or string manipulation.
They are commonly used in lambda functions in Python.
Example: lambda x: x**2
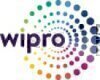
Asked in Wipro

Q. What is python How to do develop the program Which code are be use
Python is a high-level programming language known for its simplicity and readability.
Python is developed using an interpreter, which allows for easy and quick development of programs.
Python uses a syntax that emphasizes code readability and simplicity.
Commonly used libraries in Python include NumPy, Pandas, and Matplotlib for data analysis and visualization.
Python code is written in plain text files with a .py extension.
Python programs can be run using the command line or an ...read more
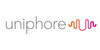
Asked in Uniphore Software Systems

Q. What are variables and data types in Python?
Variables store data values, while data types define the kind of data stored in Python.
Variables are created by assigning a value: `x = 5`.
Common data types include: `int`, `float`, `str`, `list`, `tuple`, `dict`, `set`.
Example of a string: `name = 'Alice'`.
Example of a list: `numbers = [1, 2, 3]`.
Data types can be checked using the `type()` function: `type(x)` returns `<class 'int'>`.
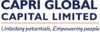
Asked in Capri Global Capital

Q. What is the difference between unit testing and integration testing?
Unit testing tests individual units of code while integration testing tests how multiple units work together.
Unit testing is done by developers and focuses on testing individual functions or methods.
Integration testing is done by testers and focuses on testing how different modules or components work together.
Unit tests are usually automated and run frequently during development.
Integration tests are usually manual and run less frequently.
Unit tests are faster and easier to d...read more
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
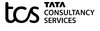
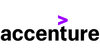
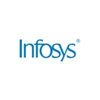
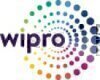
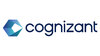
Top Interview Questions for Python Software Developer Related Skills
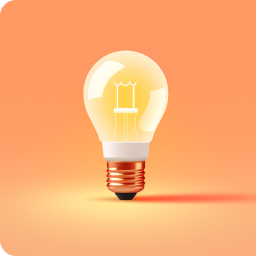
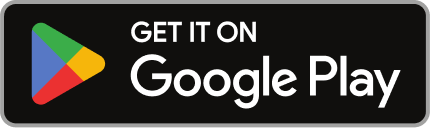
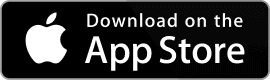
Reviews
Interviews
Salaries
Users
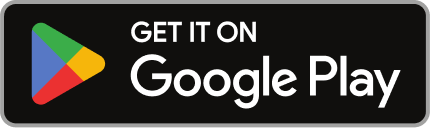
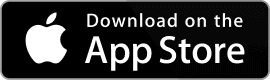