Full Stack Developer
100+ Full Stack Developer Interview Questions and Answers for Freshers
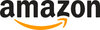
Asked in Amazon

Q. Maximum Subarray Sum Problem Statement
Given an array ARR
consisting of N
integers, your goal is to determine the maximum possible sum of a non-empty contiguous subarray within this array.
Example of Subarrays:...read more
Find the maximum sum of a contiguous subarray within an array of integers.
Iterate through the array and keep track of the maximum sum of subarrays seen so far.
Use Kadane's algorithm to efficiently find the maximum subarray sum.
Consider edge cases like all negative numbers in the array.
Example: For input [-2, 1, -3, 4, -1], the maximum subarray sum is 4.
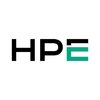
Asked in Hewlett Packard Enterprise

Q. Middle of a Linked List
You are given the head node of a singly linked list. Your task is to return a pointer pointing to the middle of the linked list.
If there is an odd number of elements, return the middle ...read more
Return the middle element of a singly linked list, or the one farther from the head if there are even elements.
Traverse the linked list with two pointers, one moving twice as fast as the other
When the fast pointer reaches the end, the slow pointer will be at the middle
If there are even elements, return the one pointed by the slow pointer

Asked in Hike

Q. Minimum Number of Swaps to Sort an Array
Find the minimum number of swaps required to sort a given array of distinct elements in ascending order.
Input:
T (number of test cases)
For each test case:
N (size of the...read more
The minimum number of swaps required to sort a given array of distinct elements in ascending order.
Use a hashmap to store the original indices of the elements in the array.
Iterate through the array and swap elements to their correct positions.
Count the number of swaps needed to sort the array.
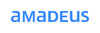
Asked in Amadeus

Q. Sort Array of Strings Problem Statement
Given an array of strings ARRSTR[]
of size N
, and a character C
, your task is to sort the ARRSTR[]
array according to a new alphabetical order that starts with the given ...read more
Sort an array of strings based on a new alphabetical order starting with a given character.
Iterate through the array of strings and compare each string based on the new alphabetical order starting with the given character.
Use a custom comparator function to sort the strings according to the new alphabetical order.
Return the sorted array of strings.
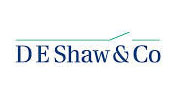
Asked in DE Shaw

Q. Buy and Sell Stock Problem Statement
Imagine you are Harshad Mehta's friend, and you have been given the stock prices of a particular company for the next 'N' days. You can perform up to two buy-and-sell transa...read more
The task is to determine the maximum profit that can be achieved by performing up to two buy-and-sell transactions on a given set of stock prices.
Iterate through the array of stock prices to find the maximum profit that can be achieved by buying and selling stocks at different points.
Keep track of the maximum profit that can be achieved by considering all possible combinations of buy and sell transactions.
Ensure that you sell the stock before buying again to adhere to the con...read more
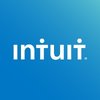
Asked in Intuit

Q. Count Pairs with Given Sum
Given an integer array/list arr
and an integer 'Sum', determine the total number of unique pairs in the array whose elements sum up to the given 'Sum'.
Input:
The first line contains ...read more
Count the total number of unique pairs in an array whose elements sum up to a given value.
Use a hashmap to store the frequency of each element in the array.
Iterate through the array and for each element, check if (Sum - current element) exists in the hashmap.
Increment the count of pairs if the complement exists in the hashmap.
Divide the count by 2 to avoid counting duplicates like (arr[i], arr[j]) and (arr[j], arr[i]) separately.
Full Stack Developer Jobs
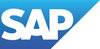


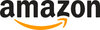
Asked in Amazon

Q. Find the Row with the Maximum Number of 1's
You are given a non-empty grid MAT
with 'N' rows and 'M' columns, where each element is either 0 or 1. All rows are sorted in ascending order.
Your task is to determi...read more
Find the row with the maximum number of 1's in a grid of 0's and 1's, returning the index of the row with the most 1's.
Iterate through each row of the grid and count the number of 1's in each row
Keep track of the row index with the maximum number of 1's seen so far
Return the index of the row with the maximum number of 1's
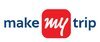
Asked in MakeMyTrip

Q. Longest Duplicate Substring Problem Statement
You are provided with a string 'S'. The task is to determine the length of the longest duplicate substring within this string. Note that duplicate substrings can ov...read more
Find the length of the longest duplicate substring in a given string.
Iterate through all possible substrings of the input string.
Use a rolling hash function to efficiently compare substrings.
Store the lengths of duplicate substrings and return the maximum length.
Share interview questions and help millions of jobseekers 🌟
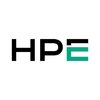
Asked in Hewlett Packard Enterprise

Q. Smallest Number with Given Digit Product
Given a positive integer 'N', find and return the smallest number 'M', such that the product of all the digits in 'M' is equal to 'N'. If such an 'M' is not possible or ...read more
Find the smallest number whose digits multiply to a given number N.
Iterate through possible digits to form the smallest number with product equal to N
Use a priority queue to keep track of the smallest possible number
Check constraints to ensure the number fits in a 32-bit signed integer
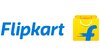
Asked in Flipkart

Q. The Skyline Problem
Compute the skyline of given rectangular buildings in a 2D city, eliminating hidden lines and forming the outer contour of the silhouette when viewed from a distance. Each building is descri...read more
Compute the skyline of given rectangular buildings in a 2D city, eliminating hidden lines and forming the outer contour of the silhouette.
Iterate through the buildings to find the critical points (start and end) of each building.
Sort the critical points based on x-coordinate and process them to find the skyline.
Merge consecutive horizontal segments of equal height in the output to ensure no duplicates.
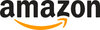
Asked in Amazon

Q. Validate Binary Search Tree (BST)
You are given a binary tree with 'N' integer nodes. Your task is to determine whether this binary tree is a Binary Search Tree (BST).
BST Definition:
A Binary Search Tree (BST)...read more
Validate if a given binary tree is a Binary Search Tree (BST) or not.
Check if the left subtree of a node contains only nodes with data less than the node's data.
Check if the right subtree of a node contains only nodes with data greater than the node's data.
Ensure that both the left and right subtrees are also binary search trees.
Traverse the tree in an inorder manner and check if the elements are in sorted order.
Recursively check each node's value against the range of values ...read more
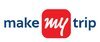
Asked in MakeMyTrip

Q. Delete Node in Binary Search Tree Problem Statement
You are provided with a Binary Search Tree (BST) containing 'N' nodes with integer data. Your task is to remove a given node from this BST.
A BST is a binary ...read more
Implement a function to delete a node from a Binary Search Tree and return the inorder traversal of the modified BST.
Understand the properties of a Binary Search Tree (BST) - left subtree contains nodes with data less than the node, right subtree contains nodes with data greater than the node.
Implement a function to delete the given node from the BST while maintaining the BST properties.
Perform inorder traversal of the modified BST to get the desired output.
Handle cases where...read more
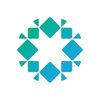
Asked in RUBRIK INDIA

Q. Minimum Time Problem Statement
In a city with ‘N’ junctions and ‘M’ bi-directional roads, each junction is connected to other junctions with specified travel times. No road connects a junction to itself, and on...read more
The problem involves finding the minimum time to travel from a source junction to a destination junction in a city with specified travel times and green light periods.
Input consists of the number of test cases, number of junctions and roads, green light periods, road connections with travel times, and source/destination junctions.
Output should be the minimum time needed from source to destination, or -1 if destination is unreachable.
Constraints include limits on test cases, j...read more
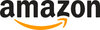
Asked in Amazon

Q. Pair Sum Problem Statement
You are given an integer array 'ARR' of size 'N' and an integer 'S'. Your task is to find and return a list of all pairs of elements where each sum of a pair equals 'S'.
Note:
Each pa...read more
Given an array of integers and a target sum, find all pairs of elements that add up to the target sum.
Use a hashmap to store the difference between the target sum and each element as keys and their indices as values.
Iterate through the array and check if the current element's complement exists in the hashmap.
Return the pairs of elements that add up to the target sum in sorted order.
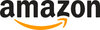
Asked in Amazon

Q. String Palindrome Verification
Given a string, your task is to determine if it is a palindrome considering only alphanumeric characters.
Input:
The input is a single string without any leading or trailing space...read more
Check if a given string is a palindrome considering only alphanumeric characters.
Remove non-alphanumeric characters from the input string.
Compare the string with its reverse to check for palindrome.
Handle edge cases like empty string or single character input.
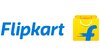
Asked in Flipkart

Q. Circular Move Problem Statement
You have a robot currently positioned at the origin (0, 0) on a two-dimensional grid, facing the north direction. You are given a sequence of moves in the form of a string of len...read more
Determine if a robot's movement path is circular on a 2D grid based on a given sequence of moves.
Iterate through the sequence of moves and keep track of the robot's position and direction.
Check if the robot returns to the starting position after completing the moves.
Use a counter-clockwise rotation (L) and clockwise rotation (R) to update the direction of the robot.
Use 'G' to move the robot one unit in the current direction.
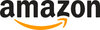
Asked in Amazon

Q. Permutation In String Problem Statement
Given two strings, str1
and str2
, determine whether str2
contains any permutation of str1
as a substring.
Input:
str1 = “ab”
str2 = “aoba”
Output:
True
Example:
Explanatio...read more
Check if a string contains any permutation of another string as a substring.
Iterate through str2 with a sliding window of length str1, check if any permutation of str1 is present.
Use a hashmap to store the frequency of characters in str1 and str2 for comparison.
If the frequencies of characters in the sliding window match the frequencies of characters in str1, return True.
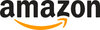
Asked in Amazon

Q. Rat in a Maze Problem Statement
You need to determine all possible paths for a rat starting at position (0, 0) in a square maze to reach its destination at (N-1, N-1). The maze is represented as an N*N matrix w...read more
Find all possible paths for a rat in a maze from start to finish, moving in 'U', 'D', 'L', 'R' directions.
Use backtracking to explore all possible paths in the maze.
Keep track of visited cells to avoid loops.
Recursively try moving in all directions and backtrack when reaching dead ends.
Return the valid paths in alphabetical order as an array of strings.
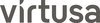
Asked in Virtusa Consulting Services

Account Statements Transcription is a full stack application for converting Excel sheets to SQL tables, fetching data in UI, and allowing downloads in various formats.
Create a front-end interface for users to upload Excel sheets
Develop a back-end system to convert Excel data into SQL tables
Implement a query system to fetch data based on user needs in the UI
Provide options for users to download data in Excel or Word formats
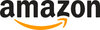
Asked in Amazon

Q. Minimum Jumps Problem Statement
Bob and his wife are in the famous 'Arcade' mall in the city of Berland. This mall has a unique way of moving between shops using trampolines. Each shop is laid out in a straight...read more
This question asks for the minimum number of trampoline jumps a person needs to make in order to reach the last shop in a mall.
The shops in the mall are laid out in a straight line and each shop has a constant value representing the maximum distance it can be jumped to.
The person starts at shop 0 and wants to reach the last shop, shop N-1.
If it is impossible to reach the last shop, the function should return -1.
The function should take the number of test cases, the number of ...read more
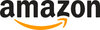
Asked in Amazon

Q. Alien Dictionary Problem Statement
You are provided with a sorted dictionary (by lexical order) in an alien language. Your task is to determine the character order of the alien language from this dictionary. Th...read more
Given a sorted alien dictionary in an array of strings, determine the character order of the alien language.
Iterate through the words in the dictionary to build a graph of character dependencies.
Perform a topological sort on the graph to determine the character order.
Return the character array representing the order of characters in the alien language.
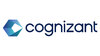
Asked in Cognizant

Q. Buy and Sell Stock - III Problem Statement
Given an array prices
where the ith element represents the price of a stock on the ith day, your task is to determine the maximum profit that can be achieved at the en...read more
Determine the maximum profit that can be achieved by selling stocks with at most two transactions.
Iterate through the array and calculate the maximum profit that can be achieved by selling stocks at each day.
Keep track of the maximum profit after the first transaction and the maximum profit after the second transaction.
Return the maximum profit that can be achieved overall.
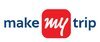
Asked in MakeMyTrip

Q. Determine the Left View of a Binary Tree
You are given a binary tree of integers. Your task is to determine the left view of the binary tree. The left view consists of nodes that are visible when the tree is vi...read more
The task is to determine the left view of a binary tree, which consists of nodes visible when viewed from the left side.
Traverse the binary tree level by level from left to right, keeping track of the first node encountered at each level.
Use a queue to perform level order traversal and keep track of the level number for each node.
Store the first node encountered at each level in a result array to get the left view of the binary tree.
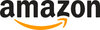
Asked in Amazon

Q. Smallest Window Problem Statement
Given two strings, S
and X
, your task is to find the smallest substring in S
that contains all the characters present in X
.
Example:
Input:
S = "abdd", X = "bd"
Output:
"bd"
Ex...read more
Find the smallest substring in a given string that contains all characters present in another string.
Use a sliding window approach to find the smallest window in S that contains all characters in X
Keep track of the characters in X using a hashmap
Move the window by adjusting the start and end pointers
Return the smallest window found
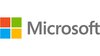
Asked in Microsoft Corporation

Q. Group Anagrams Problem Statement
Given an array or list of strings called inputStr
, your task is to return the strings grouped as anagrams. Each group should contain strings that are anagrams of one another.
An...read more
Group anagrams in an array of strings based on their characters.
Iterate through each string in the input array
Sort the characters of each string and use the sorted string as a key in a hashmap to group anagrams
Return the values of the hashmap as the grouped anagrams
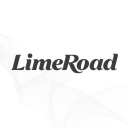
Asked in LimeRoad

Q. Problem: Ninja's Robot
The Ninja has a robot which navigates an infinite number line starting at position 0 with an initial speed of +1. The robot follows a set of instructions which includes ‘A’ (Accelerate) a...read more
Determine the minimum length of instruction sequence for a robot to reach a given target on an infinite number line.
Start at position 0 with speed +1, update position and speed based on 'A' and 'R' instructions
For each test case, find the shortest sequence of instructions to reach the target
Consider both positive and negative positions for the robot
Return the minimum length of instruction sequence for each test case
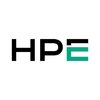
Asked in Hewlett Packard Enterprise

Q. Remove Character from String Problem Statement
Given a string str
and a character 'X', develop a function to eliminate all instances of 'X' from str
and return the resulting string.
Input:
The first line contai...read more
Develop a function to remove all instances of a given character from a string.
Create a function that takes the input string and character to be removed as parameters.
Iterate through each character in the input string and only add characters that are not equal to the given character to a new string.
Return the new string as the output.
Handle edge cases such as empty input string or character.
Example: Input string 'hello world' and character 'o' should return 'hell wrld'.
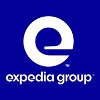
Asked in Expedia Group

Q. Valid Parentheses Problem Statement
Given a string 'STR' consisting solely of the characters “{”, “}”, “(”, “)”, “[” and “]”, determine if the parentheses are balanced.
Input:
The first line contains an integer...read more
The task is to determine if a given string of parentheses is balanced or not.
Iterate through each character in the string and use a stack to keep track of opening parentheses
If a closing parenthesis is encountered, check if it matches the top of the stack. If not, the string is not balanced
If all parentheses are matched correctly and the stack is empty at the end, the string is balanced
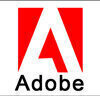
Asked in Adobe

Q. Tiling Problem Statement
Given a board with 2 rows and N columns, and an infinite supply of 2x1 tiles, determine the number of distinct ways to completely cover the board using these tiles.
You can place each t...read more
The problem involves finding the number of ways to tile a 2xN board using 2x1 tiles.
Use dynamic programming to solve the problem efficiently.
Consider the base cases for N=1 and N=2 to build up the solution for larger N.
Implement a recursive function with memoization to avoid redundant calculations.
Keep track of the number of ways modulo 10^9 + 7 to handle large values of N.
Test the solution with different values of N to ensure correctness.
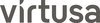
Asked in Virtusa Consulting Services

Prisoners must devise a strategy to guess the color of their own hat based on the hats of others.
Prisoners can agree on a strategy before the hats are assigned.
One strategy is for each prisoner to count the number of hats of a certain color and use that information to guess their own hat color.
Another strategy involves using parity to determine the color of their own hat.
Prisoners can also use a signaling system to convey information about their hat color to others.
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
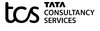
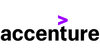
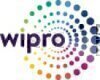
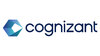
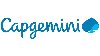
Top Interview Questions for Full Stack Developer Related Skills
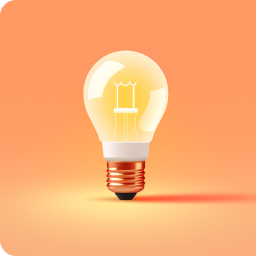
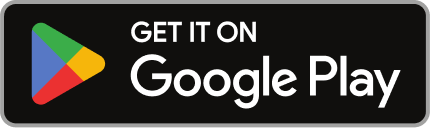
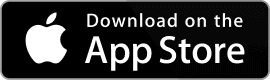
Reviews
Interviews
Salaries
Users
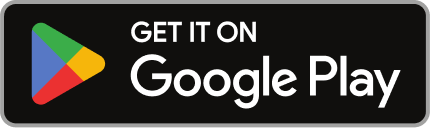
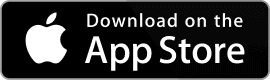