Lead Data Engineer
40+ Lead Data Engineer Interview Questions and Answers
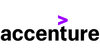
Asked in Accenture

Q. Given a DataFrame df with columns 'A', 'B','C' how would you group the data by the values in column 'A' and calculate the mean of column 'B' for each group, while also summing the values in column 'C'?
Group data by column 'A', calculate mean of column 'B' and sum values in column 'C' for each group.
Use groupby() function in pandas to group data by column 'A'
Apply mean() function on column 'B' and sum() function on column 'C' for each group
Example: df.groupby('A').agg({'B':'mean', 'C':'sum'})
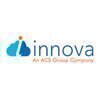
Asked in Innova Solutions

Q. Given a string containing alphanumeric characters, how would you write a function to output repeated characters if a number is present before the character in the string? For example, Input: as2d3c[x]4b, Output...
read moreThe function should output repeated characters based on the numbers present before each character in the input string.
Iterate through the input string character by character.
If a number is encountered, store it as the repeat count for the next character.
If a character is encountered, repeat it based on the stored count and append to the output string.
Handle special characters like brackets separately.
Example: Input 'as2d3c[x]4b' should output 'asddcccxbbbb'.
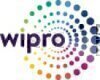
Asked in Wipro

Q. How would you build an ETL pipeline to read JSON files that are irregularly dropped into storage, transform the data, and match the schema?
Design an ETL pipeline to handle irregularly timed JSON file uploads for data transformation and schema matching.
Use a cloud storage service (e.g., AWS S3) to store incoming JSON files.
Implement a file watcher or event-driven architecture (e.g., AWS Lambda) to trigger processing when new files arrive.
Utilize a data processing framework (e.g., Apache Spark or Apache Beam) to read and transform the JSON data.
Define a schema using a tool like Apache Avro or JSON Schema to ensure...read more
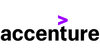
Asked in Accenture

Q. Discuss the concept of Python decorators and provide an example of how you would use decorators to measure the execution time of a function.
Python decorators are functions that modify the behavior of other functions. They are commonly used for adding functionality to existing functions without modifying their code.
Decorators are defined using the @ symbol followed by the decorator function name.
They can be used to measure the execution time of a function by wrapping the function with a timer decorator.
Example: def timer(func): def wrapper(*args, **kwargs): start_time = time.time() result = func(*args, **kwargs) e...read more
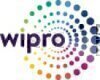
Asked in Wipro

Q. Write an SQL query to find the users who made purchases in 3 consecutive months within a year.
SQL query to find users who purchased 3 consecutive months in a year
Use a self join on the table to compare purchase months for each user
Group by user and year, then filter for counts of 3 consecutive months
Example: SELECT user_id FROM purchases p1 JOIN purchases p2 ON p1.user_id = p2.user_id WHERE p1.month = p2.month - 1 AND p2.month = p1.month + 1 GROUP BY p1.user_id, YEAR(p1.purchase_date) HAVING COUNT(DISTINCT MONTH(p1.purchase_date)) = 3
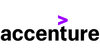
Asked in Accenture

Q. Explain the difference between the deepcopy() and copy() methods in Python's copy module. Provide a scenario where you would use deepcopy() over copy().
deepcopy() creates a new object with completely independent copies of nested objects, while copy() creates a shallow copy.
deepcopy() creates a new object and recursively copies all nested objects, while copy() creates a shallow copy of the top-level object only.
Use deepcopy() when you need to create a deep copy of an object with nested structures, to avoid any references to the original object.
Use copy() when you only need a shallow copy of the object, where changes to nested...read more
Lead Data Engineer Jobs


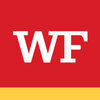
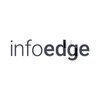
Asked in Info Edge

Q. What are the key components and design principles of pipeline architecture?
Key components and design principles of pipeline architecture
Key components: Source, Processor, Sink
Design principles: Scalability, Reliability, Maintainability
Examples: Apache Kafka, Apache NiFi, AWS Data Pipeline
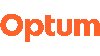
Asked in Optum Global Solutions

Q. What is the optimal Spark configuration for loading 1 TB of data split into 128MB chunks?
Set executor memory to 8GB and executor cores to 5 for optimal performance.
Set spark.executor.memory to 8g
Set spark.executor.cores to 5
Set spark.default.parallelism to 8000
Use Hadoop InputFormat to read data in 128MB chunks
Share interview questions and help millions of jobseekers 🌟
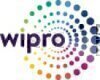
Asked in Wipro

Q. Write SQL to find the second highest salary of employees in each department.
SQL query to find the second highest salary of employees in each department
Use a subquery to rank the salaries within each department
Filter the results to only include the second highest salary for each department
Join the result with the employee table to get additional information if needed
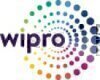
Asked in Wipro

Q. Write an SQL query using window functions to find the highest sale amount per day for each store.
Use SQL window functions to identify the highest sale amount for each store per day.
Use the ROW_NUMBER() function to rank sales within each day and store.
Partition the data by store and date to isolate daily sales.
Order the sales in descending order to get the highest sale at the top.
Example SQL query: SELECT store_id, sale_date, sale_amount, ROW_NUMBER() OVER (PARTITION BY store_id, sale_date ORDER BY sale_amount DESC) as rank FROM sales WHERE rank = 1;
Asked in NAVISTAR INTERNATIONAL

Q. Explain a scenario where you implemented an end-to-end pipeline.
Implemented end-to-end pipeline for processing and analyzing customer data in real-time
Designed data ingestion process to collect customer data from various sources
Implemented data processing and transformation steps to clean and enrich the data
Developed machine learning models to analyze customer behavior and make predictions
Deployed the pipeline on a cloud platform for scalability and reliability
Monitored the pipeline performance and made optimizations for efficiency
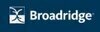
Asked in Broadridge Financial Solutions

Q. How can you open multiple sessions in PostgreSQL?
To open multiple sessions in PostgreSQL, you can use multiple connections from different clients.
Use different client applications to connect to the PostgreSQL database with different credentials
Each client connection will create a separate session in PostgreSQL
You can also use connection pooling to manage multiple sessions efficiently
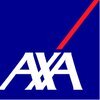
Asked in AXA

Q. How do you manage stakeholder expectations?
I manage stakeholder expectations by setting clear goals, communicating effectively, and providing regular updates.
Set clear goals and objectives with stakeholders from the beginning
Communicate regularly and effectively to keep stakeholders informed
Provide updates on progress, challenges, and any changes in plans
Manage expectations by being transparent about limitations and potential delays
Seek feedback from stakeholders to ensure alignment and address any concerns
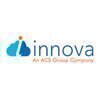
Asked in Innova Solutions

Q. Write a program for vending machine actions using object-oriented programming principles.
A program for vending machine actions using object-oriented programming principles.
Create a class for VendingMachine with attributes like items, prices, and quantities
Implement methods for adding items, selecting items, and returning change
Use encapsulation to protect data and ensure proper functionality
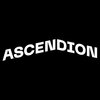
Asked in Ascendion

Q. How do you handle deeply nested JSON structures?
Handling deeply nested JSON involves parsing, flattening, and transforming data for easier access and manipulation.
Use libraries like `json` in Python or `Jackson` in Java to parse JSON data.
Flatten the JSON structure using recursion or libraries like `pandas` in Python to convert it into a DataFrame.
Access nested elements using dot notation or bracket notation, e.g., `data['key']['nestedKey']`.
Transform deeply nested JSON into a more manageable format, such as a relational d...read more
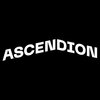
Asked in Ascendion

Q. How do you handle late-arriving data in a streaming environment?
Handling late arrival data in streaming involves strategies to ensure data accuracy and consistency despite delays.
Watermarking: Use watermarks to track the progress of data processing and define thresholds for late data handling.
Event Time vs. Processing Time: Distinguish between event time (when data was generated) and processing time (when data is processed) to manage late data effectively.
Buffering: Temporarily store late-arriving data in a buffer until it can be processe...read more
Asked in NAVISTAR INTERNATIONAL

Q. What is your experience with data modeling?
I have extensive experience in data modeling, including designing relational databases and creating data models for various business needs.
Designed and implemented data models for e-commerce platform to optimize product recommendations
Created data models for financial services company to track customer transactions and analyze spending patterns
Utilized ER diagrams and normalization techniques to ensure data integrity and efficiency
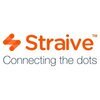
Asked in Straive

Q. Python coding on anagrams,valid parenthesis using stack
This involves checking for anagrams and validating parentheses using Python and stack data structures.
Anagrams: Two strings are anagrams if they contain the same characters in the same frequency. Example: 'listen' and 'silent'.
Valid Parentheses: A string of parentheses is valid if every opening parenthesis has a corresponding closing one. Example: '()[]{}' is valid.
Using a stack for parentheses: Push opening brackets onto the stack and pop when a closing bracket is encountere...read more
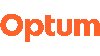
Asked in Optum Global Solutions

Q. Explain windowing functions and provide a use case where they can be applied.
Windowing functions are used to perform calculations on a subset of data within a larger dataset.
Windowing functions are used to calculate running totals, moving averages, and rank functions.
They are commonly used in time series analysis and financial analysis.
Examples of windowing functions include ROW_NUMBER(), RANK(), DENSE_RANK(), and NTILE().
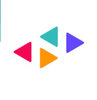
Asked in Nielsen

Q. How would you design an ETL process and ensure data quality?
Design ETL process to ensure high data quality by implementing data validation, cleansing, and transformation steps.
Identify data sources and define data extraction methods
Implement data validation checks to ensure accuracy and completeness
Perform data cleansing to remove duplicates, errors, and inconsistencies
Transform data into a consistent format for analysis and reporting
Utilize tools like Apache NiFi, Talend, or Informatica for ETL processes

Asked in Metyis

Q. Data Quality process related to the project
Data quality process ensures accuracy, completeness, and consistency of data throughout the project.
Establish data quality standards and metrics
Implement data profiling to identify issues
Perform data cleansing and normalization
Conduct regular data quality checks and audits
Involve stakeholders in data quality improvement efforts
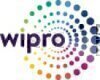
Asked in Wipro

Q. How does Kafka work with Spark Streaming?
Kafka is used as a message broker to ingest data into Spark Streaming for real-time processing.
Kafka acts as a buffer between data producers and Spark Streaming to handle high throughput of data
Spark Streaming can consume data from Kafka topics in micro-batches for real-time processing
Kafka provides fault-tolerance and scalability for streaming data processing in Spark
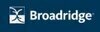
Asked in Broadridge Financial Solutions

Q. How can you flatten XML data in Python?
Use the xmltodict library in Python to flatten XML data structures.
Install the xmltodict library using pip install xmltodict
Use xmltodict.parse() to convert XML data to a Python dictionary
Use json.dumps() to convert the dictionary to a JSON string for a flattened structure
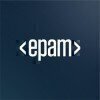
Asked in EPAM Systems

Q. What are decorators in Python?
Decorators are a way to modify or enhance the behavior of a function or class without changing its source code.
Decorators are defined using the '@' symbol followed by the decorator name.
They can be used to add functionality to a function or class, such as logging or timing.
Decorators can also be used to modify the behavior of a function or class, such as adding caching or memoization.
Multiple decorators can be applied to a single function or class, and they are executed in th...read more
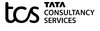
Asked in TCS

Q. What are generators in Python?
Generators are functions that allow you to declare a function that behaves like an iterator.
Generators use the yield keyword to return a generator object that can be iterated over.
They allow for lazy evaluation, meaning that they only generate values as needed.
Generators are memory efficient as they do not store all values in memory at once.
They can be used to generate an infinite sequence of values.
Example: def my_generator(): yield 1; yield 2; yield 3
Example: for num in my_...read more
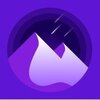
Asked in Nightfall AI

Q. Describe how you would design a data streaming pipeline for analytics.
Design a scalable data streaming architecture for real-time analytics using modern tools and best practices.
Use Apache Kafka for high-throughput data ingestion and real-time processing.
Implement stream processing with Apache Flink or Spark Streaming for analytics.
Store processed data in a data lake (e.g., AWS S3) for batch analytics.
Utilize a schema registry (e.g., Confluent Schema Registry) for data consistency.
Incorporate monitoring tools (e.g., Prometheus, Grafana) for sys...read more
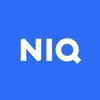
Asked in NielsenIQ

Q. How do you optimize PySpark code?
Optimizing PySpark involves tuning configurations, using efficient transformations/actions, and leveraging caching.
Tune PySpark configurations for optimal performance (e.g. adjusting memory settings, parallelism)
Use efficient transformations/actions to minimize unnecessary data shuffling (e.g. using narrow transformations like map instead of wide transformations like groupByKey)
Leverage caching to persist intermediate results in memory for faster access

Asked in JPMorgan Chase & Co.

Q. Let's do a code review of some code on screen share.
Reviewing code during interview for Lead Data Engineer position
Ensure code follows best practices and is well-documented
Check for any potential performance issues or bottlenecks
Look for any security vulnerabilities or data privacy concerns
Provide constructive feedback and suggestions for improvement
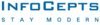
Asked in Infocepts Technologies

Q. What is ETL? Different process
ETL stands for Extract, Transform, Load. It is a process used to extract data from various sources, transform it into a consistent format, and load it into a target database.
Extract: Data is extracted from multiple sources such as databases, files, APIs, etc.
Transform: Data is cleaned, filtered, aggregated, and converted into a consistent format.
Load: Transformed data is loaded into a target database or data warehouse for analysis.
Examples: Extracting customer data from a CRM...read more
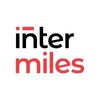
Asked in Intermiles

Q. Shift to Data Engineering from Oracle
Transitioning from Oracle to Data Engineering
Learn SQL and database concepts
Familiarize with ETL tools like Apache NiFi and Talend
Gain knowledge of big data technologies like Hadoop and Spark
Develop skills in programming languages like Python and Java
Understand data modeling and schema design
Get hands-on experience with cloud platforms like AWS and Azure
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
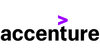
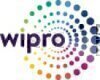
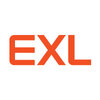

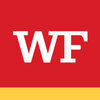
Top Interview Questions for Lead Data Engineer Related Skills
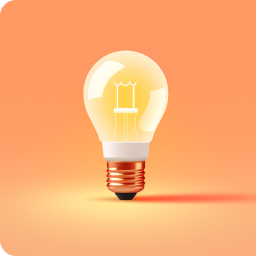
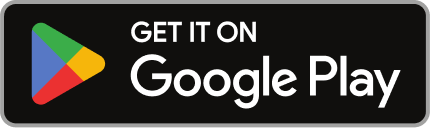
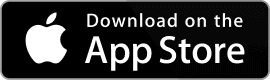
Reviews
Interviews
Salaries
Users
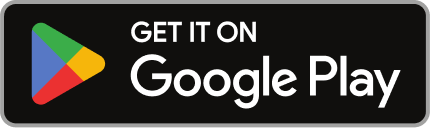
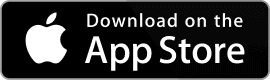