Mts1
30+ Mts1 Interview Questions and Answers
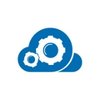
Asked in 42Gears Mobility Systems

Q. Expression Equality Checker
Given two strings representing expressions in variables, determine if they are equivalent. Return 'YES' if the expressions are identical and 'NO' if they are different. Each expressi...read more
Check if two expressions are equivalent by evaluating them with different variable assignments.
Parse the expressions to evaluate them with different variable assignments.
Use a stack to keep track of operands and operators while evaluating the expressions.
Compare the results of both expressions to determine if they are equivalent.
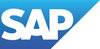
Asked in SAP

Q. Minimum Number of Platforms Problem
Your task is to determine the minimum number of platforms required at a railway station so that no train has to wait.
Explanation:
Given two arrays:
AT
- representing the ar...read more
Determine the minimum number of platforms needed at a railway station so that no train has to wait.
Sort the arrival and departure times arrays in ascending order.
Initialize two pointers for arrival and departure times, and a variable to keep track of the maximum number of platforms needed.
Increment the platform count when a train arrives and decrement when a train departs.
Update the maximum platform count as needed.
Return the maximum platform count at the end.
Mts1 Interview Questions and Answers for Freshers
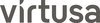
Asked in Virtusa Consulting Services

Number and Digits Problem Statement
You are provided with a positive integer N
. Your task is to identify all numbers such that the sum of the number and its digits equals N
.
Example:
Input:
N = 21
Output:
[15]
Identify numbers whose sum with digits equals given integer N.
Iterate through numbers from 1 to N and check if sum of number and its digits equals N.
Use a helper function to calculate sum of digits for a given number.
Return -1 if no such number exists for a test case.
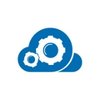
Asked in 42Gears Mobility Systems

Q. Matrix Element Cube Sum Problem
For a given M x N sized 2D array 'MATRIX', find and return the value of (i * i + j * j) for elements where the sum of the cubes of its digits equals the element itself. Here, 'i'...read more
Find and return the value of (i * i + j * j) for elements in a 2D array where the sum of the cubes of its digits equals the element itself.
Iterate through the 2D array and check if the sum of the cubes of the digits equals the element itself.
Calculate (i * i + j * j) for elements that satisfy the condition.
Return the calculated values as output.
If no element satisfies the condition, return -1.
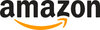
Asked in Amazon

Q. Longest Increasing Subsequence Problem Statement
Given an array of integers with 'N' elements, determine the length of the longest subsequence where each element is greater than the previous element. This subse...read more
Find the length of the longest strictly increasing subsequence in an array of integers.
Use dynamic programming to solve this problem efficiently.
Initialize an array to store the length of the longest increasing subsequence ending at each index.
Iterate through the array and update the length of the longest increasing subsequence for each element.
Return the maximum value in the array as the result.
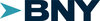
Asked in BNY

Q. Cycle Detection in a Singly Linked List
Determine if a given singly linked list of integers forms a cycle or not.
A cycle in a linked list occurs when a node's next
points back to a previous node in the list. T...read more
Detect if a singly linked list forms a cycle by checking if a node's next points back to a previous node.
Traverse the linked list using two pointers, one moving one step at a time and the other moving two steps at a time.
If the two pointers meet at any point, it indicates the presence of a cycle in the linked list.
To optimize, use Floyd's cycle detection algorithm for O(N) time complexity and O(1) space complexity.
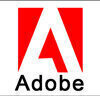
Asked in Adobe

Q. Maximum Sum Problem Statement
You are given an array ARR
of N integers. Your task is to perform operations on this array until it becomes empty, and maximize the sum of selected elements. In each operation, sel...read more
The task is to maximize the sum of selected elements from an array by following specific rules.
Select elements strategically to maximize the sum
Remove occurrences of selected element, and its neighbors
Repeat the process until the array becomes empty
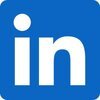
Asked in LinkedIn

Q. Split the String Problem Statement
You are given a string str
consisting of N
lowercase alphabets. Your task is to determine if it is possible to divide the string into three non-empty substrings such that one ...read more
Determine if it is possible to split a string into three non-empty substrings where one is a substring of the other two.
Check if any substring of the string is a substring of the other two substrings.
Iterate through all possible divisions of the string into three non-empty substrings.
Use two pointers to find all possible substrings efficiently.
Share interview questions and help millions of jobseekers 🌟
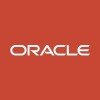
Asked in Oracle

Q. Preorder Traversal to BST Problem Statement
Given an array or list PREORDER
representing the preorder traversal of a Binary Search Tree (BST) with N
nodes, construct the BST which matches the given preorder tra...read more
Given a preorder traversal of a BST, construct the BST and print its inorder traversal.
Parse the input to get the number of test cases and preorder traversal for each case
Construct the BST using the preorder traversal by recursively building the tree
Print the inorder traversal of the constructed BST for each test case
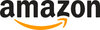
Asked in Amazon

Q. Loot Houses Problem Statement
A thief is planning to steal from several houses along a street. Each house has a certain amount of money stashed. However, the thief cannot loot two adjacent houses. Determine the...read more
Determine the maximum amount of money a thief can steal from houses without looting two consecutive houses.
Create an array 'dp' to store the maximum money that can be stolen up to the i-th house.
Iterate through the houses and update 'dp' based on whether the current house is stolen or not.
Return the maximum value in 'dp' as the answer.
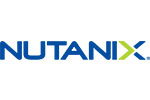
Asked in Nutanix

Q. Ninja and Stack of Boxes Problem
Help Ninja to create the tallest stack possible using given 3-D boxes with dimensions Length 'L', Breadth 'B', and Height 'H'. Each box can be rotated to use any side as the bas...read more
Implement a function to find the maximum possible height of a stack of boxes given their dimensions.
Create all possible rotations of each box to consider all orientations for stacking
Sort the boxes based on their base dimensions in non-increasing order
Use dynamic programming to find the maximum height of the stack
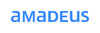
Asked in Amadeus

Q. Rearrange The Array Problem Statement
You are given an array/list 'NUM' of integers. Rearrange the elements of 'NUM' such that no two adjacent elements are the same in the rearranged array.
Example:
Input:
NUM[...read more
The task is to rearrange an array such that no two adjacent elements are the same.
Iterate through the array and check if any adjacent elements are the same.
If adjacent elements are the same, swap one of them with a different element.
Return 'YES' if a valid rearrangement is possible, 'NO' otherwise.
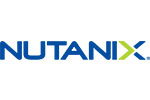
Asked in Nutanix

Q. Reverse Edges Problem Statement
You are given a directed graph with ‘N’ nodes and ‘M’ edges, along with two specific nodes, ‘A’ and ‘B’. Your task is to find the minimum number of operations required to create ...read more
The task is to find the minimum number of operations required to create a valid path from node A to node B by reversing edges in a directed graph.
Iterate through the graph to find the shortest path from node A to node B.
Use a graph traversal algorithm like BFS or DFS to explore possible paths.
Track the number of edge reversals needed to reach node B from node A.
Consider edge directions and reverse them as needed to create a valid path.
Return the minimum number of edge reversa...read more

Asked in JPMorgan Chase & Co.

Q. Longest Increasing Path in Matrix Problem Statement
Given a 2-D matrix mat
with 'N' rows and 'M' columns, where each element at position (i, j) is mat[i][j]
, determine the length of the longest increasing path ...read more
The task is to find the length of the longest increasing path in a matrix starting from a given cell.
Create a recursive function to explore all possible paths from the starting cell, keeping track of the length of each path.
Use dynamic programming to avoid redundant calculations and optimize the solution.
At each cell, check if moving to the right or down is possible and leads to an increasing path.
Update the length of the longest increasing path found so far as you explore di...read more
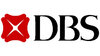
Asked in DBS Bank

Q. Query and Matrix Problem Statement
You are given a binary matrix with 'M' rows and 'N' columns, initially consisting of all 0s. You will receive 'Q' queries, which can be of four types:
Query 1: 1 R index
Query ...read more
Implement a function to process queries on a binary matrix by flipping elements and counting zeros in rows/columns.
Create a binary matrix with all elements initialized to 0.
Process queries of type 1 by flipping elements in the specified row/column.
Process queries of type 2 by counting the number of zeros in the specified row/column.
Return the count of zeros for type 2 queries.
Ensure to handle the constraints provided in the problem statement.
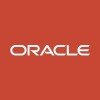
Asked in Oracle

Q. Check if a Number is Binary
Determine if a given string of integers bin
represents a valid binary number. Return 'true'
if the string represents a valid binary number, otherwise return 'false'
. A binary number ...read more
Check if a given string represents a valid binary number composed of 0s and 1s.
Iterate through each character in the string and check if it is either '0' or '1'.
If any character is not '0' or '1', return 'false'.
Return 'true' if all characters are '0' or '1'.
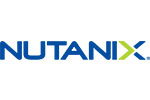
Asked in Nutanix

Q. Lexicographic Permutation Rank Problem Statement
Given a distinct string, determine the lexicographic permutation rank of the string.
Example:
Input:
T = 2
S = "abc"
S = "bac"
Output:
1
2
Explanation:
For the firs...read more
The problem involves determining the lexicographic permutation rank of a distinct string.
Iterate through all permutations of the string and compare with the given string to determine the rank.
Use a recursive function to generate all permutations of the string.
Keep track of the count of permutations smaller than the given string to determine the rank.
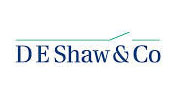
Asked in DE Shaw

Q. Union and Intersection of Two Arrays
Given two arrays 'A' and 'B' of size 'N' and 'M' respectively, both sorted in non-decreasing order, find the intersection of these two arrays.
The intersection of two arrays...read more
Yes, we can solve this problem using a time complexity of O(max(N, M)) by using a two-pointer approach.
Use two pointers to iterate through both arrays simultaneously.
Compare the elements at the pointers and move the pointers accordingly to find the intersection.
This approach ensures linear time complexity based on the size of the larger array.
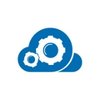
Asked in 42Gears Mobility Systems

Q. Convert Binary Tree to Mirror Tree
Convert a given binary tree into its mirror tree, where the left and right children of all non-leaf nodes are interchanged.
Input:
An integer ‘T’ denoting the number of test c...read more
Convert a binary tree into its mirror tree by interchanging left and right children of non-leaf nodes.
Traverse the tree in a recursive manner and swap the left and right children of each node.
Modify the binary tree in place to get the mirror, without creating a new tree.
Use a temporary variable to swap the left and right children of each node.
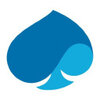
Asked in Capgemini Engineering

Q. Level Order Traversal of Binary Tree
Given a binary tree of integers, return its level order traversal.
Input:
The first line contains an integer 'T' which represents the number of test cases. For each test cas...read more
Level Order Traversal of Binary Tree returns nodes in level order traversal.
Perform a level order traversal of the binary tree starting from the root node.
Visit nodes level by level, printing them in the order they are encountered.
Use a queue data structure to keep track of nodes at each level.
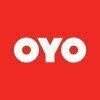
Asked in Oyo Rooms

Q. Top View of Binary Tree Problem Statement
Given a binary tree, your task is to print the Top View of the Binary Tree. The Top View is the set of nodes visible when the tree is viewed from the top. Please ensure...read more
The task is to print the Top View of a Binary Tree, which is the set of nodes visible when viewed from the top, in left to right order.
Traverse the binary tree in level order and maintain a map to store the horizontal distance of each node from the root.
For each node, if the horizontal distance is not already in the map, add the node's value to the result.
Print the values from the map in ascending order of horizontal distance to get the Top View of the Binary Tree.
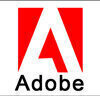
Asked in Adobe

Q. Ways to Reach Nth Stair
Given the number of stairs, initially at the 0th stair, you need to reach the Nth stair. Each time, you can either climb one step or two steps. Determine the number of distinct ways to c...read more
Calculate the number of distinct ways to climb N stairs by either taking one or two steps at a time.
Use dynamic programming to keep track of the number of ways to reach each stair
The number of ways to reach a stair is the sum of the number of ways to reach the previous two stairs
Return the result modulo 10^9+7
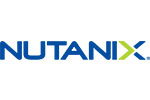
Asked in Nutanix

Designed a scalable system for Pastebin with various requirements.
Implemented a distributed system architecture to handle high traffic and ensure reliability.
Used load balancing techniques to evenly distribute incoming requests across multiple servers.
Implemented data sharding to partition data across multiple databases for efficient storage and retrieval.
Utilized caching mechanisms to improve performance and reduce latency.
Implemented access control mechanisms to ensure data...read more
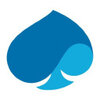
Asked in Capgemini Engineering

Q. Reverse a String Problem Statement
Given a string STR
containing characters from [a-z], [A-Z], [0-9], and special characters, determine the reverse of the string.
Input:
The input starts with a single integer '...read more
Reverse a given string containing characters from [a-z], [A-Z], [0-9], and special characters.
Iterate through each character in the string and append them in reverse order to a new string
Use built-in functions like reverse() or slice() to reverse the string
Handle special characters and numbers along with alphabets
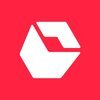
Asked in Snapdeal

Q. Inorder Traversal of Binary Tree Without Recursion
Given a Binary Tree consisting of 'N' nodes with integer values, your task is to perform an In-Order traversal of the tree without using recursion.
Input:
The ...read more
Implement in-order traversal of a binary tree without recursion.
Use a stack to simulate the recursive in-order traversal process.
Start with the root node and keep traversing left until reaching a null node, pushing nodes onto the stack.
Pop nodes from the stack, print the value, and move to the right child if it exists.
Continue this process until the stack is empty and all nodes have been visited.
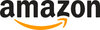
Asked in Amazon

Q. Left View of a Binary Tree Problem Statement
Given a binary tree, your task is to print the left view of the tree.
Example:
Input:
The input will be in level order form, with node values separated by a space. U...read more
The task is to print the left view of a binary tree given in level order form.
Traverse the tree level by level and print the first node of each level (leftmost node).
Use a queue to keep track of nodes at each level.
Consider null nodes as well while traversing the tree.
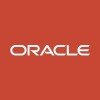
Asked in Oracle

The result of adding two binary numbers in a 64-bit and a 32-bit operating system will differ due to the different number of bits used for calculations.
In a 64-bit operating system, the result will be more accurate and can handle larger numbers compared to a 32-bit system.
Overflow may occur in a 32-bit system when adding large binary numbers, leading to incorrect results.
Example: Adding 1111 (15 in decimal) and 1111 (15 in decimal) in a 32-bit system may result in overflow an...read more
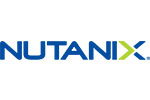
Asked in Nutanix

Effective paging techniques help reduce page faults, which are counted by tracking the number of times a page is accessed from disk.
Implementing LRU (Least Recently Used) algorithm to replace the page that has not been used for the longest time.
Using FIFO (First In, First Out) algorithm to replace the oldest page in memory.
Utilizing optimal page replacement algorithm to replace the page that will not be used for the longest time in the future.
Counting page faults by tracking ...read more
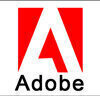
Asked in Adobe

Design a system to store incoming characters in sorted manner and answer queries about character presence.
Use a data structure like a balanced binary search tree to store characters in sorted order.
Implement functions to insert characters into the tree and check for character presence.
Optimize search queries by using binary search or tree traversal techniques.
Consider edge cases like duplicate characters and handling special characters.
Example: Insert 'a', 'c', 'b' -> Tree: [...read more
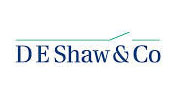
Asked in DE Shaw

Static loading is done at compile time while dynamic loading is done at runtime in operating systems.
Static loading involves loading all the necessary libraries and dependencies at compile time.
Dynamic loading involves loading libraries and dependencies at runtime when they are needed.
Static loading is faster but consumes more memory, while dynamic loading is slower but more memory efficient.
Examples of static loading include C/C++ programs, while examples of dynamic loading ...read more
Interview Experiences of Popular Companies
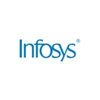
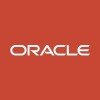
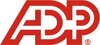
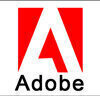
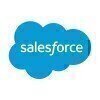
Top Interview Questions for Mts1 Related Skills
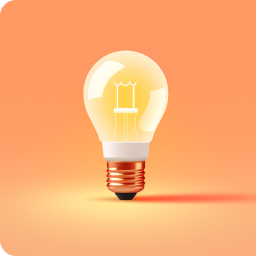
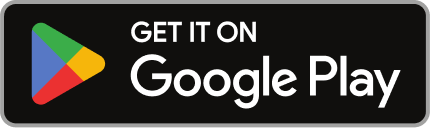
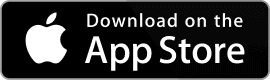
Reviews
Interviews
Salaries
Users
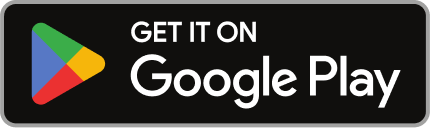
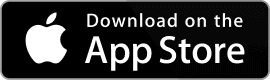