Software Developer
1500+ Software Developer Interview Questions and Answers for Freshers
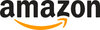
Asked in Amazon

Q. Maximum Subarray Sum Problem Statement
Given an array of integers, determine the maximum possible sum of any contiguous subarray within the array.
Example:
Input:
array = [34, -50, 42, 14, -5, 86]
Output:
137
E...read more
Find the maximum sum of any contiguous subarray within an array of integers.
Iterate through the array and keep track of the maximum sum of subarrays encountered so far.
At each index, decide whether to include the current element in the subarray or start a new subarray.
Use Kadane's algorithm to efficiently find the maximum subarray sum in O(N) time complexity.
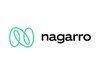
Asked in Nagarro

Q. Crazy Numbers Pattern Challenge
Ninja enjoys arranging numbers in a sequence. He plans to arrange them in 'N' rows such that:
- The first row contains 1 number.
- The second row contains 2 numbers.
- The third row c...read more
Arrange numbers in a sequence in 'N' rows with increasing order and repeating after 9.
Iterate through each test case and for each row, print numbers in increasing order with a loop.
Keep track of the current number to print and reset to 1 after reaching 9.
Handle formatting to align numbers correctly in each row.
Ensure to print the correct number of rows based on the input 'N'.
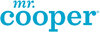
Asked in Mr Cooper

Q. Connect Ropes Problem Statement
Given a number of ropes denoted as 'N' and an array containing the lengths of these ropes, your task is to connect the ropes into one single rope. The cost to connect two ropes i...read more
The task is to find the minimum cost required to connect all the ropes by summing their lengths.
Iterate through the ropes and connect the two shortest ropes at each step to minimize cost
Use a priority queue to efficiently find the shortest ropes
Keep track of the total cost as you connect the ropes
Example: For input [4, 3, 2, 6], connect 2 and 3 (cost 5), then connect 4 and 5 (cost 9), then connect 9 and 6 (cost 15) for a total cost of 29

Asked in IQVIA

Q. Palindromic Numbers Finder
Given an integer 'N', your task is to identify all palindromic numbers from 1 to 'N'. These are numbers that read the same way forwards and backwards.
Input:
The first line provides a...read more
Implement a function to find all palindromic numbers from 1 to N.
Iterate from 1 to N and check if each number is a palindrome
Use string manipulation to check for palindromes
Consider edge cases like single-digit numbers and 11
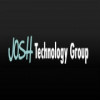
Asked in Josh Technology Group

Q. Validate Binary Tree Nodes Problem
You are provided with 'N' binary tree nodes labeled from 0 to N-1, where node i has two potential children, recorded in the LEFT_CHILD[i]
and RIGHT_CHILD[i]
arrays. Determine ...read more
The task is to determine if the given binary tree nodes form exactly one valid binary tree.
Check if there is only one root node (a node with no parent)
Check if each node has at most one parent
Check if there are no cycles in the tree
Check if all nodes are connected and form a single tree
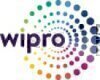
Asked in Wipro

Q. Minimum Operations to Make Strings Equal
Given two strings, A
and B
, consisting of lowercase English letters, determine the minimum number of pre-processing moves required to make string A
equal to string B
usi...read more
Determine the minimum number of pre-processing moves required to make two strings equal by swapping characters and replacing characters in one string.
Iterate through both strings simultaneously and count the number of characters that need to be swapped.
Consider all possible swaps and replacements to find the minimum number of pre-processing moves.
If the lengths of the strings are different, it's impossible to make them equal.
Handle edge cases like empty strings or strings wit...read more
Software Developer Jobs

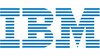
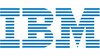
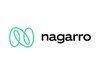
Asked in Nagarro

Q. Ninja and the New Year Guests Problem
Ninja has organized a new year party and wants to verify if the guests are programmers by challenging them with a coding task. As an invited programmer, you're tasked to so...read more
Compute the number of valid permutations of integers from 0 to N-1 such that at least K positions satisfy ARR[I] = I.
Use dynamic programming to solve the problem efficiently.
Consider the cases where K is equal to N or N-1 separately.
Modulo the result by 10^9 + 7 to avoid overflow issues.
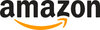
Asked in Amazon

Q. Sort 0s, 1s, and 2s Problem Statement
You are provided with an integer array/list ARR
of size 'N' which consists solely of 0s, 1s, and 2s. Your task is to write a solution to sort this array/list.
Input:
The fi...read more
Sort an array of 0s, 1s, and 2s in linear time with a single scan approach.
Use three pointers to keep track of the positions of 0s, 1s, and 2s in the array.
Iterate through the array once and swap elements based on their values and the pointers.
After the single scan, the array will be sorted in place with 0s, 1s, and 2s in order.
Share interview questions and help millions of jobseekers 🌟
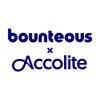
Asked in Bounteous x Accolite

Q. Maximum Subarray Problem Statement
Ninja has been given an array, and he wants to find a subarray such that the sum of all elements in the subarray is maximum.
A subarray 'A' is considered greater than a subarr...read more
The task is to find the subarray with the maximum sum in a given array.
Iterate through the array and keep track of the current sum and maximum sum seen so far.
If the current sum becomes negative, reset it to 0 as it won't contribute to the maximum sum.
Compare the maximum sum with the sum of the current subarray to update the result.
Handle cases where all elements are negative by returning the maximum element in the array.
Consider edge cases like empty array or array with only...read more
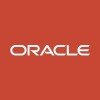
Asked in Oracle

Q. Greatest Common Divisor Problem Statement
You are tasked with finding the greatest common divisor (GCD) of two given numbers 'X' and 'Y'. The GCD is defined as the largest integer that divides both of the given...read more
Find the greatest common divisor (GCD) of two given numbers 'X' and 'Y'.
Iterate from 1 to the minimum of X and Y, check if both X and Y are divisible by the current number, update GCD if true
Use Euclidean algorithm to find GCD: GCD(X, Y) = GCD(Y, X % Y)
If one of the numbers is 0, the other number is the GCD
Handle edge cases like when one of the numbers is 0 or negative
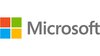
Asked in Microsoft Corporation

Q. Buses Origin Problem Statement
You have been provided with an array where each element specifies the number of buses that can be boarded at each respective bus stop. Buses will only stop at locations that are m...read more
Given an array representing number of buses at each bus stop, determine how many buses originate from each stop.
Iterate through the array and for each element, increment the count of buses originating from the corresponding bus stop.
Use an array to store the count of buses originating from each stop.
Remember to consider the constraint of 1-based indexing for bus stops.
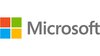
Asked in Microsoft Corporation

Q. Find K'th Character of Decrypted String
You are given an encrypted string where repeated substrings are represented by the substring followed by its count. Your task is to find the K'th character of the decrypt...read more
Given an encrypted string with repeated substrings represented by counts, find the K'th character of the decrypted string.
Parse the encrypted string to extract substrings and their counts
Iterate through the substrings and counts to build the decrypted string
Track the position in the decrypted string to find the K'th character
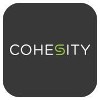
Asked in Cohesity

Q. Balanced Sequence After Replacement
Given a string of length 'N' containing only the characters: '[', '{', '(', ')', '}', ']'. At certain places, the character 'X' appears in place of any bracket. Your goal is ...read more
Determine if a valid balanced sequence can be achieved by replacing 'X's with suitable brackets.
Iterate through the string and keep track of the count of opening and closing brackets.
If at any point the count of closing brackets exceeds the count of opening brackets, return False.
If all 'X's can be replaced to form a valid balanced sequence, return True.
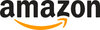
Asked in Amazon

Q. Flip Bits Problem Explanation
Given an array of integers ARR
of size N, consisting of 0s and 1s, you need to select a sub-array and flip its bits. Your task is to return the maximum count of 1s that can be obta...read more
Maximize the count of 1s in a binary array by flipping a sub-array of 0s to 1s at most once.
Initial Count: Start by counting the number of 1s in the original array.
Flipping Logic: For each sub-array, calculate the effect of flipping 0s to 1s and 1s to 0s.
Kadane's Algorithm: Use a modified version of Kadane's algorithm to find the maximum gain from flipping a sub-array.
Edge Cases: Consider cases where the array is all 1s or all 0s.
Example: For ARR = [1, 0, 0, 1], flipping the ...read more
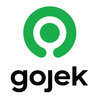
Asked in Go-Jek

Q. Beautiful String Problem Statement
Given a binary string STR
containing either '0' or '1', determine the minimum number of operations needed to make it beautiful. A binary string is called beautiful if it conta...read more
The problem involves determining the minimum number of operations needed to make a binary string beautiful by ensuring it contains alternating 0s and 1s.
Iterate through the binary string and count the number of operations needed to make it beautiful by flipping the bits as required.
Keep track of the current bit and compare it with the next bit to determine if a flip operation is needed.
Return the total number of operations needed for each test case.
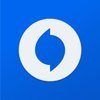
Asked in JUSPAY

Q. Largest Cycle in Maze Problem Statement
Given a maze represented by 'N' cells numbered from 0 to N-1, and an array arr
of 'N' integers where arr[i]
denotes the cell number that can be reached from the 'i'-th ce...read more
Identify the length of the largest cycle in a maze represented by cells and an array of integers.
Iterate through each cell and find the cycle length using DFS or Floyd's Tortoise and Hare algorithm.
Handle self-cycles and cells with no exit by checking arr[i] = i and arr[i] = -1 respectively.
Output the length of the largest cycle found or -1 if no cycles exist.
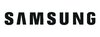
Asked in Samsung

Q. Reverse Linked List Problem Statement
Given a singly linked list of integers, return the head of the reversed linked list.
Example:
Initial linked list: 1 -> 2 -> 3 -> 4 -> NULL
Reversed linked list: 4 -> 3 -> 2...read more
Reverse a singly linked list of integers and return the head of the reversed linked list.
Iterate through the linked list and reverse the pointers to point to the previous node instead of the next node.
Use three pointers to keep track of the current, previous, and next nodes while reversing the linked list.
Update the head of the reversed linked list as the last node encountered during the reversal process.
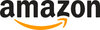
Asked in Amazon

Q. Find Duplicate in Array Problem Statement
You are provided with an array of integers 'ARR' consisting of 'N' elements. Each integer is within the range [1, N-1], and the array contains exactly one duplicated el...read more
The task is to find the duplicate element in an array of integers.
Iterate through the array and keep track of the frequency of each element using a hash map.
Return the element with a frequency greater than 1.
Alternatively, sort the array and check for adjacent elements with the same value.

Asked in Visa

Q. Maximum Equal Elements After K Operations
You are provided with an array/list of integers named 'ARR' of size 'N' and an integer 'K'. Your task is to determine the maximum number of elements that can be made eq...read more
Determine the maximum number of elements that can be made equal by performing at most K operations on an array of integers.
Sort the array in non-decreasing order to easily identify the elements that need to be increased
Calculate the difference between adjacent elements to determine the number of operations needed to make them equal
Keep track of the total number of operations used and the maximum number of elements that can be made equal
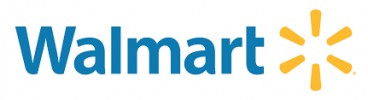
Asked in Walmart

Q. Maximum Frequency Number Problem Statement
Given an array of integers with numbers in random order, write a program to find and return the number which appears the most frequently in the array.
If multiple elem...read more
Find the number with the highest frequency in an array, returning the first one in case of ties.
Count Frequencies: Use a hash map to count occurrences of each number in the array.
Track First Occurrence: Store the index of the first occurrence of each number to resolve ties.
Iterate Efficiently: Loop through the array once to build the frequency map and another pass to determine the maximum frequency.
Example: For input [1, 2, 3, 1, 2], the output is 1 since it appears first wit...read more
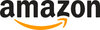
Asked in Amazon

Q. Permutation in String Problem Statement
Determine if the string str2
contains a permutation of the string str1
as one of its substrings.
Input:
The first line contains an integer 'T', representing the number of...read more
Check if a permutation of one string is a substring of another string.
Create a frequency map of characters in str1.
Iterate through str2 with a window of size N and check if the frequency map matches.
Return 'Yes' if a permutation is found, 'No' otherwise.
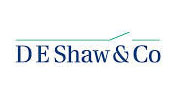
Asked in DE Shaw

Q. Tower Building Problem Statement
Given an array 'ARR' of 'N' cubes, you need to construct towers such that each cube can either be placed on top of an existing tower or start a new one. The restriction is that ...read more
The task is to determine the minimum number of towers needed to stack cubes in a specific order.
Iterate through the array of cubes and maintain a stack to keep track of towers.
For each cube, check if it can be placed on an existing tower or start a new one.
Update the stack based on the cube's size and count the number of towers needed.
Return the minimum number of towers required.
Example: For input N = 3, ARR = [3, 2, 1], the output should be 1.
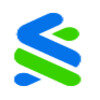
Asked in Standard Chartered

Q. Ways To Make Coin Change
Given an infinite supply of coins of varying denominations, determine the total number of ways to make change for a specified value using these coins. If it's not possible to make the c...read more
The task is to find the total number of ways to make change for a specified value using given denominations.
Use dynamic programming to solve this problem efficiently.
Create a 1D array to store the number of ways to make change for each value from 0 to the target value.
Iterate through the denominations and update the array based on the current denomination.
The final answer will be the value at the target index of the array.
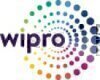
Asked in Wipro

Q. Linear Search Problem Statement
Given a random integer array/list ARR
of size N
, and an integer X
, you are required to search for the integer X
in the given array/list using Linear Search.
Return the index at w...read more
Linear search algorithm to find the first occurrence of an integer in an array.
Iterate through the array and compare each element with the target integer.
Return the index if the target integer is found, else return -1.
Time complexity is O(N) where N is the size of the array.
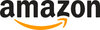
Asked in Amazon

Q. Alien Dictionary Problem Statement
You are provided with a sorted dictionary (by lexical order) in an alien language. Your task is to determine the character order of the alien language from this dictionary. Th...read more
Determine the character order of an alien language from a sorted dictionary of words.
Graph Representation: Treat each character as a node and establish directed edges based on the order derived from adjacent words.
Topological Sorting: Use topological sorting to determine the order of characters, ensuring that for every directed edge from character A to B, A comes before B.
Example: For words ['caa', 'aaa', 'aab'], the order derived is 'c' -> 'a' -> 'b'.
Handling Edge Cases: Con...read more
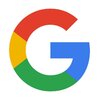
Asked in Google

Q. There are three rooms labeled Princess, Flowers, and Snake, but all the labels are incorrect. How can you identify the room containing the Princess without entering the room labeled Snake?
Identify the Princess's room by using the incorrect nameplates without entering the Snake's room.
Each room has a nameplate that is incorrect.
If you check the room labeled 'Flowers', it cannot contain flowers.
Since the room labeled 'Flowers' cannot have flowers, it must either have the Princess or the Snake.
If the room labeled 'Flowers' has the Princess, then the room labeled 'Princess' must have the Snake.
If the room labeled 'Flowers' has the Snake, then the room labeled 'Pri...read more
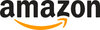
Asked in Amazon

Q. Biggest Number Formation Problem
Your task is to construct the largest number possible by concatenating each of the provided positive integers in the array exactly once.
Input:
Integer T denoting the number of ...read more
Construct the largest number by concatenating positive integers in the array exactly once.
Sort the array of integers in a way that the concatenation of the numbers forms the largest possible number.
Use a custom comparator function to sort the numbers based on their concatenated values.
Join the sorted array elements to form the final largest number.
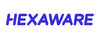
Asked in Hexaware Technologies

Q. Ninja Competition Problem Statement
Ninja is organizing a coding competition where two teams compete at a time. To keep it fair and interesting, both teams must have an equal number of members. Ninja’s task is ...read more
Check if Ninja can create two teams with equal members given an integer N and its divisors.
Iterate through all divisors of N and assign members to the first or second team based on whether the divisor is even or odd.
Keep track of the total members in each team and check if they are equal at the end.
Return true if the total members in both teams are equal, false otherwise.
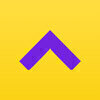
Asked in Housing.com

Q. Painter's Partition Problem Statement
Given an array/list representing boards, where each element denotes the length of a board, and a number ‘K’ of available painters, determine the minimum time required to pa...read more
Determine the minimum time required to paint all boards with given constraints.
Use binary search to find the minimum and maximum possible time to paint all boards.
Iterate through the boards and assign them to painters based on the time constraints.
Calculate the total time taken to paint all boards with the assigned painters.
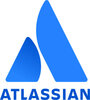
Asked in Atlassian

Q. Structurally Unique Binary Trees of Dragon Balls
Goku has ‘N’ Dragon Balls, where each Dragon Ball is unique. The ith Dragon Ball has ‘i’ stars on it, meaning the first Dragon Ball has 1 star, the second has 2 ...read more
Count the number of structurally unique binary trees that can be constructed with given Dragon Balls.
Use dynamic programming to solve this problem efficiently.
The number of structurally unique binary trees can be calculated using Catalan numbers.
For each test case, calculate the number of structurally unique binary trees modulo 10^9 + 7.
Return the count of unique binary trees for each test case.
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
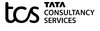
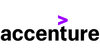
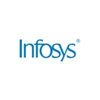
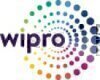
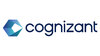
Top Interview Questions for Software Developer Related Skills
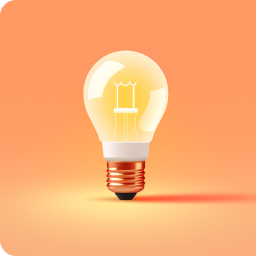
Calculate your in-hand salary
Confused about how your in-hand salary is calculated? Enter your annual salary (CTC) and get your in-hand salary
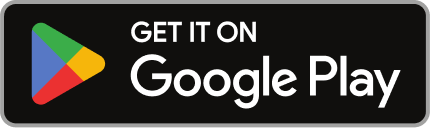
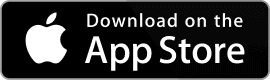
Reviews
Interviews
Salaries
Users
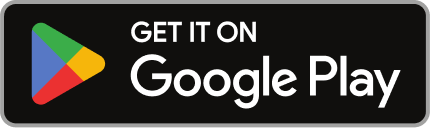
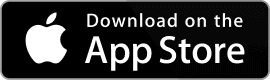