Computer Scientist
10+ Computer Scientist Interview Questions and Answers
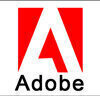
Asked in Adobe

Q. Given a matrix filled with directions, is there a path from a start point to an end point?
Determine if a path exists in a matrix filled with directional indicators from a start to an end point.
Use Depth-First Search (DFS) or Breadth-First Search (BFS) to explore paths.
Check if the current position is within matrix bounds and not visited.
Example: In a 2D grid, 'U' means up, 'D' means down, 'L' means left, 'R' means right.
Track visited nodes to avoid cycles and infinite loops.
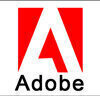
Asked in Adobe

Q. Given an array of integers nums and an integer k, return the total number of subarrays whose sum equals to k.
Given an array of integers, find if a subarray exists whose sum equals to k.
Use a hashmap to store the prefix sum and its frequency.
Iterate through the array and check if the difference between current prefix sum and k exists in the hashmap.
If it exists, then a subarray with sum k exists.
Time complexity: O(n), Space complexity: O(n).
Computer Scientist Interview Questions and Answers for Freshers
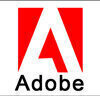
Asked in Adobe

Q. How would you break a linked list into two lists, one containing positive numbers and the other containing negative numbers?
To break a linked list into two lists, we can traverse the list and add nodes to respective lists based on their value.
Traverse the linked list and add nodes to positive or negative list based on their value
Create two empty lists for positive and negative nodes
Iterate through the linked list and add nodes to respective lists
Join the two lists at the end to get the final result
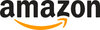
Asked in Amazon

Q. You are given an array of k linked-lists lists, each linked-list is sorted in ascending order. Merge all the linked-lists into one sorted linked-list and return it.
Merge K-Sorted Linked List
Create a min heap of size k
Insert the first element of each linked list into the heap
Pop the minimum element from the heap and add it to the result list
Insert the next element from the linked list of the popped element into the heap
Repeat until all elements are processed
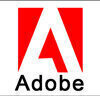
Asked in Adobe

Q. Given a string s and a dictionary of strings wordDict, return true if s can be segmented into a space-separated sequence of one or more dictionary words.
Determine if a string can be segmented into space-separated words from a given dictionary.
Use dynamic programming to build a boolean array that tracks segmentability.
Example: For s = 'leetcode' and dict = ['leet', 'code'], return true.
Iterate through the string, checking if the substring can be formed using the dictionary.
Example: For s = 'applepenapple' and dict = ['apple', 'pen'], return true.
Asked in Adobe Metal Products

Q. Given movie start times and durations, what is the minimum number of movie theaters required to schedule all movies?
Calculate the minimum number of movie theatres needed based on start times and durations of movies.
Sort the movies by their start times.
Use a priority queue to track end times of movies currently being shown.
For each movie, check if it can fit in an existing theatre or if a new one is needed.
Example: Start times [1, 2, 3] and durations [2, 2, 2] require 2 theatres.
Computer Scientist Jobs
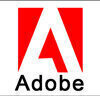
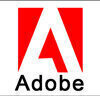
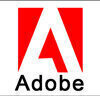
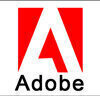
Asked in Adobe

Q. How would you complete a React application with the given functionalities?
The react application needs to be completed with given functionalities.
Implement state management using Redux or Context API
Create components for different sections of the application
Fetch data from an API and display it in the application
Add routing using React Router for navigation
Implement form handling for user input
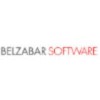
Asked in Belzabar Software Design

Q. Implement Breadth-First Search (BFS) in a graph.
BFS (Breadth-First Search) is a graph traversal algorithm that explores all the vertices of a graph in breadth-first order.
Create a queue to store the visited vertices
Start with the initial vertex and enqueue it
While the queue is not empty, dequeue a vertex and mark it as visited
Enqueue all the adjacent vertices of the dequeued vertex that are not yet visited
Repeat until the queue is empty
Share interview questions and help millions of jobseekers 🌟
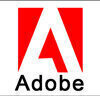
Asked in Adobe

Q. Find left view of binary tree. Two sum problem
Left view of a binary tree is the set of nodes visible when the tree is viewed from the left side.
Traverse the binary tree level by level using BFS or DFS.
Keep track of the first node encountered at each level (leftmost node).
Store the leftmost nodes in an array to get the left view of the binary tree.
Asked in Adobe Metal Products

Q. Describe the process of coding an auto-complete system.
An autocomplete system predicts user input based on a given prefix using a trie data structure.
Use a Trie (prefix tree) to store words for efficient prefix searching.
Insert words into the Trie, e.g., 'apple', 'app', 'application'.
Implement a search function that retrieves suggestions based on the prefix.
Return suggestions as an array of strings, e.g., ['apple', 'app', 'application'] for prefix 'app'.
Consider using a priority queue to rank suggestions based on frequency or rec...read more
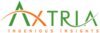
Asked in Axtria

Q. Explain the four pillars of OOP.
The four pillars of OOP are encapsulation, inheritance, polymorphism, and abstraction.
Encapsulation: Bundling of data and methods into a single unit (class) to hide implementation details.
Inheritance: Ability of a class to inherit properties and behaviors from another class.
Polymorphism: Ability of objects of different classes to be treated as objects of a common superclass.
Abstraction: Simplifying complex systems by breaking them down into smaller, more manageable units.
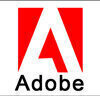
Asked in Adobe

Q. Given an array nums of n integers, are there elements a, b, c in nums such that a + b + c = 0? Find all the unique triplets in the array which gives the sum of zero. Notice that the solution set must not contai...
read moreThe Three Sum problem involves finding unique triplets in an array that sum to zero.
Use a sorted array to simplify the search for triplets.
Utilize a two-pointer technique to find pairs that complement a fixed element.
Example: For array [-1, 0, 1, 2, -1, -4], the triplets are [-1, -1, 2], [-1, 0, 1].
Avoid duplicates by skipping over repeated elements in the sorted array.
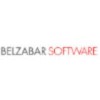
Asked in Belzabar Software Design

Q. Given an array of integers nums and an integer k, return the total number of continuous subarrays whose sum equals to k.
Find all continuous subarrays in an array that sum up to a given value K.
Use a hashmap to store the cumulative sum and its frequency.
Iterate through the array, updating the cumulative sum.
Check if (cumulative sum - K) exists in the hashmap.
If it exists, it indicates that a subarray summing to K has been found.
Example: For array [1, 1, 1] and K=2, the subarrays are [1, 1] (at indices 0-1 and 1-2).
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
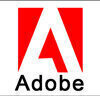
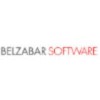

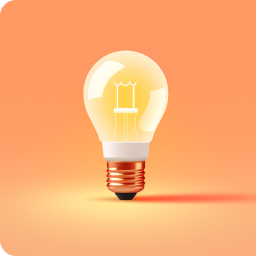
Calculate your in-hand salary
Confused about how your in-hand salary is calculated? Enter your annual salary (CTC) and get your in-hand salary
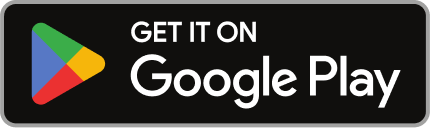
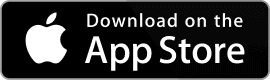
Reviews
Interviews
Salaries
Users
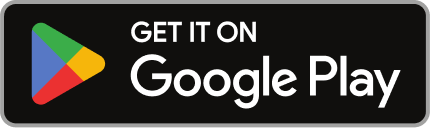
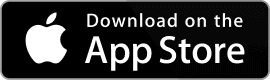