Java Developer Intern
20+ Java Developer Intern Interview Questions and Answers for Freshers
Asked in Xciteducation Worldwide

Q. What is java ? Whay is java is platform independent language? Why is java not a pure object oriented language? How is java different from c++ ?
Java is a popular programming language known for its platform independence and object-oriented approach.
Java is platform independent because it uses the concept of bytecode and virtual machine (JVM).
Bytecode is a platform-neutral code that can be executed on any operating system or architecture.
JVM interprets the bytecode and translates it into machine-specific instructions.
Java is not a pure object-oriented language because it supports primitive data types like int, float, e...read more
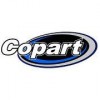
Asked in Copart

Q. How do you create a singleton class in a multi-threaded environment?
To create a singleton class in a multi-threaded environment, we can use double-checked locking or synchronized block.
Use double-checked locking to minimize the use of synchronization and improve performance.
In double-checked locking, check if the instance is null, then synchronize the block and create the instance.
Use volatile keyword to ensure visibility of the instance across threads.
Alternatively, use synchronized block to create a thread-safe singleton class.
In synchroniz...read more
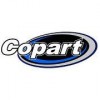
Asked in Copart

Q. How do you implement a circular linked list in Java?
A circular linked list is a linked list where the last node points back to the first node, forming a loop.
Create a Node class with data and next pointer
Initialize the head and tail pointers to null
When adding a node, if the list is empty, set head and tail to the new node
If the list is not empty, set the next pointer of the tail to the new node and update the tail
To traverse the circular linked list, start from the head and keep moving to the next node until you reach the hea...read more
Asked in Xciteducation Worldwide

Q. Tell us something about jit compiler. Why string is immutable. Defference between interface and abtract. Defference between process and threads.
JIT compiler compiles bytecode to machine code at runtime. String is immutable to ensure security and thread safety. Interface defines only method signatures while abstract class can have method implementations. Process is an instance of a program while thread is a subset of a process.
JIT compiler stands for Just-In-Time compiler and is used to improve the performance of Java programs by compiling bytecode to machine code at runtime.
String is immutable to ensure security and ...read more
Asked in Xciteducation Worldwide

Q. Explain run time polymorphism. what is garbage collector. Defference between while loop and do while loop. Explain main method in java.
Explaining run time polymorphism, garbage collector, while loop, do while loop, and main method in Java.
Run time polymorphism is the ability of an object to take on many forms. It is achieved through method overriding.
Garbage collector is a part of JVM that automatically frees up memory by removing objects that are no longer in use.
While loop executes the code block only if the condition is true. Do while loop executes the code block at least once before checking the conditio...read more

Asked in Visa

Q. How do you create an immutable class in Java?
An immutable class in Java is a class whose state cannot be modified after it is created.
Declare the class as final to prevent inheritance
Declare all fields as private and final
Do not provide any setter methods
Ensure that any mutable objects within the class are not accessible or modifiable
Provide only getter methods to access the fields
If a field is mutable, return a copy of it instead of the original object
Java Developer Intern Jobs
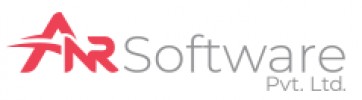

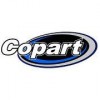
Asked in Copart

Q. Why do we have a wait method in the Object class?
The wait method in the Object class is used for inter-thread communication and synchronization.
wait() is used to make a thread wait until another thread notifies it.
It is used in multi-threaded applications to coordinate the execution of threads.
wait() releases the lock held by the current thread, allowing other threads to acquire it.
It is typically used in conjunction with notify() and notifyAll() methods.
Example: wait() can be used to implement the producer-consumer pattern...read more
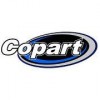
Asked in Copart

Q. What is the difference between RDBMS and NoSQL?
RDBMS is a relational database management system that uses structured data, while NoSQL is a non-relational database that uses unstructured data.
RDBMS stores data in tables with predefined schemas, while NoSQL stores data in various formats like key-value, document, columnar, or graph.
RDBMS supports ACID (Atomicity, Consistency, Isolation, Durability) properties, while NoSQL sacrifices some of these properties for scalability and performance.
RDBMS is suitable for structured d...read more
Share interview questions and help millions of jobseekers 🌟
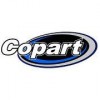
Asked in Copart

Q. Are Generics only limited to collections?
No, generics are not limited to collections.
Generics can be used with any type of class or interface, not just collections.
They provide type safety and allow for code reusability.
For example, generics can be used with classes like ArrayList, LinkedList, HashMap, etc.
They can also be used with interfaces like Comparable, Iterator, etc.
Asked in Code Vyasa

Q. What are the differences between HashMap and LinkedHashMap?
HashMap is an unordered collection while LinkedHashMap maintains insertion order.
HashMap uses hash table to store key-value pairs.
LinkedHashMap uses doubly-linked list to maintain the insertion order.
HashMap provides faster access and retrieval time complexity.
LinkedHashMap provides predictable iteration order based on insertion order.
Example: HashMap - {1=A, 2=B, 3=C}, LinkedHashMap - {1=A, 2=B, 3=C}
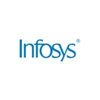
Asked in Infosys

Q. What are the key concepts of Object-Oriented Programming (OOP)?
Key concepts of OOP include encapsulation, inheritance, polymorphism, and abstraction.
Encapsulation: Bundling data and methods that operate on the data into a single unit (class). Example: class Car { private String model; public void drive() { ... } }
Inheritance: Creating new classes based on existing classes, inheriting their attributes and methods. Example: class SUV extends Car { ... }
Polymorphism: Objects of different classes can be treated as objects of a common supercl...read more
Asked in UnoCareer

Q. what is java and who was developed java
Java is a high-level, object-oriented programming language used for developing applications and software.
Developed by James Gosling at Sun Microsystems in 1995
Runs on a variety of platforms, including Windows, Mac OS, and Linux
Used for developing web, mobile, and desktop applications
Known for its security, reliability, and portability
Popular frameworks include Spring, Hibernate, and Struts
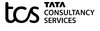
Asked in TCS

Q. What is normalization?
Normalization is the process of organizing data in a database to eliminate redundancy and improve data integrity.
Normalization helps in reducing data redundancy by breaking down a database into multiple tables.
It ensures that each table has a single purpose and avoids data duplication.
Normalization follows a set of rules called normal forms, such as First Normal Form (1NF), Second Normal Form (2NF), etc.
By eliminating redundancy, normalization improves data integrity and redu...read more
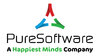
Asked in PureSoftware

Q. What is ORM and entity class ,controller layer ,REST ,springboot, hibernate
ORM is Object-Relational Mapping, entity class represents a database table, controller layer handles HTTP requests, REST is an architectural style, Spring Boot is a Java framework, Hibernate is an ORM tool.
ORM (Object-Relational Mapping) is a technique to map objects to database tables and vice versa.
Entity class represents a database table and its fields as Java objects.
Controller layer in a web application handles HTTP requests and routes them to appropriate methods.
REST (R...read more
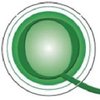
Asked in Kvaliteta Systems Solutions

Q. What are the steps to connect JDBC with SQL?
To connect JDBC with SQL, you need to load the JDBC driver, establish a connection, create a statement, execute the query, and process the results.
Load the JDBC driver using Class.forName() method
Establish a connection to the database using DriverManager.getConnection() method
Create a statement object using the connection.createStatement() method
Execute the query using statement.executeQuery() method
Process the results by iterating through the ResultSet object
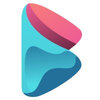
Asked in BEEU TECH

Q. Where do you see yourself in the next five years?
In five years, I envision myself as a skilled Java developer, contributing to innovative projects and leading teams effectively.
I aim to deepen my expertise in Java and related technologies, such as Spring and Hibernate.
I aspire to take on leadership roles, mentoring junior developers and guiding project teams.
I plan to contribute to open-source projects to enhance my skills and give back to the community.
I hope to stay updated with industry trends, possibly earning certifica...read more
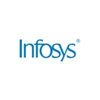
Asked in Infosys

Q. How do you swap two numbers without using a third variable?
Swap two numbers without using a third variable in Java
Use XOR operation to swap two numbers without using a third variable
Example: int a = 5, b = 10; a = a ^ b; b = a ^ b; a = a ^ b; // Now a = 10, b = 5
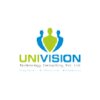
Asked in Univision Technology Consulting

Q. what is inheritance and polymorphisim
Inheritance is a mechanism in which a new class inherits properties and behaviors from an existing class. Polymorphism allows objects of different classes to be treated as objects of a common superclass.
Inheritance allows for code reusability and promotes the concept of 'is-a' relationship.
Polymorphism allows for flexibility in code design and enables dynamic method binding.
Example of inheritance: class Dog extends Animal, inheriting properties and behaviors of Animal class.
E...read more
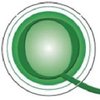
Asked in Kvaliteta Systems Solutions

Q. What is an outer join?
An outer join is a type of join operation that includes all rows from both tables being joined, even if there is no match between the rows.
Includes all rows from both tables, even if there is no match
Returns NULL values for columns where there is no match
Types of outer joins include LEFT OUTER JOIN, RIGHT OUTER JOIN, and FULL OUTER JOIN
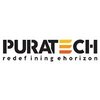
Asked in Puratech Solutions

Q. Mcq and write the output of code
The question involves answering multiple choice questions and predicting the output of Java code.
Understand the concepts of Java programming language thoroughly.
Practice solving MCQs and predicting code outputs to improve accuracy.
Pay attention to details and syntax in the code snippets provided.
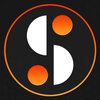
Asked in Synarion IT Solutions

Q. Tools that are used in java development.
Various tools used in Java development include IDEs, build tools, version control systems, and testing frameworks.
IDEs: Eclipse, IntelliJ IDEA, NetBeans
Build tools: Maven, Gradle, Ant
Version control systems: Git, SVN, Mercurial
Testing frameworks: JUnit, TestNG, Mockito
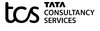
Asked in TCS

Q. What is Java?
Java is a high-level programming language known for its portability, security, and object-oriented features.
Java is platform-independent, meaning it can run on any device with a Java Virtual Machine (JVM).
It is known for its strong security features, such as automatic memory management and built-in exception handling.
Java is an object-oriented language, allowing for the creation of reusable code through classes and objects.
Popular frameworks and libraries in Java include Spri...read more
Asked in HulkHire Tech

Q. what is spring and springboot?
Spring is a framework for building Java applications, while Spring Boot is a tool for easily creating stand-alone, production-grade Spring-based applications.
Spring is a framework that provides comprehensive infrastructure support for developing Java applications.
Spring Boot is a tool that simplifies the process of creating stand-alone, production-grade Spring-based applications.
Spring Boot eliminates the need for a lot of configuration, allowing developers to quickly set up ...read more
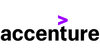
Asked in Accenture

Q. What is Exception Handling?
Exception handling is a mechanism to handle runtime errors in a program to prevent it from crashing.
It allows the program to gracefully handle unexpected situations.
Exceptions can be caught and handled using try-catch blocks.
Common exceptions include NullPointerException, ArrayIndexOutOfBoundsException, and IOException.
Handling exceptions improves the robustness and reliability of the program.
Asked in Brained

Q. What is OOPS? Explain.
OOPs stands for Object-Oriented Programming. It is a programming paradigm based on the concept of objects.
OOPs focuses on creating objects that contain data and methods to manipulate that data.
It involves concepts like inheritance, encapsulation, polymorphism, and abstraction.
Java is an example of an object-oriented programming language that follows OOPs principles.
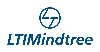
Asked in LTIMindtree

Q. How do you remove duplicates from an array?
Use a Set to remove duplicates from an array of strings.
Create a Set to store unique elements.
Iterate through the array and add each element to the Set.
Convert the Set back to an array to get the array without duplicates.
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
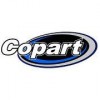
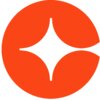
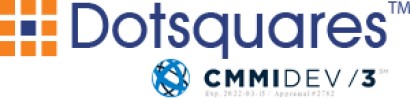
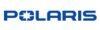

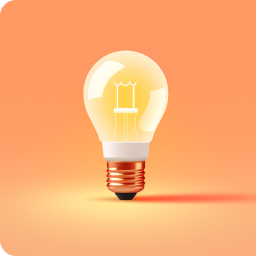
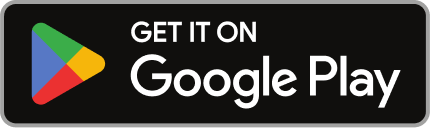
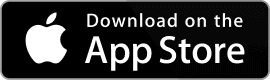
Reviews
Interviews
Salaries
Users
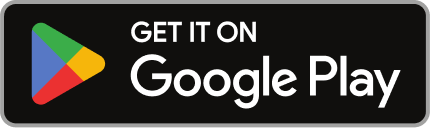
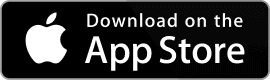