Senior Developer
100+ Senior Developer Interview Questions and Answers
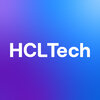
Asked in HCLTech

Q. What’s routing ? Explain exception handling
Routing is the process of selecting a path for network traffic to travel.
Routing is used in networking to determine the best path for data to travel between devices.
It involves analyzing the network topology and selecting the most efficient path.
Routing protocols like OSPF and BGP are used to automate the process.
Exception handling is the process of dealing with errors and exceptions in code.
It involves catching and handling errors to prevent the program from crashing.
Try-cat...read more
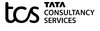
Asked in TCS

Q. What are the ways you can add authentication to the .NET app service?
There are multiple ways to add authentication to .NET app service.
Using Azure Active Directory
Using IdentityServer
Using OAuth2
Using OpenID Connect
Using JWT Tokens
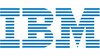
Asked in IBM

Q. In Kafka, how can multiple consumers access the same messages?
Multiple consumers can access the same messages in Kafka by using consumer groups.
Consumers can be grouped together to share the workload of processing messages.
Each consumer in a group will receive a subset of the messages from the same topic.
Consumers in the same group will coordinate to ensure that each message is processed only once.
Consumer offsets are used to track the progress of each consumer in the group.
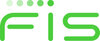
Asked in FIS

Q. What is the difference between static and dynamic method calls?
Static method calls are resolved at compile time, while dynamic method calls are resolved at runtime based on the object's type.
Static method calls are bound to the class, not the instance. Example: ClassName.methodName().
Dynamic method calls are bound to the object instance, allowing for polymorphism. Example: object.methodName().
Static methods cannot be overridden, while dynamic methods can be overridden in subclasses.
Static method calls are faster due to compile-time resol...read more
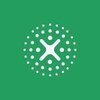
Asked in Xerago

Q. What is react js virtual dom? what is diff algorithm?
React's Virtual DOM optimizes UI updates by minimizing direct manipulation of the real DOM, enhancing performance.
The Virtual DOM is a lightweight copy of the actual DOM, allowing React to manage changes efficiently.
When a component's state changes, React creates a new Virtual DOM tree and compares it to the previous one.
The diffing algorithm identifies changes between the old and new Virtual DOM trees, determining the minimal updates needed.
For example, if a list item is add...read more
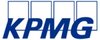
Asked in KPMG India

Q. What is essential for programming?
Programming is the process of writing instructions for a computer to perform specific tasks.
Programming involves writing code using programming languages.
It requires problem-solving skills to break down complex problems into smaller, manageable tasks.
Programmers need to understand algorithms and data structures to optimize code efficiency.
Debugging and testing are essential to identify and fix errors in the code.
Continuous learning and staying updated with new technologies ar...read more
Senior Developer Jobs
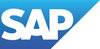
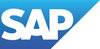
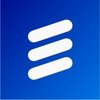
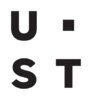
Asked in UST

Q. Write a controller class, service class, entity, and repository.
Code a controller, service, entity, and repository classes for a software application.
Create a controller class to handle incoming requests and interact with the service layer.
Develop a service class to implement business logic and interact with the repository.
Define an entity class to represent data in the application.
Implement a repository class to handle database operations for the entity.
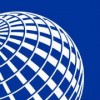
Asked in United Airlines

Q. Ask fewer questions about the 25 horses racing problem.
Determine the fastest horse among 25 with minimal questions about their performance in a race.
Use a tournament-style elimination method to narrow down the fastest horses.
First, divide the 25 horses into 5 groups of 5 and race each group.
Identify the top 3 horses from each group, leading to 15 potential candidates.
Race the top 15 horses to find the fastest horse overall.
Consider using a ranking system to track performance across races.
Share interview questions and help millions of jobseekers 🌟
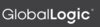
Asked in GlobalLogic

Q. 1. What is ETL and DWH?
ETL stands for Extract, Transform, Load and DWH stands for Data Warehouse.
ETL is a process of extracting data from various sources, transforming it into a format suitable for analysis, and loading it into a target system.
DWH is a system used for storing and managing data from various sources for business intelligence purposes.
ETL is a crucial step in populating a DWH with data.
ETL involves data extraction, data transformation, and data loading.
DWH is designed to support decis...read more
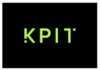
Asked in KPIT Technologies

Q. What are the Projects? What is the experience in Linux QT Docker?
Projects include web applications, mobile apps, and desktop software.
Developed a web application for a retail company using AngularJS and Node.js
Created a mobile app for a fitness startup using React Native
Built a desktop software for a financial institution using Java Swing
Experience in Linux QT Docker includes developing a containerized application for a healthcare company
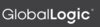
Asked in GlobalLogic

Q. What is the difference between a normal function and an arrow function, and can you provide an example?
Arrow functions are concise syntax for writing functions in JavaScript, compared to normal functions.
Arrow functions do not have their own 'this' keyword, they inherit it from the parent scope.
Arrow functions do not have 'arguments' object.
Arrow functions cannot be used as constructors.
Arrow functions are more concise and easier to read compared to normal functions.
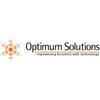
Asked in Optimum Infosystem

Q. How does Microservice work How do you align OOO to JAO Used MongoDB? like that
Microservices are a software architecture style where applications are composed of small, independent services that communicate over well-defined APIs.
Microservices break down a large application into smaller, loosely coupled services that can be developed, deployed, and scaled independently.
Each microservice is responsible for a specific business function and communicates with other services through APIs.
Object-Oriented Programming (OOO) focuses on modeling real-world entiti...read more
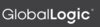
Asked in GlobalLogic

Q. What are the differences between OLTP and OLAP systems?
OLTP is a transactional system for day-to-day operations, while OLAP is analytical system for decision-making.
OLTP deals with real-time data processing, while OLAP deals with historical data analysis.
OLTP is optimized for write operations, while OLAP is optimized for read operations.
OLTP is used for operational tasks like order processing, while OLAP is used for strategic tasks like sales forecasting.
OLTP databases are normalized, while OLAP databases are denormalized.
Example...read more
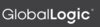
Asked in GlobalLogic

Q. Can you join different fact tables?
Yes, fact tables can be joined together in a data warehouse to combine related information.
Fact tables contain quantitative data and are typically joined using common dimensions.
Joining fact tables allows for more comprehensive analysis and reporting.
For example, joining a sales fact table with a customer fact table can provide insights on customer behavior and purchasing patterns.
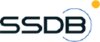
Asked in SSDB Tech Services

Q. How do you create an NPM SDK?
To create an NPM SDK, you need to structure your code as a package, publish it to NPM registry, and document its usage.
Structure your code as a package with a package.json file specifying dependencies, entry point, and scripts.
Publish your package to the NPM registry using 'npm publish' command after logging in with 'npm login'.
Document the usage of your SDK with README.md file, examples, and API documentation.
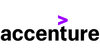
Asked in Accenture

Q. Please describe the end-to-end flow and solution of a Purchase Order (PO), Order Management (OM), or any related module.
End to end flow and solution of PO/OM module
PO/OM module is used for procurement and order management
The flow starts with creating a purchase requisition
The requisition is then approved and converted into a purchase order
The purchase order is sent to the vendor for fulfillment
Once the goods are received, they are inspected and the receipt is recorded
The invoice is matched with the purchase order and receipt
Payment is made to the vendor
The module also includes inventory manage...read more
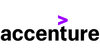
Asked in Accenture

Q. What is inheritance? Hae you lead any team? What is Sevice bus and real time explaination.
Inheritance is a concept in object-oriented programming where a class inherits attributes and methods from another class.
Inheritance allows for code reusability and promotes a hierarchical structure in programming.
Subclasses can inherit properties and behaviors from a superclass.
Example: A 'Vehicle' class can be a superclass, with subclasses like 'Car' and 'Truck' inheriting attributes like 'color' and methods like 'drive'.
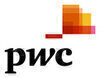
Asked in PwC

Q. What is Hash map? What app did you build How do u maintain app life cycle
A hash map is a data structure that allows for efficient storage and retrieval of key-value pairs.
A hash map uses a hash function to convert keys into array indices.
It provides constant-time average case complexity for insertion, deletion, and retrieval operations.
Hash maps are commonly used in programming languages and databases for fast data access.
Example: HashMap<String, Integer> map = new HashMap<>();
map.put("apple", 5);
int value = map.get("apple"); // returns 5
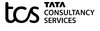
Asked in TCS

Q. When and how do you troubleshoot modules?
I troubleshoot modules by identifying the problem, analyzing the code, and testing solutions.
Identify the problem by reproducing the issue and gathering information
Analyze the code to understand how it works and where the issue may be
Test solutions by implementing and verifying the fix
Document the troubleshooting process and solution for future reference
Asked in EQL Financial Technologies

Q. What are the different operators available in SQL?
SQL operators are symbols that specify operations to be performed on data, including arithmetic, comparison, and logical operations.
Arithmetic Operators: + (addition), - (subtraction), * (multiplication), / (division). Example: SELECT price * quantity FROM orders;
Comparison Operators: = (equal), != (not equal), > (greater than), < (less than). Example: SELECT * FROM products WHERE price > 100;
Logical Operators: AND, OR, NOT. Example: SELECT * FROM users WHERE age > 18 AND cit...read more
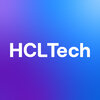
Asked in HCLTech

Q. What is classloader? It's types
Classloader is a part of JVM that loads classes into memory. It has three types: Bootstrap, Extension, and Application.
Classloader loads classes into memory when they are needed by the program
Bootstrap classloader loads core Java classes from the JDK's rt.jar file
Extension classloader loads classes from the JDK's lib/ext directory
Application classloader loads classes from the classpath of the application
Classloader follows delegation model to load classes
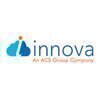
Asked in Innova Solutions

Q. What is CVS? How it is installed in IIB
CVS stands for Concurrent Versions System, a version control system. It can be installed in IIB using the command line or GUI.
CVS is a version control system used to track changes in files and manage multiple versions of the same project.
To install CVS in IIB, you can use the command line by running 'sudo apt-get install cvs' or use a GUI tool like TortoiseCVS.
Once installed, you can configure CVS in IIB by setting up a repository, checking out projects, committing changes, a...read more
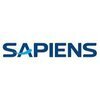
Asked in Sapiens

Q. String S1 = "nav"; String s2 = "nav"; What is the output of Sysout(S1 == s2)?
The code compares two string variables and prints whether they are equal.
String variables in Java are compared using the '==' operator for reference comparison and the equals() method for value comparison.
In this case, since both S1 and s2 have the same value 'nav', the comparison will result in true.
Output: true
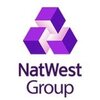
Asked in NatWest Group

Q. How do you use gather stats?
Gather stats is used to collect statistics about database objects in order to optimize query performance.
Gather stats is a command in database management systems like Oracle and PostgreSQL.
It collects information about the distribution of data in tables and indexes.
This information helps the query optimizer to generate efficient execution plans.
Gather stats can be used on individual tables, indexes, or the entire database.
It is typically run after significant data changes or ...read more
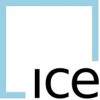
Asked in Intercontinental Exchange

Q. How can you modify an object's data from outside its class?
To change an object's data outside its class, use public methods, properties, or direct access if allowed.
Use public setter methods: `object.setData(value)`.
Access public properties directly: `object.property = value;`.
Utilize friend classes in C++ for access.
In Python, use properties with decorators for controlled access.
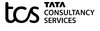
Asked in TCS

Q. Tell some springboot annotations ? What is maven? What are Java 8 features ?
Springboot annotations include @RestController, @Autowired, @RequestMapping. Maven is a build automation tool. Java 8 features include lambda expressions, streams, and functional interfaces.
@RestController - used to define RESTful web services
@Autowired - used for automatic dependency injection
@RequestMapping - maps HTTP requests to handler methods
Maven is a build automation tool that manages project dependencies and builds the project
Java 8 features include lambda expression...read more
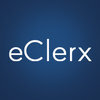
Asked in eClerx

Q. What is the difference between clustered and non-clustered indexes?
Clustered indexes physically order the data rows in the table while non-clustered indexes do not.
Clustered indexes determine the physical order of data rows in a table.
Non-clustered indexes store a separate structure that contains the index key values and pointers to the actual data rows.
Tables can only have one clustered index but multiple non-clustered indexes.
Clustered indexes are faster for retrieval of data but slower for inserts and updates compared to non-clustered ind...read more
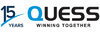
Asked in Quess

Q. What is the position property in CSS?
Position property in CSS specifies the positioning method of an element.
It is used to set the position of an element relative to its parent or to the browser window.
It can take values like static, relative, absolute, fixed, sticky.
Static is the default value which means the element is positioned according to the normal flow of the document.
Relative positions the element relative to its normal position.
Absolute positions the element relative to its nearest positioned ancestor....read more
Asked in OptiValue Tek Consulting

Q. How do you create a report data model and template?
Creating a report data model involves structuring data for analysis, while templates define the report's layout and design.
Identify Data Sources: Determine where the data will come from, such as databases, APIs, or spreadsheets. For example, using SQL queries to fetch data.
Define Data Structure: Organize the data into a logical structure, such as tables or objects, that reflects the relationships between different data points.
Create Data Model: Use tools like ER diagrams to v...read more
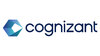
Asked in Cognizant

Q. What is the difference between Delete and Truncate?
DELETE removes specific rows; TRUNCATE removes all rows quickly without logging individual row deletions.
DELETE can remove specific rows based on a condition (e.g., DELETE FROM table WHERE id = 1).
TRUNCATE removes all rows in a table without logging individual row deletions (e.g., TRUNCATE TABLE table).
DELETE can be rolled back if used within a transaction, while TRUNCATE cannot be rolled back.
TRUNCATE is generally faster than DELETE because it does not generate individual ro...read more
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
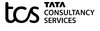
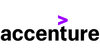
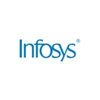
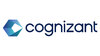
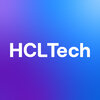
Top Interview Questions for Senior Developer Related Skills
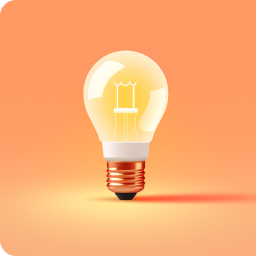
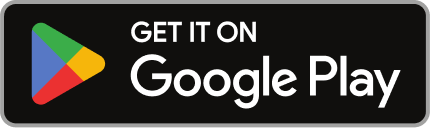
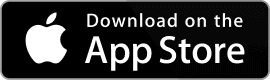
Reviews
Interviews
Salaries
Users
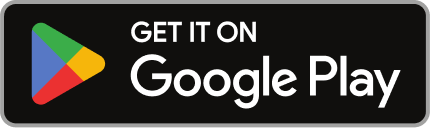
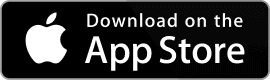