Programmer Analyst Trainee
200+ Programmer Analyst Trainee Interview Questions and Answers
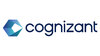
Asked in Cognizant

Q. How can you add two numbers without using the + operator?
Adding two numbers without using + operator
Use bitwise operators like XOR, AND, and left shift to perform addition
Addition can be done recursively until there is no carry left
Example: 5 + 3 = 8 can be done as 5 XOR 3 = 6, 5 AND 3 = 1, 1 left shift 1 = 2, 6 + 2 = 8
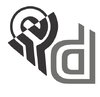
Asked in Qedtek

Q. How do you implement JavaScript in HTML code?
JavaScript can be implemented in HTML code using <script> tags or by linking an external JavaScript file.
Use <script> tags within the <head> or <body> section of the HTML document to write JavaScript code directly in the HTML file.
Link an external JavaScript file using the <script> tag with the src attribute pointing to the file location.
Ensure that the JavaScript code is enclosed within <script> tags to indicate to the browser that it is JavaScript code.
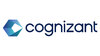
Asked in Cognizant

Q. Write a function to swap two numbers without using pointers.
Code to swap 2 numbers without using pointer, using function
Create a function that takes two integer parameters
Inside the function, swap the values of the parameters using a temporary variable
Return the swapped values
Call the function and pass the two numbers to be swapped as arguments
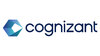
Asked in Cognizant

Q. What will you do if the technology you are working on becomes obsolete?
I will adapt and learn new technologies to stay relevant in the industry.
Research and analyze emerging technologies
Attend training and workshops to learn new skills
Collaborate with colleagues to share knowledge and expertise
Update resume and portfolio to showcase new skills
Stay proactive and open-minded towards change
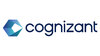
Asked in Cognizant

Q. what is form factor, difference between generator and alternator
Form factor is the ratio of RMS value to average value of a waveform. Generator converts mechanical energy to electrical energy while alternator converts electrical energy to mechanical energy.
Form factor is a measure of waveform distortion
Generator produces DC or AC power by converting mechanical energy
Alternator produces AC power by converting mechanical energy
Generator has a commutator while alternator has slip rings
Examples of generators are hydroelectric power plants and...read more
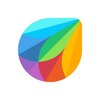
Asked in Freshworks

Q. Do you know C Programming?
Yes, I know C Programming.
I have experience in writing programs in C language.
I am familiar with the syntax and structure of C language.
I have worked on projects using C language, such as developing a simple calculator program.
I am comfortable with concepts such as pointers, arrays, and structures in C language.
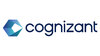
Asked in Cognizant

Q. Given a number n, write a function to print all prime factors of n.
A prime number is a number that is only divisible by 1 and itself.
Loop through numbers 2 to n-1 and check if n is divisible by any of them
Use the modulo operator to check for divisibility
Optimization: only check up to the square root of n
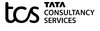
Asked in TCS

Q. What is inheritance in Java?
Inheritance is a mechanism in Java where a class acquires the properties and methods of another class.
It allows for code reusability and promotes a hierarchical structure of classes.
The subclass inherits all the non-private members (fields, methods) of the superclass.
The keyword 'extends' is used to create a subclass.
Example: class Dog extends Animal { ... }
Multiple inheritance is not allowed in Java.
Share interview questions and help millions of jobseekers 🌟
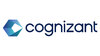
Asked in Cognizant

Q. Write a program to print the prime numbers between 1 and n.
Program to print prime numbers between 1 to n.
Iterate from 2 to n and check if each number is prime
A number is prime if it is only divisible by 1 and itself
Use a nested loop to check if the number is divisible by any number between 2 and itself-1
If not divisible, print the number as prime
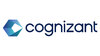
Asked in Cognizant

Q. Explain any two OOP concepts with real-life examples.
Encapsulation and Inheritance are two OOPs concepts with real-life examples.
Encapsulation: Wrapping data and methods into a single unit. Example: A car's engine is encapsulated as a single unit, and the driver only interacts with it through the dashboard controls.
Inheritance: Creating a new class from an existing class. Example: A sports car is a subclass of a car, inheriting all the properties and methods of a car but with additional features specific to sports cars.
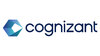
Asked in Cognizant

Q. Explain linked list,stack and queue concept
Linked list, stack, and queue are data structures used to store and manipulate data.
Linked list is a collection of nodes where each node points to the next node. Example: Singly linked list, Doubly linked list.
Stack is a LIFO (Last In First Out) data structure. Example: Undo/Redo functionality in text editors.
Queue is a FIFO (First In First Out) data structure. Example: Print queue in a printer.
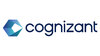
Asked in Cognizant

Q. What is the difference between SQL UNION and UNION ALL?
SQL UNION combines and removes duplicates, UNION ALL combines without removing duplicates.
UNION merges the results of two or more SELECT statements into a single result set
UNION ALL includes all the rows from each SELECT statement, including duplicates
UNION removes duplicates from the result set, while UNION ALL does not
UNION requires the same number of columns in all SELECT statements, while UNION ALL does not have this requirement
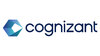
Asked in Cognizant

Q. What is an Integrated Circuit?
An Integrated Circuit is a miniaturized electronic circuit consisting of interconnected semiconductor devices.
ICs are used in almost all electronic devices
They can be analog, digital or mixed-signal
ICs can be classified as SSI, MSI, LSI or VLSI based on the number of transistors they contain
Examples of ICs include microprocessors, memory chips, and amplifiers
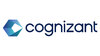
Asked in Cognizant

Q. What is dynamic memory allocation and what are its types?
Dynamic memory allocation is the process of allocating memory during runtime.
It allows programs to allocate memory as needed, rather than at compile time.
Types include malloc(), calloc(), realloc(), and free().
malloc() allocates a block of memory of specified size.
calloc() allocates a block of memory for an array of elements, initializing them to zero.
realloc() changes the size of a previously allocated block of memory.
free() deallocates the memory previously allocated by mal...read more
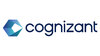
Asked in Cognizant

Q. Why is Python your preferred programming language?
Python is my preferred language due to its simplicity, versatility, and strong community support, making it ideal for various projects.
Easy to learn and read: Python's syntax is clear and concise, making it accessible for beginners. For example, a simple 'print' statement is intuitive.
Versatile applications: Python is used in web development (Django, Flask), data analysis (Pandas, NumPy), and machine learning (TensorFlow, scikit-learn).
Strong community support: A vast number ...read more
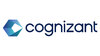
Asked in Cognizant

Q. Explain the OOP concepts in detail with real-world examples.
Object-oriented programming concepts with real-world examples.
Encapsulation: Hiding implementation details of a class. Example: A car's engine is encapsulated.
Inheritance: A class inheriting properties and methods from another class. Example: A dog is an animal.
Polymorphism: Ability of an object to take many forms. Example: A shape can be a circle, square, or triangle.
Abstraction: Focusing on essential features of an object. Example: A TV remote has buttons for essential func...read more
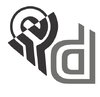
Asked in Qedtek

Q. What is CSS and How we can add with HTML?
CSS is a styling language used to control the look and feel of a website. It can be added to HTML using the <style> tag or external CSS files.
CSS stands for Cascading Style Sheets
It is used to define the layout, colors, fonts, and other visual aspects of a website
CSS can be added to HTML using the <style> tag within the <head> section or by linking an external CSS file using the <link> tag
Inline CSS can also be added directly to HTML elements using the style attribute
Example:...read more
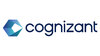
Asked in Cognizant

Q. What is shortest path algorithm
Shortest path algorithm is used to find the shortest path between two points in a graph.
It is commonly used in GPS navigation systems.
Dijkstra's algorithm and Bellman-Ford algorithm are two popular shortest path algorithms.
The algorithm works by exploring all possible paths and selecting the one with the lowest cost.
The cost can be distance, time, or any other metric depending on the problem.
Shortest path algorithms are used in various fields such as transportation, network r...read more
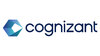
Asked in Cognizant

Q. Write code to print all the even number indexes from an array.
Print all even number indexes from an array of strings.
Iterate through the array and check if the index is even using modulo operator.
Print the element at even indexes.
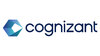
Asked in Cognizant

Q. Explain the concepts of OOP with real-life examples.
OOPs concepts like encapsulation, inheritance, and polymorphism can be understood through relatable real-life examples.
Encapsulation: A car is a good example; it has a complex engine but the driver only interacts with the steering wheel and pedals.
Inheritance: A child inherits traits from parents; in programming, a subclass inherits properties and methods from a superclass.
Polymorphism: A person can be a teacher, a parent, or a friend; in OOP, a method can perform different f...read more
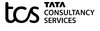
Asked in TCS

Q. What is a primary key and a foreign key?
Primary key uniquely identifies a record in a table, while foreign key refers to a field in another table.
Primary key is used to enforce data integrity and ensure uniqueness of records
Foreign key is used to establish a relationship between two tables
Example: CustomerID in Orders table is a foreign key that references the Customer table's primary key
Foreign key constraints ensure referential integrity
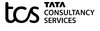
Asked in TCS

Q. What is object-oriented programming?
Object oriented programming language is a type of programming language that uses objects and classes to organize code.
Uses objects and classes to model real-world entities
Encapsulates data and behavior within objects
Supports inheritance, polymorphism, and encapsulation
Examples include Java, C++, Python
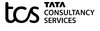
Asked in TCS

Q. What is the difference between C and C++?
C++ is an extension of C with object-oriented programming features.
C++ supports object-oriented programming while C does not.
C++ has classes and templates while C does not.
C++ has better support for exception handling than C.
C++ has a standard library that includes many useful functions.
C++ is more complex than C and can be harder to learn.
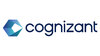
Asked in Cognizant

Q. What is an example of a Python IDE?
PyCharm is a popular Python IDE.
PyCharm is a powerful and feature-rich IDE for Python development.
It provides intelligent code completion, debugging tools, and version control integration.
Other examples of Python IDEs include Visual Studio Code, Spyder, and Jupyter Notebook.
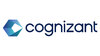
Asked in Cognizant

Q. Write a program to check for palindrome.
A program to check if a given string is a palindrome or not.
Remove all spaces and convert the string to lowercase for accurate results.
Compare the first and last characters of the string, then move towards the center.
If all characters match, the string is a palindrome.
If any character doesn't match, the string is not a palindrome.
Asked in Fungurukul Edu Systems

Q. How do you prepare for a difficult technical interview?
Prepare thoroughly, stay calm, and approach problems methodically during tough technical interviews.
Research common interview questions and practice coding problems on platforms like LeetCode or HackerRank.
Break down complex problems into smaller, manageable parts. For example, if asked to sort an array, discuss different sorting algorithms.
Communicate your thought process clearly. Explain your reasoning as you work through a problem, which helps interviewers understand your ...read more
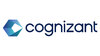
Asked in Cognizant

Q. What is inheritance and why do we use it?
Inheritance is a mechanism in object-oriented programming where a new class is created by inheriting properties of an existing class.
Inheritance allows code reusability and saves time and effort in writing new code.
The existing class is called the parent or base class, and the new class is called the child or derived class.
The child class inherits all the properties and methods of the parent class and can also add new properties and methods.
For example, a class 'Vehicle' can ...read more
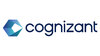
Asked in Cognizant

Q. Why do we use normalization?
Normalization is done to eliminate data redundancy and improve data integrity.
To avoid data duplication
To reduce storage space
To improve data consistency
To prevent update anomalies
To simplify queries
To follow database design principles
To ensure data accuracy and reliability
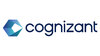
Asked in Cognizant

Q. Why do we use object-oriented programming?
Object oriented programming allows for modular, reusable, and maintainable code.
Encapsulation allows for data hiding and protects code from external interference.
Inheritance allows for code reuse and promotes consistency.
Polymorphism allows for flexibility and extensibility.
Objects can be easily tested and debugged.
Object oriented programming promotes code organization and readability.
Examples include Java, Python, and C++.
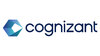
Asked in Cognizant

Q. Write a basic query to fetch data from a table.
Basic query to fetch data from a table in SQL
Use SELECT statement to fetch data
Specify the columns you want to retrieve after SELECT
Specify the table name after FROM
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
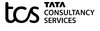
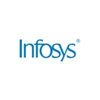
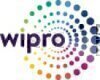
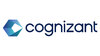
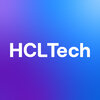
Top Interview Questions for Programmer Analyst Trainee Related Skills
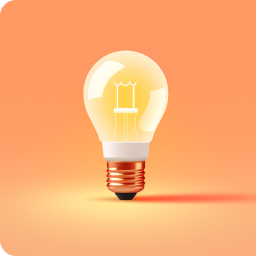
Calculate your in-hand salary
Confused about how your in-hand salary is calculated? Enter your annual salary (CTC) and get your in-hand salary
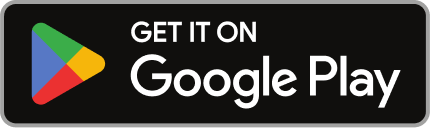
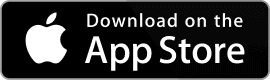
Reviews
Interviews
Salaries
Users
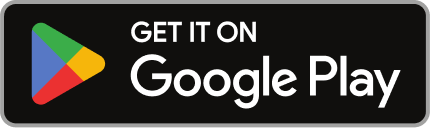
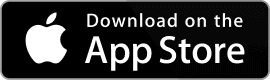