Top 50 PHP Interview Questions and Answers
Updated 16 Jul 2025
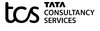
Asked in TCS

Q. What are cookies in PHP?
Cookies in PHP are small text files that are stored on the user's computer to track and store information about the user's activities on a website.
Cookies are used to remember user preferences and login information.
They can be set, retrieved, and del...read more
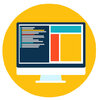
Asked in Neoistone

Q. Explain the difference between $var and $$var.
The difference between $var and $$var is that $var is a variable with a fixed name, while $$var is a variable with a variable name.
The $var variable is a regular variable with a fixed name, while $$var is a variable with a variable name.
The $$var var...read more
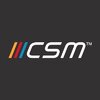
Asked in CSM Technologies

Q. What is the difference between GET and POST methods in PHP?
GET and POST are HTTP methods used to send data to a server in PHP.
GET method appends data to the URL as query parameters
POST method sends data in the body of the HTTP request
GET is less secure as data is visible in the URL
POST is more secure as data...read more
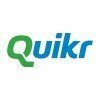
Asked in Quikr

Q. How do sessions work in PHP?
Session in PHP allows to store user data on the server for later use.
Session starts when a user logs in and ends when the user logs out or the session expires.
Session data is stored on the server and identified by a unique session ID.
Session variable...read more
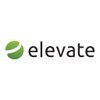
Asked in Elevate Services

Q. What is HTML, PHP and CSS
HTML, PHP, and CSS are programming languages used for creating and designing websites.
HTML (Hypertext Markup Language) is used for creating the structure and content of web pages.
PHP (Hypertext Preprocessor) is a server-side scripting language used f...read more
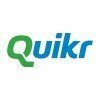
Asked in Quikr

Q. How do you write a Connection class to a MySQL database using PHP?
To connect to MySQL database using PHP, create a Connection class.
Use mysqli_connect() function to establish a connection
Pass the database credentials as parameters to the function
Create a constructor method to initialize the connection
Create a query...read more
Asked in Perfect

Q. What is MySQL Database Connectivity to PHP?
MySQL Database Connectivity to PHP is a way to connect PHP scripts to MySQL databases.
MySQLi and PDO are two PHP extensions used for database connectivity.
MySQLi is an object-oriented extension while PDO is a data access abstraction layer.
Both extens...read more
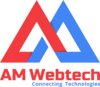
Asked in AM Webtech

Q. What happens if we use $$ in a variable in PHP?
Using $$ in a variable in PHP creates a variable variable.
It creates a new variable with the name of the value of the original variable.
The new variable will contain the value of the original variable.
It can be useful in certain situations, but can a...read more
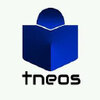
Asked in Tneos Eduloutions

Q. How do you code PHP in a Blade template?
PHP code can be written in Blade templates using the @php directive.
Use the @php directive to write PHP code in Blade templates
Wrap the PHP code in opening and closing PHP tags
Use Blade syntax to output the result of the PHP code
Example: @php echo 'H...read more
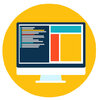
Asked in Neoistone

Q. What are the ways to include a file in PHP?
There are multiple ways to include files in PHP, such as include, require, include_once, and require_once.
include: includes and evaluates a specified file
require: includes and evaluates a specified file, but generates a fatal error if the file is not...read more
PHP Jobs
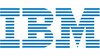
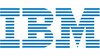
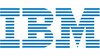
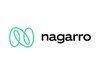
Asked in Nagarro

Q. What are the major skills of a PHP Developer?
A PHP Developer should possess skills in PHP, frameworks, databases, version control, and problem-solving for effective web development.
Proficiency in PHP: Understanding of PHP syntax, functions, and object-oriented programming.
Framework Knowledge: F...read more
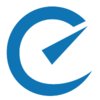
Asked in Esolz Technologies

Q. How do you submit a form using PHP?
To post a form in PHP, use the method attribute in the form tag to specify 'POST' and set the action attribute to the PHP file that will process the form data.
Create a form in HTML with method='POST' and action='process.php'
Use input elements within ...read more
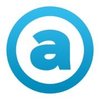
Asked in Alive Inc

Q. Show live projects you have made using PHP.
I have worked on multiple live projects using PHP.
Developed an e-commerce website using PHP and MySQL
Created a social networking platform using PHP and Laravel framework
Built a content management system using PHP and CodeIgniter
Developed a custom CRM...read more
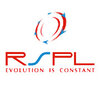
Asked in RSPL Group

Q. What are action and filter hooks?
Action and filter hooks are mechanisms in WordPress that allow developers to modify or add functionality to a theme or plugin.
Action hooks allow you to execute custom code at specific points in the WordPress execution process.
Filter hooks allow you t...read more
Asked in Businesslabs Internet Services

Q. Add PHP filters to your form and store the data in the database.
Use PHP filters to validate form data and store in database.
Use filter_input() function to validate input data.
Sanitize data using filter_var() function.
Connect to database and insert data using prepared statements.
Handle errors and exceptions approp...read more
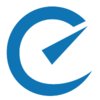
Asked in Esolz Technologies

Q. How do you upload a file using PHP?
To upload a file in PHP, use the built-in function move_uploaded_file().
Create a form with enctype='multipart/form-data'
Use $_FILES['file']['name'] to get the name of the file
Use $_FILES['file']['tmp_name'] to get the temporary location of the file
Us...read more
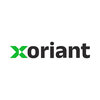
Asked in Xoriant

Q. How do you enable Twig debugging?
To enable twig debug, set debug to true in the twig configuration file.
Edit the development.services.yml file in the sites/default folder
Set debug to true under the twig.config section
Clear the cache to apply changes
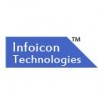
Asked in Infoicon Technologies

Q. How do you define a constant in PHP?
Constants in PHP are identifiers whose value cannot be changed during the script's execution.
Constants are defined using the define() function in PHP.
Constants are case-sensitive by default.
Constants can be accessed globally.
Example: define('PI', 3.1...read more
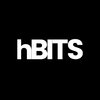
Asked in HumaneBITS

Q. Explain PDO in PHP.
PDO in PHP is a PHP Data Objects extension that provides a consistent interface for accessing databases.
PDO stands for PHP Data Objects
It is a database access layer providing a uniform method of access to multiple databases
It is more secure than the ...read more
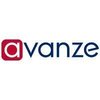
Asked in Avanze Technologies

Q. Php array functions methods
PHP array functions are built-in functions that allow manipulation of arrays in PHP.
Some common array functions include array_push(), array_pop(), array_merge(), and array_filter().
Array functions can be used to add or remove elements, merge arrays, ...read more
Asked in Unidemy Global

Q. Describe a project task with a similar pattern to Oyo implemented in PHP.
Implementing a hotel booking system like Oyo in PHP
Use PHP frameworks like Laravel or CodeIgniter for efficient development
Implement user authentication and authorization for secure access
Create a database schema to store hotel details, room availabi...read more
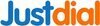
Asked in JustDial

Q. How would you handle cookies and cache in a PHP application?
Cookies and cache in a PHP application can be handled by setting and retrieving cookies, using caching mechanisms like memcached or Redis, and implementing cache control headers.
Set cookies using setcookie() function in PHP
Retrieve cookies using $_CO...read more
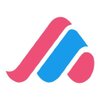
Asked in Appinop Technologies

Q. Tell me about some basic PHP functions you have used.
Some basic PHP functions include echo, strlen, substr, array_push, and file_get_contents.
echo - outputs one or more strings
strlen - returns the length of a string
substr - returns a part of a string
array_push - adds one or more elements to the end of ...read more
Asked in Adnectics It Solutions

Q. What is the difference between sessions and cookies in PHP?
Session and cookies are used to store data in PHP, but session data is stored on the server while cookies are stored on the client's browser.
Session data is stored on the server, while cookies are stored on the client's browser.
Sessions are more secu...read more
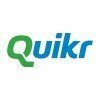
Asked in Quikr

Session management in PHP involves storing user data on the server to maintain state between multiple requests.
Sessions are started using session_start() function in PHP.
Session data is stored on the server and a unique session ID is sent to the clie...read more
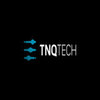
Asked in TNQ Tech Private Limited

Q. What is the use of namespace in PHP?
Namespace in PHP is used to avoid naming conflicts between classes, functions, and variables.
Namespace allows grouping of related classes, functions, and variables under a common name.
It helps in organizing code and makes it easier to maintain.
Namesp...read more
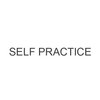
Asked in Self Practice

Q. What is a PHP session and how does it work?
PHP session is a way to store user data on the server and maintain it across multiple pages.
Session data is stored on the server and identified by a unique session ID.
Session ID is usually stored in a cookie on the user's browser.
Session data can be ...read more
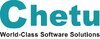
Asked in Chetu

Q. What is Artisan in the Laravel PHP framework?
Artisan is the command-line interface included with Laravel that provides a number of helpful commands for developers.
Artisan is used to generate boilerplate code, run database migrations, and execute unit tests.
Developers can also create their own c...read more
Asked in K Hospitality Corp

Q. What are the differences between unset and unlink in PHP?
unset and unlink are functions in PHP used to remove variables and files respectively.
unset is used to destroy a variable and free up memory
unlink is used to delete a file from the server
Both functions return a boolean value indicating success or fai...read more
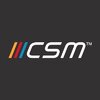
Asked in CSM Technologies

Q. What is the difference between a session and a cookie in PHP?
Session and cookie are used to store data in PHP. Session is stored on the server, while cookie is stored on the client's browser.
Session data is stored on the server and is accessible across different pages of a website.
Cookie data is stored on the ...read more
Top Interview Questions for Related Skills
Interview Experiences of Popular Companies
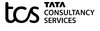
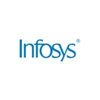
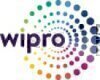
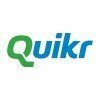
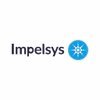
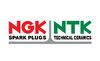
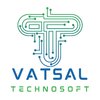
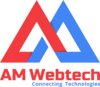
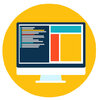

Interview Questions of PHP Related Designations
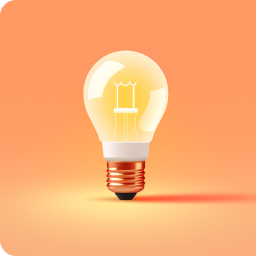
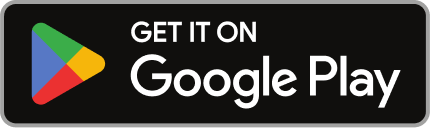
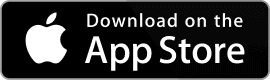
Reviews
Interviews
Salaries
Users
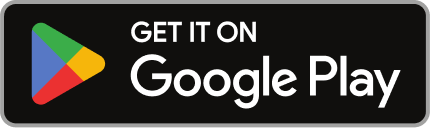
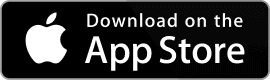