Associate Software Engineer
1500+ Associate Software Engineer Interview Questions and Answers
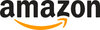
Asked in Amazon

Q. Infix to Postfix Conversion
You are provided with a string EXP
which represents a valid infix expression. Your task is to convert this given infix expression into a postfix expression.
Explanation:
An infix exp...read more
Convert a given infix expression to postfix expression.
Use a stack to keep track of operators and operands.
Follow the rules of precedence for operators (*, / have higher precedence than +, -).
Handle parentheses by pushing them onto the stack and popping when closing parenthesis is encountered.
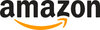
Asked in Amazon

Q. Minimum Direction Changes Problem Statement
Given a 2D grid with 'N' rows and 'M' columns, where each cell contains a character from the set { 'U', 'L', 'D', 'R' } indicating the allowed direction to move to a ...read more
The problem involves finding the minimum number of direction changes needed to create a valid path from the top-left to the bottom-right corner of a 2D grid.
Create a graph representation of the grid with directions as edges.
Use a shortest path algorithm like Dijkstra or BFS to find the minimum number of changes needed.
Handle edge cases like unreachable bottom-right corner or invalid input.
Consider implementing a helper function to convert directions to movements for easier tr...read more
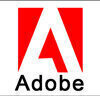
Asked in Adobe

Q. Tiling Problem Statement
Given a board with 2 rows and N columns, and an infinite supply of 2x1 tiles, determine the number of distinct ways to completely cover the board using these tiles.
You can place each t...read more
The problem involves finding the number of distinct ways to completely cover a 2xN board using 2x1 tiles.
Use dynamic programming to solve the tiling problem efficiently.
Define a recursive function to calculate the number of ways to tile the board.
Consider both horizontal and vertical placements of tiles.
Implement the function to handle large values of N by using modulo arithmetic.
Optimize the solution to avoid redundant calculations.
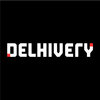
Asked in Delhivery

Q. Decode String Problem Statement
Your task is to decode a given encoded string back to its original form.
Explanation:
An encoded string format is <count>[encoded_string], where the 'encoded_string' inside the s...read more
The task is to decode an encoded string back to its original form by repeating the encoded string 'count' times.
Parse the input string to extract the count and the encoded string within the brackets
Use recursion to decode the encoded string by repeating it 'count' times
Handle nested encoded strings by recursively decoding them
Output the decoded string for each test case
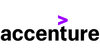
Asked in Accenture

Q. Find Middle of Linked List
Given the head node of a singly linked list, your task is to return a pointer pointing to the middle node of the linked list.
When the number of elements is odd, return the middle ele...read more
Return the middle node of a singly linked list, selecting the one farther from the head node in case of even number of elements.
Traverse the linked list using two pointers, one moving twice as fast as the other.
When the fast pointer reaches the end, the slow pointer will be at the middle node.
Return the node pointed by the slow pointer as the middle node.
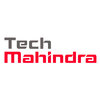
Asked in Tech Mahindra

Q. Can you call the base class method without creating an instance?
Yes, by using the super() method in the derived class.
super() method calls the base class method
Derived class must inherit from the base class
Example: class Derived(Base): def method(self): super().method()
Associate Software Engineer Jobs
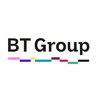
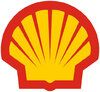
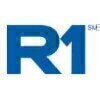
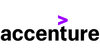
Asked in Accenture

Q. Find the sum of all numbers in the range from 1 to m (inclusive) that are not divisible by n. Return the difference between the sum of integers not divisible by n and the sum of numbers divisible by n.
Find sum of numbers in range 1 to m (both inclusive) not divisible by n. Return difference between sum of non-divisible and divisible numbers.
Iterate through range 1 to m and check if number is divisible by n.
If not divisible, add to sum of non-divisible numbers.
If divisible, add to sum of divisible numbers.
Return difference between sum of non-divisible and divisible numbers.
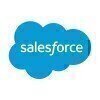
Asked in Salesforce

Q. Longest Path In Directed Graph Problem Statement
Given a Weighted Directed Acyclic Graph (DAG) comprising 'N' nodes and 'E' directed edges, where nodes are numbered from 0 to N-1, and a source node 'Src'. Your ...read more
The task is to find the longest distances from a source node to all nodes in a weighted directed acyclic graph.
Implement a function that takes the number of nodes, edges, source node, and edge weights as input.
Use a topological sorting algorithm to traverse the graph and calculate the longest distances.
Return an array of integers where each element represents the longest distance from the source node to the corresponding node.
Share interview questions and help millions of jobseekers 🌟
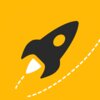
Asked in Cuemath

Q. N-th Term Of Geometric Progression
Find the N-th term of a Geometric Progression (GP) series given the first term A, the common ratio R, and the term position N.
Explanation:
The general form of a GP series is ...read more
Calculate the N-th term of a Geometric Progression series given the first term, common ratio, and term position.
Iterate through each test case and apply the formula A * R^(N-1) to find the N-th term
Use modular arithmetic to handle large calculated terms by returning the result modulo 10^9 + 7
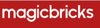
Asked in MagicBricks

Q. Ninja and Substrings Problem Statement
Ninja has to determine all the distinct substrings of size two that can be formed from a given string 'STR' comprising only lowercase alphabetic characters. These substrin...read more
The task is to find all the different possible substrings of size two that appear in a given string as contiguous substrings.
Iterate through the string and extract substrings of size two
Store the substrings in an array
Return the array of substrings
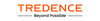
Asked in Tredence

Q. Find First Repeated Character in a String
Given a string 'STR' composed of lowercase English letters, identify the character that repeats first in terms of its initial occurrence.
Example:
Input:
STR = "abccba"...read more
The task is to find the first repeated character in a given string of lowercase English letters.
Iterate through the string and keep track of characters seen so far in a set.
If a character is already in the set, return it as the first repeated character.
If no repeated character is found, return '%'.
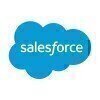
Asked in Salesforce

Q. Largest BST Subtree Problem
Given a binary tree with 'N' nodes, determine the size of the largest subtree that is also a BST (Binary Search Tree).
Input:
The first line contains an integer 'T', representing the...read more
The problem involves finding the size of the largest subtree that is also a Binary Search Tree in a given binary tree.
Traverse the binary tree in a bottom-up manner to check if each subtree is a BST.
Keep track of the size of the largest BST subtree encountered so far.
Use recursion to solve the problem efficiently.
Consider edge cases like empty tree or single node tree.
Example: For input 1 2 3 4 -1 5 6 -1 7 -1 -1 -1 -1 -1 -1, the largest BST subtree has size 3.
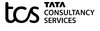
Asked in TCS

Q. Prime Time Again Problem Statement
You are given two integers DAY_HOURS
and PARTS
. Consider a day with DAY_HOURS
hours, which can be divided into PARTS
equal parts. Your task is to determine the total instances...read more
Calculate total instances of equivalent prime groups in a day divided into equal parts.
Divide the day into equal parts and find prime numbers in each part.
Identify prime groups where prime numbers occur at the same position in different parts.
Return the total number of equivalent prime groups found.
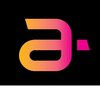
Asked in Amdocs

Q. First Unique Character in a String Problem Statement
Given a string STR
consisting of lowercase English letters, identify the first non-repeating character in the string and return it. If no such character exis...read more
Identify the first non-repeating character in a given string and return it, or '#' if none exists.
Iterate through the string to count the frequency of each character
Iterate through the string again to find the first character with frequency 1
Return the first non-repeating character or '#' if none exists
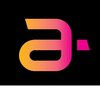
Asked in Amdocs

Q. Implement Atoi Function
You have a string 'STR' of length 'N'. Your task is to implement the atoi function, which converts the string into an integer. If the string does not contain any numbers, the function sh...read more
Implement a function to convert a string to an integer, ignoring non-numeric characters and considering negative numbers.
Iterate through the characters of the string and check if they are numeric.
Handle negative numbers by checking the first character of the string.
Ignore non-numeric characters while converting the string to an integer.
Return 0 if there are no numbers in the string.
Ensure the resulting number is within the given constraints.
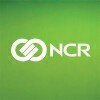
Asked in NCR Corporation

Q. Job Sequencing Problem Statement
You are provided with a N x 2 2-D array called Jobs
consisting of N
jobs. In this array, Jobs[i][0]
represents the deadline of the i-th job, while Jobs[i][1]
indicates the profi...read more
The objective is to determine the maximum profit by scheduling jobs within their deadlines.
Sort the jobs array based on the profit in descending order.
Iterate through the sorted array and schedule jobs based on their deadlines.
Keep track of the maximum profit achieved by scheduling jobs within their deadlines.
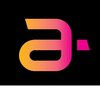
Asked in Amdocs

Q. Palindrome String Validation
Determine if a given string 'S' is a palindrome, considering only alphanumeric characters and ignoring spaces and symbols.
Note:
The string 'S' should be evaluated in a case-insensi...read more
Check if a given string is a palindrome after removing special characters, spaces, and converting to lowercase.
Remove special characters and spaces from the string
Convert the string to lowercase
Check if the modified string is a palindrome by comparing characters from start and end
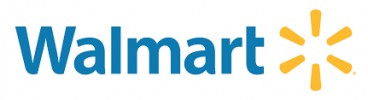
Asked in Walmart

Q. Bipartite Graph Problem Statement
Determine if a given graph is bipartite. A graph is bipartite if its vertices can be divided into two independent sets, 'U' and 'V', such that every edge ('u', 'v') connects a ...read more
The function checks whether a given graph is bipartite or not.
A bipartite graph can be divided into two independent sets such that every edge connects a vertex from one set to the other.
We can use graph coloring algorithm to check if the graph is bipartite.
Start by coloring the first vertex with one color and all its neighbors with the other color.
Continue coloring the remaining vertices, making sure that no adjacent vertices have the same color.
If at any point, we find that ...read more
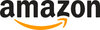
Asked in Amazon

Q. Minimum Number of Platforms Needed Problem Statement
You are given the arrival and departure times of N trains at a railway station for a particular day. Your task is to determine the minimum number of platform...read more
The task is to determine the minimum number of platforms needed at a railway station based on arrival and departure times of trains.
Sort the arrival and departure times in ascending order.
Use two pointers to keep track of overlapping schedules.
Increment platform count when a new train arrives before the previous one departs.
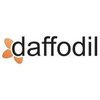
Asked in Daffodil Software

Q. Find the Longest Palindromic Substring
Given a string ‘S’ composed of lowercase English letters, your task is to identify the longest palindromic substring within ‘S’.
If there are multiple longest palindromic ...read more
Find the longest palindromic substring in a given string, returning the rightmost one if multiple substrings are of the same length.
Iterate through each character in the string and expand around it to check for palindromes
Keep track of the longest palindromic substring found so far
Return the rightmost longest palindromic substring
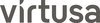
Asked in Virtusa Consulting Services

Number and Digits Problem Statement
You are provided with a positive integer N
. Your task is to identify all numbers such that the sum of the number and its digits equals N
.
Example:
Input:
N = 21
Output:
[15]
Identify numbers where sum of number and its digits equals given integer N.
Iterate through numbers from 1 to N and check if sum of number and its digits equals N
Use a helper function to calculate sum of digits for a given number
Return the numbers that satisfy the condition in increasing order, else return -1
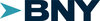
Asked in BNY

Q. Palindromic Substrings Problem Statement
You are given a string 'STR'. Your task is to determine the total number of palindromic substrings present in 'STR'.
Example:
Input:
"abbc"
Output:
5
Explanation:
The pa...read more
Count the total number of palindromic substrings in a given string.
Iterate through each character in the string and expand around it to find palindromic substrings.
Use dynamic programming to store the results of subproblems to avoid redundant calculations.
Consider both odd-length and even-length palindromes while counting.
Example: For input 'abbc', the palindromic substrings are ['a', 'b', 'b', 'c', 'bb'], totaling 5.
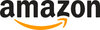
Asked in Amazon

Q. Spiral Order Traversal of a Binary Tree
Given a binary tree with N
nodes, your task is to output the Spiral Order traversal of the binary tree.
Input:
The input consists of a single line containing elements of ...read more
Implement a function to return the spiral order traversal of a binary tree.
Traverse the binary tree in a spiral order, alternating between left to right and right to left.
Use a queue to keep track of nodes at each level and a stack to reverse the order when necessary.
Handle null nodes appropriately to maintain the spiral order traversal.
Example: For input 1 2 3 -1 -1 4 5, the output should be 1 3 2 4 5.
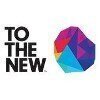
Asked in TO THE NEW

Q. Word Occurrence Counting
Given a string 'S' of words, the goal is to determine the frequency of each word in the string. Consider a word as a sequence of one or more non-space characters. The string can have mu...read more
Count the frequency of each word in a given string.
Split the input string by spaces to get individual words.
Use a hashmap to store the frequency of each word.
Iterate through the words and update the hashmap accordingly.
Print the unique words and their frequencies from the hashmap.
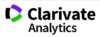
Asked in Clarivate

Abstract classes and interfaces in inheritance allow for defining common behavior and function signatures, while function overloading and overriding enable polymorphism.
Abstract classes are classes that cannot be instantiated and may contain abstract methods that must be implemented by subclasses.
Interfaces are similar to abstract classes but can only contain method signatures and constants, with no method implementations.
Function overloading refers to having multiple methods...read more
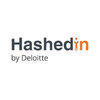
Asked in HashedIn by Deloitte

Q. Detect and Remove Loop in Linked List
For a given singly linked list, identify if a loop exists and remove it, adjusting the linked list in place. Return the modified linked list.
Expected Complexity:
Aim for a...read more
Detect and remove loop in a singly linked list in place with O(n) time complexity and O(1) space complexity.
Use Floyd's Cycle Detection Algorithm to identify the loop in the linked list.
Once the loop is detected, use two pointers approach to find the start of the loop.
Adjust the pointers to remove the loop and return the modified linked list.
Example: For input 5 2 and 1 2 3 4 5, output should be 1 2 3 4 5.
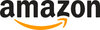
Asked in Amazon

Q. Implementing a Priority Queue Using Heap
Ninja has been tasked with implementing a priority queue using a heap data structure. However, he is currently busy preparing for a tournament and has requested your ass...read more
Implement a priority queue using a heap data structure by completing the provided functions: push(), pop(), getMaxElement(), and isEmpty().
Understand the operations: push() to insert element, pop() to remove largest element, getMaxElement() to return largest element, and isEmpty() to check if queue is empty.
Implement a heap data structure to maintain the priority queue.
Handle different types of queries based on the input provided.
Ensure correct output for each type 3 query.
Te...read more
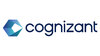
Asked in Cognizant

Q. Nth Fibonacci Number Problem Statement
Calculate the Nth term in the Fibonacci sequence, where the sequence is defined as follows: F(n) = F(n-1) + F(n-2)
, with initial conditions F(1) = F(2) = 1
.
Input:
The inp...read more
Calculate the Nth Fibonacci number efficiently using dynamic programming.
Use dynamic programming to store previously calculated Fibonacci numbers to avoid redundant calculations.
Start with base cases F(1) and F(2) as 1, then iterate to calculate subsequent Fibonacci numbers.
Return the Nth Fibonacci number as the final result.
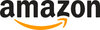
Asked in Amazon

Q. Power Set Generation
Given a sorted array of 'N' integers, your task is to generate the power set for this array. Each subset of this power set should be individually sorted.
A power set of a set 'ARR' is the s...read more
Generate power set of a sorted array of integers with individually sorted subsets.
Iterate through all possible combinations using bitwise operations.
Sort each subset before adding it to the power set.
Handle empty subset separately.
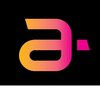
Asked in Amdocs

Q. Pythagorean Triplets Detection
Determine if an array contains a Pythagorean triplet by checking whether there are three integers x, y, and z such that x2 + y2 = z2 within the array.
Input:
The first line contai...read more
Detect if an array contains a Pythagorean triplet by checking if there are three integers x, y, and z such that x^2 + y^2 = z^2.
Iterate through all possible combinations of three integers in the array and check if x^2 + y^2 = z^2.
Use a nested loop to generate all possible combinations efficiently.
Return 'yes' if a Pythagorean triplet is found, otherwise return 'no'.
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
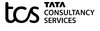
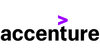
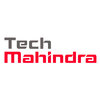
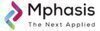
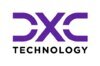
Top Interview Questions for Associate Software Engineer Related Skills
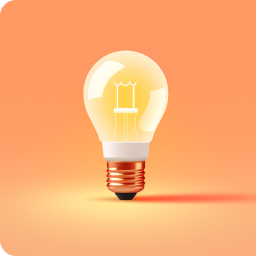
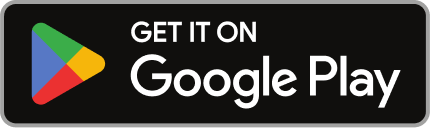
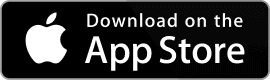
Reviews
Interviews
Salaries
Users
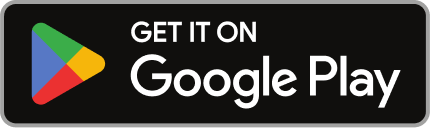
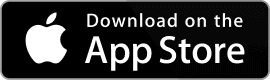