Front end Engineer
60+ Front end Engineer Interview Questions and Answers
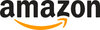
Asked in Amazon

Q. Pair Sum Problem Statement
You are given an integer array 'ARR' of size 'N' and an integer 'S'. Your task is to find and return a list of all pairs of elements where each sum of a pair equals 'S'.
Note:
Each pa...read more
Find pairs of elements in an array that sum up to a given value, sorted in a specific order.
Iterate through the array and use a hashmap to store the difference between the target sum and each element.
Check if the difference exists in the hashmap, if so, add the pair to the result list.
Sort the result list based on the criteria mentioned in the question.
Return the sorted list of pairs.
Asked in FactWise

Q. What is a callback? How is it accompanied with asynchronous programming
A callback is a function passed as an argument to another function to be executed later. It is commonly used in asynchronous programming.
A callback function is often used in event handling, AJAX requests, and setTimeout functions.
It allows the program to continue running while waiting for a response, improving efficiency.
Callbacks can be synchronous or asynchronous, with the latter being more common in modern web development.
Front end Engineer Interview Questions and Answers for Freshers
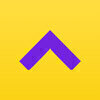
Asked in Housing.com

Deep copy creates a new copy of an object/array with all nested values copied, while shallow copy creates a new object/array with references to the original nested values.
Deep copy creates a completely new copy of an object/array, including all nested values, while shallow copy only creates a new object/array with references to the original nested values.
In JavaScript, deep copy can be achieved using methods like JSON.parse(JSON.stringify(obj)) or libraries like Lodash's _.cl...read more
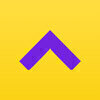
Asked in Housing.com

Absolute positioning is relative to the nearest positioned ancestor, while fixed positioning is relative to the viewport.
Absolute positioning is relative to the nearest positioned ancestor.
Fixed positioning is relative to the viewport.
Absolute positioned elements are removed from the normal document flow.
Fixed positioned elements stay in the same position even when the page is scrolled.
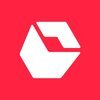
Asked in Snapdeal

Q. Write code to determine if an input date is today or tomorrow based on the current date. If it's neither, output the number of days difference between the input date and the current date.
Code to find if input date is today/tomorrow or no of days difference from current date.
Get current date using Date() constructor
Convert input date to Date object
Compare input date with current date to check if it's today/tomorrow
If not, calculate the difference in days using getTime() method
Output the result accordingly
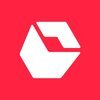
Asked in Snapdeal

Q. Explain box model in css, and what is specificity in CSS. What are render-blocking statements?
Box model defines how elements are rendered in CSS. Specificity determines which CSS rule applies to an element. Render-blocking statements delay page rendering.
Box model includes content, padding, border, and margin.
Specificity is calculated based on the number of selectors and their types.
Render-blocking statements are CSS or JavaScript files that prevent the page from rendering until they are loaded.
Use media queries to optimize rendering and reduce render-blocking stateme...read more
Front end Engineer Jobs
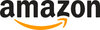
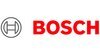
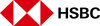
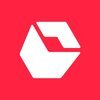
Asked in Snapdeal

Q. What is event bubbling, event capturing, and their uses?
Event bubbling and event capturing are two mechanisms in JavaScript that describe the order in which events are handled.
Event bubbling is the process where an event is first captured by the innermost element and then propagated to its parent elements.
Event capturing is the opposite process where an event is first captured by the outermost element and then propagated to its child elements.
Event bubbling is the default behavior in most browsers.
Event.stopPropagation() can be us...read more
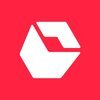
Asked in Snapdeal

Q. How would you implement infinite scrolling in React.js?
Implement infinite scrolling in React JS using Intersection Observer API.
Use Intersection Observer API to detect when the user has scrolled to the bottom of the page.
Fetch new data and append it to the existing data using setState.
Use a loading spinner to indicate that new data is being fetched.
Add a debounce function to prevent multiple API calls while scrolling.
Use a key prop when rendering the list of data to avoid re-rendering of existing elements.
Share interview questions and help millions of jobseekers 🌟
Asked in FactWise

Q. Implement a React app that fetches data from API endpoints, performs operations, and includes a search bar with debounce functionality.
Implement a React app that fetches data from APIs with search functionality and debounce for performance.
Use `useEffect` to fetch data from API when the component mounts.
Implement a search bar using controlled components in React.
Use `useState` to manage search input and fetched data.
Implement debounce using `setTimeout` to limit API calls during typing.
Example of debounce: `const debounce = (func, delay) => { let timeout; return (...args) => { clearTimeout(timeout); timeout ...read more
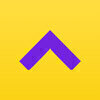
Asked in Housing.com

Yes, event delegation can be achieved by attaching an event listener to a parent element and then checking the target element of the event.
Attach an event listener to a parent element that contains the child elements you want to delegate events to.
Check the target element of the event to determine if it matches the desired child element.
Perform the appropriate action based on the target element.
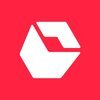
Asked in Snapdeal

Q. Tell me about Sass (Syntactically Awesome Style Sheets).
Saas is a CSS preprocessor that extends the functionality of CSS with variables, mixins, and more.
Saas stands for Syntactically Awesome Style Sheets
It allows for the use of variables, mixins, and functions in CSS
Saas code must be compiled into CSS before it can be used in a web page
Saas is often used in conjunction with build tools like Gulp or Webpack
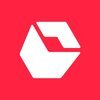
Asked in Snapdeal

Q. Write code to determine if two objects are equal in JavaScript.
Code to check equality of two objects in JavaScript
Use the JSON.stringify() method to convert the objects into strings
Compare the string representations of the objects using the === operator
If the strings are equal, the objects are considered equal
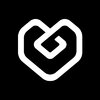
Asked in Fynd

Q. Implement traffic light functionality using HTML, CSS, and Vanilla JavaScript. Initially, the light should be RED. After 60 seconds, it should turn GREEN, then after 5 seconds, YELLOW, and after 3 seconds, turn...
read moreImplement Traffic Light functionality using HTML+CSS+Vanilla Javascript
Create HTML elements for the traffic light
Use CSS to style the elements to represent the different lights (red, green, yellow)
Use Vanilla Javascript to toggle the lights based on the specified time intervals
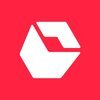
Asked in Snapdeal

Q. What is bind in JavaScript, and can you write its polyfill?
Bind creates a new function with a specified 'this' value and arguments.
Bind returns a new function with the same body as the original function.
The 'this' value of the new function is bound to the first argument passed to bind().
The subsequent arguments are passed as arguments to the new function.
Polyfill for bind() can be created using call() or apply() methods.
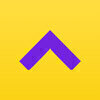
Asked in Housing.com

A browser renders a webpage by parsing HTML, CSS, and JavaScript, constructing the DOM tree, rendering the layout, and painting the pixels on the screen.
Browser requests the HTML file from the server
Browser parses the HTML and constructs the DOM tree
Browser fetches external resources like CSS and JavaScript files
Browser applies CSS styles to the DOM elements and constructs the render tree
Browser executes JavaScript code and updates the DOM accordingly
Browser calculates the la...read more
Asked in FactWise

Q. Explain the DOM and Virtual DOM. What are the differences between them?
DOM is a tree-like structure representing the HTML elements of a webpage. Virtual DOM is a lightweight copy of the DOM used for efficient updates.
DOM stands for Document Object Model and represents the structure of HTML elements on a webpage.
Virtual DOM is a lightweight copy of the DOM kept in memory by frameworks like React for efficient updates.
Changes made to the Virtual DOM are compared with the actual DOM, and only the differences are updated on the real DOM.
Virtual DOM ...read more
Asked in FactWise

Q. What is useCallback()? Explain in depth.
useCallback() is a React hook that returns a memoized callback function.
useCallback() is used to optimize performance by memoizing functions.
It is useful when passing callbacks to child components that rely on reference equality.
It takes a callback function and an array of dependencies as arguments.
The callback function is only re-created if any of the dependencies change.
Example: const memoizedCallback = useCallback(() => { // callback function }, [dependency1, dependency2])...read more
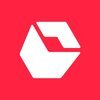
Asked in Snapdeal

Q. What is the difference between async and defer?
async loads script while page continues to load, defer loads script after page has loaded
async loads scripts asynchronously while page continues to load
defer loads scripts after the page has loaded
async scripts may not execute in order, while defer scripts do
async scripts may cause rendering issues, while defer scripts do not
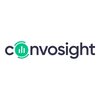
Asked in Convosight

Q. Have you worked on PWA and explain the benefits of using one?
Yes, I have worked on PWA. PWA stands for Progressive Web App and offers benefits like offline access, fast loading, and push notifications.
PWA provides offline access to users, allowing them to use the app even without an internet connection.
PWA loads quickly, providing a seamless user experience similar to native apps.
PWA can send push notifications to engage users and increase retention.
Examples of popular PWAs include Twitter Lite, Pinterest, and Starbucks.
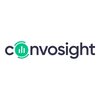
Asked in Convosight

Q. How can you apply certain CSS to three div elements?
You can apply a certain CSS on three div elements by using a common class or ID for all three divs.
Add a common class or ID to all three div elements
Define the CSS properties for that class or ID in your stylesheet
Apply the class or ID to each of the three div elements in your HTML markup
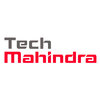
Asked in Tech Mahindra

Closures in JavaScript are functions that have access to variables from their outer scope even after the outer function has finished executing.
Closures allow functions to access variables from their parent function's scope
They are created whenever a function is defined within another function
Closures can be used to create private variables and functions in JavaScript
Asked in Bridge Healthcare

Q. Which data structure would you use in a music player to navigate to the next and previous song?
A circular linked list is ideal for navigating songs in a music player, allowing seamless next and previous song access.
Use a circular linked list to store songs, enabling easy traversal to the next and previous songs.
Each node in the list can represent a song, containing the song data and pointers to the next and previous nodes.
Example: If the current song is 'Song A', the next pointer leads to 'Song B', and the previous pointer leads back to 'Song C'.
This structure allows f...read more
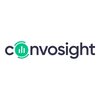
Asked in Convosight

Q. How would you architect logging events for your project?
I would architect logging events by defining clear event types, implementing structured logging, utilizing a centralized logging system, and setting up alerts for critical events.
Define clear event types to categorize different types of logs (e.g. error, info, warning)
Implement structured logging to include relevant metadata with each log entry (e.g. timestamp, user ID)
Utilize a centralized logging system like ELK stack or Splunk to aggregate and analyze logs efficiently
Set u...read more
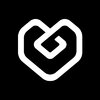
Asked in Fynd

Q. Write a program to reverse a string without using any built-in methods in JavaScript. (Two-pointer and One-pointer)
Program to reserve a string without using inbuilt methods in JavaScript
Use two pointers to swap characters at the beginning and end of the string until they meet in the middle
Create a function that takes a string as input and returns the reversed string
Example: Input 'hello', Output 'olleh'
Asked in Omniful

Q. How event loop work in js, hoisting, closure, find second largest in array and other code snippets.
Event loop in JS manages asynchronous operations, hoisting moves variable declarations to the top, closures allow functions to access outer scope variables, find second largest in array using sorting or looping.
Event loop in JS manages the execution of asynchronous tasks by placing them in a queue and executing them in order.
Hoisting in JS moves variable and function declarations to the top of their containing scope.
Closures in JS allow functions to access variables from thei...read more
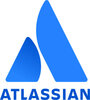
Asked in Atlassian

Q. Create a tic tac toe game taking the number of boards as a parameter.
Create a tic tac toe game with customizable number of boards.
Accept number of boards as a parameter
Create a 3x3 grid for each board
Implement logic to check for winning combinations on each board
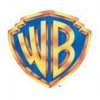
Asked in Warner Bros.

Q. Find second highest number in an array. Find whether linkedlist contains a loop, if so tell the starting node
To find the second highest number in an array and detect if a linked list contains a loop and identify the starting node.
To find the second highest number in an array, sort the array in descending order and return the second element.
To detect a loop in a linked list, use Floyd's Cycle Detection Algorithm by having two pointers, one moving twice as fast as the other. If they meet, there is a loop. To find the starting node, reset one pointer to the head and move both pointers ...read more
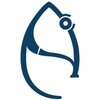
Asked in Appgenix Infotech LLP

Q. What is the difference between useState() and useRef()?
useState() is used to manage state in functional components, while useRef() is used to persist values between renders.
useState() re-renders the component when the state changes, useRef() does not trigger a re-render.
useState() returns a pair: the current state value and a function that lets you update it, useRef() returns a mutable ref object.
useState() is used for managing component state, useRef() is used for accessing DOM nodes or persisting values between renders.
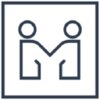
Asked in Memorres Digital

Q. How would you define that ReactJS is good for UI development?
ReactJS is good for UI development due to its component-based architecture, virtual DOM, and reusable code.
ReactJS uses a component-based architecture which allows for reusability and easier maintenance of UI elements.
Virtual DOM in ReactJS helps in optimizing performance by only updating the necessary parts of the UI when data changes.
ReactJS promotes the use of reusable code through components, making it easier to build complex UIs with consistent design patterns.
ReactJS ha...read more
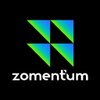
Asked in Zomentum

Q. Implement a Tic-Tac-Toe game using a JavaScript framework (e.g., React).
A tic-tac-toe game built using React framework in JavaScript.
Create a board component to display the game grid.
Implement logic to handle player moves and check for win conditions.
Use state management to update the game state.
Consider adding features like a reset button or keeping track of player scores.
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
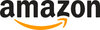
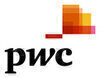
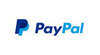
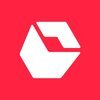
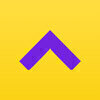
Top Interview Questions for Front end Engineer Related Skills
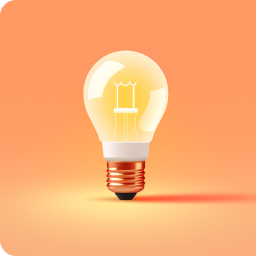
Calculate your in-hand salary
Confused about how your in-hand salary is calculated? Enter your annual salary (CTC) and get your in-hand salary
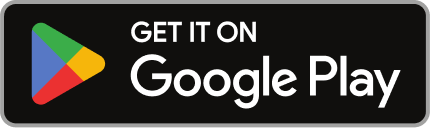
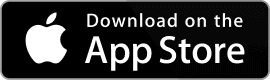
Reviews
Interviews
Salaries
Users
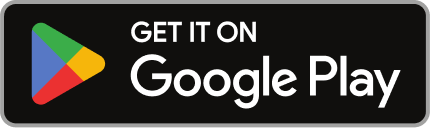
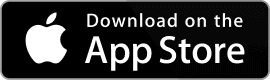