Python and Django Developer
100+ Python and Django Developer Interview Questions and Answers
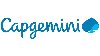
Asked in Capgemini

Q. What is the python How to use python You can join immed
Python is a high-level programming language known for its simplicity and readability.
Python is used for web development, data analysis, artificial intelligence, and more.
To use Python, you need to install it on your computer and write code in a text editor or an integrated development environment (IDE).
Python code is executed line by line, and indentation is crucial for defining code blocks.
Python has a vast standard library and a large community that provides numerous third-...read more
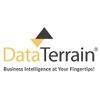
Asked in DataTerrain

Q. What is the difference between Class-based views and function-based views?
Class-based views are based on classes and provide more structure and functionality, while function-based views are based on functions and are simpler and more flexible.
Class-based views are defined as classes and inherit from Django's generic view classes, providing built-in functionality such as mixins and decorators.
Function-based views are defined as functions and are more flexible, allowing for custom logic and control over the request-response cycle.
Class-based views ar...read more
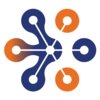
Asked in Medikabazaar

Q. Given an integer array, find pairs of two numbers whose difference is the least among others.
Find pairs of integers with least difference in an array
Sort the array in ascending order
Iterate through the array and find the minimum difference between adjacent elements
Return the pairs of elements with the minimum difference
Asked in Xeroeta Technologies

Q. What is OAuth 2.0? And how it works?
OAuth 2.0 is an authorization framework that allows third-party applications to access user data without sharing passwords.
OAuth 2.0 is used to grant access to resources on behalf of a user.
It uses access tokens to grant access to resources.
OAuth 2.0 has four roles: resource owner, resource server, client, and authorization server.
Examples of OAuth 2.0 providers include Google, Facebook, and Twitter.
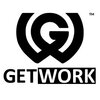
Asked in GetWork

Q. Explain the design pattern used in Django.
Django follows the Model-View-Template (MVT) architectural pattern for web development.
MVT is a variation of the MVC (Model-View-Controller) pattern.
Model: Represents the data structure. Example: A Django model for a blog post.
View: Contains the business logic and interacts with the model. Example: A view function that retrieves blog posts.
Template: Handles the presentation layer. Example: An HTML template that displays blog posts.
Asked in Geninvo Technologies

Q. If there's a telephone company which type of factors can be used to predict that it's users will leave or stay. Which ml model would be best suited for the same.
Factors like call duration, frequency of calls, payment history, customer service interactions can predict user churn. Logistic Regression model is best suited.
Call duration: Longer calls may indicate higher satisfaction and lower likelihood of leaving.
Frequency of calls: Higher frequency may indicate engagement and loyalty.
Payment history: Timely payments may indicate commitment to the service.
Customer service interactions: Negative interactions may lead to churn.
Logistic Re...read more
Python and Django Developer Jobs
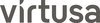
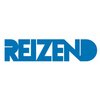
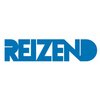
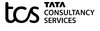
Asked in TCS

Q. Tell me about how you access the API to the application using Python.
To access APIs in Python, use libraries like requests or urllib.
Use the requests library to make HTTP requests to APIs.
Authenticate with APIs using tokens or keys.
Parse JSON responses using the json library.
Handle errors and exceptions when accessing APIs.
Example: Using requests library to access Twitter API.
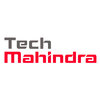
Asked in Tech Mahindra

Q. What do you understand about tokens in the 'C' language?
Tokens in 'C' language are the smallest unit of a program that is meaningful to the compiler.
Tokens include keywords, identifiers, constants, strings, operators, and punctuation symbols.
Examples of tokens in 'C' language: int, main, 5, 'hello', +, ;
Share interview questions and help millions of jobseekers 🌟
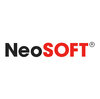
Asked in NeoSOFT

Q. What are examples of method overloading and method overriding in Object-Oriented Programming (OOP)?
Method overloading allows multiple methods with the same name but different parameters; overriding replaces a method in a subclass.
Method Overloading: Same method name with different parameters in the same class.
Example: def add(self, a: int, b: int) and def add(self, a: float, b: float).
Method Overriding: Subclass provides a specific implementation of a method already defined in its superclass.
Example: class Animal has a method speak(), class Dog overrides it with its own sp...read more
Asked in Hajela's IAS Academy

Q. Are you responsible for handling Excel data import and export in Django?
Yes, I handle Excel data import/export in Django using libraries like pandas and openpyxl for efficient data processing.
Utilize pandas for reading and writing Excel files: `df = pd.read_excel('file.xlsx')`.
Use openpyxl for more complex Excel manipulations, such as formatting cells.
Implement Django views to handle file uploads and downloads seamlessly.
Create serializers to convert data to and from Excel format for API integration.
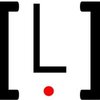
Asked in Lincode Labs

Q. How can we optimize a MongoDB database?
Optimising MongoDB Database involves indexing, proper query optimization, sharding, and using the right data model.
Create indexes on fields frequently used in queries to improve query performance.
Use the explain() method to analyze and optimize query performance.
Implement sharding to distribute data across multiple servers for scalability.
Use the appropriate data model for your application to reduce the number of queries needed.
Regularly monitor and optimize the database perf...read more
Asked in Xeroeta Technologies

Q. What is JWT? Why is it used?
JWT stands for JSON Web Token. It is used for secure transmission of information between parties.
JWT is a compact, URL-safe means of representing claims to be transferred between two parties.
It is used for authentication and authorization purposes.
JWT consists of three parts: header, payload, and signature.
The header contains the algorithm used to sign the token.
The payload contains the claims or information being transmitted.
The signature is used to verify the integrity of t...read more
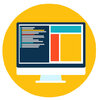
Asked in Neoistone

Q. Explain the Django project directory structure.
Django project directory structure organizes files and folders in a specific way.
The project root directory contains manage.py file and project settings file.
Apps are created inside the project directory.
Static files are stored in a 'static' directory.
Templates are stored in a 'templates' directory.
Migrations are stored in a 'migrations' directory.
Media files are stored in a 'media' directory.
Virtual environment is created outside the project directory.
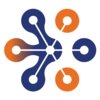
Asked in Medikabazaar

Q. Given an integer array, count the occurrences of all items with a count greater than 1.
Count occurrence of items with count greater than 1 in an integer array.
Create a dictionary to store the count of each item in the array.
Iterate through the dictionary and count the items with count greater than 1.
Return the count of items with count greater than 1.
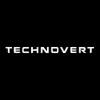
Asked in Technovert Solutions

Q. What is an updated query in a database?
An updated query in a database is used to modify existing data in the database.
An updated query is used to change the values of one or more columns in a database table.
It is typically used with the UPDATE statement in SQL.
The updated query specifies the table to be updated, the columns to be modified, and the new values for those columns.
It can also include conditions to specify which rows should be updated.
For example, an updated query can be used to change the status of all...read more
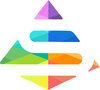
Asked in Speqto Technologies

Q. What is python , what is oops concept , difference between list and tuple ,
Python is a high-level programming language known for its simplicity and readability. OOPs is a programming paradigm. List and tuple are both sequence data types in Python.
Python is a versatile and powerful language used for web development, data analysis, artificial intelligence, and more.
OOPs (Object-Oriented Programming) is a programming paradigm that organizes data and behavior into reusable structures called objects.
List and tuple are both sequence data types in Python, ...read more
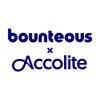
Asked in Bounteous x Accolite

Q. You are climbing a staircase. It takes n steps to reach the top. Each time you can either climb 1 or 2 steps. In how many distinct ways can you climb to the top?
Maximum possible ways of climbing stairs with 1 or 2 steps at a time.
Use dynamic programming approach
Fibonacci sequence can be used to solve the problem
For n stairs, the answer is fib(n+1)
Example: for 3 stairs, answer is 3
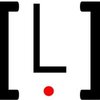
Asked in Lincode Labs

Q. Explain Indexing and all its types in MongoDB
Indexing in MongoDB improves query performance by allowing the database to quickly locate and retrieve specific documents.
Indexes in MongoDB are similar to indexes in relational databases, allowing for efficient data retrieval.
Types of indexes in MongoDB include single field, compound, multikey, text, hashed, and geospatial indexes.
Single field indexes are created on a single field in a document, while compound indexes are created on multiple fields.
Multikey indexes are used ...read more
Asked in Qubedynamics Technology Solution

Q. Explain the Django architecture (URLs, views, models, etc.).
Django follows Model-View-Controller (MVC) architecture with URL routing, views, and models.
URL routing maps URLs to views
Views handle HTTP requests and return HTTP responses
Models define the database schema and interact with the database
Templates render HTML pages using data from views
Django ORM provides an abstraction layer for database operations
Middleware provides hooks for modifying request/response objects
Admin site provides a pre-built interface for managing models
Stat...read more
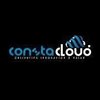
Asked in Constacloud

Q. What is Object-Relational Mapping (ORM), and what are its uses?
ORM is a programming technique that allows developers to interact with databases using Python objects instead of SQL queries.
Simplifies database interactions by mapping database tables to Python classes.
Allows developers to use Python syntax instead of SQL, making code more readable.
Supports CRUD operations (Create, Read, Update, Delete) through Python methods.
Example: Using Django's ORM, you can create a new user with 'User.objects.create(username='john')'.
Facilitates databa...read more
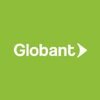
Asked in Globant

Q. What is the difference between range and xrange?
range returns a list of numbers while xrange returns an iterator object.
range creates a list of numbers from start to end with a step size of 1.
xrange returns an iterator object that generates numbers on the fly.
range takes more memory as it creates a list while xrange is memory efficient.
In Python 3, range is similar to xrange in Python 2.
Asked in OneFin

Q. What is the sum of the two diagonals in a matrix?
The sum of the two diagonals in a matrix is calculated by adding the elements from both the primary and secondary diagonals.
Primary Diagonal: This diagonal runs from the top-left to the bottom-right of the matrix. For example, in a 3x3 matrix [[1,2,3],[4,5,6],[7,8,9]], the primary diagonal elements are 1, 5, and 9.
Secondary Diagonal: This diagonal runs from the top-right to the bottom-left. In the same matrix, the secondary diagonal elements are 3, 5, and 7.
Sum Calculation: T...read more
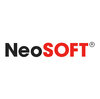
Asked in NeoSOFT

Q. What are the key concepts and features of multithreading?
Multithreading allows concurrent execution of tasks in Python, enhancing performance and responsiveness in applications.
Concurrency: Multiple threads run simultaneously, improving application responsiveness.
Thread Creation: Use the 'threading' module to create threads. Example: 'threading.Thread(target=func).start()'.
Synchronization: Use locks (e.g., 'threading.Lock') to prevent race conditions when accessing shared resources.
Thread Lifecycle: Threads can be in states like 'n...read more
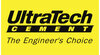
Asked in UltraTech Cement

Q. What is method overloading in Python?
Method overloading is not supported in Python.
Method overloading is the ability to define multiple methods with the same name but different parameters.
Python does not support method overloading as it allows default arguments and variable-length arguments.
Instead, we can use default arguments or variable-length arguments to achieve similar functionality.
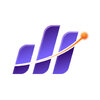
Asked in Bluestock ™

Q. What is the difference between Multithreading and Multiprocessing?
Multithreading involves multiple threads within the same process, while multiprocessing involves multiple processes.
Multithreading shares the same memory space, while multiprocessing has separate memory space for each process.
Multithreading is more lightweight and efficient for I/O-bound tasks, while multiprocessing is better for CPU-bound tasks.
Multithreading can lead to race conditions and synchronization issues, while multiprocessing avoids these problems by using separate...read more
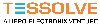
Asked in Tessolve

Q. What are Middleware, Signals and Django Rest Framework
Middleware, Signals, and Django Rest Framework are key components of Django framework.
Middleware is a Django feature that allows you to process requests and responses globally.
Signals are a way to allow certain senders to notify a set of receivers when some action has taken place.
Django Rest Framework is a powerful and flexible toolkit for building Web APIs.
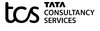
Asked in TCS

Q. What is your preferred programming language?
My perfect programming language is one that is versatile, efficient, and has a strong community support.
Versatile - able to handle a wide range of tasks and projects
Efficient - optimized for performance and speed
Strong community support - active community for help and resources
Examples: Python, JavaScript, Java
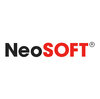
Asked in NeoSOFT

Q. Types of pandas and what the role of backend developer
Pandas is a powerful data manipulation library in Python, providing data structures like Series and DataFrame for efficient data analysis.
Series: A one-dimensional labeled array capable of holding any data type, similar to a list or array. Example: pd.Series([1, 2, 3])
DataFrame: A two-dimensional labeled data structure with columns of potentially different types, akin to a spreadsheet or SQL table. Example: pd.DataFrame({'A': [1, 2], 'B': [3, 4]})
Panel: A three-dimensional da...read more
Asked in Kapil Group

Q. Give an example of a project where you optimized performance.
Optimizing performance in a Django project involves efficient database queries, caching, and using asynchronous tasks.
Use Django's select_related and prefetch_related to reduce database hits. Example: `queryset = Book.objects.select_related('author').all()`.
Implement caching with Django's caching framework to store frequently accessed data. Example: `cache.set('my_data', data, timeout=60)`.
Utilize Django's built-in pagination to handle large datasets efficiently. Example: `Pa...read more
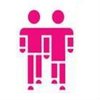
Asked in HireXtra

Q. What techniques are used for scraping social networking sites?
Techniques for scraping social networking sites involve using APIs, web scraping tools, and custom scripts.
Utilize APIs provided by social networking sites for accessing data in a structured manner
Use web scraping tools like BeautifulSoup or Scrapy to extract information from HTML pages
Write custom scripts in Python using libraries like requests and selenium to automate the scraping process
Respect the terms of service of the social networking site to avoid legal issues
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
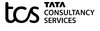
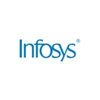
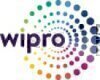
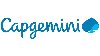
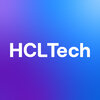
Top Interview Questions for Python and Django Developer Related Skills
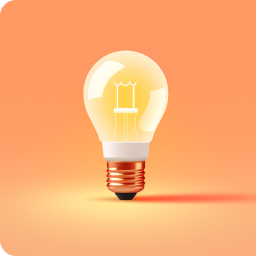
Calculate your in-hand salary
Confused about how your in-hand salary is calculated? Enter your annual salary (CTC) and get your in-hand salary
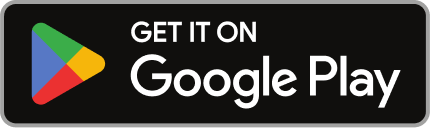
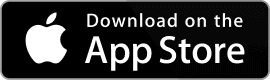
Reviews
Interviews
Salaries
Users
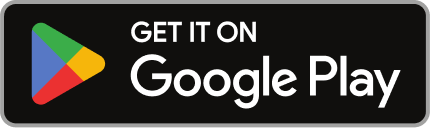
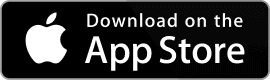