Senior PHP Developer
60+ Senior PHP Developer Interview Questions and Answers
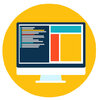
Asked in Neoistone

Q. What are the differences between a function and a method?
Functions are standalone blocks of code while methods are functions that belong to a class.
Functions are defined outside of a class while methods are defined inside a class.
Functions can be called directly while methods are called on an object of a class.
Methods have access to the data and properties of the class while functions do not.
Example of a function: function addNumbers($a, $b) { return $a + $b; }
Example of a method: class Calculator { public function addNumbers($a, $...read more
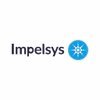
Asked in Impelsys

Q. How do you retrieve an image (BLOB) from a database and display it in a PHP view page?
To display an image from a database in a PHP view page, retrieve the image as a blob and output it with appropriate headers.
Retrieve the image data from the database as a blob.
Set the appropriate content-type header for the image format.
Output the image data to the response body.
Senior PHP Developer Interview Questions and Answers for Freshers
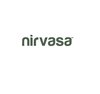
Asked in Nirvasa Healthcare

Q. What is the difference between GROUP BY and DISTINCT functions?
Group by is used to group rows based on a column, while distinct function is used to remove duplicate values from a column.
Group by is used with aggregate functions like count, sum, avg, etc.
Distinct function is used to retrieve unique values from a column.
Group by can be used with multiple columns to create groups based on multiple criteria.
Distinct function can be used with multiple columns to retrieve unique combinations of values.
Group by is often used in conjunction with...read more
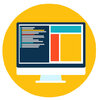
Asked in Neoistone

Q. Why did you select PHP for web development?
PHP is selected for web development due to its ease of use, flexibility, and large community support.
PHP is easy to learn and use, making it accessible for beginners
PHP is flexible and can be used for a variety of web development tasks
PHP has a large community of developers who contribute to its development and provide support
PHP is open source and free to use, making it a cost-effective option for web development
PHP integrates well with other technologies, such as databases ...read more
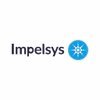
Asked in Impelsys

Q. Write a PHP program to print stars in a pyramid shape.
PHP program to print *(stars) in pyramid shape
Use nested for loops to print the pyramid shape
The outer loop controls the number of rows
The inner loop controls the number of stars to print in each row
Use a combination of spaces and stars to create the pyramid shape
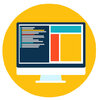
Asked in Neoistone

Q. Explain the difference between $var and $$var.
The difference between $var and $$var is that $var is a variable with a fixed name, while $$var is a variable with a variable name.
The $var variable is a regular variable with a fixed name, while $$var is a variable with a variable name.
The $$var variable is also known as a variable variable.
The value of $$var is determined by the value of $var.
Example: $var = 'foo'; $$var = 'bar'; echo $foo; // Output: bar'
Senior PHP Developer Jobs
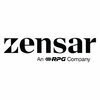
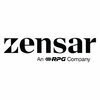
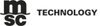
Asked in Duplex Technologies

Q. What is the process for building APIs for mobile app integration, and which security types should be utilized?
Building APIs for mobile apps involves design, development, and security measures to ensure safe data exchange.
Define API requirements: Identify the data and functionalities needed by the mobile app.
Choose the right architecture: RESTful APIs are commonly used for their simplicity and scalability.
Implement authentication: Use OAuth 2.0 for secure access control.
Data encryption: Utilize HTTPS to encrypt data in transit.
Rate limiting: Protect the API from abuse by limiting the ...read more
Asked in eDigital Infosolutions

Q. 1)PHP OOPs definition 2)difference between abstract class and interface 3)what is index in MySql 4)Difference between GROUP BY and HAVING clause in MySql
PHP OOPs is a programming paradigm that uses objects and classes to organize code. Abstract classes provide partial implementation, while interfaces provide a contract for classes to implement. Index in MySQL is a data structure that improves the speed of data retrieval. GROUP BY is used to group rows that have the same values, while HAVING is used to filter groups based on a specified condition.
PHP OOPs is a programming paradigm that uses objects and classes to organize code...read more
Share interview questions and help millions of jobseekers 🌟
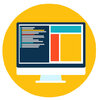
Asked in Neoistone

Q. What is the difference between variables and constants?
Variables can be changed while constants cannot be changed once defined.
Variables are used to store data that can be changed during program execution
Constants are used to store data that cannot be changed once defined
Variables are declared using the $ symbol while constants are declared using the define() function
Constants are useful for defining values that are used throughout the program and should not be changed
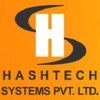
Asked in Hashtech Systems

Q. How would you fetch parent data from a database based on a searched name?
To fetch parents data from database whose name is searched.
Use SQL query to fetch data from database
Join parent table with child table using foreign key
Use WHERE clause to filter data based on searched name
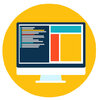
Asked in Neoistone

Q. What is Composer and why is it used?
Composer is a dependency manager for PHP that simplifies the process of installing and managing third-party libraries.
Composer allows developers to easily add external libraries to their PHP projects
It manages dependencies and ensures that all required libraries are installed and up-to-date
Composer uses a JSON file called 'composer.json' to define project dependencies
It also provides a command-line interface for installing, updating, and removing dependencies
Composer is widel...read more
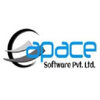
Asked in Capace Software

Q. What will be the output of the code below and why?
The code will output 'Hello World!' because the variable $x is set to true.
The code will check if $x is true, which it is, so it will output 'Hello World!'
The if statement will only execute if the condition is true, in this case $x is true.
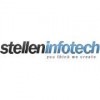
Asked in Stellen Infotech

Q. Difference between React Js and next js and which one is better for seo?
React.js is a library for building UIs, while Next.js is a framework for server-side rendering and static site generation.
React.js is primarily a JavaScript library for building user interfaces, focusing on the view layer.
Next.js is a framework built on top of React.js that enables server-side rendering (SSR) and static site generation (SSG).
Next.js improves SEO by allowing search engines to crawl fully rendered pages, unlike React.js which relies on client-side rendering.
Exa...read more
Asked in Duplex Technologies

Q. How can a payment gateway be integrated with high security measures?
Integrating a payment gateway securely involves encryption, compliance, and best practices to protect sensitive data.
Use HTTPS for secure communication between the client and server.
Implement PCI DSS compliance to ensure secure handling of cardholder data.
Utilize tokenization to replace sensitive card information with unique identifiers.
Incorporate 3D Secure for an additional layer of authentication during transactions.
Regularly update and patch your software to protect again...read more
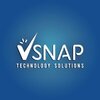
Asked in Vsnap Technology Solutions

Q. What is the process for performing CRUD operations using a PHP framework?
CRUD operations in PHP frameworks involve creating, reading, updating, and deleting data using MVC architecture.
1. **Setup Database Connection**: Use PDO or framework-specific methods to connect to the database.
2. **Create Operation**: Use POST requests to insert data. Example: `INSERT INTO users (name, email) VALUES (?, ?)`.
3. **Read Operation**: Use GET requests to retrieve data. Example: `SELECT * FROM users WHERE id = ?`.
4. **Update Operation**: Use PUT/PATCH requests to ...read more
Asked in Duplex Technologies

Q. What types of security measures should be implemented in the coding process?
Implementing security measures in PHP coding is crucial to protect applications from vulnerabilities and attacks.
Input Validation: Always validate and sanitize user inputs to prevent SQL injection and XSS attacks. Use functions like filter_var() for validation.
Prepared Statements: Use prepared statements with PDO or MySQLi to prevent SQL injection. Example: $stmt = $pdo->prepare('SELECT * FROM users WHERE id = ?');
Password Hashing: Store passwords securely using password_hash...read more
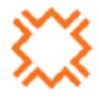
Asked in Wall Street Consulting Services

Q. What are traits in PHP? How are they different from interfaces?
Traits in PHP allow code reuse in classes, differing from interfaces which define method contracts without implementation.
Traits enable the inclusion of methods in multiple classes without inheritance.
Unlike interfaces, traits can contain method implementations.
Traits can be used to avoid code duplication across different classes.
Example: A trait 'Logger' can provide logging methods that can be used in multiple classes.
Interfaces only define method signatures, requiring imple...read more
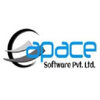
Asked in Capace Software

Q. What is the difference between variables and constants in PHP?
Variables can be changed during the execution of a script, while constants cannot be changed once they are defined.
Variables can be reassigned with new values, while constants cannot be changed once defined
Constants are defined using the define() function, while variables are assigned using the $ symbol
Constants are case-sensitive by default, while variables are case-insensitive
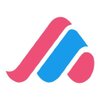
Asked in Appinop Technologies

Q. Tell me about some basic PHP functions you have used.
Some basic PHP functions include echo, strlen, substr, array_push, and file_get_contents.
echo - outputs one or more strings
strlen - returns the length of a string
substr - returns a part of a string
array_push - adds one or more elements to the end of an array
file_get_contents - reads the contents of a file into a string
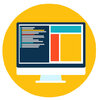
Asked in Neoistone

Q. What does PEAR stand for?
PEAR stands for PHP Extension and Application Repository.
PEAR is a framework and distribution system for reusable PHP components.
It provides a structured library of code, a system for package maintenance, and a standard for code style.
PEAR packages can be easily installed and managed using the PEAR command-line tool.
Examples of popular PEAR packages include PHPUnit, PHPMailer, and HTML_QuickForm.
PEAR is no longer actively maintained and has been superseded by Composer in mode...read more
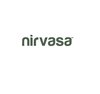
Asked in Nirvasa Healthcare

Q. What are the different storage engines?
Different storage engines are used in databases to manage data storage and retrieval efficiently.
InnoDB: ACID-compliant, supports transactions and foreign keys
MyISAM: Fast and efficient for read-heavy workloads, no transaction support
Memory: Stores data in memory for fast access, but data is lost on restart
CSV: Stores data in CSV files, suitable for importing and exporting data
Archive: Optimized for high compression, suitable for storing historical data
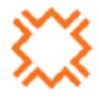
Asked in Wall Street Consulting Services

Q. How does PHP handle variable scope within functions?
PHP uses local and global scopes to manage variable visibility within functions, affecting accessibility and lifetime.
Variables declared inside a function are local to that function and cannot be accessed outside of it.
Global variables can be accessed inside a function using the 'global' keyword.
Example: function test() { $a = 5; } $a is not accessible outside test().
To access a global variable: global $a; inside the function.
PHP also supports static variables, which retain t...read more
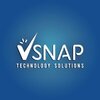
Asked in Vsnap Technology Solutions

Q. What are the steps involved in creating a static website?
Creating a static website involves planning, designing, coding, and deploying HTML, CSS, and JavaScript files.
1. Planning: Define the purpose and structure of the website, such as a portfolio or a blog.
2. Design: Create wireframes and mockups using tools like Figma or Adobe XD.
3. Development: Write HTML for structure, CSS for styling, and JavaScript for interactivity.
4. Testing: Check for responsiveness and browser compatibility using tools like BrowserStack.
5. Deployment: Ho...read more
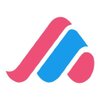
Asked in Appinop Technologies

Q. On which type of frameworks have you worked?
I have worked on various PHP frameworks including Laravel, CodeIgniter, and Symfony.
Laravel - used for building web applications with elegant syntax and features like routing, authentication, and ORM
CodeIgniter - lightweight framework with a small footprint and easy to learn
Symfony - used for building complex web applications with reusable components and best practices
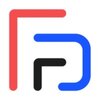
Asked in First Due

Q. What are the SOLID principles in software development?
SOLID principles are five design principles aimed at making software designs more understandable, flexible, and maintainable.
S - Single Responsibility Principle: A class should have one, and only one, reason to change. Example: A User class should only handle user data.
O - Open/Closed Principle: Software entities should be open for extension but closed for modification. Example: Use interfaces to add new features.
L - Liskov Substitution Principle: Subtypes must be substitutab...read more
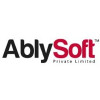
Asked in Ably Soft

Q. What is the purpose of interfaces?
Interfaces in PHP define a contract for classes to implement certain methods, allowing for polymorphism and code reusability.
Interfaces in PHP are used to define a set of methods that a class must implement.
They allow for polymorphism, where different classes can implement the same interface but have different implementations for the methods.
Interfaces promote code reusability by enforcing a common structure for classes that implement them.
Interfaces can be used to create API...read more
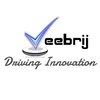
Asked in Veebrij

Q. What is TDD? What is use of it.
TDD stands for Test-Driven Development. It is a software development process where tests are written before the actual code.
Tests are written before writing the actual code
Helps in defining the requirements and design of the code
Ensures that the code meets the requirements and is working correctly
Improves code quality and maintainability
Examples: PHPUnit, Jest, Mocha
Asked in Idzyner Reality

Q. Have you developed any PHP applications in the past?
Yes, I have developed multiple PHP applications in the past.
Developed a custom e-commerce website using PHP, MySQL, and JavaScript
Created a content management system (CMS) using PHP and Laravel framework
Built a web application for tracking employee attendance using PHP and Bootstrap
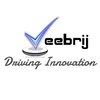
Asked in Veebrij

Q. What is lumen laravel? Why we use it.
Lumen Laravel is a lightweight micro-framework by Laravel for building fast and efficient APIs and microservices.
Built by Laravel for creating APIs and microservices
Lightweight and faster than Laravel
Ideal for small projects or when performance is crucial
Provides a subset of features compared to Laravel
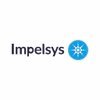
Asked in Impelsys

Q. Design patterns & Explain Them.
Design patterns are reusable solutions to common software development problems.
Design patterns provide proven solutions to recurring problems in software development.
They help in creating code that is more flexible, reusable, and maintainable.
Some common design patterns include Singleton, Factory, Observer, and Decorator.
Singleton pattern ensures that only one instance of a class is created.
Factory pattern provides a way to create objects without specifying the exact class of...read more
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
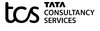
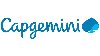
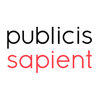
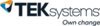
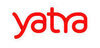
Top Interview Questions for Senior PHP Developer Related Skills
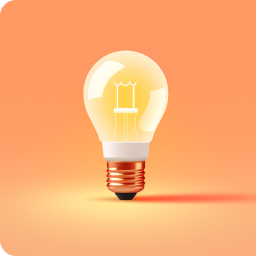
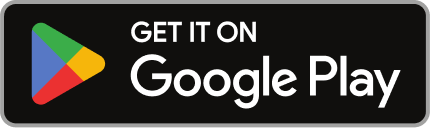
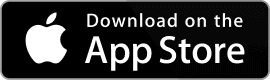
Reviews
Interviews
Salaries
Users
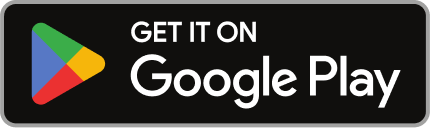
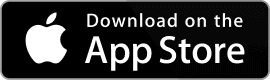