Full Stack Developer
1000+ Full Stack Developer Interview Questions and Answers
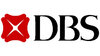
Asked in DBS Bank

Q. Query and Matrix Problem Statement
You are given a binary matrix with 'M' rows and 'N' columns, initially consisting of all 0s. You will receive 'Q' queries, which can be of four types:
Query 1: 1 R index
Query ...read more
Given a binary matrix, perform queries to flip elements in rows/columns and count zeros in rows/columns.
Iterate through each query and update the matrix accordingly
For type 1 queries, flip the elements in the specified row/column
For type 2 queries, count the number of zeros in the specified row/column
Return the count of zeros for type 2 queries
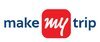
Asked in MakeMyTrip

Q. Tower of Hanoi Problem Statement
You have three rods numbered from 1 to 3, and 'N' disks initially stacked on the first rod in increasing order of their sizes (largest disk at the bottom). Your task is to move ...read more
Tower of Hanoi problem involves moving 'N' disks from one rod to another following specific rules in less than 2^N moves.
Implement a recursive function to move disks from one rod to another while following the rules.
Use the concept of recursion and backtracking to solve the Tower of Hanoi problem efficiently.
Maintain a count of moves and track the movement of disks in a 2-D array/list.
Ensure that larger disks are not placed on top of smaller disks during the movement.
The solu...read more
Full Stack Developer Interview Questions and Answers for Freshers
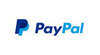
Asked in PayPal

Q. Maximum Difference Problem Statement
Given an array ARR
of N
elements, your task is to find the maximum difference between any two elements in ARR
.
If the maximum difference is even, print EVEN; if it is odd, p...read more
Find the maximum difference between any two elements in an array and determine if it is even or odd.
Iterate through the array to find the maximum and minimum elements
Calculate the difference between the maximum and minimum elements
Check if the difference is even or odd and return the result
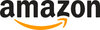
Asked in Amazon

Q. LCA of Binary Tree Problem Statement
You are given a binary tree consisting of distinct integers and two nodes, X
and Y
. Your task is to find and return the Lowest Common Ancestor (LCA) of these two nodes.
The ...read more
Find the Lowest Common Ancestor of two nodes in a binary tree.
Traverse the binary tree to find the paths from the root to nodes X and Y.
Compare the paths to find the last common node, which is the Lowest Common Ancestor.
Implement a recursive function to find the LCA efficiently.
Handle edge cases such as when X or Y is the root node or when X or Y is not present in the tree.
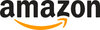
Asked in Amazon

Q. Count Set Bits Problem Statement
Given a positive integer N
, compute the total number of '1's in the binary representation of all numbers from 1 to N. Return this count modulo 1e9+7 because the result can be ve...read more
Count the total number of set bits in the binary representation of numbers from 1 to N modulo 1e9+7.
Iterate through numbers from 1 to N and count the set bits in their binary representation
Use bitwise operations to count the set bits efficiently
Return the count modulo 1e9+7 for each test case
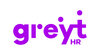
Asked in greytHR

Q. Most Frequent Non-Banned Word Problem Statement
Given a paragraph consisting of letters in both lowercase and uppercase, spaces, and punctuation, along with a list of banned words, your task is to find the most...read more
Find the most frequent word in a paragraph that is not in a list of banned words.
Split the paragraph into words and convert them to uppercase for case-insensitivity.
Count the frequency of each word, excluding banned words.
Return the word with the highest frequency in uppercase.
Full Stack Developer Jobs
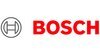
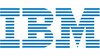
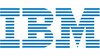
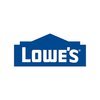
Asked in Lowe's

Q. Excel Sheet Column Number Problem Statement
You are provided with a string STR
representing a column title in an Excel Sheet. Your task is to determine the corresponding column number.
Example:
A typical exampl...read more
Convert Excel sheet column title to corresponding column number.
Iterate through the characters in the string from right to left, starting with a result of 0.
For each character, calculate its value by subtracting 'A' and adding 1, then multiply by 26 raised to the power of its position from the right.
Add up all the calculated values to get the final column number.
Example: For input 'AB', A=1, B=2, so result = (1 * 26^1) + (2 * 26^0) = 28.
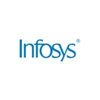
Asked in Infosys

Q. Distinct Strings With Odd and Even Swapping Allowed Problem Statement
You are provided with an array of strings, and your objective is to determine the number of unique strings within it.
A string is deemed uni...read more
Determine the number of unique strings in an array by applying swapping operations on odd and even indices.
Iterate through each string in the array and check if it can be transformed into another string by swapping characters at odd and even indices.
Keep track of unique strings by comparing them with all other strings in the array.
Return the count of unique strings found in the array.
Share interview questions and help millions of jobseekers 🌟
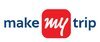
Asked in MakeMyTrip

Q. Reach the Destination Problem Statement
You are given a source point (sx, sy) and a destination point (dx, dy). Determine if it is possible to reach the destination point using only the following valid moves:
- ...read more
Determine if it is possible to reach the destination point from the source point using specified moves.
Use depth-first search (DFS) to explore all possible paths from source to destination.
Keep track of visited points to avoid infinite loops.
Check if the destination point is reachable by following the valid moves.
Return true if destination is reachable, false otherwise.
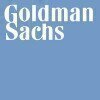
Asked in Goldman Sachs

Q. Matrix Rank Calculation
Given a matrix ARR
of dimensions N * M
, your task is to determine the rank of the matrix ARR
.
Explanation:
The rank of a matrix is defined as:
(a) The maximum number of linearly independ...read more
Calculate the rank of a matrix based on linearly independent column or row vectors.
Determine the number of linearly independent column vectors or row vectors in the matrix.
Use Gaussian elimination or row reduction to simplify the matrix and find the rank.
The rank of a matrix is the maximum number of linearly independent vectors it contains.
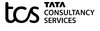
Asked in TCS

Q. Find Duplicates in an Array
Given an array ARR
of size 'N', where each integer is in the range from 0 to N - 1, identify all elements that appear more than once.
Return the duplicate elements in any order. If n...read more
The task is to find all duplicate elements in an array of integers.
Iterate through the array and keep track of the count of each element using a hashmap.
Return all elements from the hashmap with count greater than 1 as the duplicate elements.
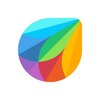
Asked in Freshworks

Q. Reverse Integer Problem Statement
Given a 32-bit signed integer N
, your task is to return the reversed integer. If reversing the integer causes overflow, return -1
.
Input:
The first line contains an integer 'T'...read more
The task is to reverse a 32-bit signed integer and handle overflow cases.
Create a function that takes a 32-bit signed integer as input
Reverse the integer while handling overflow cases
Return the reversed integer or -1 if overflow occurs
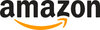
Asked in Amazon

Q. Find All Pairs Adding Up to Target
Given an array of integers ARR
of length N
and an integer Target
, your task is to return all pairs of elements such that they add up to the Target
.
Input:
The first line conta...read more
Find all pairs of elements in an array that add up to a given target.
Iterate through the array and store each element in a hashmap along with its index.
For each element, check if the target minus the element exists in the hashmap.
If found, print the pair of elements. If not found, print (-1, -1).
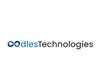
Asked in OodlesTechnologies

Q. Find a Node in Linked List
Given a singly linked list of integers, your task is to implement a function that returns the index/position of an integer value 'N' if it exists in the linked list. Return -1 if the ...read more
Implement a function to find the index of a given integer in a singly linked list.
Traverse the linked list while keeping track of the index of each element.
Compare each element with the target integer 'N'.
Return the index if the element is found, otherwise return -1.
Handle cases where the target integer is not found in the linked list.
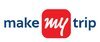
Asked in MakeMyTrip

Q. Minimum Operations Problem Statement
You are given an array 'ARR'
of size 'N'
consisting of positive integers. Your task is to determine the minimum number of operations required to make all elements in the arr...read more
Minimum number of operations to make all elements in an array equal by performing addition, subtraction, multiplication, or division.
Iterate through the array to find the maximum and minimum elements.
Calculate the difference between the maximum and minimum elements.
The minimum number of operations needed is the difference between the maximum and minimum elements.
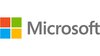
Asked in Microsoft Corporation

Q. Alternating Largest Problem Statement
Given a list of numbers, rearrange them such that every second element is greater than its adjacent elements. Implement a function to achieve this rearrangement.
Input:
The...read more
Rearrange a list of numbers such that every second element is greater than its adjacent elements.
Iterate through the array and swap elements if needed to satisfy the condition
Keep track of the current and next elements to compare and swap
Ensure that the swapped elements maintain the desired order
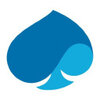
Asked in Capgemini Engineering

Q. Factorial Calculation Problem Statement
Develop a program to compute the factorial of a given integer 'n'.
The factorial of a non-negative integer 'n', denoted as n!
, is the product of all positive integers les...read more
Program to compute factorial of a given integer 'n', with error handling for negative values.
Create a function to calculate factorial using a loop or recursion
Check if input is negative, return 'Error' if true
Handle edge cases like 0 and 1 separately
Return the calculated factorial value
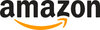
Asked in Amazon

Q. Add Two Numbers as Linked Lists
You are given two singly linked lists, where each list represents a positive number without any leading zeros.
Your task is to add these two numbers and return the sum as a linke...read more
Add two numbers represented as linked lists and return the sum as a linked list.
Traverse both linked lists simultaneously while keeping track of carry from previous sum
Create a new linked list to store the sum of the two numbers
Handle cases where one linked list is longer than the other by padding with zeros
Update the current node with the sum of corresponding nodes from both lists and carry
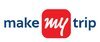
Asked in MakeMyTrip

Q. Maximum Distance Problem Statement
Given an integer array 'A' of size N, your task is to find the maximum value of j - i, with the restriction that A[i] <= A[j], where 'i' and 'j' are indices of the array.
Inpu...read more
Find the maximum distance between two elements in an array where the element at the first index is less than or equal to the element at the second index.
Iterate through the array and keep track of the minimum element encountered so far along with its index.
Calculate the maximum distance by subtracting the current index from the index of the minimum element.
Update the maximum distance if a greater distance is found while iterating.
Return the maximum distance calculated.
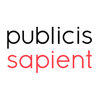
Asked in Publicis Sapient

Q. Count Substrings with K Distinct Characters
Given a lowercase string 'STR' and an integer K, the task is to count all possible substrings that consist of exactly K distinct characters. These substrings are not ...read more
Count substrings with exactly K distinct characters in a given lowercase string.
Iterate through all substrings of length K and count distinct characters.
Use a set to keep track of distinct characters in each substring.
Increment count if number of distinct characters equals K.
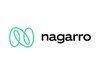
Asked in Nagarro

Q. Count Ways to Reach the N-th Stair Problem Statement
You are provided with a number of stairs, and initially, you are located at the 0th stair. You need to reach the Nth stair, and you can climb one or two step...read more
The task is to find the number of distinct ways to climb from the 0th step to the Nth step, where each time you can climb either one step or two steps.
Use dynamic programming to solve this problem
Create an array to store the number of ways to reach each step
Initialize the first two elements of the array as 1 and 2
For each subsequent step, the number of ways to reach that step is the sum of the number of ways to reach the previous two steps
Return the number of ways to reach th...read more
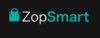
Asked in Zopsmart Technology

Q. Find Indices for Local Minima and Maxima
Given an integer array arr
of size N
, your task is to determine all indices of local minima and local maxima within the array. Return these indices in a 2-D list, where ...read more
The task is to find and return the indices of local minima and local maxima in the given array.
Iterate through the array and compare each element with its neighbors to determine if it is a local minima or maxima.
Consider corner elements separately by comparing them with only one neighbor.
Store the indices of local minima and maxima in separate lists.
If there are no local minima or maxima, return -1 as the only row element in the 2-D list.
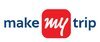
Asked in MakeMyTrip

Q. Sub Sort Problem Statement
You are given an integer array ARR
. Determine the length of the shortest contiguous subarray which, when sorted in ascending order, results in the entire array being sorted in ascendi...read more
Determine the length of the shortest contiguous subarray that needs to be sorted to make the entire array sorted in ascending order.
Iterate from left to right to find the first element out of order.
Iterate from right to left to find the last element out of order.
Calculate the length of the subarray between the two out of order elements.

Asked in Samsung Research

Array Sum Calculation
Calculate the sum of all elements in an array of length N
.
Input:
Line 1: An integer N indicating the size of the array.
Line 2: N integers, the elements of the array, separated by spaces.
Calculate the sum of all elements in an array of length N.
Read the size of the array N and then read N integers as elements of the array.
Iterate through the array and add each element to a running total to calculate the sum.
Return the final sum as the output.
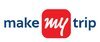
Asked in MakeMyTrip

Q. Make Array Elements Equal Problem Statement
Given an integer array, your objective is to change all elements to the same value, minimizing the cost. The cost of changing an element from x
to y
is defined as |x ...read more
Find the minimum cost to make all elements of an array equal by changing them to the same value.
Calculate the median of the array and find the sum of absolute differences between each element and the median.
Sort the array and find the median element, then calculate the sum of absolute differences between each element and the median.
If the array has an even number of elements, consider the average of the two middle elements as the median.
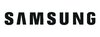
Asked in Samsung

Q. Reverse Linked List Problem Statement
Given a singly linked list of integers, return the head of the reversed linked list.
Example:
Initial linked list: 1 -> 2 -> 3 -> 4 -> NULL
Reversed linked list: 4 -> 3 -> 2...read more
Reverse a singly linked list of integers and return the head of the reversed linked list.
Iterate through the linked list and reverse the pointers to point to the previous node instead of the next node.
Use three pointers to keep track of the current, previous, and next nodes while reversing the linked list.
Update the head of the reversed linked list as the last node encountered during the reversal process.
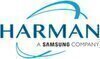
Asked in HARMAN

Q. What steps do you take to ensure accurate project estimates?
To ensure accurate project estimates, I follow a structured approach that includes analyzing requirements, breaking down tasks, and considering potential risks.
Analyze project requirements thoroughly
Break down tasks into smaller, manageable chunks
Consider potential risks and challenges
Use historical data to inform estimates
Collaborate with team members to validate estimates
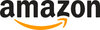
Asked in Amazon

Q. Count Inversions Problem Statement
Given an integer array ARR
of size N
, your task is to find the total number of inversions that exist in the array.
An inversion is defined for a pair of integers in the array ...read more
Count the total number of inversions in an integer array.
Iterate through the array and for each pair of elements, check if the inversion condition is met.
Use a nested loop to compare each pair of elements efficiently.
Keep a count of the inversions found and return the total count at the end.
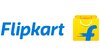
Asked in Flipkart

Q. Ceil Value from BST Problem Statement
Given a Binary Search Tree (BST) and an integer, write a function to return the ceil value of a particular key in the BST.
The ceil of an integer is defined as the smallest...read more
Ceil value of a key in a Binary Search Tree (BST) is the smallest integer greater than or equal to the given number.
Traverse the BST to find the closest integer greater than or equal to the given key.
Compare the key with the current node value and update the ceil value accordingly.
Recursively traverse left or right subtree based on the key value to find the ceil value.
Return the ceil value once found for each test case.
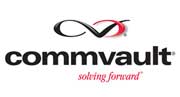
Asked in CommVault

Q. Collect Maximum Coins from Matrix
You are provided with a matrix consisting of 'M' rows and 'N' columns. Each cell in this matrix either contains a coin or is empty.
Your task is to collect the maximum number o...read more
Given a matrix with coins and empty cells, collect maximum coins from boundary cells and adjacent cells.
Iterate through the boundary cells and collect coins from them and their adjacent cells
Keep track of visited cells to avoid collecting the same coin multiple times
Use depth-first search or breadth-first search to explore adjacent cells
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
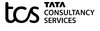
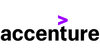
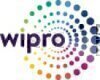
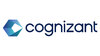
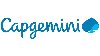
Top Interview Questions for Full Stack Developer Related Skills
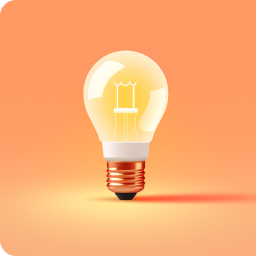
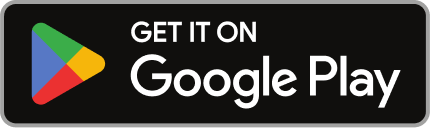
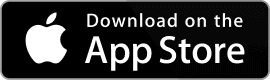
Reviews
Interviews
Salaries
Users
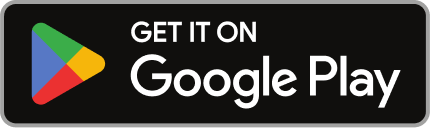
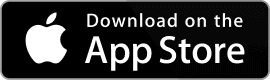